Understanding the nuances of a dart union in Dart programming is crucial for building robust and efficient applications. This article will explain what a dart union is, its benefits, and how to effectively use it in your projects. We’ll also explore related concepts and best practices.
⚠️ Still Using Pen & Paper (or a Chalkboard)?! ⚠️
Step into the future! The Dart Counter App handles all the scoring, suggests checkouts, and tracks your stats automatically. It's easier than you think!
Try the Smart Dart Counter App FREE!Ready for an upgrade? Click above!
A dart union, also known as a tagged union or discriminated union, allows you to represent a value that can be one of several different types. This is incredibly useful when dealing with situations where a variable might hold different kinds of data depending on the context. Think about representing the result of an operation: it could be a successful result containing data, or an error indicating why it failed. A dart union elegantly handles such scenarios.
Imagine you’re building a system that processes user inputs. The input could be a number, a string, or even a boolean value. Using a traditional approach, you might resort to type checking and conditional logic. However, this can become complex and cumbersome, especially with many different input types. This is where the power of a dart union shines.
Understanding Dart Unions: A Deep Dive
At its core, a dart union is a type that can hold one of several distinct types. This is achieved using classes and enums to represent the different possible states or values. Each state typically holds its own associated data, leading to type-safe and efficient code. Unlike nullable types which indicate the possibility of a value being absent, a dart union defines that a value *must* be one of the explicitly defined states. This leads to improved clarity and reduces runtime errors caused by unexpected types.
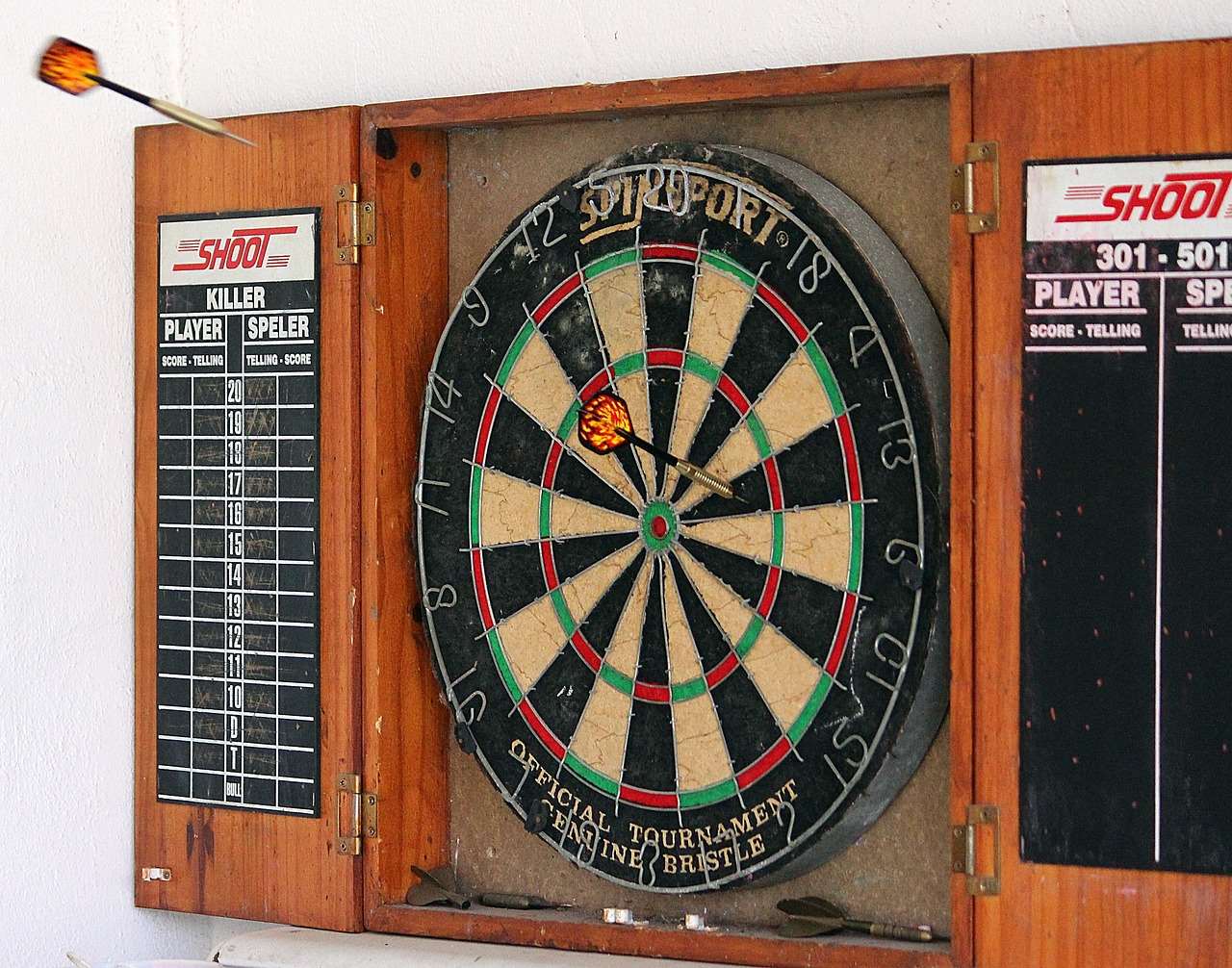
Let’s consider a simple example. Suppose we want to model a user’s authentication status. It could be either LoggedIn
with a user ID, LoggedOut
, or Error
with an error message. A traditional approach would involve using a nullable type combined with several conditional checks to manage this. By contrast, using a dart union provides a more readable and safer solution. Using enums to define the different states makes the code much more concise and manageable.
Implementing a Dart Union
There are several ways to implement a dart union in Dart. One common approach involves using sealed classes. A sealed class is a class that is declared with the sealed
keyword. This means that only the classes declared within its scope can extend or implement it. This ensures type safety and eliminates the possibility of unexpected types being introduced into your dart union. You can then define subclasses for each possible state, each with its associated data.
Another approach involves using enums combined with data classes. This is particularly useful if the data associated with each state is relatively simple. However, for more complex scenarios, sealed classes offer better structure and maintainability. Remember to leverage features like code generation tools to reduce boilerplate code when working with dart union implementations.
Benefits of Using Dart Unions
The advantages of incorporating dart unions into your Dart projects are substantial. First and foremost, they significantly improve code readability and maintainability. By explicitly defining all possible states, you eliminate ambiguity and make your code easier to understand and reason about. This reduces potential errors stemming from unexpected data types.
Secondly, dart unions enhance type safety. The compiler enforces that only valid states are used, preventing runtime errors caused by attempting to access data in an incorrect state. This leads to more robust and reliable applications. Using dart union ensures that you only access relevant data for each state and enhances error detection at compile time.
Finally, dart unions contribute to better code organization. They promote a more structured and modular approach to your code, making it easier to manage and scale your project. This simplifies debugging and significantly reduces technical debt.
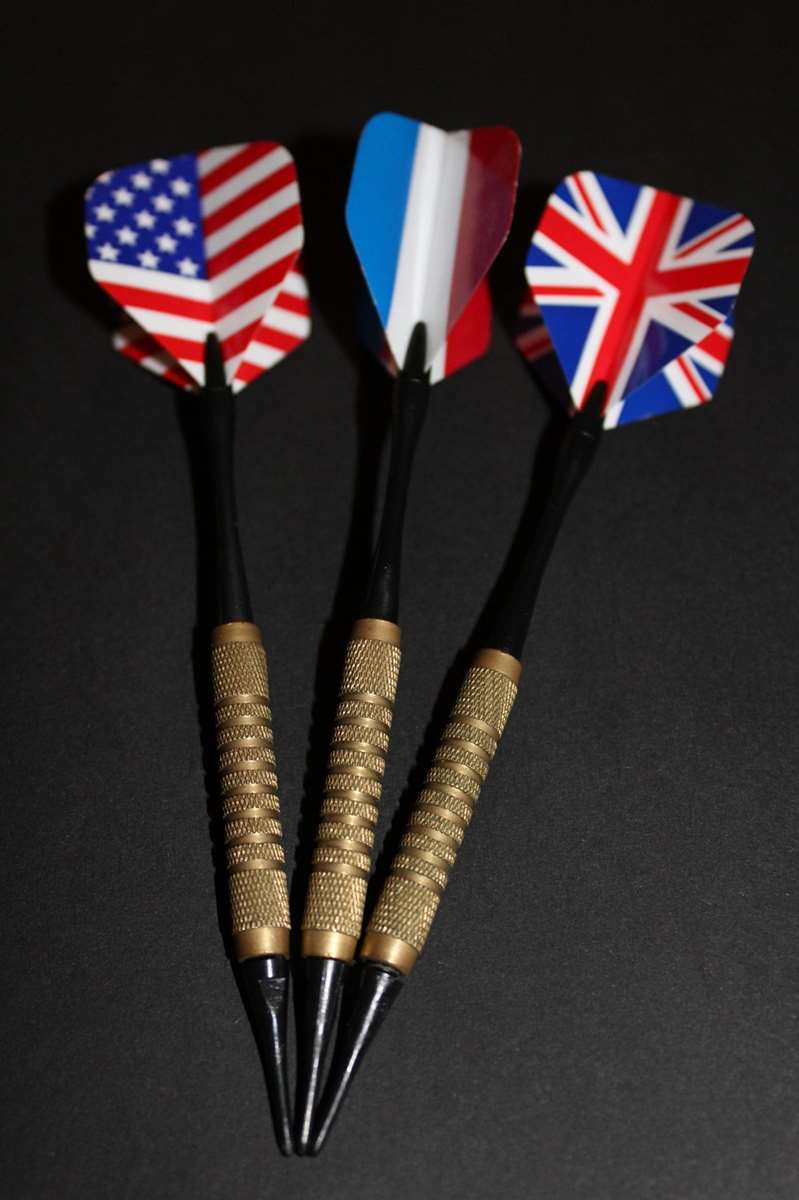
Practical Application and Examples
Let’s explore a practical scenario where a dart union is beneficial: handling asynchronous operations. Consider a function that fetches data from a remote server. The result could be either a successful response with the data, or an error indicating a failure. Using a dart union, you can define a type that represents both outcomes:
sealed class Result<T> {
const Result._(); // Private constructor
const factory Result.success(T data) = Success<T>;
const factory Result.error(String message) = Error;
}
class Success<T> extends Result<T> {
final T data;
const Success(this.data) : super._();
}
class Error extends Result {
final String message;
const Error(this.message) : super._();
}
This code defines a sealed class Result
with two subclasses: Success
and Error
. The Success
subclass holds the fetched data, while the Error
subclass holds an error message. This type-safe approach prevents runtime errors related to unexpected data.
To further illustrate the versatility of dart unions, consider a scenario involving user profiles. A user profile might contain various data points such as personal details, contact information, preferences, or even social media links. Using a dart union, you can model these different optional pieces of information without the need for complex null checks. The dart union ensures that the data structures are handled safely and efficiently.
Advanced Techniques and Considerations
While the basic implementation is relatively straightforward, more advanced techniques can enhance the usage of dart unions in complex applications. For instance, leveraging extension methods can streamline pattern matching and enhance readability. Additionally, integrating dart unions with other advanced Dart features like generics and streams can further improve the flexibility and efficiency of your code. Remember to explore and utilize these advanced techniques when tackling more sophisticated projects.
Furthermore, consider using tools that automate parts of the dart union creation and management. Code generation can help maintain consistency, reduce boilerplate, and streamline the development process. This also helps enhance maintainability and reduces the risk of errors. By integrating code generation into your workflow, you are optimizing the entire development lifecycle related to dart unions within your project.
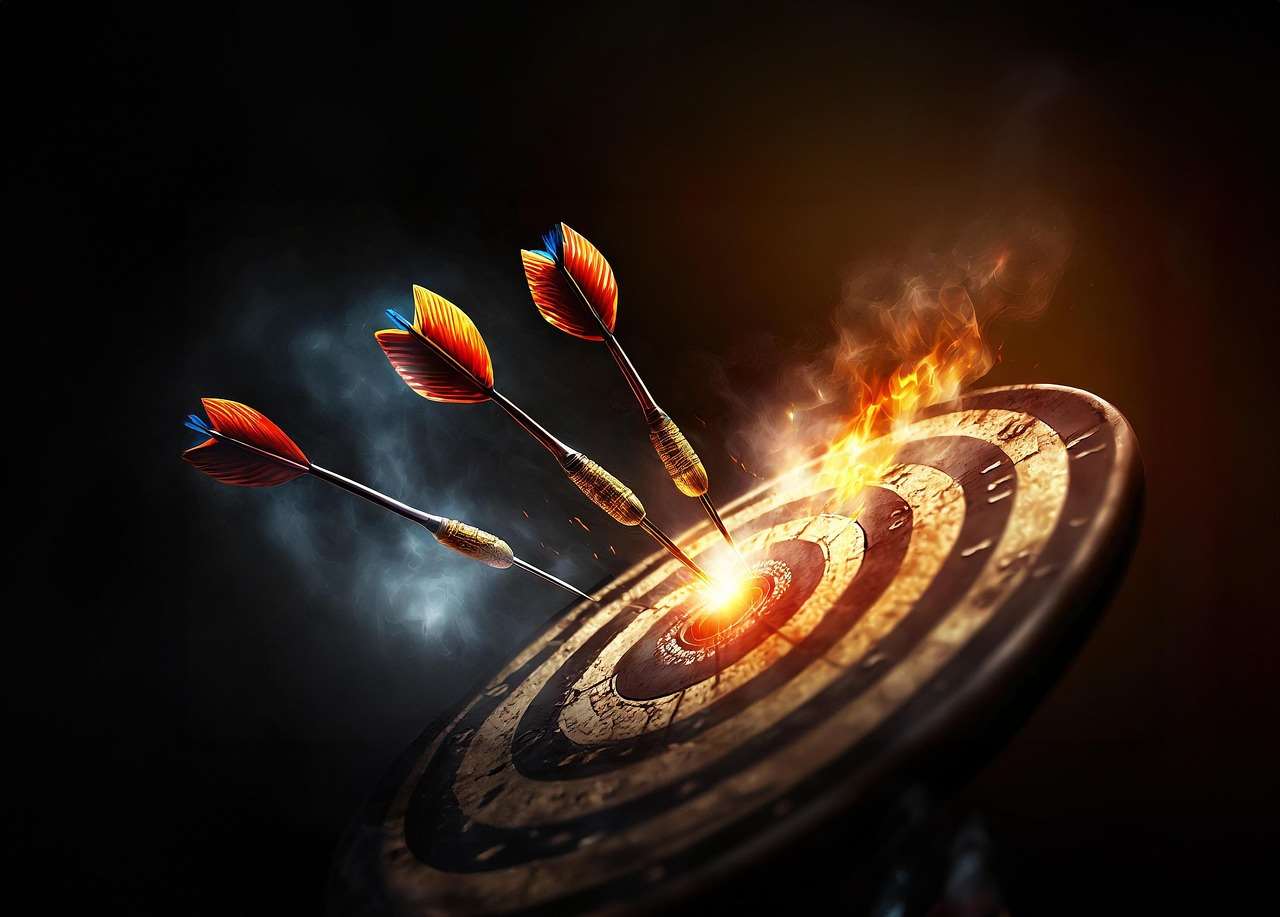
Choosing the Right Approach: Unions vs. Other Options
Before adopting dart unions, it’s important to weigh the benefits against alternative approaches. In situations where only a few simple data types are involved, simpler null-checking mechanisms may suffice. However, for complex scenarios with numerous possible states or significant data associated with each state, a dart union offers a vastly superior solution. The type safety and clarity they provide far outweigh the initial implementation effort.
Consider the scalability of your project. If your application is likely to expand and grow, the maintainability and robustness offered by a dart union becomes critical. A well-structured dart union contributes to a more robust and resilient application that is easier to maintain and scale over time.
Troubleshooting and Common Pitfalls
While dart unions offer many advantages, some common pitfalls should be addressed. One potential issue is over-engineering. Avoid creating overly complex dart unions for situations that can be more simply handled. Always strive for a balance between structure and simplicity.
Another area to watch out for is excessive nesting of dart unions. If you find yourself creating deeply nested structures, it might indicate a need for a redesign or a different approach to your data modeling. Refactoring to simplify overly complex structures improves maintainability and reduces the chances of runtime errors. This is where careful planning and design choices are crucial for effective implementation.
Remember to utilize efficient pattern matching techniques when working with dart unions. This makes the code more readable, easier to maintain, and reduces the possibility of errors. Using appropriate tools and techniques will lead to a well-organized and efficient codebase.
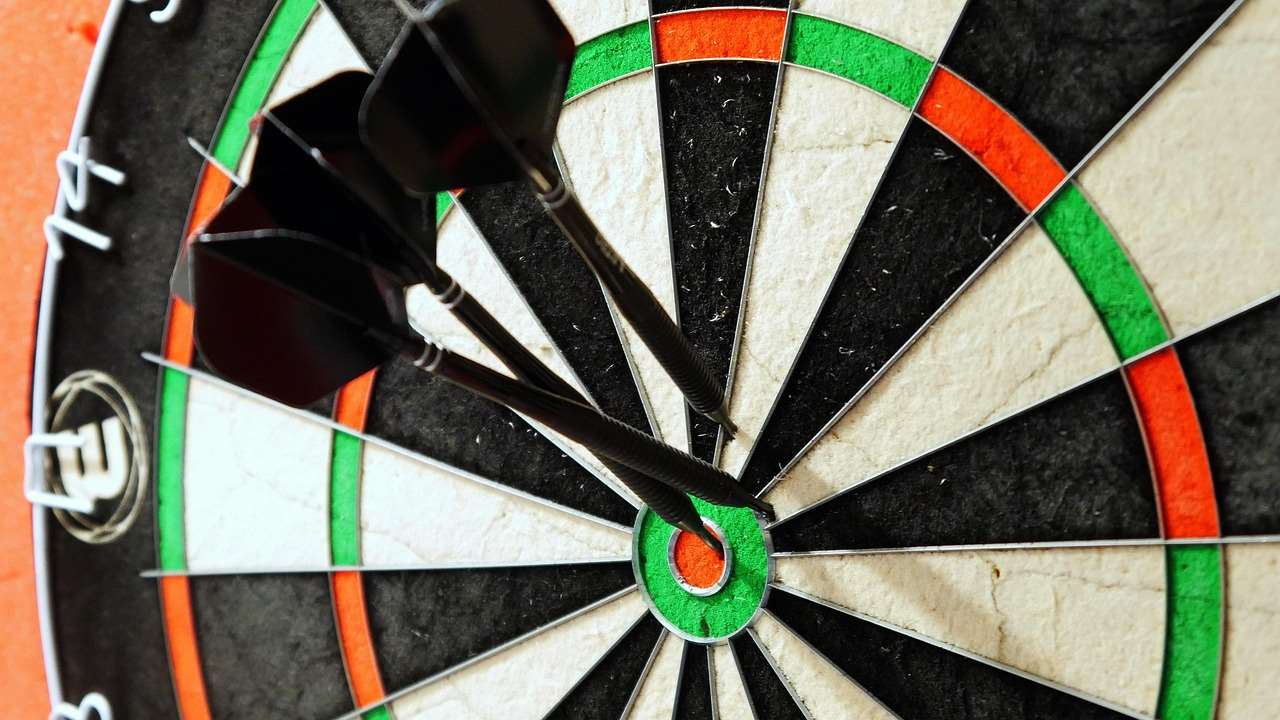
For beginners, start with simple examples and gradually increase complexity as your understanding grows. Experimenting with different approaches will help you learn the best practices for using dart unions in your projects.
Resources and Further Learning
To deepen your understanding of dart unions and their applications, consider exploring additional resources. The official Dart documentation provides comprehensive information on various aspects of Dart programming, including advanced concepts like dart unions and sealed classes. You can also find numerous online tutorials and articles that demonstrate practical examples and best practices.
Engaging with the Dart community through forums and online groups can provide valuable insights and solutions to challenges you might encounter. Many experienced developers are willing to share their knowledge and assist in troubleshooting. Don’t hesitate to ask questions and seek support from the community when needed. It’s a great way to learn and collaborate.
Remember to practice consistently. The best way to master dart unions is through hands-on experience. Start by building small applications, gradually increasing the complexity of your projects. This approach allows you to learn by doing and apply your knowledge in practical scenarios.
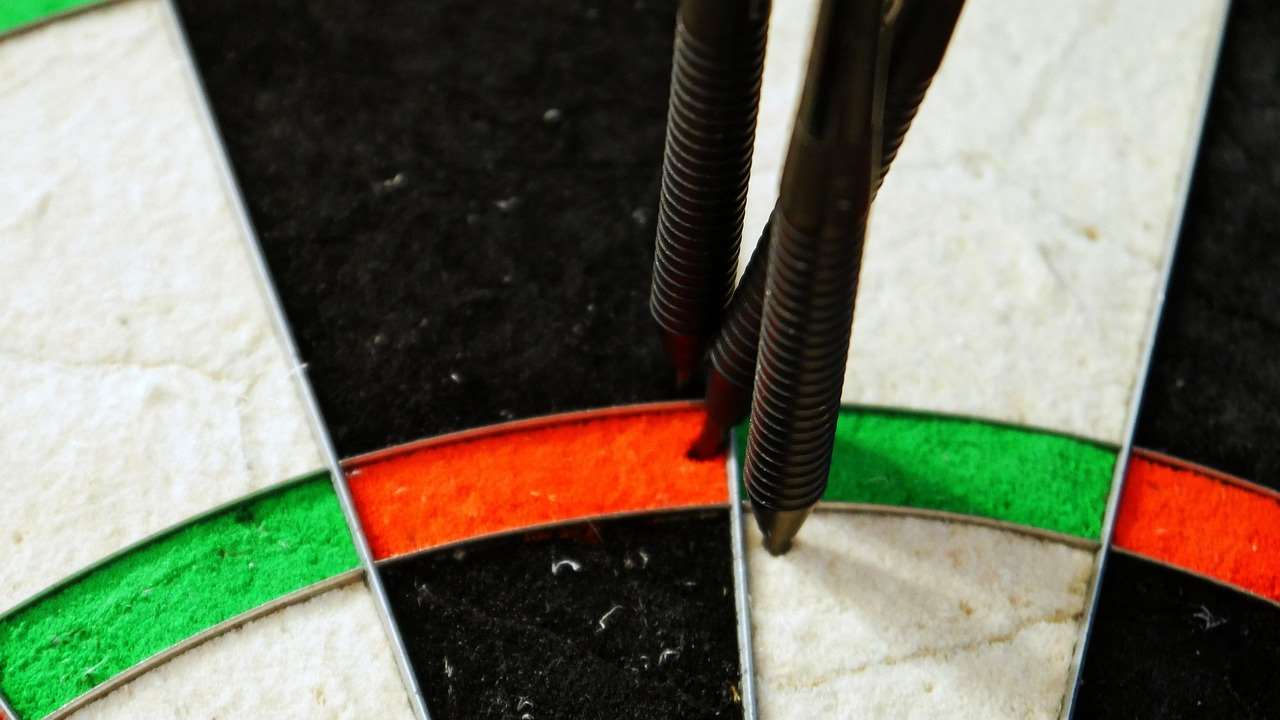
Conclusion
Incorporating dart unions into your Dart projects provides significant benefits in terms of code clarity, type safety, and maintainability. By understanding the fundamentals and implementing best practices, you can leverage the power of dart unions to build robust and efficient applications. Remember to explore advanced techniques, use appropriate tooling, and engage with the community to maximize your development efficiency and code quality. Start experimenting today and see the positive impact on your codebase!
Ready to enhance your Dart development skills? Check out our free dart score app for additional resources and practical learning opportunities! You can also explore other helpful resources such as gawlas darts, darts corner uk, dart ncaa wbk matches, or darts championship time tonight to further enrich your understanding of the topic.
Hi, I’m Dieter, and I created Dartcounter (Dartcounterapp.com). My motivation wasn’t being a darts expert – quite the opposite! When I first started playing, I loved the game but found keeping accurate scores and tracking stats difficult and distracting.
I figured I couldn’t be the only one struggling with this. So, I decided to build a solution: an easy-to-use application that everyone, no matter their experience level, could use to manage scoring effortlessly.
My goal for Dartcounter was simple: let the app handle the numbers – the scoring, the averages, the stats, even checkout suggestions – so players could focus purely on their throw and enjoying the game. It began as a way to solve my own beginner’s problem, and I’m thrilled it has grown into a helpful tool for the wider darts community.