Effective Dart Testing In Different Conditions is crucial for ensuring your Dart applications are robust and reliable; understanding how to test in various scenarios leads to better code and fewer bugs. This article explores different testing methodologies in Dart, covering unit tests, widget tests, integration tests, and strategies for handling edge cases and varying environments.
⚠️ Still Using Pen & Paper (or a Chalkboard)?! ⚠️
Step into the future! The Dart Counter App handles all the scoring, suggests checkouts, and tracks your stats automatically. It's easier than you think!
Try the Smart Dart Counter App FREE!Ready for an upgrade? Click above!
Understanding the Importance of Dart Testing In Different Conditions
The goal of testing is to verify that your code behaves as expected under all sorts of conditions. This is especially important in Dart, where you might be building anything from command-line tools to complex Flutter applications. Comprehensive testing helps you catch bugs early, refactor with confidence, and improve the overall quality of your software. Let’s dive into why testing is so critical:
- Early Bug Detection: Identifying issues during development is far cheaper and easier than fixing them in production.
- Regression Prevention: Tests act as a safety net, ensuring that new changes don’t break existing functionality.
- Improved Code Design: Writing testable code often leads to better-structured and more modular designs.
- Documentation: Tests serve as executable documentation, illustrating how your code is intended to be used.
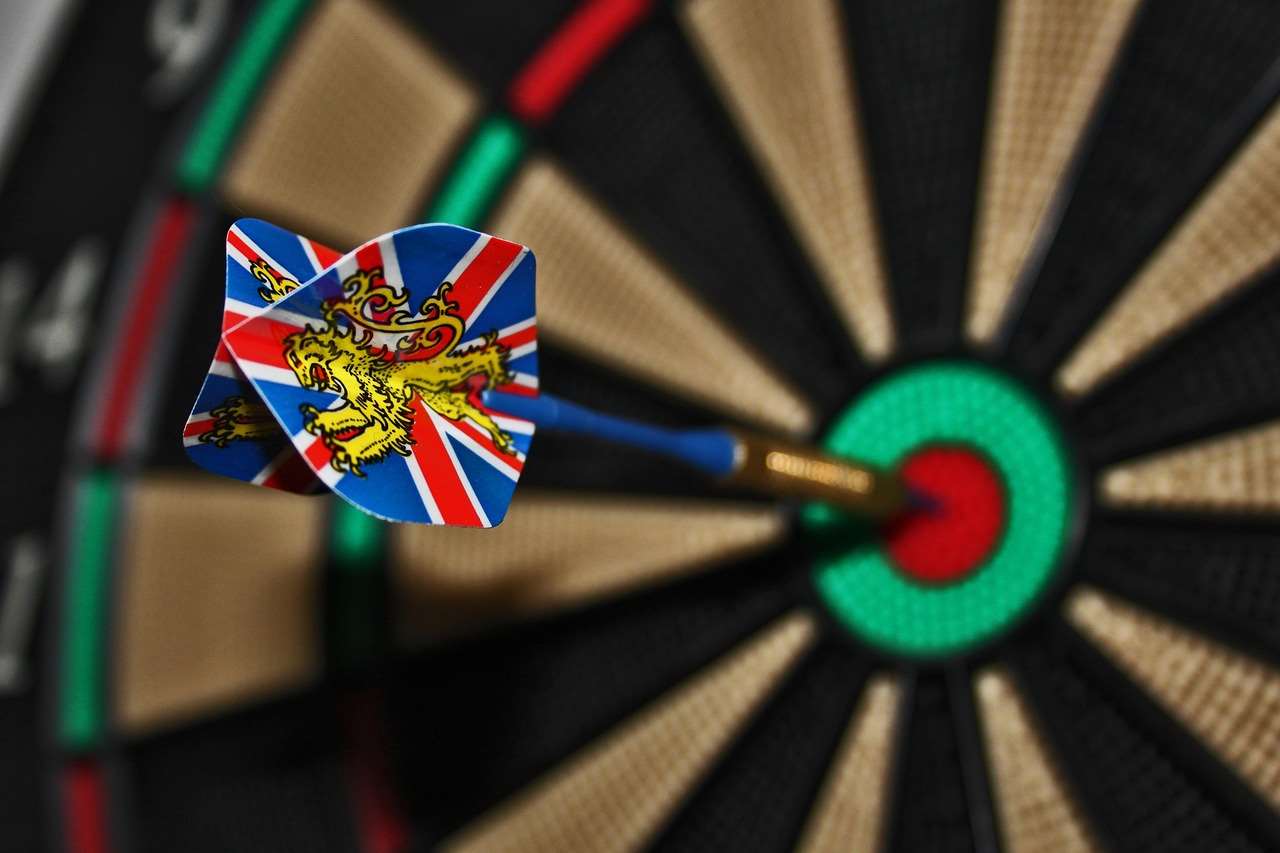
Types of Dart Tests
Dart offers different types of tests to cater to various aspects of your application. Understanding these types and their purposes is essential for creating a comprehensive testing strategy.
Unit Tests
Unit tests focus on isolated pieces of code, typically individual functions or classes. They verify that each unit performs its intended task correctly, independent of other parts of the system. Unit tests should be fast and focused.
For example, if you have a function that calculates the area of a circle, a unit test would specifically test that function with different radius values and assert that the calculated area is correct. You could use mocking frameworks for this purpose, but consider the tradeoffs that come with them.
Key aspects of unit testing:
- Isolation: Test each unit in isolation, without dependencies on external resources or other units.
- Speed: Unit tests should execute quickly, allowing for frequent testing during development.
- Focus: Each test should focus on a single, specific aspect of the unit’s behavior.
Widget Tests
Widget tests, specific to Flutter, focus on verifying the behavior and appearance of individual widgets. They allow you to simulate user interactions and ensure that widgets render correctly and respond as expected. Widget tests provide a critical layer of assurance for your Flutter UI.
For instance, you can simulate tapping a button and verify that a specific function is called or that the UI updates accordingly. To improve the user experience, widget tests can be used to verify that UI elements render correctly in different screen sizes and device orientations.
Key aspects of widget testing:
- UI Verification: Ensure widgets render correctly and display the expected content.
- Interaction Simulation: Simulate user interactions like taps, swipes, and text input.
- State Management: Verify that widgets update their state correctly in response to user interactions.
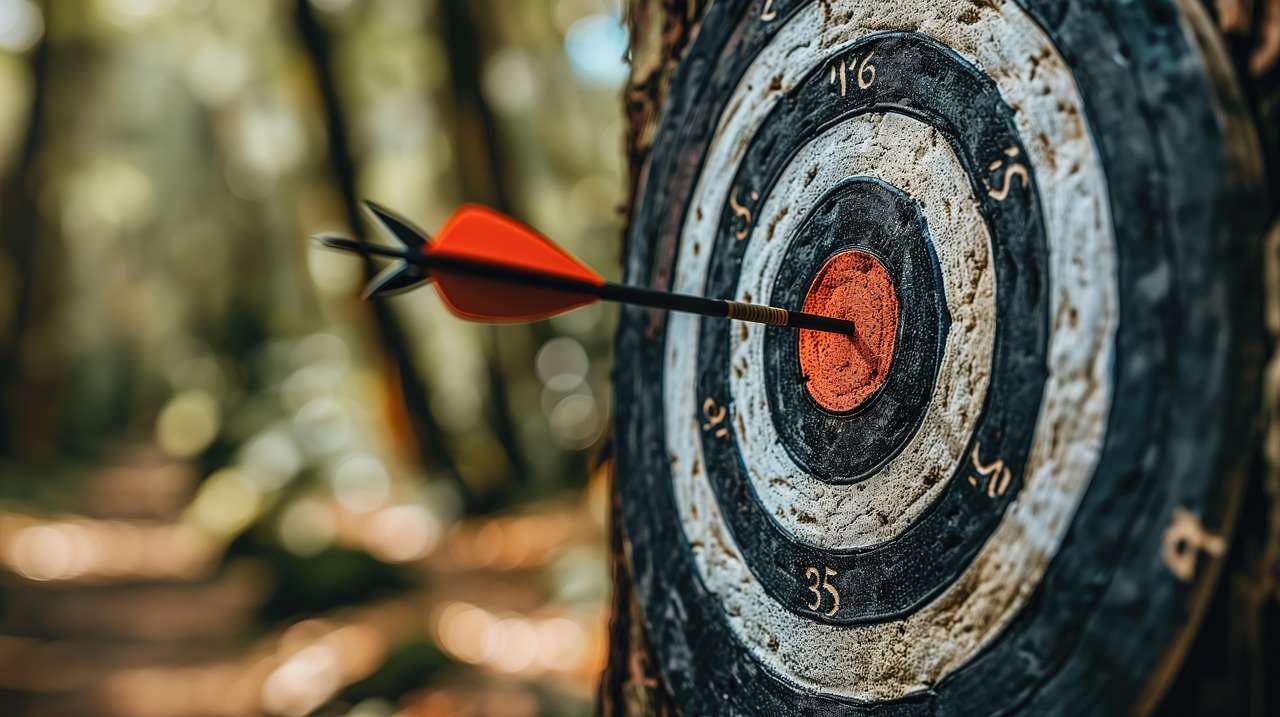
Integration Tests
Integration tests verify the interaction between different parts of your application. They test how units work together, ensuring that data flows correctly between them and that the overall system functions as intended. Integration tests provide a broader view of your application’s behavior than unit tests.
For example, an integration test might verify that a user can successfully log in to the application by testing the interaction between the UI, the authentication service, and the database. This requires setting up the environment in a realistic way.
Key aspects of integration testing:
- Component Interaction: Test how different parts of your application work together.
- Data Flow: Verify that data is passed correctly between components.
- System-Level Behavior: Ensure the overall system functions as intended.
End-to-End (E2E) Tests
End-to-end (E2E) tests simulate real user scenarios, testing the entire application from start to finish. They verify that the application functions correctly in a production-like environment, ensuring that all components work together seamlessly. E2E tests are the most comprehensive type of testing, but they can also be the most time-consuming to set up and maintain.
For instance, an E2E test might simulate a user creating an account, logging in, adding items to a shopping cart, and checking out. This type of testing typically requires setting up a complete test environment, including databases and external services.
Key aspects of E2E testing:
- Real User Scenarios: Simulate how users interact with the application in a real-world setting.
- Full System Verification: Test the entire application stack, from the UI to the database.
- Production-Like Environment: Run tests in an environment that closely resembles the production environment.
Testing for Edge Cases and Boundary Conditions
Edge cases and boundary conditions are inputs or situations that lie at the extreme ends of the expected range or represent unusual or unexpected scenarios. Testing these cases is crucial for ensuring your application handles unexpected situations gracefully and doesn’t crash or produce incorrect results. For example, testing with extremely large numbers, empty strings, or null values can reveal potential vulnerabilities.
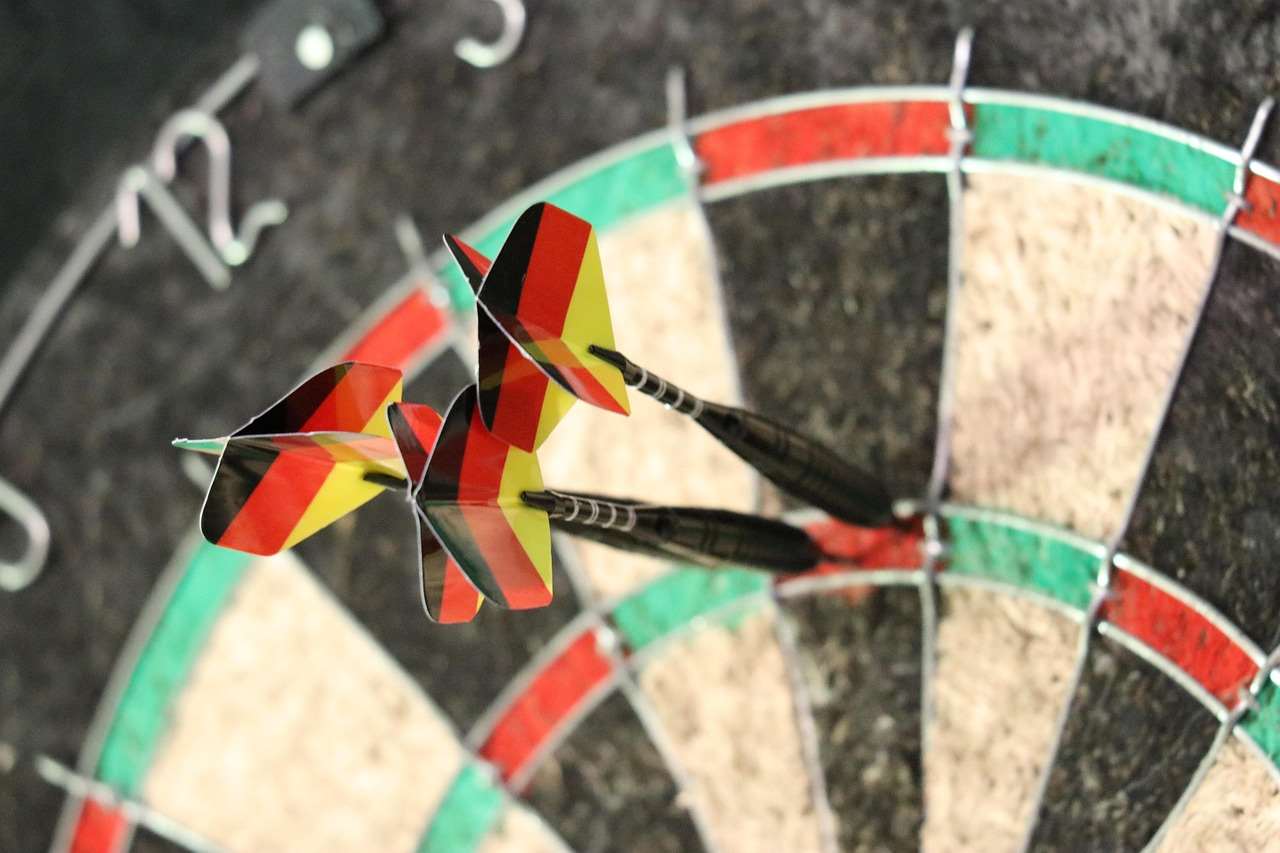
Common Edge Cases
Some common edge cases to consider include:
- Empty Inputs: Test with empty strings, empty lists, or empty objects.
- Null Values: Test with null values for parameters and variables.
- Large Numbers: Test with extremely large or small numbers.
- Invalid Data: Test with invalid data types or formats.
- Unexpected User Input: Test with unexpected or malicious user input.
Strategies for Testing Edge Cases
Here are some strategies for effectively testing edge cases:
- Boundary Value Analysis: Test values at the boundaries of the input range (e.g., the minimum and maximum values).
- Equivalence Partitioning: Divide the input range into equivalence partitions and test one value from each partition.
- Error Injection: Intentionally introduce errors into your code to see how it handles them.
- Fuzzing: Use automated tools to generate random inputs and test how your code responds.
Testing Asynchronous Code
Dart, especially in Flutter, relies heavily on asynchronous operations. Testing asynchronous code requires special considerations to ensure that your tests wait for the asynchronous operations to complete before making assertions. Using `async` and `await` keywords is central here, in conjunction with libraries like `completer`. It’s equally important to use proper timeouts and handle errors that might arise.
Testing asynchronous code is often more complex than testing synchronous code, but it’s essential for ensuring that your application handles asynchronous operations correctly. Dart’s testing framework provides tools and techniques to simplify the process, allowing you to write robust and reliable tests for your asynchronous code. By carefully testing asynchronous interactions, you ensure overall stability.

Using async
and await
The async
and await
keywords are essential for testing asynchronous code. The async
keyword marks a function as asynchronous, while the await
keyword pauses the execution of the function until a Future completes.
Handling Timeouts
When testing asynchronous code, it’s important to handle timeouts to prevent tests from running indefinitely. The timeout
parameter in the test
function allows you to specify a maximum duration for the test. You can also explore high quality gear with solid warranties to reduce overall risk.
Testing Streams
Streams are another important aspect of asynchronous programming in Dart. Testing streams requires verifying that data is emitted correctly and that the stream completes as expected. The expectLater
function can be used to assert that a stream emits a specific sequence of values.
Simulating Different Environments
Your application might behave differently in different environments, such as development, staging, and production. It’s essential to simulate these environments during testing to ensure that your application functions correctly in each environment. Factors like database connections, API endpoints, and feature flags can vary between environments, so testing with realistic configurations is critical.
This involves simulating different operating systems, screen sizes, network conditions, and device capabilities. By considering these factors, you can write tests that are more representative of real-world usage scenarios.
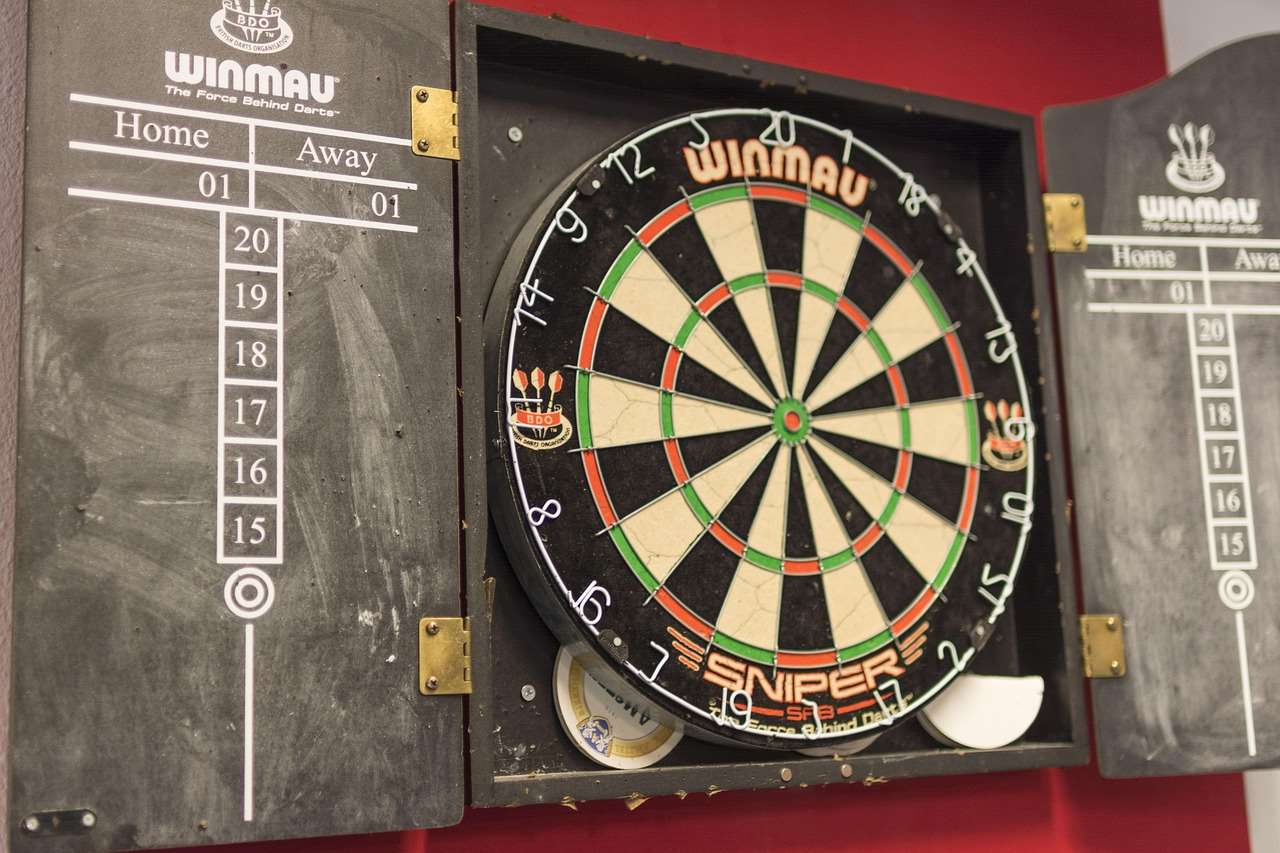
Using Mocking Frameworks
Mocking frameworks allow you to replace real dependencies with mock objects during testing. This is useful for isolating your code and simulating different environment conditions. Mockito is a popular mocking framework for Dart. Choosing dart equipment well can help with focus.
Setting Environment Variables
Environment variables can be used to configure your application for different environments. You can set environment variables during testing to simulate different configurations. The best approach is to load environment variables from a configuration file that matches the intended environment. This ensures that the correct settings are used for each test run.
Testing with Different Locales
Applications that support multiple languages need to be tested with different locales to ensure that text is displayed correctly and that date and time formats are appropriate. Dart’s intl
package provides tools for handling localization, and you can use the testWidgets
function in Flutter to test UI elements with different locales. Don’t just think about language, you also need to consider currencies and other region-specific formats.
Conclusion
Dart Testing In Different Conditions is vital for building robust and reliable applications. By understanding the different types of tests, testing for edge cases, handling asynchronous code, and simulating different environments, you can create a comprehensive testing strategy that ensures your code functions correctly in all situations. Remember to write clear, concise, and maintainable tests that cover all critical aspects of your application. Start small, automate frequently, and continuously improve your testing processes. Embrace the benefits of thorough testing and elevate the quality of your Dart applications.
Explore the ultimate dart equipment to take your game to the next level!
Hi, I’m Dieter, and I created Dartcounter (Dartcounterapp.com). My motivation wasn’t being a darts expert – quite the opposite! When I first started playing, I loved the game but found keeping accurate scores and tracking stats difficult and distracting.
I figured I couldn’t be the only one struggling with this. So, I decided to build a solution: an easy-to-use application that everyone, no matter their experience level, could use to manage scoring effortlessly.
My goal for Dartcounter was simple: let the app handle the numbers – the scoring, the averages, the stats, even checkout suggestions – so players could focus purely on their throw and enjoying the game. It began as a way to solve my own beginner’s problem, and I’m thrilled it has grown into a helpful tool for the wider darts community.