Understanding dart uint8list is crucial for efficient data handling in Dart applications. This article will not only explain what a dart uint8list is and how it works, but will also delve into practical usage scenarios and provide you with the knowledge to effectively implement it in your projects.
⚠️ Still Using Pen & Paper (or a Chalkboard)?! ⚠️
Step into the future! The Dart Counter App handles all the scoring, suggests checkouts, and tracks your stats automatically. It's easier than you think!
Try the Smart Dart Counter App FREE!Ready for an upgrade? Click above!
The dart uint8list, a fundamental data structure in Dart, provides a way to work with raw bytes. It’s essentially an array of unsigned 8-bit integers, offering a versatile approach to handling binary data such as images, audio files, or network packets. Mastering its use opens doors to powerful functionalities within your Dart applications.
Understanding Dart Uint8List: A Deep Dive
Let’s start by defining what a dart uint8list actually is. It’s a typed list that holds unsigned 8-bit integers, each ranging from 0 to 255. This makes it incredibly efficient for representing binary data. Think of it like a container specifically designed for storing raw bytes, unlike other Dart lists which can hold various data types. Understanding its capabilities allows you to leverage the full power of low-level data manipulation within your Dart projects. This is particularly useful when working with external libraries, APIs, or any scenario requiring direct interaction with binary data. You’ll find dart uint8list indispensable when dealing with cryptographic operations, image processing, or network communication. For instance, if you are building a Dart application that processes images, the image data is usually stored and manipulated using a dart uint8list. A strong grasp of this concept will greatly improve your coding efficiency and project robustness.
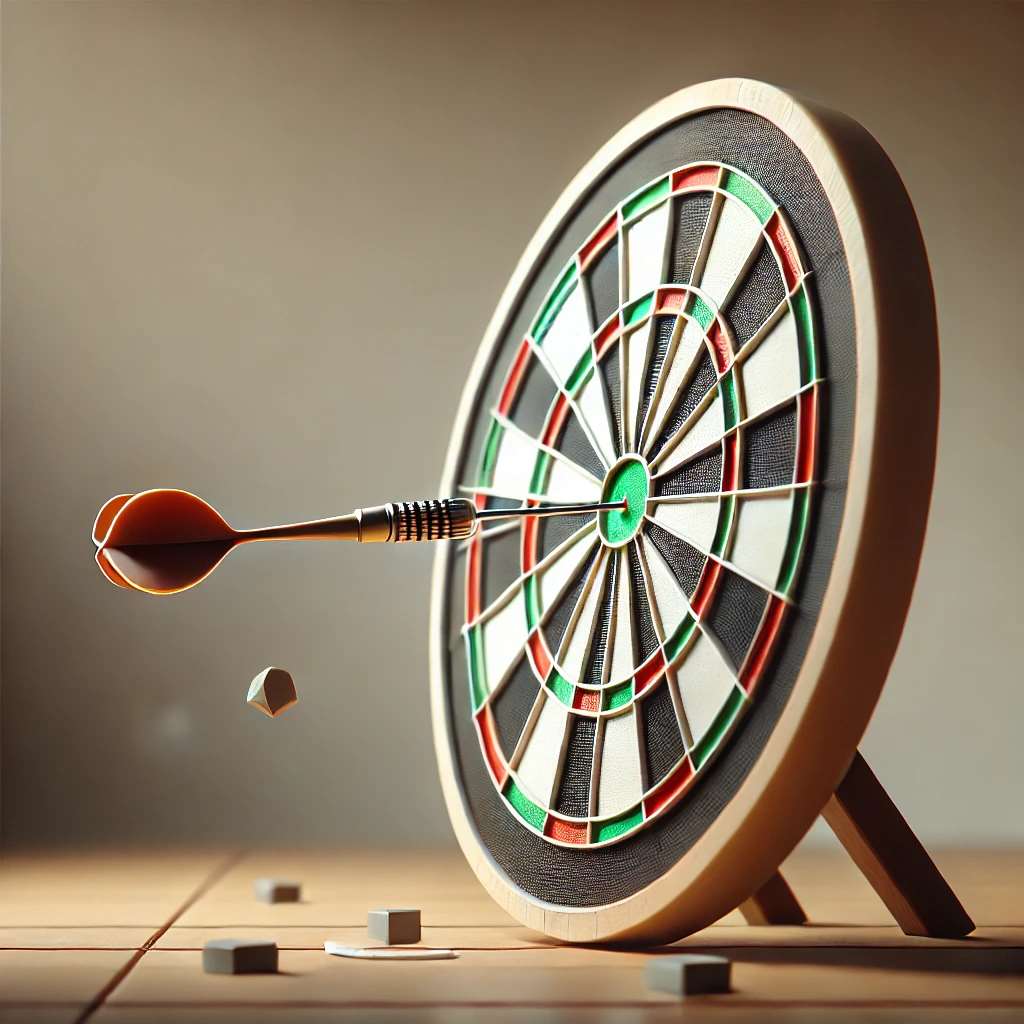
Creating a Dart Uint8List
Creating a dart uint8list is straightforward. You can initialize it with a specified length or from an existing list of integers. Here are a few common ways:
Uint8List(10);
// Creates a dart uint8list with a length of 10, initialized to all zeros.Uint8List.fromList([1, 2, 3, 4, 5]);
// Creates a dart uint8list from an existing list of integers.Uint8List.fromUint8List(anotherUint8List);
// Creates a new dart uint8list as a copy of an existingUint8List
object.
Remember that the elements in the list you provide to Uint8List.fromList
must all be integers within the range of 0-255. Any values outside this range will result in an error. This precision is essential for accurate binary data representation.
Manipulating Dart Uint8List
Once you have a dart uint8list, you can manipulate its contents using various methods. These methods are crucial for performing operations like data modification, concatenation, or extraction.
Accessing and Modifying Elements
You can access and modify individual elements using array indexing, just like with regular lists. For example, myUint8List[0] = 255;
sets the first element of myUint8List
to 255.
Adding and Removing Elements
While you can’t directly add or remove elements in the same way as a standard Dart List (due to its fixed size), you can create a new dart uint8list incorporating additions or removals using functions like sublist()
and by utilizing the Uint8List.fromList()
constructor.
Copying and Concatenating
Copying and concatenating dart uint8list objects is often necessary. The Uint8List.fromList()
constructor helps in concatenating multiple dart uint8list objects. You can also use the copyRange
method to efficiently copy parts of a dart uint8list. This is particularly important when working with large datasets to avoid unnecessary memory allocation or data duplication. You’ll often need this when handling large binary files, ensuring efficient data management.
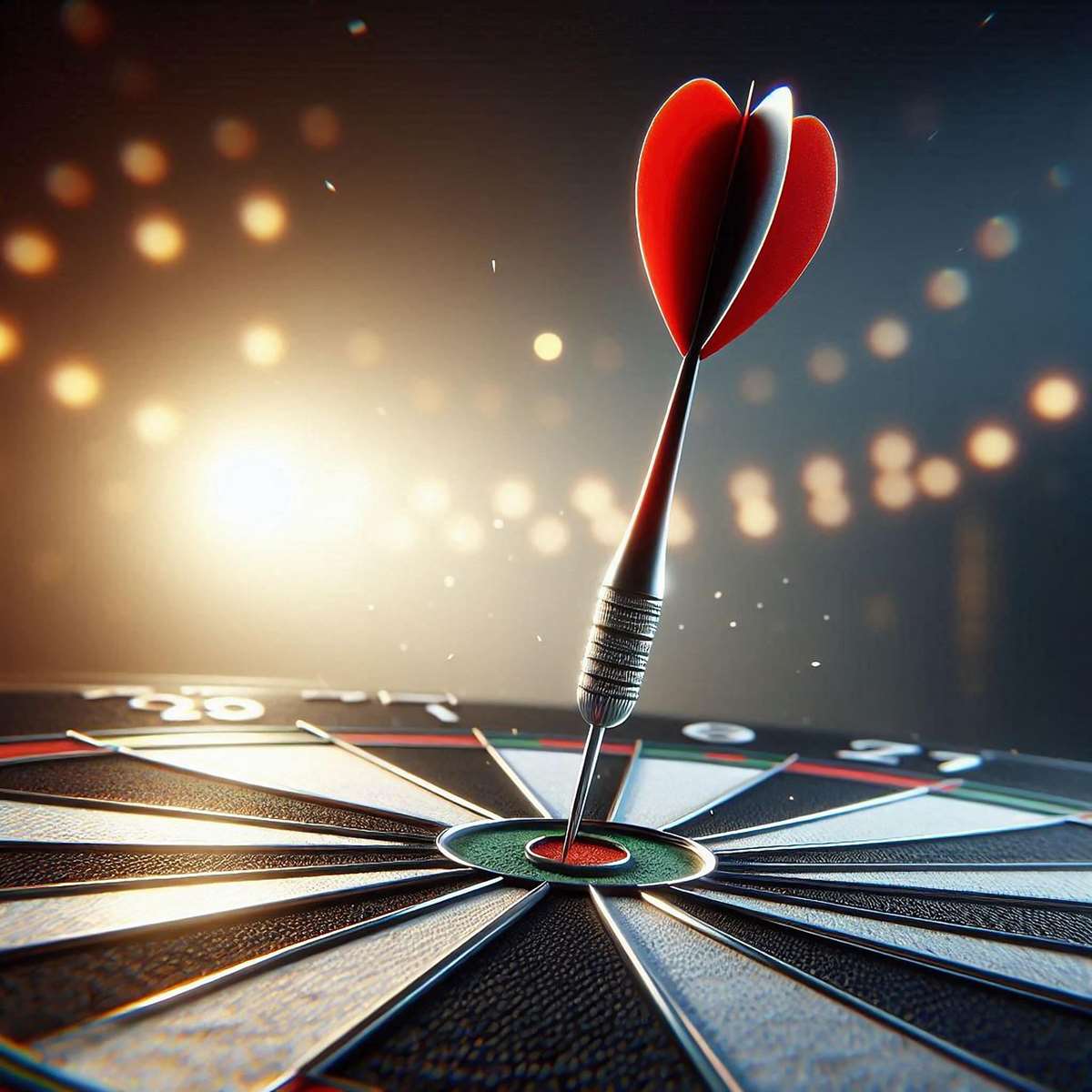
Real-World Applications of Dart Uint8List
The applications of dart uint8list extend far beyond simple integer storage. Let’s explore some real-world scenarios where it shines:
Image Processing
Images are often represented as streams of bytes. A dart uint8list is the perfect tool for manipulating image data directly, allowing for efficient encoding, decoding, and image manipulation. This is essential for image editing applications, image filters, and efficient image loading within your Dart applications.
Networking
When communicating over networks, data is often transmitted as bytes. A dart uint8list provides a structured way to handle incoming and outgoing network packets, ensuring seamless data handling and avoiding data corruption or misinterpretation. This is vital when developing client-server applications or network protocols. Efficient handling of network data is crucial for the performance and reliability of your applications.
File I/O
Reading and writing binary files often involves dealing with raw bytes. A dart uint8list allows for direct interaction with the file’s binary content, enabling efficient file reading, writing, and manipulation. This is essential when building applications that handle large binary files or those that require precise control over file data.
Cryptography
Cryptographic operations frequently rely on byte manipulation. A dart uint8list provides a secure and efficient way to handle encryption keys, ciphertext, and other cryptographic data, ensuring data integrity and security within your applications. This is an area where efficiency and precision are absolutely critical.
Error Handling and Best Practices
When working with dart uint8list, it’s important to consider potential errors and adopt best practices to avoid issues:
- IndexOutOfRangeException: Always ensure your index is within the bounds of the list to prevent this common error.
- Type Errors: Remember that a dart uint8list only accepts unsigned 8-bit integers (0-255). Attempting to use other data types will cause errors.
- Memory Management: When working with large dart uint8list objects, be mindful of memory usage to avoid performance bottlenecks or memory leaks. Proper garbage collection is crucial, and in specific circumstances, manual memory management might be needed for optimization.
Effective error handling and following these best practices is key to developing robust and efficient Dart applications that utilize dart uint8list. This will prevent unexpected crashes and ensure your application functions correctly under various conditions.
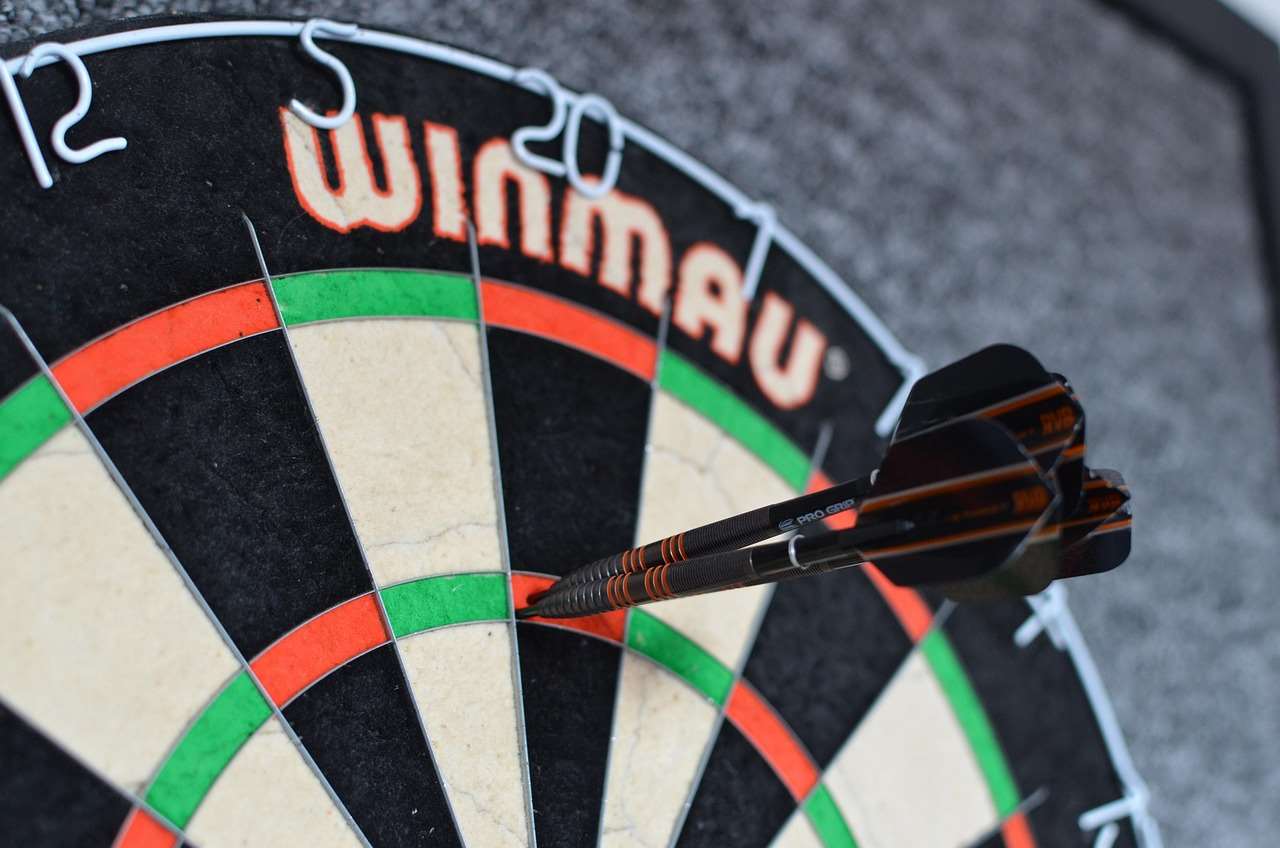
Advanced Techniques and Optimizations
For more advanced scenarios, consider these techniques:
Using Views
Sometimes, you need to access a portion of a larger dart uint8list without creating a complete copy. Dart provides the ability to create a view of a section of the dart uint8list, improving efficiency by avoiding needless duplication.
Working with External Libraries
Many external libraries in Dart require interaction with binary data. Understanding how to efficiently work with dart uint8list is essential for integrating these libraries seamlessly and making the most of their capabilities. A thorough understanding of dart uint8list will greatly facilitate this integration process.
Remember to consult the dart documentation for the most up-to-date information and detailed API specifications.
Example: Reading an Image File
Here’s a simplified example of how to read an image file into a dart uint8list:
import 'dart:io';
import 'dart:typed_data';
Future readImageFile(String filePath) async {
final file = File(filePath);
final bytes = await file.readAsBytes();
return Uint8List.fromList(bytes);
}
This snippet demonstrates a basic approach; more robust error handling and other optimizations may be necessary depending on your specific needs. This should help in understanding the practical application of dart uint8list.
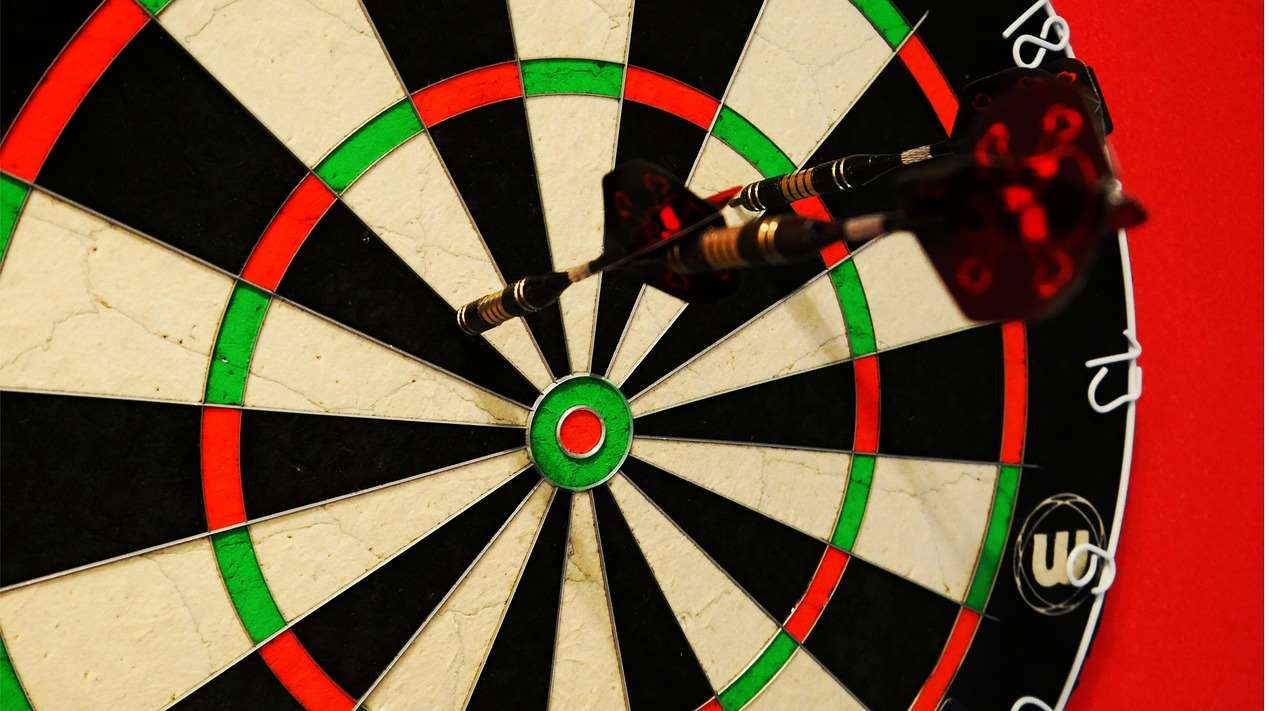
Conclusion
The dart uint8list is a powerful and versatile tool for handling binary data in Dart applications. Mastering its use opens up possibilities for efficient image processing, network communication, file I/O, and cryptographic operations. By understanding its features, utilizing best practices, and applying the techniques outlined above, you can significantly enhance your Dart development skills and create more efficient and robust applications. For more information on Dart development, explore our other articles, including guidance on where to find where to buy cheap darts, or learn about popular dart accessories like dart flight punch argos. You can also check out our review on the darts counter camera review to improve your dart game.
Remember to check out the official Dart documentation for the most updated information. Start experimenting with dart uint8list in your projects to experience its power firsthand! Don’t forget to try out our amazing Darts scoreboard app to elevate your dart game tracking! Finally, consider exploring high-quality dartboards like the xqmax mvg training dartboard to enhance your practice sessions.
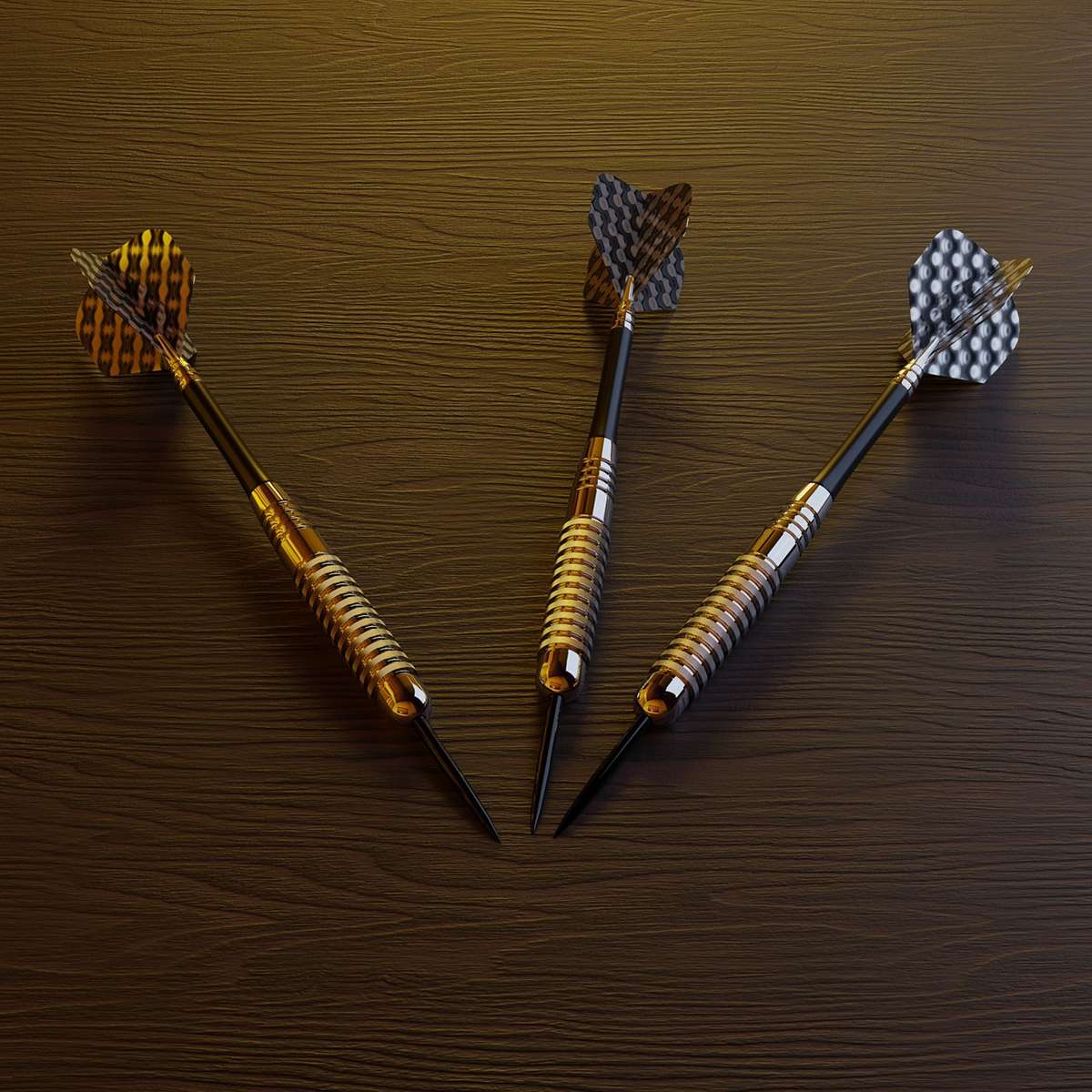
Hi, I’m Dieter, and I created Dartcounter (Dartcounterapp.com). My motivation wasn’t being a darts expert – quite the opposite! When I first started playing, I loved the game but found keeping accurate scores and tracking stats difficult and distracting.
I figured I couldn’t be the only one struggling with this. So, I decided to build a solution: an easy-to-use application that everyone, no matter their experience level, could use to manage scoring effortlessly.
My goal for Dartcounter was simple: let the app handle the numbers – the scoring, the averages, the stats, even checkout suggestions – so players could focus purely on their throw and enjoying the game. It began as a way to solve my own beginner’s problem, and I’m thrilled it has grown into a helpful tool for the wider darts community.