Need a robust and efficient dart xml parser for your Flutter application? This article provides a comprehensive guide to choosing and implementing the best solution for your needs, covering various libraries, common challenges, and best practices. We’ll also explore error handling and optimization techniques.
⚠️ Still Using Pen & Paper (or a Chalkboard)?! ⚠️
Step into the future! The Dart Counter App handles all the scoring, suggests checkouts, and tracks your stats automatically. It's easier than you think!
Try the Smart Dart Counter App FREE!Ready for an upgrade? Click above!
XML parsing is a common task in many applications, and Dart, the language behind Flutter, offers several effective approaches. Understanding the nuances of different parsing methods can significantly impact your app’s performance and maintainability. This guide will help you navigate the world of dart xml parser libraries and techniques, empowering you to make informed decisions for your project.
Choosing the Right Dart XML Parser
The choice of dart xml parser depends heavily on your specific needs. Factors to consider include the size and complexity of your XML data, performance requirements, and the level of error handling needed. Some popular options include the `xml` package and custom solutions using the `dart:convert` library. Let’s explore these in more detail. The `xml` package, for example, provides a relatively straightforward approach to parsing XML in Dart. But keep in mind that there are scenarios where a custom implementation, particularly if dealing with very large or complex XML, can become superior.
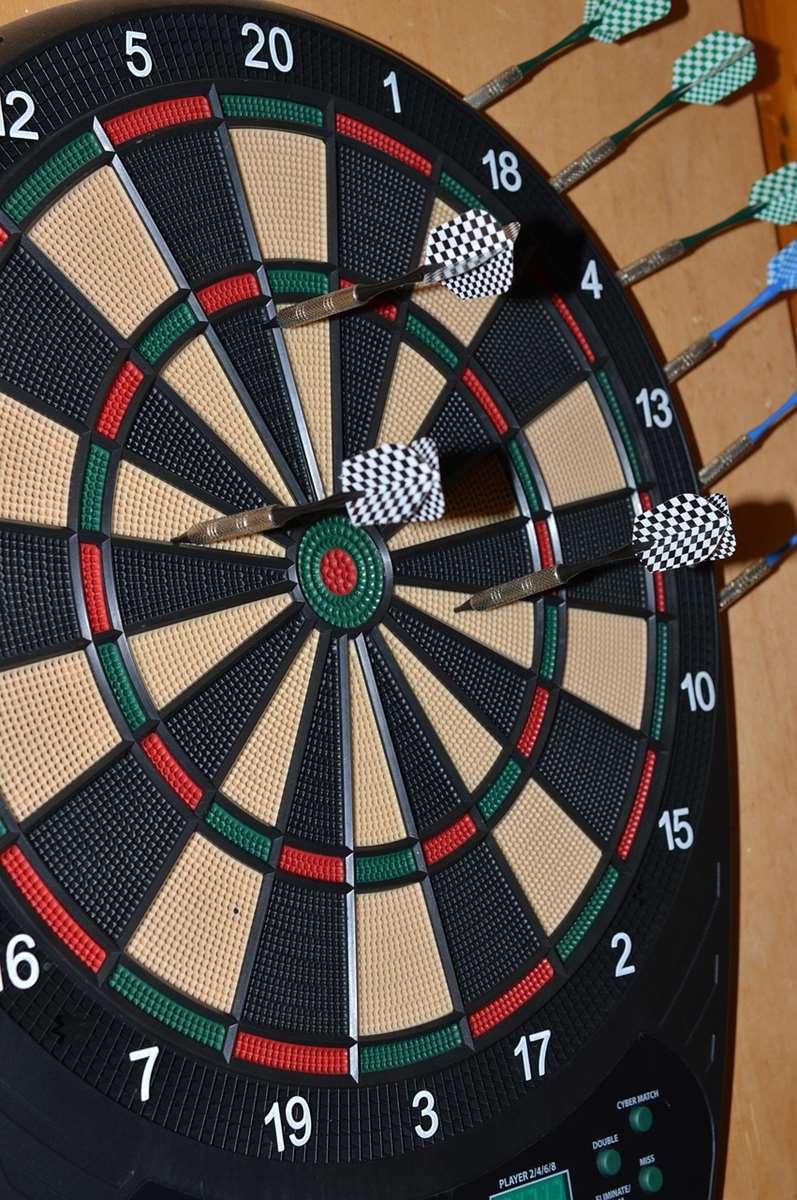
For smaller XML files, the built-in Dart capabilities are often sufficient. However, if your XML data is exceptionally large or complex, you might want to consider implementing more complex parsing techniques. This often involves utilizing a streaming parser to improve performance. Remember, a well-structured XML file is easier to handle than a poorly structured one. As such, validating your XML against a schema prior to parsing is a good practice.
The `xml` Package
The popular `xml` package is a widely used dart xml parser. Its ease of use and robust features make it a good starting point for many projects. It provides methods for parsing XML into a tree-like structure, making it easy to navigate and access specific elements and attributes. This package handles many edge cases, offering a solid foundation for your parsing needs. Before integrating this or any other package, be sure to always check for the latest updates and compatibility issues. It is best to rely on recently maintained and actively developed packages to minimize the possibility of unexpected bugs or security vulnerabilities.
Custom Parsing with `dart:convert`
For situations where you need more control or are working with very specific XML structures, you can create a custom dart xml parser leveraging the `dart:convert` library. This provides a lower-level approach, offering more flexibility but requiring more manual effort. Custom parsing, while offering the most control, is usually only the best solution if the `xml` package is completely inappropriate for your specific project needs. This approach will take more time to develop, but could offer superior efficiency if tailored expertly to your data structure. The initial time investment in a custom parser can often pay off in increased efficiency for large-scale projects.
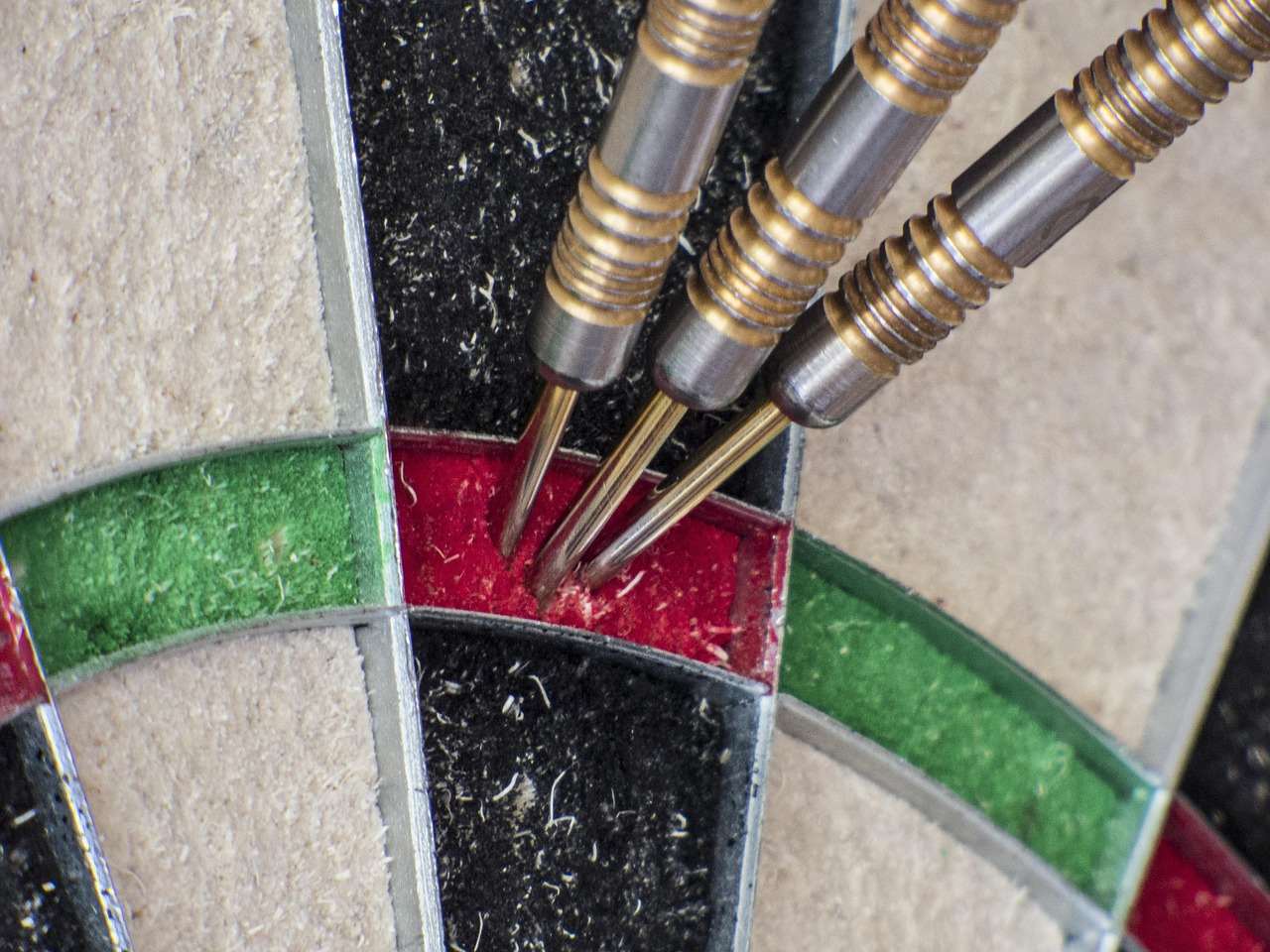
Handling Errors in XML Parsing
Robust error handling is crucial when working with XML. Unexpected data or malformed XML can easily lead to crashes if not handled properly. A well-designed dart xml parser anticipates potential issues and gracefully handles them. This includes catching exceptions, validating the XML structure against a schema, and implementing fallback mechanisms. This process helps guarantee the smooth operation of your application, preventing unexpected failures caused by invalid XML input.
Exception Handling
The use of `try-catch` blocks is essential for handling potential exceptions during parsing. The `xml` package provides its own mechanisms to help identify and manage any parsing errors that might occur. Custom parsing with `dart:convert` will require more manual error handling. You should be meticulously checking for errors at each step of your parsing to ensure that no potentially damaging situations are left unchecked. If you are working with large amounts of XML data, logging the errors and the data that caused the failure can also assist in debugging.
XML Validation
Validating your XML data against a schema before parsing is a powerful preventive measure. This ensures that the data conforms to the expected structure, reducing the chances of parsing errors. This step alone can save considerable time and effort in debugging and error correction. Ensuring the data’s validity beforehand makes the parsing process much smoother and more efficient.
Optimizing Your Dart XML Parser
For large XML files, optimization is essential to ensure responsiveness. Techniques include streaming parsers and efficient data structures. A dart xml parser optimized for larger datasets will be more performant. Consider asynchronous processing for significant improvements in UI responsiveness, especially in applications dealing with large amounts of XML data in real time.
Streaming Parsers
Unlike traditional parsers that load the entire XML document into memory, streaming parsers process the data incrementally. This drastically reduces memory consumption, improving performance for large files. Using streaming parsers allows for handling extremely large files without sacrificing the performance of your application.
Efficient Data Structures
Choosing the appropriate data structures to represent the parsed XML data can significantly impact performance. Consider using efficient data structures, such as maps or custom classes, to optimize data access and manipulation. The use of more efficient structures during the parsing and post-processing will increase the speed of the entire process.
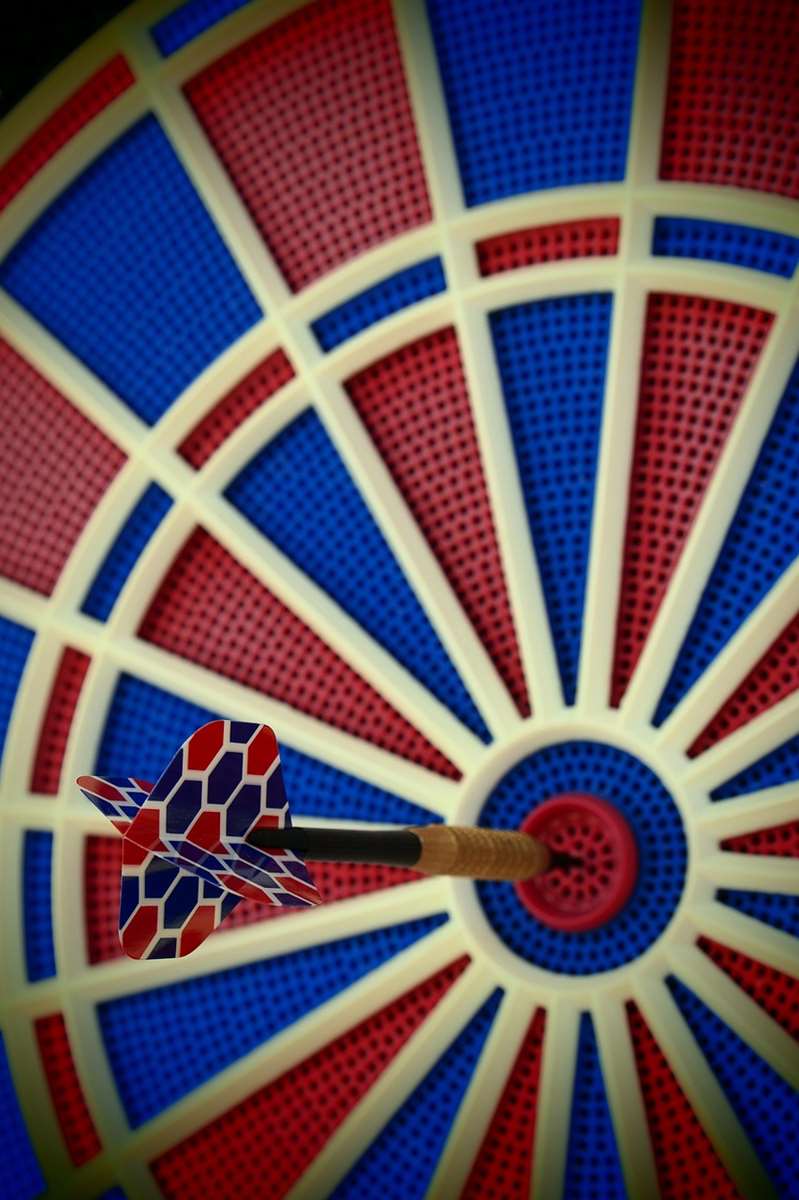
Advanced Techniques and Considerations
While basic parsing is often sufficient, more advanced techniques can enhance functionality and robustness. These include handling namespaces, CDATA sections, and integrating with other libraries for further processing.
Namespaces
Many XML documents use namespaces to avoid naming conflicts. A robust dart xml parser should properly handle namespaces during parsing to ensure accurate data retrieval. Understanding how namespaces are used in your XML data is key to properly implementing your parser.
CDATA Sections
CDATA sections allow you to include unescaped text within your XML. Your parser must be able to correctly interpret and handle these sections to avoid data corruption. Your dart xml parser should be able to identify and properly handle these CDATA sections to avoid incorrect parsing of the content within the section. Understanding and handling these special sections can avoid many common errors.
Integration with Other Libraries
Your dart xml parser might need to interact with other libraries for tasks like data transformation or serialization. This integration might involve using JSON serialization or other data processing tools to simplify your post-parsing operations. For example, you may want to convert the parsed XML data into a JSON format for easy integration with other services.
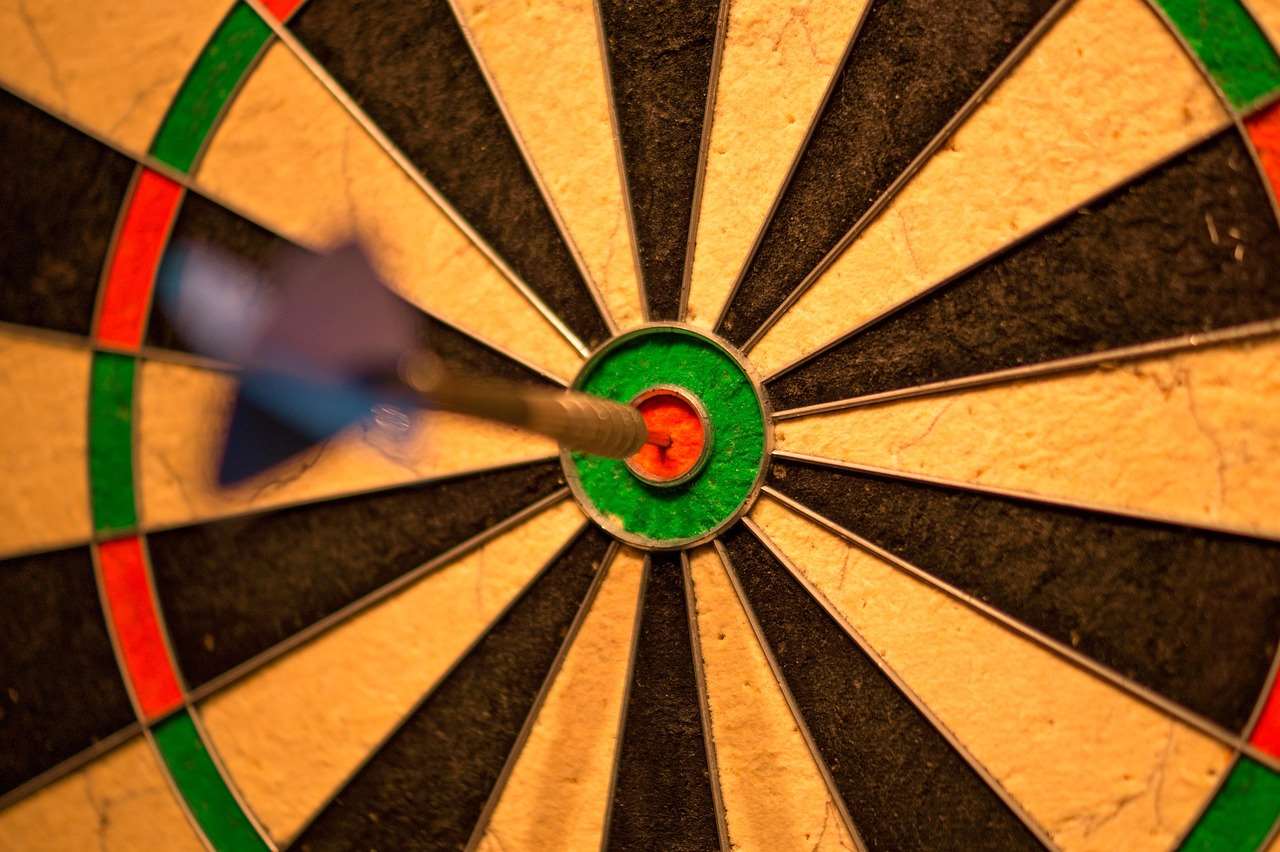
Practical Examples
Let’s look at a simple example using the `xml` package:
import 'package:xml/xml.dart';
void main() {
final xmlString = '''
Everyday Italian
Giada De Laurentiis
2005
30.00
''';
final document = XmlDocument.parse(xmlString);
final bookTitle = document.findAllElements('title').first.text;
print('Book Title: $bookTitle');
}
This code snippet demonstrates the basic usage of the `xml` package to parse a simple XML string and extract the book title. Remember to add the `xml` package to your `pubspec.yaml` file.
For more complex scenarios, you might explore using a streaming parser or a custom implementation, as previously discussed. When dealing with larger XML files, always consider the optimization techniques previously mentioned to ensure your application’s stability and performance.
Using the dart online editor can assist in testing your code snippets.
For those interested in the history of the language, check out the dart language release history.
Are you building a dartboard cabinet? Check out our guide on dartboard cabinet build.
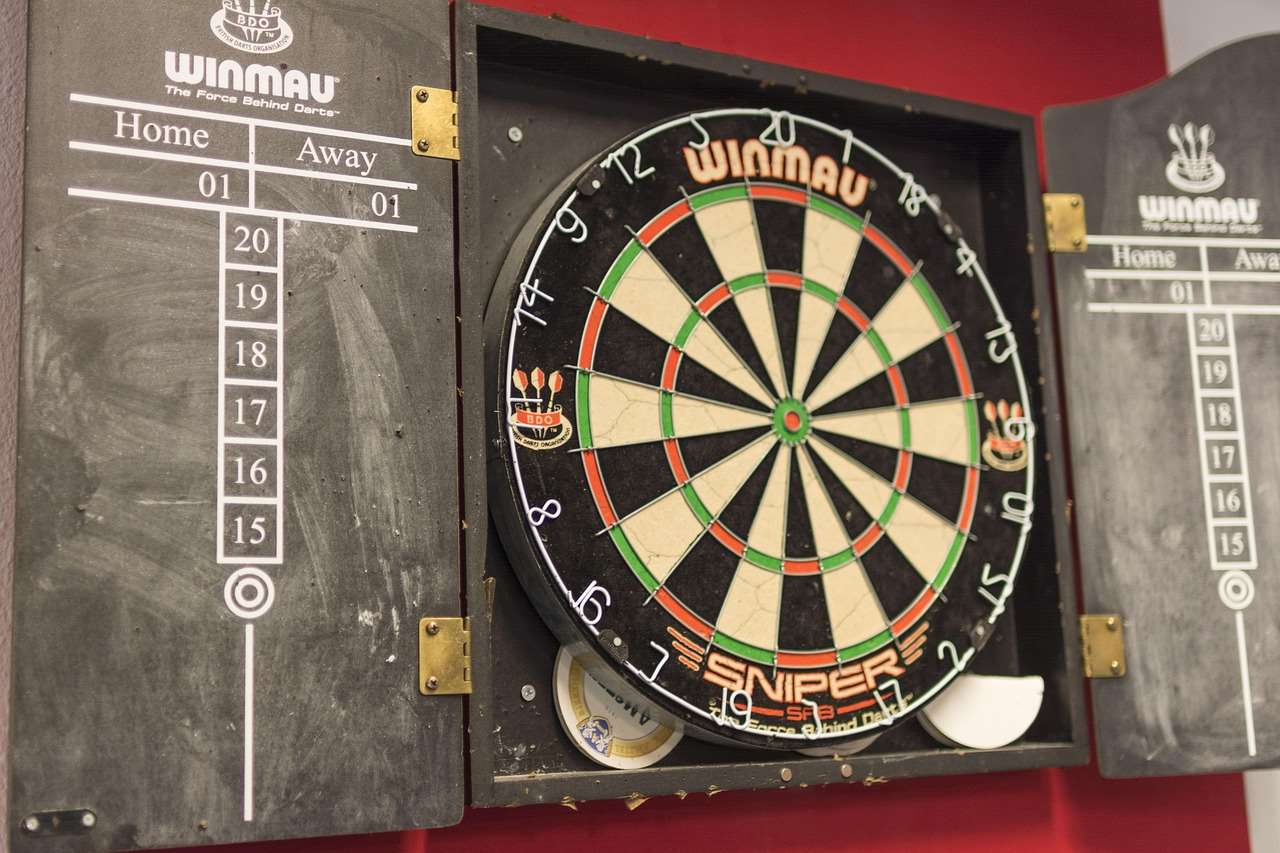
Conclusion
Choosing the right dart xml parser is crucial for building robust and efficient Flutter applications. This article has explored various options, including the popular `xml` package and custom solutions. We’ve also discussed essential aspects like error handling, optimization, and advanced techniques. By understanding these concepts and applying the best practices outlined here, you can successfully integrate XML parsing into your Dart projects. Remember to always consider the size and complexity of your XML data when selecting a parsing method. Regularly update your packages to benefit from bug fixes and performance improvements. Whether you’re a seasoned developer or just starting out, mastering XML parsing in Dart is a valuable skill.
Ready to elevate your Flutter application’s XML handling? Start exploring the options discussed above and choose the method best suited for your project’s unique requirements. Remember to always prioritize robust error handling and consider optimization techniques for larger datasets. Using a mobile dart scorer app like Mobile dart scorer can also enhance your dart-related applications.
Hi, I’m Dieter, and I created Dartcounter (Dartcounterapp.com). My motivation wasn’t being a darts expert – quite the opposite! When I first started playing, I loved the game but found keeping accurate scores and tracking stats difficult and distracting.
I figured I couldn’t be the only one struggling with this. So, I decided to build a solution: an easy-to-use application that everyone, no matter their experience level, could use to manage scoring effortlessly.
My goal for Dartcounter was simple: let the app handle the numbers – the scoring, the averages, the stats, even checkout suggestions – so players could focus purely on their throw and enjoying the game. It began as a way to solve my own beginner’s problem, and I’m thrilled it has grown into a helpful tool for the wider darts community.