Need to work with XML data in your Dart projects? Dit artikel biedt een uitgebreide gids voor dart xml parsing and manipulation, covering essential libraries, common tasks, and best practices. You’ll learn how to efficiently handle XML data within your Dart applications and build robust, scalable solutions.
⚠️ Still Using Pen & Paper (Of een schoolbord)?! ⚠️
Stap in de toekomst! De Dart Teller -app behandelt alle scoren, stelt kassa voor, en volgt uw statistieken automatisch. It's easier than you think!
Probeer de Smart Dart Teller -app gratis!Klaar voor een upgrade? Klik hierboven!
Before diving into the specifics of dart xml processing, it’s crucial to understand the fundamental concepts involved. XML, or Extensible Markup Language, is a widely used markup language for encoding documents in a format that is both human-readable and machine-readable. Many applications, from configuration files to data exchange formats, leverage XML’s structured approach. The ability to effectively parse and manipulate this data is a key skill for any Dart developer.
This guide covers everything from setting up your development environment and selecting the right libraries to handling complex XML structures and troubleshooting common issues. Whether you are a beginner or an experienced Dart programmer, you will find valuable insights and actionable advice within. We’ll explore best practices for writing efficient and maintainable code when working with dart xml.
Getting Started with Dart XML Parsing
The first step in working with dart xml is choosing the right library. Several excellent packages are available on pub.dev, each offering unique features and capabilities. A popular choice is the `xml` package, which provides a straightforward and efficient way to parse XML documents. Once you’ve chosen a library, the process typically involves these key steps:
- Add the package dependency: Include the selected package in your
pubspec.yaml
file. - Import the library: Include the necessary import statement in your Dart code.
- Parse the XML data: Use the library’s functions to parse your XML data, whether it’s from a file, a string, or a network request.
- Access and manipulate the data: Navigate the parsed XML structure to access the elements and attributes you need. Modify or extract the required information as needed for your application.
Remember to carefully consider error handling and edge cases throughout this process. Efficient dart xml handling often involves robust error checking to ensure that your application handles unexpected input gracefully. Bijvoorbeeld, consider what happens if the XML document is malformed or missing crucial data.
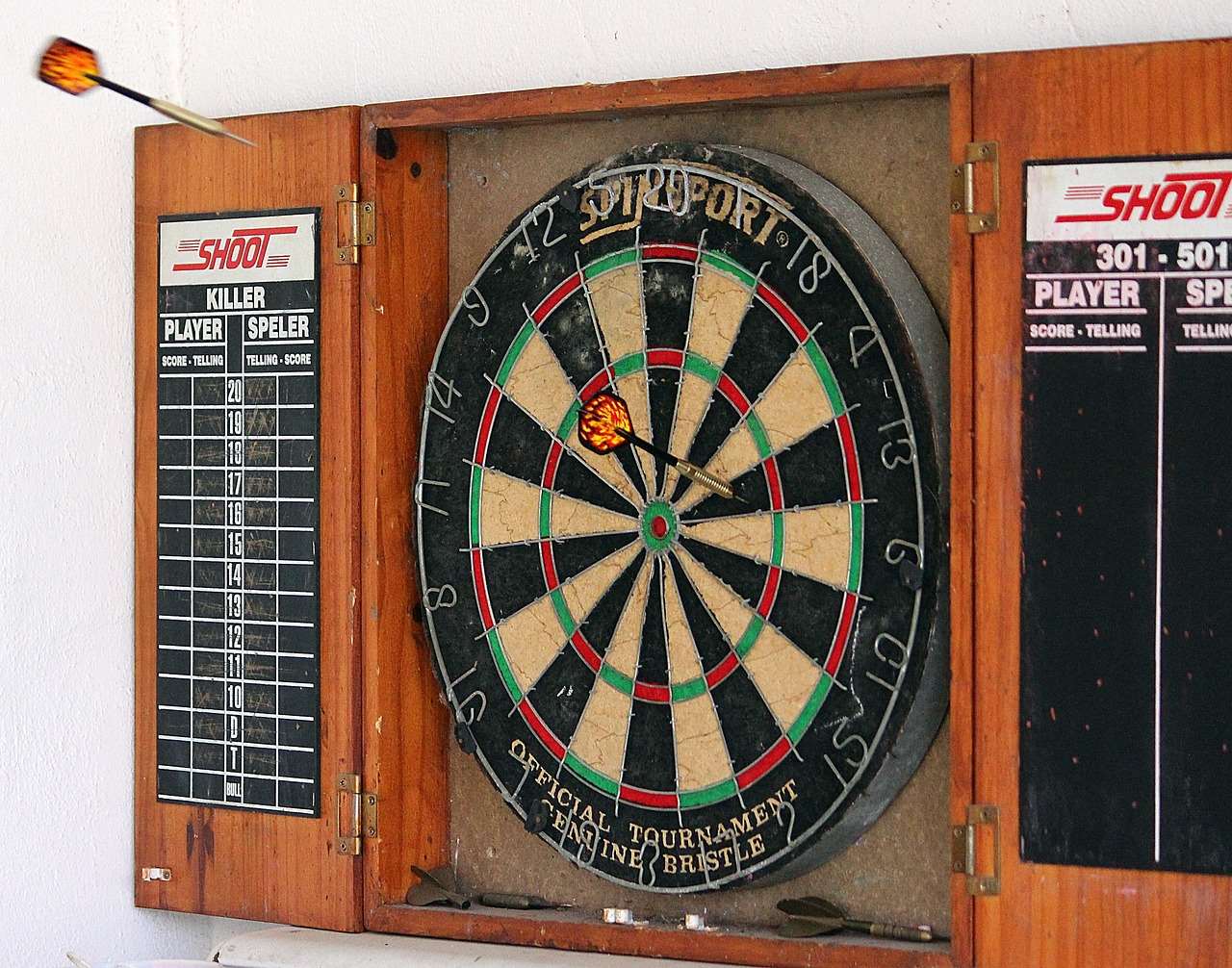
Common Dart XML Tasks
Parsing XML from a File
Reading XML from a file is a frequent task. Here’s a basic example using the xml
package:
import 'dart:io';
import 'package:xml/xml.dart';
Future main() async {
final file = File('path/to/your/file.xml');
final xmlString = await file.readAsString();
final document = XmlDocument.parse(xmlString);
// Access elements and attributes
final root = document.rootElement;
print(root.findAllElements('elementName').map((e) => e.text).toList());
}
Remember to replace 'path/to/your/file.xml'
with the actual path to your XML file. This example showcases how to read, parse, and access specific data from within the XML file.
Parsing XML from a String
If your XML data comes as a string, the process is similar. Instead of reading from a file, you simply pass the string directly to the XmlDocument.parse()
methode. This is particularly useful when dealing with XML received from an API or other data source.
Creating XML Documents
Sometimes you’ll need to generate XML from scratch. The `xml` package makes this straightforward with its powerful methods for creating and manipulating XML structures. Mastering this is key for building dart xml applications.
Efficient dart xml handling also includes understanding how to create well-formed XML documents that are easy to parse and use in other contexts. Validating your XML output is often a good practice.
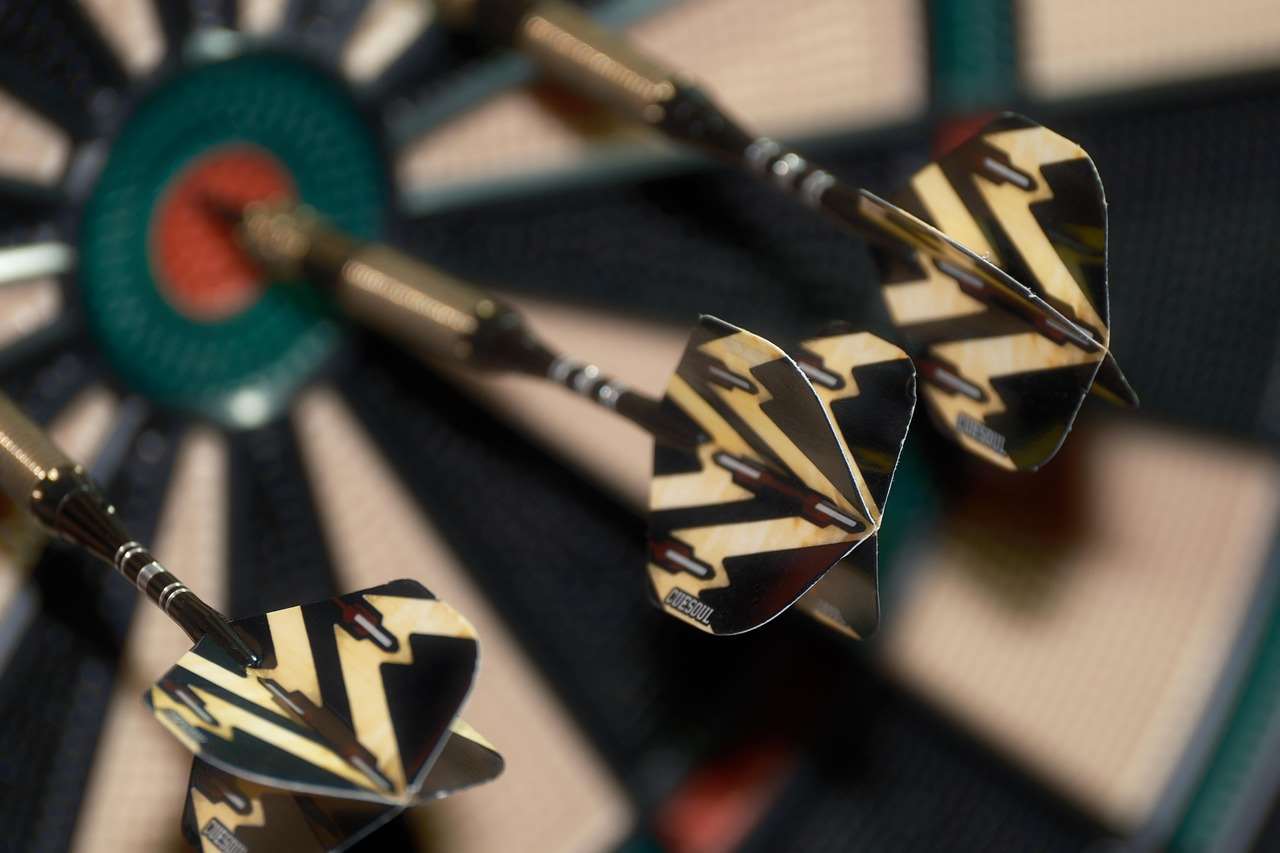
Advanced Dart XML Techniques
Handling Namespaces
Many XML documents use namespaces to avoid naming conflicts. De xml
package provides tools to handle namespaces efficiently. Understanding how to correctly handle namespaces is vital when working with complex XML structures in your dart xml projects.
Working with Large XML Files
Processing large XML files efficiently requires specific strategies. Techniques like streaming parsers can be essential to avoid memory issues. Understanding these techniques is a key element of building robust dart xml applications. Consider using iterative parsing methods instead of loading the entire document into memory at once.
Error Handling and Validation
Implementing thorough error handling is crucial for building reliable applications. Handle potential exceptions during parsing and use validation techniques to ensure data integrity. Robust error handling ensures your dart xml applications handle unexpected or invalid input gracefully.
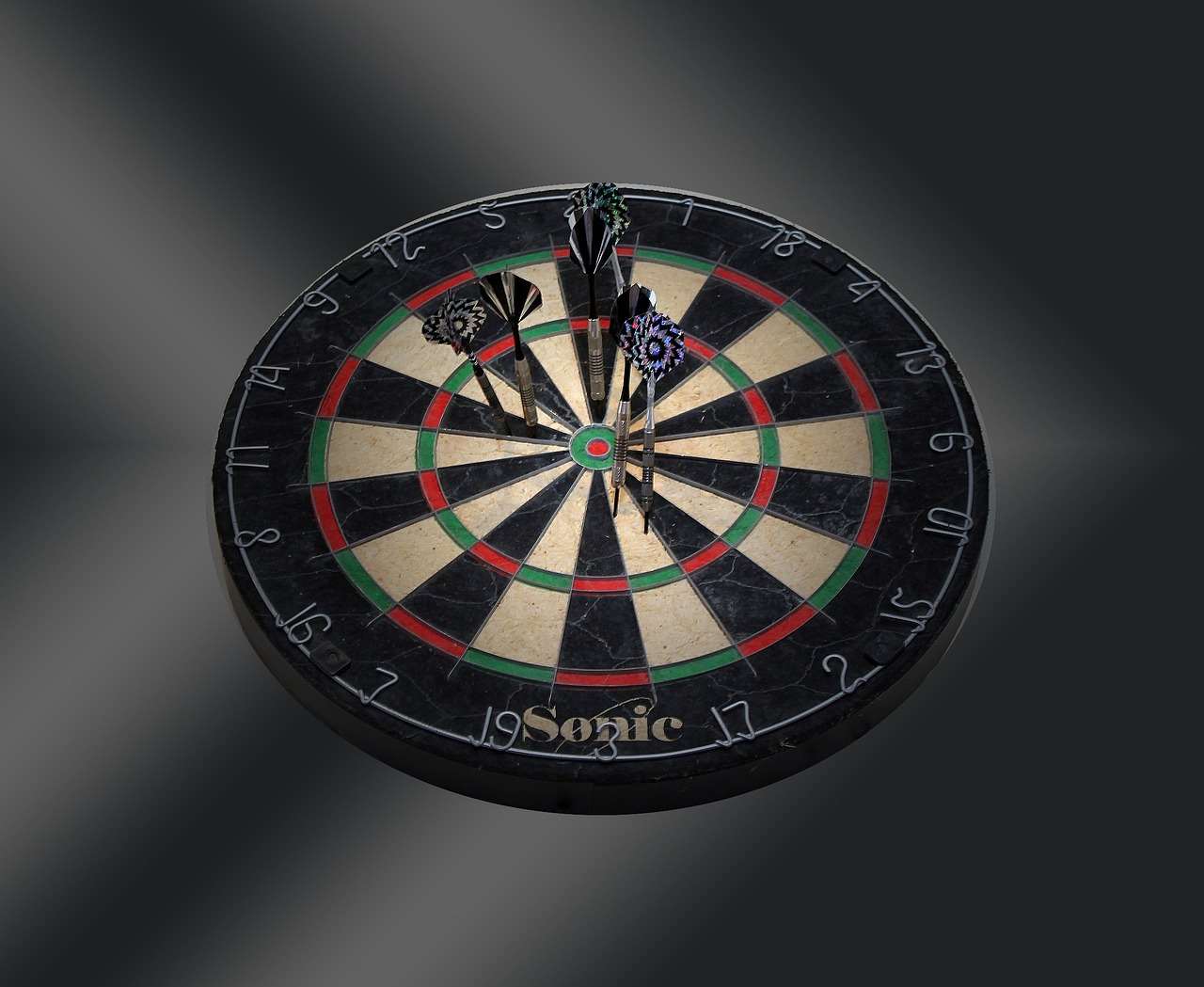
Choosing the Right Dart XML Library
The choice of library depends on your specific needs. While `xml` is a popular and robust option, other packages offer alternative functionalities. Researching and comparing different libraries before committing to one can save time and effort in the long run. Consider the performance characteristics of each library, especially when dealing with large XML files. Explore various libraries to discover the best fit for your project’s specific requirements and scale.
Remember to carefully review the documentation and examples provided for each library to ensure it aligns well with your project’s technical architecture. A well-documented library is often easier to integrate and maintain. This minimizes potential roadblocks as you incorporate dart xml processing into your applications.
Best Practices for Dart XML Development
- Use a well-maintained library: Choose a library with active development and a large community for support.
- Write clean, well-documented code: Make your code easy to understand and maintain.
- Implement thorough error handling: Handle potential exceptions gracefully.
- Validate your XML data: Ensure data integrity.
- Use efficient parsing techniques: Avoid loading large files entirely into memory.
Following these best practices will ensure your dart xml applications are robust, efficient, and maintainable over time. Good coding practices are vital for ensuring the long-term success and sustainability of your project.
Bijvoorbeeld, if you’re building a pro darts counter app, efficient dart xml handling can significantly impact performance, especially if you are storing game data in XML format. Similarly, if you are working on a complex project that involves large datasets, choosing the right library and implementing efficient parsing techniques will be crucial to avoid performance bottlenecks. Consider the implications of your choice when building for different scales of data.
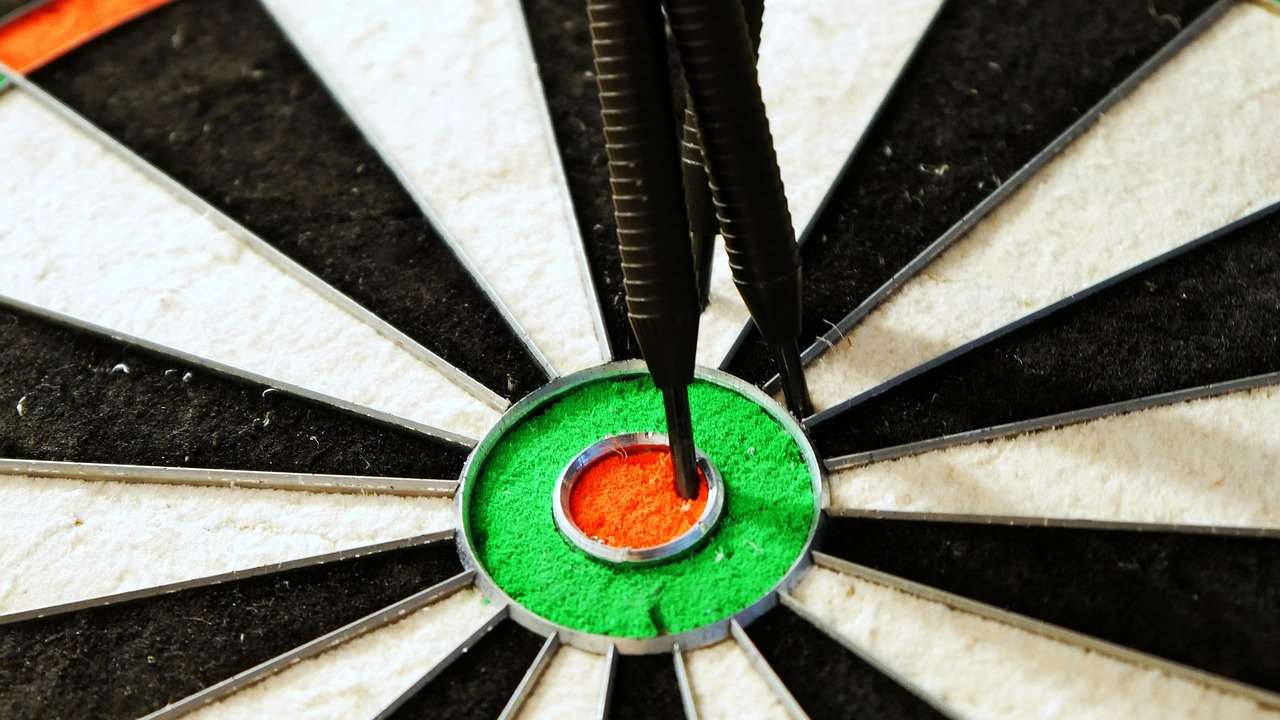
Troubleshooting Common Dart XML Issues
Here are some common problems you might encounter when working with dart xml and potential solutions:
- Parsing errors: Double-check your XML for well-formedness. Use a validator to identify errors.
- Namespace issues: Ensure your code correctly handles namespaces.
- Memory issues with large files: Use streaming parsers to avoid loading entire files into memory.
- Unexpected data: Implement robust error handling to gracefully manage unexpected data formats.
Herinneren, efficient dart xml processing often involves anticipating potential issues and having strategies in place to address them. Thorough testing and debugging are critical aspects of building reliable applications.
Bijvoorbeeld, if you’re working with darts points explained in your application, you may need to handle different data formats, which requires robust error handling to ensure your app can interpret and process various input formats correctly. Likewise, when dealing with darts flights and shafts data, you would want to ensure your parsing is accurate.
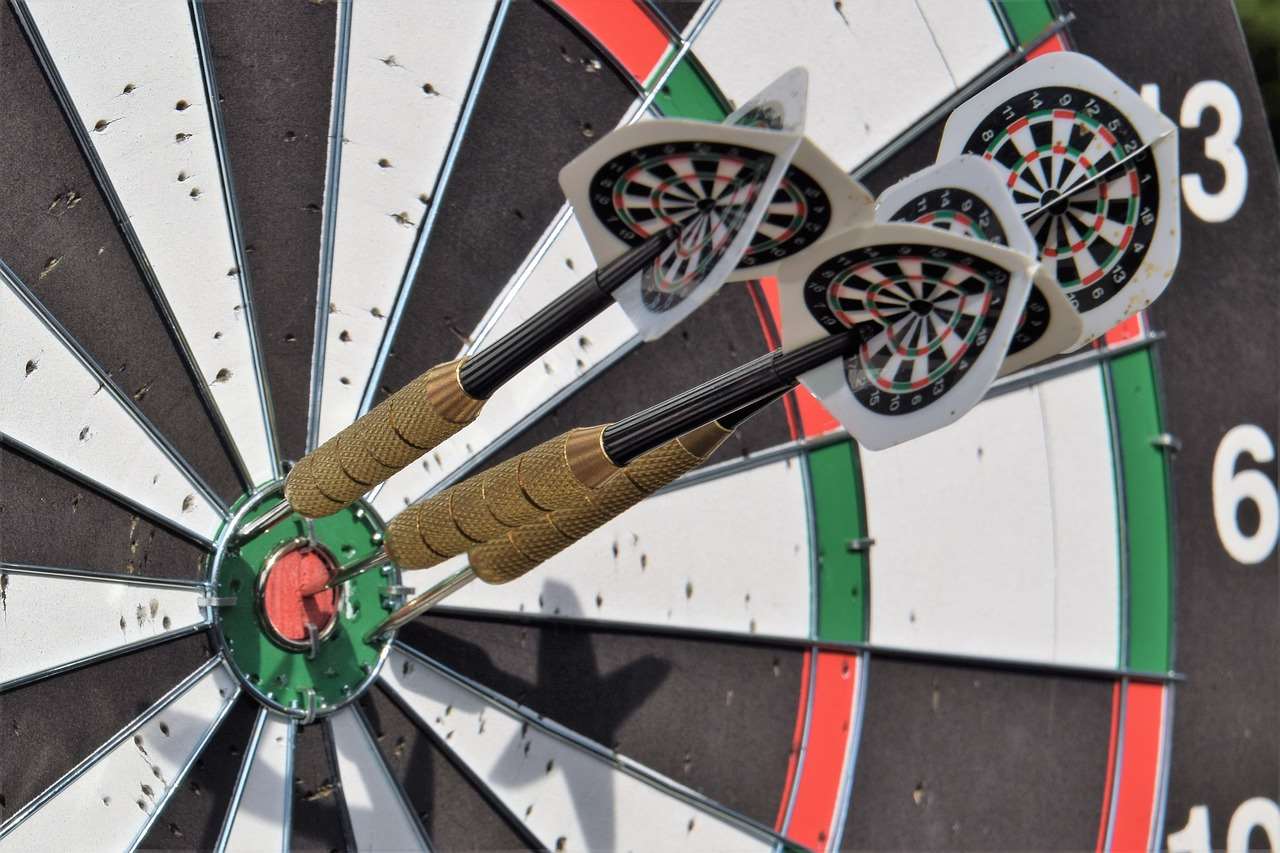
Conclusie
Working with dart xml is a fundamental skill for Dart developers. This article has covered the essential techniques and best practices for handling XML data effectively. By choosing the right library, implementing robust error handling, and following best practices, you can ensure your applications handle XML data efficiently and reliably. Herinneren, efficient and clean code is crucial for maintainability and scalability in your projects. Utilize these strategies to streamline your workflow and achieve optimal performance.
To further enhance your Dart development skills, consider exploring other related topics such as working with JSON data or integrating with various APIs. Remember to always check the latest documentation and updates for the libraries you use to stay current with any changes or improvements.
Ready to start building your own dart xml application? Check out the Dart Teller -app for more inspiration and practical examples. Happy coding!
Hoi, Ik ben Dieter, En ik heb Dartcounter gemaakt (Dartcounterapp.com). Mijn motivatie was geen darts -expert - helemaal tegenovergestelde! Toen ik voor het eerst begon te spelen, Ik hield van het spel, maar vond het moeilijk en afleidend om nauwkeurige scores te houden en statistieken te volgen.
Ik dacht dat ik niet de enige kon zijn die hiermee worstelde. Dus, Ik besloot om een oplossing te bouwen: een eenvoudig te gebruiken applicatie die iedereen, Ongeacht hun ervaringsniveau, zou kunnen gebruiken om moeiteloos te scoren.
Mijn doel voor Dartcounter was eenvoudig: Laat de app de nummers afhandelen - het scoren, de gemiddelden, de statistieken, Zelfs checkout suggesties - zodat spelers puur kunnen richten op hun worp en genieten van het spel. Het begon als een manier om het probleem van mijn eigen beginners op te lossen, En ik ben heel blij dat het is uitgegroeid tot een nuttig hulpmiddel voor de bredere darts -community.