The cornerstone of reliable Dart applications is thorough testing, and this Dart Testing Methodology Guide provides a roadmap to building robust and maintainable software. We’ll delve into various testing types, frameworks, and best practices to ensure your Dart code performs flawlessly. You’ll learn about unit, widget, and integration testing, plus how to leverage tools to streamline your testing workflow.
⚠️ Still Using Pen & Paper (or a Chalkboard)?! ⚠️
Step into the future! The Dart Counter App handles all the scoring, suggests checkouts, and tracks your stats automatically. It's easier than you think!
Try the Smart Dart Counter App FREE!Ready for an upgrade? Click above!
Why a Solid Dart Testing Methodology Matters
In the world of software development, a robust testing strategy is not merely an option – it’s a necessity. For Dart, this translates to adopting a comprehensive Dart Testing Methodology that encompasses different types of tests and best practices. Poorly tested code can lead to unpredictable behavior, reduced reliability, and ultimately, a negative user experience. Investing in a well-defined testing process ensures your application functions as expected, scales effectively, and remains maintainable over time.
A proper test suite acts as a safety net, catching potential bugs and errors before they reach your end-users. This is particularly important for complex applications with intricate logic and numerous dependencies. Furthermore, effective testing promotes a more agile development process, enabling you to confidently refactor and add new features without introducing regressions.
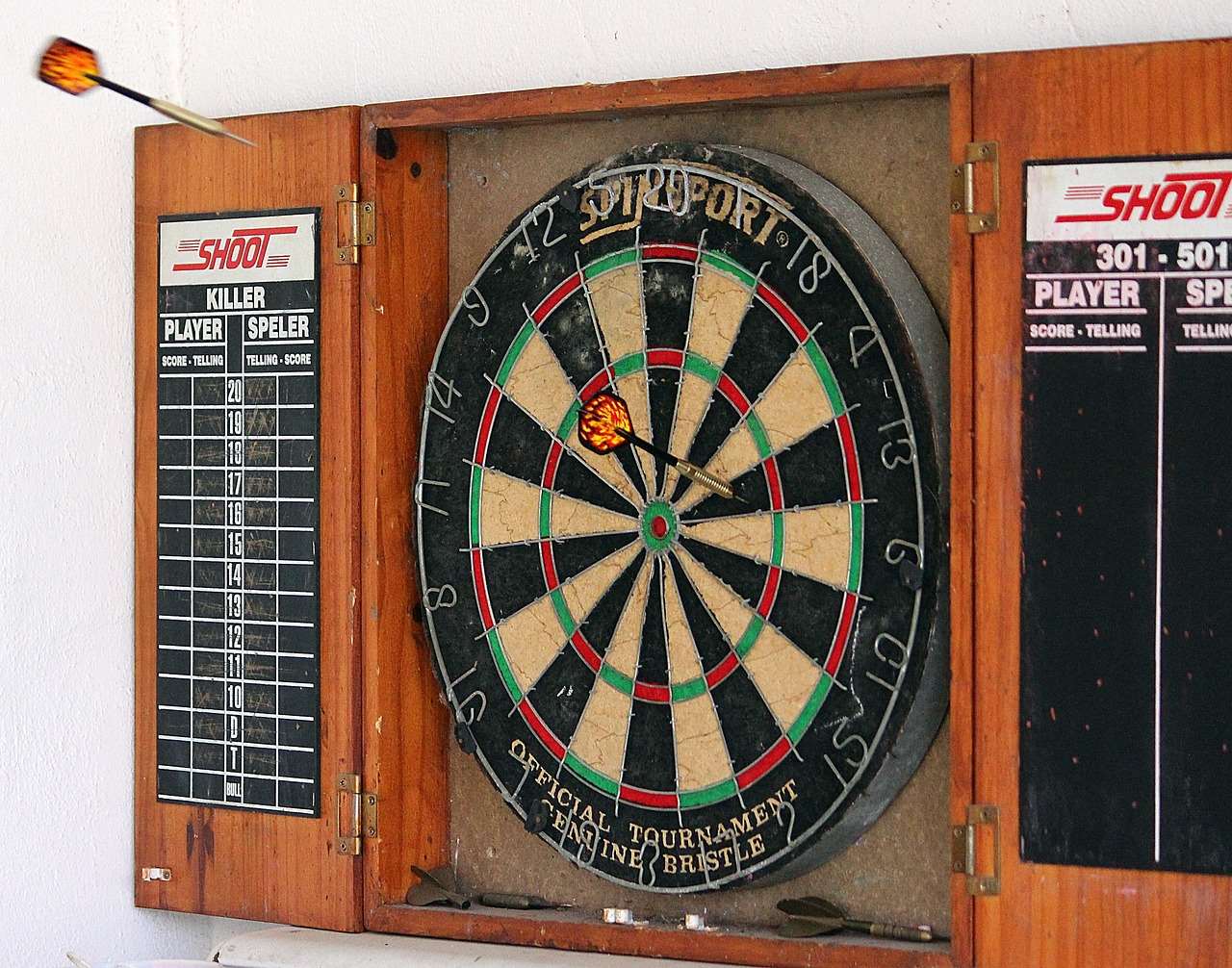
Understanding Different Types of Dart Tests
A core element of any Dart Testing Methodology Guide is understanding the different types of tests and when to apply them. Each type serves a specific purpose and contributes to overall code quality.
Unit Tests
Unit tests are the most basic form of testing. They focus on verifying the functionality of individual units of code, such as functions or classes, in isolation. This means mocking or stubbing out any dependencies to ensure the unit under test is the sole focus. The goal is to confirm that each unit performs its intended task correctly.
- Benefits: Fast execution, easy to write, pinpoint issues quickly.
- Tools: The core `test` package in Dart provides all the necessary tools for writing unit tests. You might also use mocking libraries like `mockito`.
- Example: Testing a function that calculates the area of a rectangle.
Widget Tests
Widget tests are specific to Flutter and focus on verifying the behavior and appearance of individual widgets. These tests simulate user interactions and verify that the widget responds as expected. They are crucial for ensuring the UI is functioning correctly and that the user interface components are rendering properly.
- Benefits: Ensures UI components work as expected, verifies layout and rendering.
- Tools: Flutter’s `flutter_test` package provides a rich set of tools for writing widget tests.
- Example: Testing that a button displays the correct text and triggers the expected action when tapped. You can also find the Optimal Dartboard Lighting Solutions Guide for visual enhancements to your Dart project.
Integration Tests
Integration tests examine the interaction between different parts of your application, such as multiple classes, widgets, or external services. They verify that these components work together harmoniously and that data flows correctly between them. Unlike unit tests, integration tests involve multiple modules and dependencies, offering a broader view of system behavior.
- Benefits: Verifies interactions between different modules, detects integration issues.
- Tools: Flutter’s `flutter_test` package and integration_test package are commonly used.
- Example: Testing the flow of data from a user input field, through a data processing service, and into a database.
End-to-End (E2E) Tests
End-to-End (E2E) tests simulate real user scenarios, testing the entire application from start to finish. They typically involve interacting with the UI and verifying that the application behaves as expected in a production-like environment. E2E tests are the most comprehensive type of testing, but also the slowest and most complex to set up.
- Benefits: Verifies the entire application flow, simulates real user behavior.
- Tools: Tools like Flutter Driver and Patrol are often used for E2E testing.
- Example: Testing the entire user registration process, from filling out the form to verifying the email confirmation.
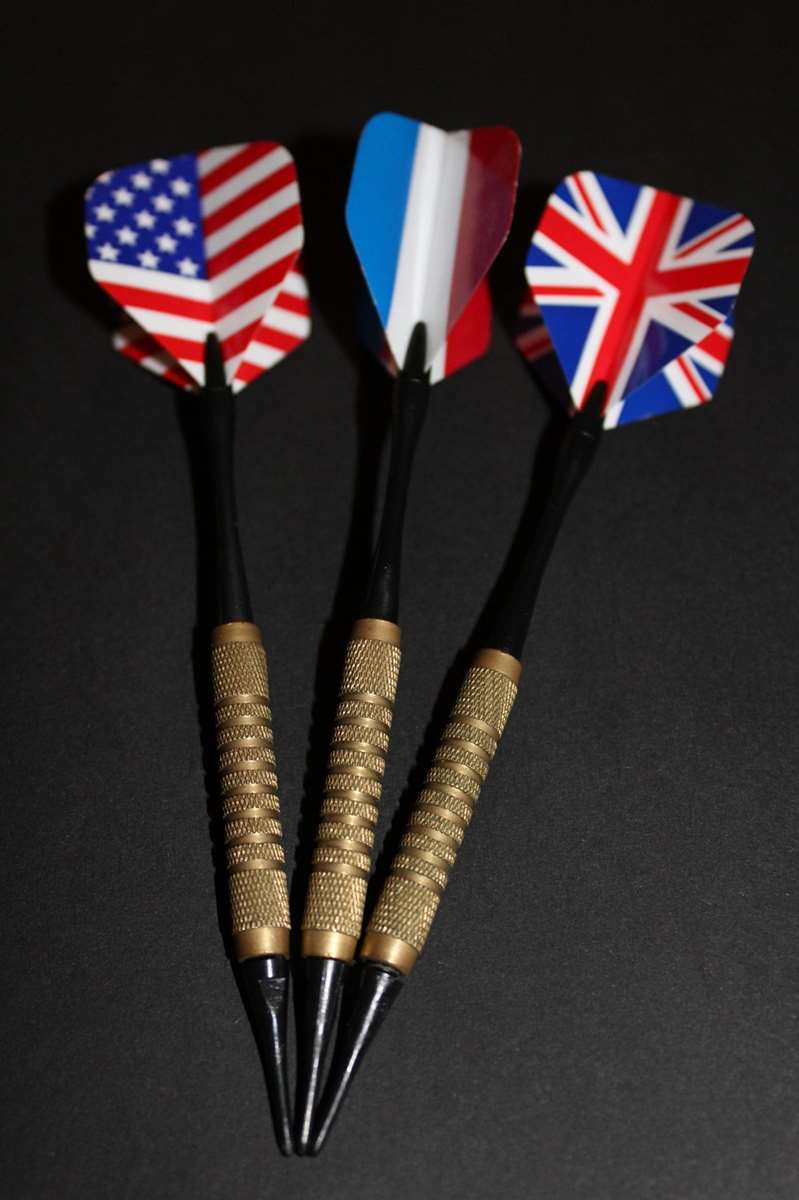
Setting Up Your Dart Testing Environment
Before diving into writing tests, it’s crucial to set up a proper testing environment. This involves installing the necessary dependencies, configuring your project, and choosing the right tools. Here’s a breakdown of the key steps:
- Add Dependencies: Add the `test` package to your `dev_dependencies` in your `pubspec.yaml` file. For widget tests, you’ll also need `flutter_test` (which is often included by default in Flutter projects). For integration testing, add `integration_test`.
- Create a Test Directory: Create a dedicated `test` directory in your project’s root. This helps organize your test files.
- Configure Test Files: Create test files with the `_test.dart` suffix (e.g., `my_widget_test.dart`).
- Use a Test Runner: Dart comes with a built-in test runner. You can run your tests from the command line using `dart test` or `flutter test` (for Flutter projects). IDEs like VS Code and Android Studio also provide built-in test runners.
Writing Effective Dart Tests: Best Practices
Writing effective tests goes beyond simply checking if your code runs without errors. It involves following best practices to ensure your tests are reliable, maintainable, and provide valuable feedback.
- Write Clear and Concise Tests: Each test should focus on a specific aspect of the code. Avoid writing overly complex tests that are difficult to understand and debug.
- Use Descriptive Test Names: Give your tests meaningful names that clearly describe what they are testing. This makes it easier to understand the purpose of each test and identify failures.
- Follow the Arrange-Act-Assert (AAA) Pattern: Structure your tests using the AAA pattern:
- Arrange: Set up the necessary preconditions for the test.
- Act: Execute the code being tested.
- Assert: Verify that the code produced the expected result.
- Keep Tests Independent: Each test should be independent of other tests. Avoid relying on shared state or dependencies that could cause tests to fail unexpectedly.
- Test Edge Cases and Error Conditions: Don’t just test the happy path. Test edge cases, boundary conditions, and error conditions to ensure your code handles unexpected inputs and situations gracefully.
- Write Tests First (Test-Driven Development – TDD): Consider using Test-Driven Development (TDD), where you write the tests before writing the actual code. This helps you define the expected behavior of your code upfront and ensures that you write code that is testable.
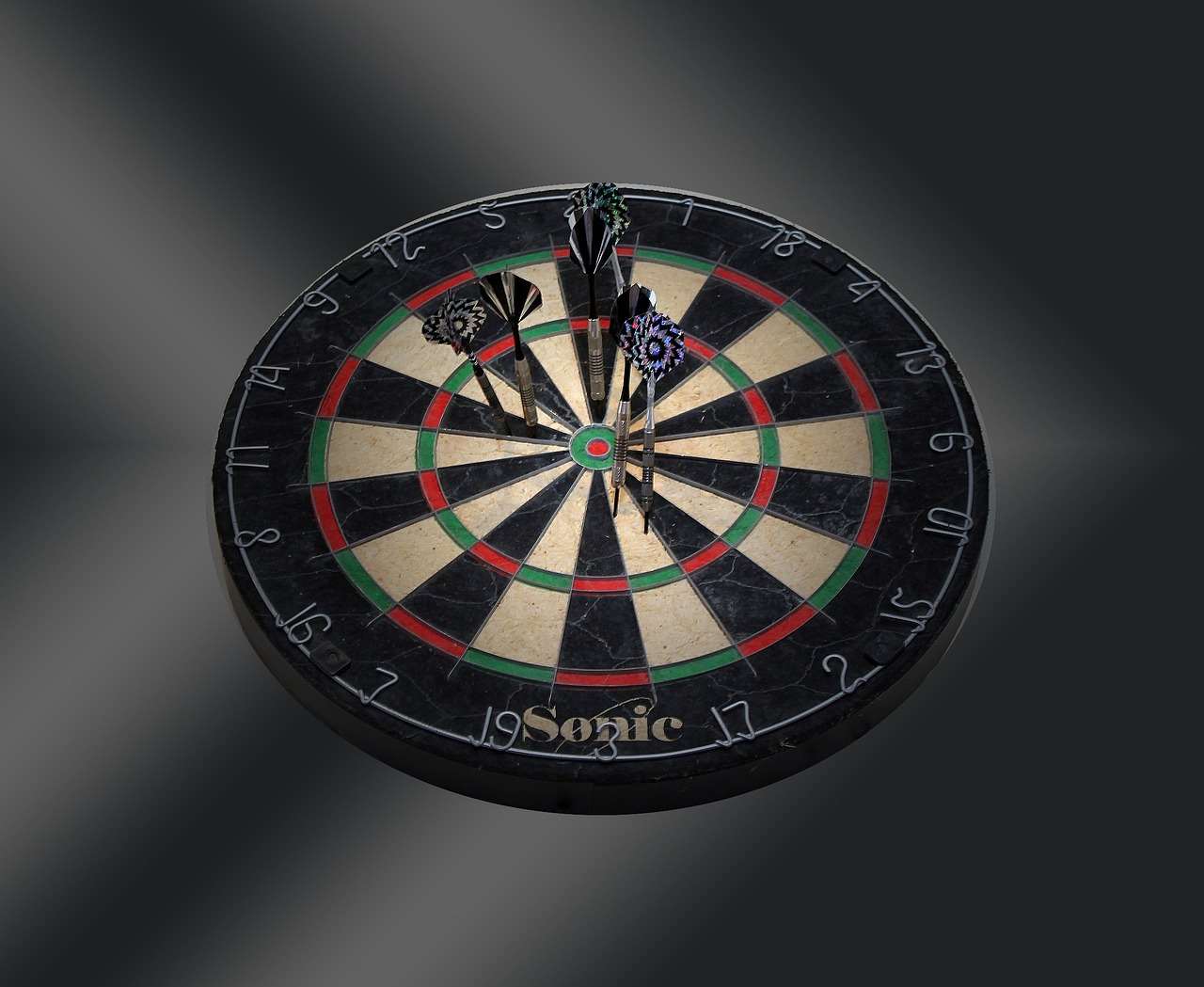
Dart Testing Frameworks and Tools
Dart offers a variety of frameworks and tools to assist with testing. Some of the most popular options include:
- `test` Package: The built-in `test` package provides the core functionality for writing and running tests in Dart. It offers a simple and intuitive API for defining tests, grouping tests, and making assertions.
- `flutter_test` Package: This package is specifically designed for testing Flutter widgets. It provides tools for interacting with widgets, verifying their state, and simulating user interactions.
- `mockito` Package: A powerful mocking library that allows you to create mock objects for dependencies, enabling you to isolate the unit under test.
- `integration_test` Package: Provides APIs for writing end-to-end tests for Flutter apps.
- Code Coverage Tools: Tools like `coverage` can help you measure the percentage of your code that is covered by tests. This provides valuable insights into the effectiveness of your testing efforts.
The Choose Best Dart Equipment guide can help you find resources for improving your development environment and workflow.
Automating Your Dart Testing Process
To ensure consistent and reliable testing, it’s essential to automate your testing process. This involves integrating your tests into your build pipeline so that they are automatically executed whenever code is changed. Here are some tips for automating your Dart tests:
- Use a Continuous Integration (CI) System: Integrate your tests with a CI system like GitHub Actions, GitLab CI, or Travis CI. This will automatically run your tests whenever you push code to your repository.
- Configure Code Coverage Reporting: Set up your CI system to collect code coverage data and report it as part of the build process. This will help you track the effectiveness of your testing efforts and identify areas where you need to add more tests.
- Set Up Notifications: Configure your CI system to send notifications when tests fail. This will allow you to quickly identify and address issues before they impact your users.
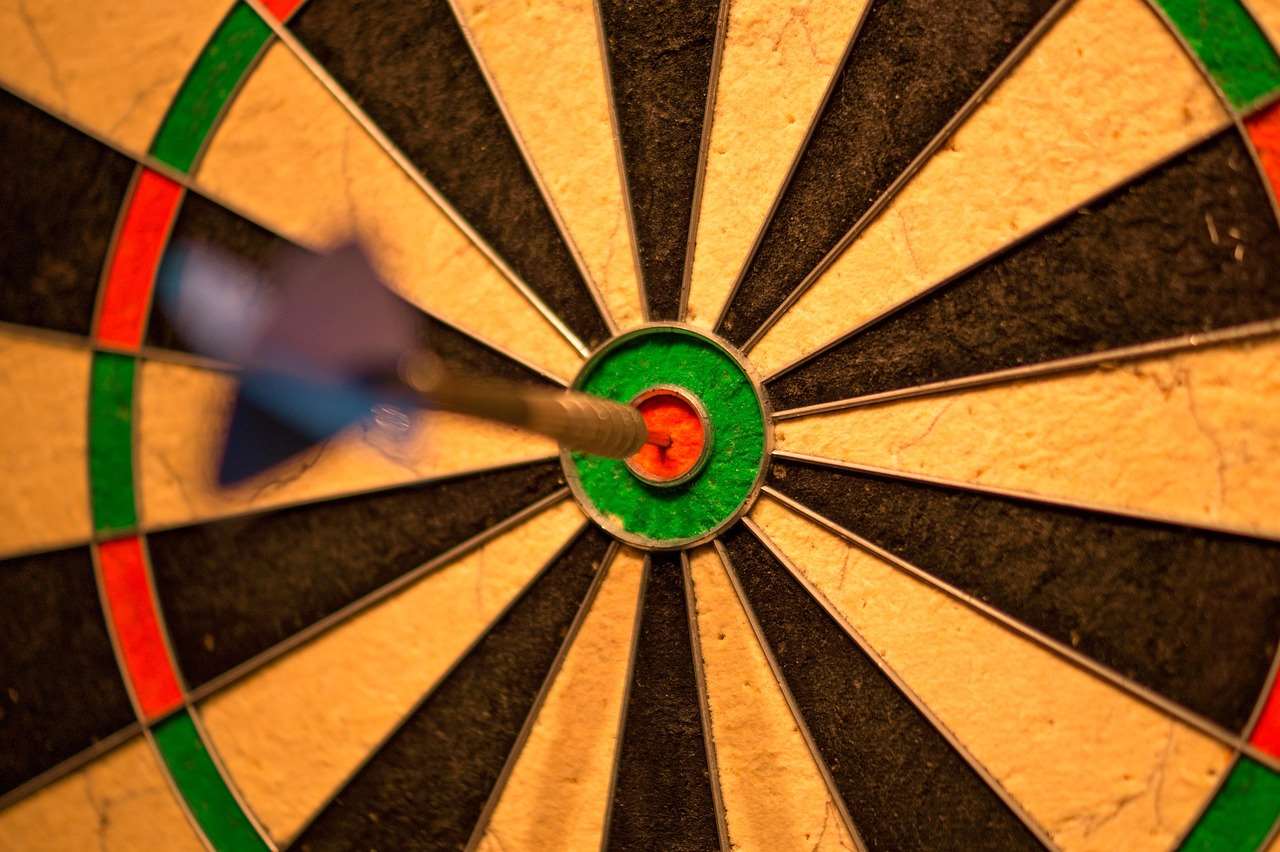
Debugging Failed Tests
When tests fail, it’s important to have a systematic approach to debugging. Here are some tips:
- Read the Error Message: Start by carefully reading the error message. It often provides valuable clues about the cause of the failure.
- Use a Debugger: Use a debugger to step through the code and examine the state of variables at different points in the execution.
- Write Debugging Statements: Add print statements to your code to output the values of variables or track the flow of execution.
- Isolate the Issue: Try to isolate the issue by commenting out parts of the code or simplifying the test case.
Maintaining Your Test Suite
Your test suite is a living document that needs to be maintained and updated as your code evolves. Here are some tips for keeping your test suite in good shape:
- Keep Tests Up-to-Date: As you refactor or change your code, make sure to update your tests accordingly.
- Remove Redundant Tests: Over time, some tests may become redundant or obsolete. Remove these tests to keep your test suite lean and efficient.
- Refactor Tests: Just like your production code, your tests should be refactored periodically to improve their readability and maintainability.
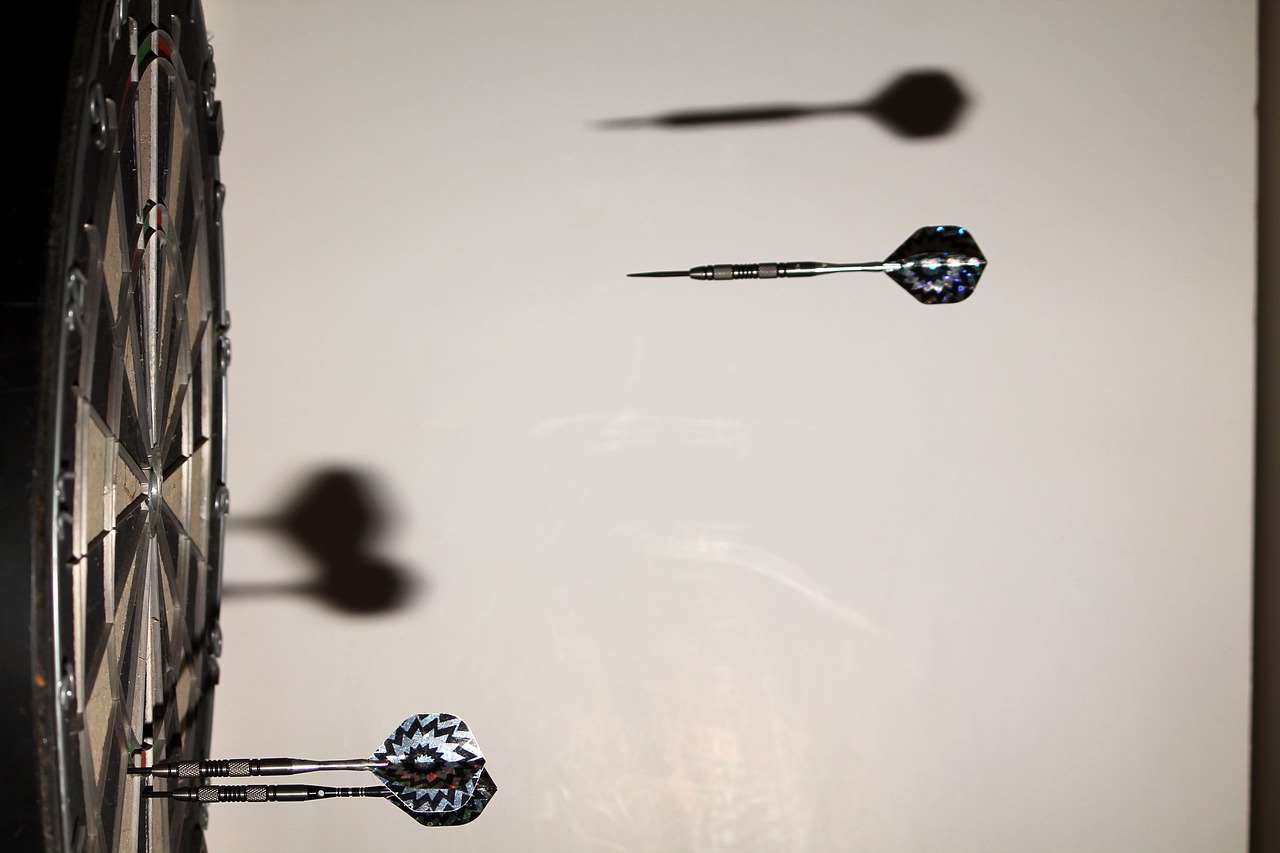
Conclusion
Implementing a solid Dart Testing Methodology Guide is an investment that pays dividends in the form of more reliable, maintainable, and scalable applications. By understanding the different types of tests, setting up a proper testing environment, following best practices, and automating your testing process, you can build high-quality Dart applications that meet the needs of your users. Remember to continuously maintain and improve your test suite to keep it relevant and effective. Start building your robust testing strategy today and witness the improved quality and reliability of your Dart projects. Consider further reading on Types Optimal Dartboard Lighting to enhance your testing and debugging environment.
Hi, I’m Dieter, and I created Dartcounter (Dartcounterapp.com). My motivation wasn’t being a darts expert – quite the opposite! When I first started playing, I loved the game but found keeping accurate scores and tracking stats difficult and distracting.
I figured I couldn’t be the only one struggling with this. So, I decided to build a solution: an easy-to-use application that everyone, no matter their experience level, could use to manage scoring effortlessly.
My goal for Dartcounter was simple: let the app handle the numbers – the scoring, the averages, the stats, even checkout suggestions – so players could focus purely on their throw and enjoying the game. It began as a way to solve my own beginner’s problem, and I’m thrilled it has grown into a helpful tool for the wider darts community.