Understanding dart with extends is crucial for building robust and maintainable Dart applications. This article will show you how to effectively leverage inheritance in Dart using the `extends` keyword, covering its core functionality and practical applications. We’ll also explore common use cases and best practices to help you write cleaner, more efficient code.
⚠️ Still Using Pen & Paper (or a Chalkboard)?! ⚠️
Step into the future! The Dart Counter App handles all the scoring, suggests checkouts, and tracks your stats automatically. It's easier than you think!
Try the Smart Dart Counter App FREE!Ready for an upgrade? Click above!
Inheritance is a powerful feature in object-oriented programming, and Dart’s implementation is straightforward and elegant. The extends
keyword allows you to create a new class (a subclass or child class) that inherits properties and methods from an existing class (a superclass or parent class). This promotes code reusability and reduces redundancy. Using dart with extends effectively is a fundamental skill for any Dart developer.
One of the primary benefits of using dart with extends is the ability to create specialized classes based on existing ones. Instead of writing the same code multiple times, you can create a base class with common functionality and then extend it to create more specific classes with additional features. This makes your code more organized, easier to maintain, and less prone to errors. For example, you might have a base Animal
class with properties like name
and age
, and then extend it to create specialized classes like Dog
and Cat
, each with their own unique characteristics. This demonstrates the power of dart with extends in practice. This approach is particularly useful in larger projects where code organization is critical.
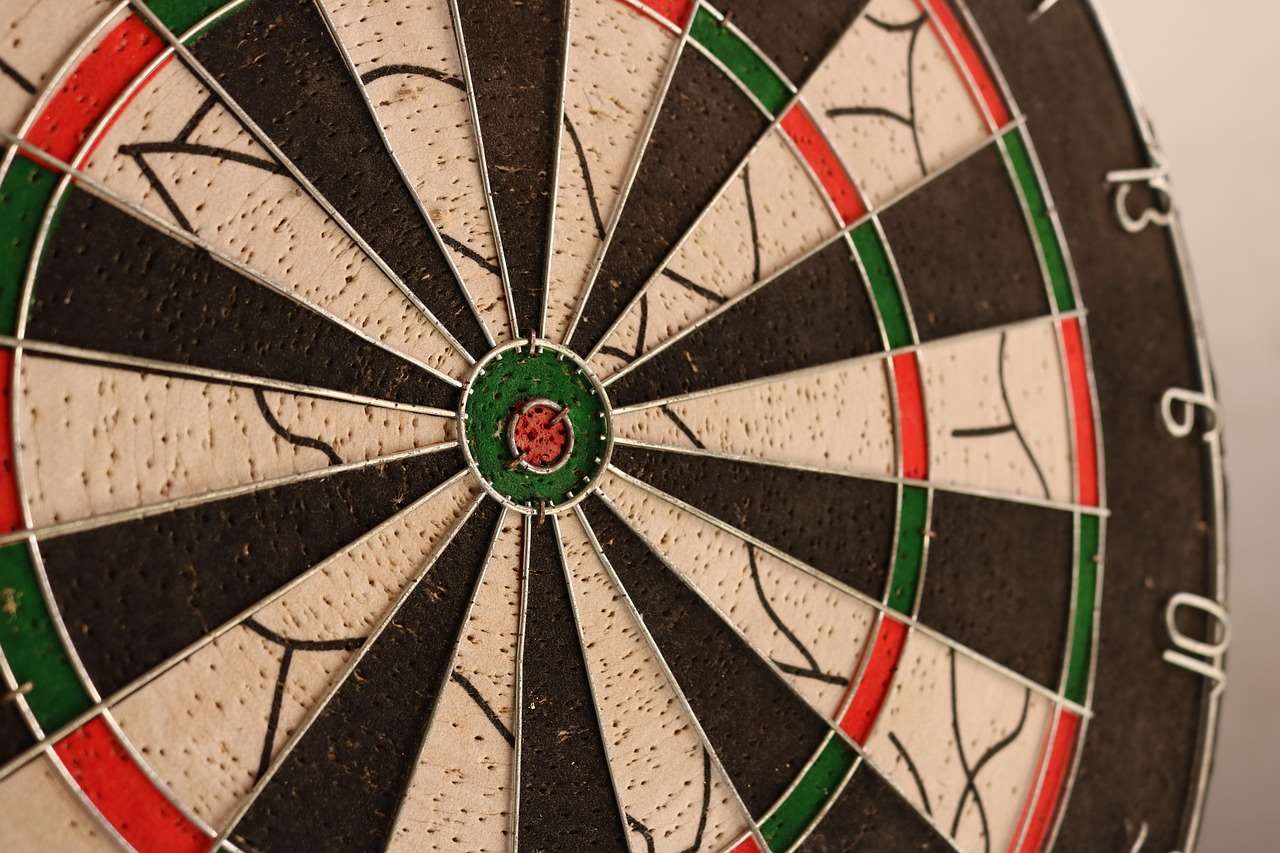
Understanding the Basics of Dart with Extends
Let’s delve into the syntax and mechanics of using dart with extends. The basic syntax is simple: class Subclass extends Superclass { ... }
. The Subclass
inherits all the public and protected members (fields and methods) of the Superclass
. This means that you can access and use these inherited members directly within your subclass without redefining them.
Constructor Usage with Extends
When working with dart with extends, you’ll often need to consider how constructors interact with inheritance. You can call the superclass’s constructor using the super()
keyword within the subclass’s constructor. This ensures that the superclass’s initialization logic is executed before the subclass’s initialization. The super()
keyword must be called before any other statements in the subclass constructor.
For example, if your superclass has a constructor that requires parameters, your subclass’s constructor must either provide those parameters to super()
or define its own parameters that are then passed to super()
. This ensures proper initialization of both the superclass and subclass.
Overriding Methods in Subclasses
One of the key features of inheritance is the ability to override methods. This allows you to provide a specialized implementation of a method that already exists in the superclass. By overriding, you’re essentially replacing the superclass’s implementation with your own. This is extremely valuable in situations where you need to modify the behavior of a method for a specific subclass.
Consider a scenario where you have a Vehicle
class with a move()
method. You might create subclasses like Car
and Bicycle
, each overriding the move()
method to reflect their specific movement characteristics. The Car
might implement move()
using an internal combustion engine, while the Bicycle
uses human power. This demonstrates the flexibility of dart with extends in tailoring behavior.
When overriding methods, remember that the subclass method should have the same signature (return type and parameters) as the superclass method. Failure to do so will result in a compile-time error. You can use the @override
annotation to explicitly indicate that you’re overriding a superclass method. This improves code readability and helps catch potential errors during development.
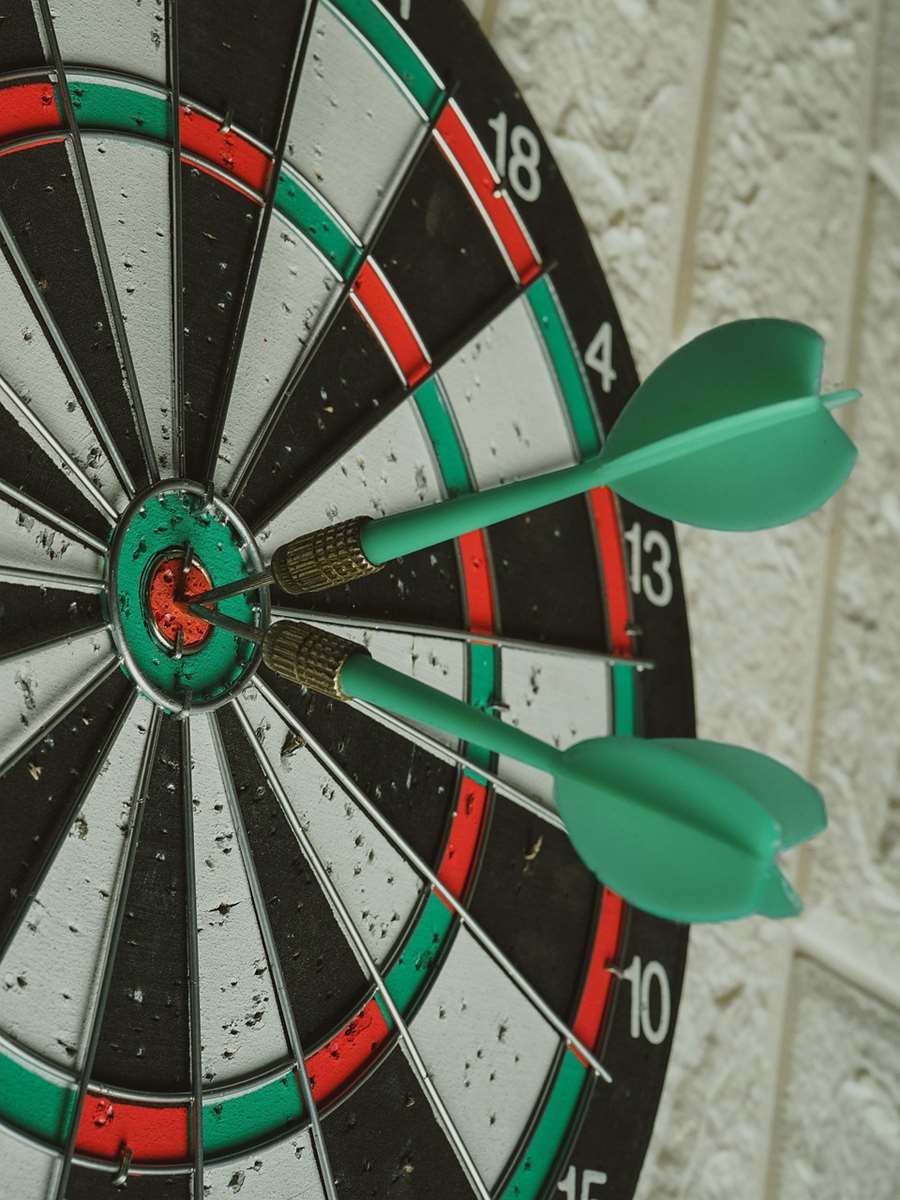
Advanced Concepts in Dart with Extends
Beyond the basics, there are several advanced concepts to consider when working with dart with extends in Dart. Understanding these concepts will allow you to write more sophisticated and efficient code.
Abstract Classes
An abstract class is a class that cannot be instantiated directly. It serves as a blueprint for other classes and is often used to define a common interface or set of methods that subclasses must implement. You can use abstract
keyword to define abstract classes and methods. Abstract classes are frequently used when you have a common interface but different implementations.
This allows for enforcing a consistent structure across related classes without requiring concrete implementations in the base class. Subclasses must provide concrete implementations for abstract methods, ensuring that the interface is fully realized in each derived class. This is a powerful tool for maintaining consistency and code quality.
Interfaces
While Dart doesn’t have explicit interface keywords like some other languages (e.g., Java’s `interface`), you can achieve similar functionality using abstract classes with only abstract methods. This effectively creates an interface that subclasses must adhere to. This is an essential pattern for implementing polymorphism, where different classes can respond to the same method call in their own specific ways. Consider using interfaces when you need to enforce a set of methods without needing concrete implementations in the abstract class.
Mixins
Mixins provide a mechanism to reuse code across multiple classes without using traditional inheritance. They are particularly useful for adding functionality to classes without creating complex inheritance hierarchies. A mixin is a class that can be ‘mixed in’ to another class, adding its members (methods and fields) to the host class. This can be more flexible than extending a class directly, especially when you want to combine capabilities from multiple sources without hierarchical constraints.
Mixins are a powerful tool to promote code reusability and reduce redundancy. They can be seen as a way of “plugging in” functionality to classes, enhancing them without the need for tight coupling through direct inheritance.
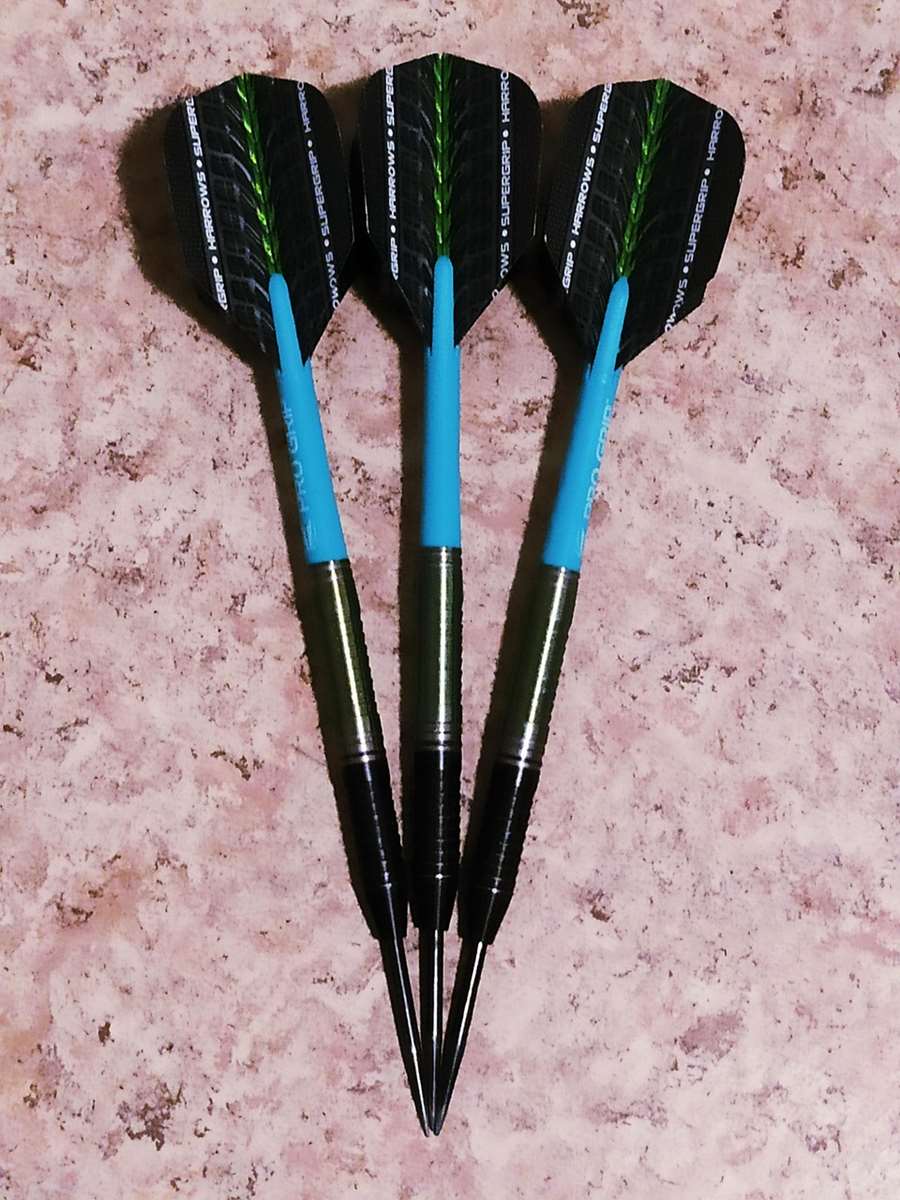
Practical Examples and Best Practices
Let’s explore some practical examples of using dart with extends and discuss best practices to ensure clean and maintainable code.
Example: Modeling Animals
Consider a scenario where you need to model different types of animals. You can create a base Animal
class with common properties (e.g., name
, age
) and methods (e.g., makeSound()
). Then, create subclasses like Dog
, Cat
, and Bird
, each extending the Animal
class and overriding the makeSound()
method to produce animal-specific sounds. This showcases a typical and highly effective use case for dart with extends.
Example: UI Components
In a UI framework like Flutter, you can leverage dart with extends to create custom widgets based on existing ones. For example, you could extend a standard Button
widget to create a custom ElevatedButton
with additional styling or functionality. This type of inheritance is a fundamental practice in Flutter development for building reusable UI elements.
Best Practices
- Keep inheritance hierarchies shallow: Deep inheritance trees can become difficult to manage and understand. Aim for a shallower hierarchy to improve code maintainability.
- Favor composition over inheritance: In some cases, composition (using objects of different classes within a class) can be a more flexible and maintainable approach than inheritance.
- Use abstract classes and mixins effectively: Leverage these features to enhance code reusability and structure.
- Document your inheritance structure: This helps others (and your future self) understand the relationships between classes.
Remember to choose the most suitable approach – inheritance, composition, or mixins – based on the specific requirements of your application and strive to maintain a clean and well-structured codebase. Effective use of dart with extends is a cornerstone of efficient Dart development.
To further enhance your Dart development skills, consider exploring resources like the official Dart documentation and online tutorials. Understanding dart with extends thoroughly will significantly improve your ability to create modular and reusable code in your projects. You can also check out some useful tools to assist you like Dart game scoring app for managing scores during your development testing.
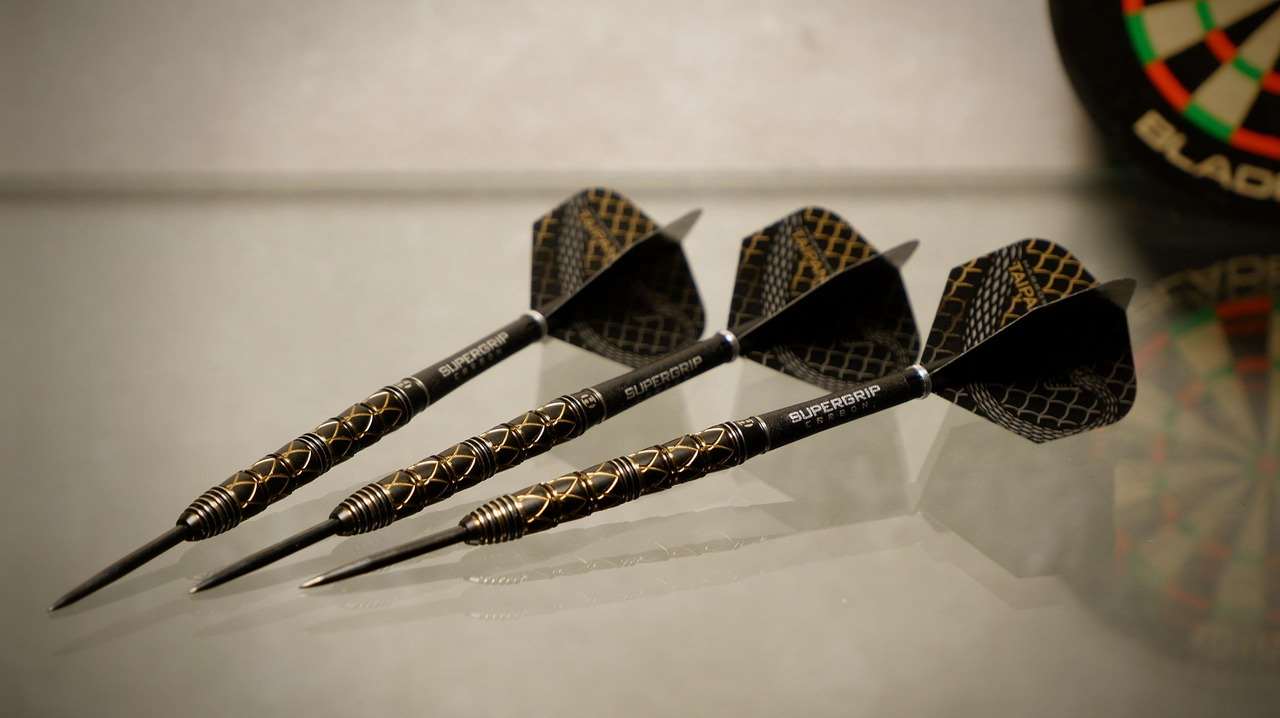
Troubleshooting Common Issues
While using dart with extends is generally straightforward, you may encounter certain issues. Let’s address some common problems and their solutions:
Compile-time Errors
Incorrectly overriding methods (mismatched signatures) or improper usage of super()
often lead to compile-time errors. Carefully review your code for any such inconsistencies. The compiler’s error messages are usually helpful in identifying the exact problem.
Runtime Errors
Issues might arise if you don’t properly initialize fields in your superclass or subclass constructors. Always ensure that all necessary fields are initialized before accessing them.
Code Complexity
Overusing inheritance or creating overly complex inheritance hierarchies can lead to hard-to-maintain code. Strive for simplicity and clarity in your class structures, favoring composition when appropriate.
Remember to thoroughly test your code after making changes involving inheritance. This helps catch potential errors early in the development cycle.
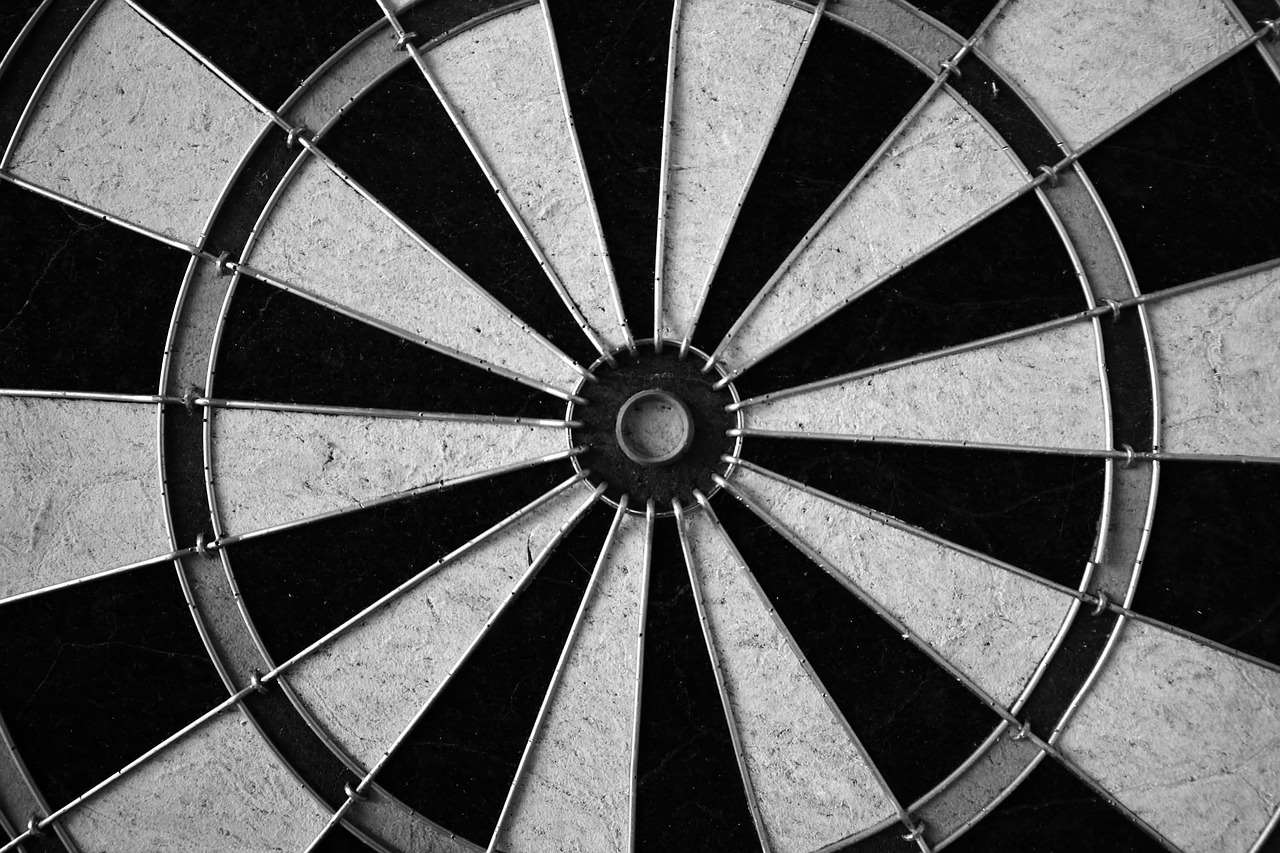
Conclusion
Mastering dart with extends is a critical skill for any Dart developer. This comprehensive guide has covered the fundamentals, advanced concepts, practical examples, and best practices to enable you to build robust and maintainable Dart applications. Remember to leverage inheritance judiciously, balancing its power with the principles of composition and code simplicity. Through understanding inheritance and employing the techniques highlighted here, you can write more efficient and organized code. By combining the power of dart with extends with best practices, you’ll create clean, reusable, and scalable applications. Start implementing these techniques in your projects today, and you’ll notice a significant improvement in your code quality and development efficiency. Happy coding!
For further learning, explore Dart’s official documentation and consider deepening your knowledge on topics such as dart legs definition, darts master out, and dart barrel dimensions to broaden your understanding of related concepts within the Dart ecosystem. You might also find valuable resources on advanced techniques like darts beste leg aller zeiten and james wade bullseye.
Hi, I’m Dieter, and I created Dartcounter (Dartcounterapp.com). My motivation wasn’t being a darts expert – quite the opposite! When I first started playing, I loved the game but found keeping accurate scores and tracking stats difficult and distracting.
I figured I couldn’t be the only one struggling with this. So, I decided to build a solution: an easy-to-use application that everyone, no matter their experience level, could use to manage scoring effortlessly.
My goal for Dartcounter was simple: let the app handle the numbers – the scoring, the averages, the stats, even checkout suggestions – so players could focus purely on their throw and enjoying the game. It began as a way to solve my own beginner’s problem, and I’m thrilled it has grown into a helpful tool for the wider darts community.