Effectively grouping tests in Dart enhances organization, readability, and maintainability, allowing for focused execution and streamlined debugging. This article will guide you through the principles and practices of Dart Testing For Grouping, covering everything from basic group definitions to advanced nesting and conditional execution.
⚠️ Still Using Pen & Paper (or a Chalkboard)?! ⚠️
Step into the future! The Dart Counter App handles all the scoring, suggests checkouts, and tracks your stats automatically. It's easier than you think!
Try the Smart Dart Counter App FREE!Ready for an upgrade? Click above!
Understanding Test Groups in Dart
In Dart, test groups are a fundamental way to organize your tests logically. Instead of having a long list of individual tests, you can categorize them based on functionality, modules, or any other relevant criteria. This not only makes your test suite easier to navigate but also allows you to run specific subsets of tests, saving time and effort.
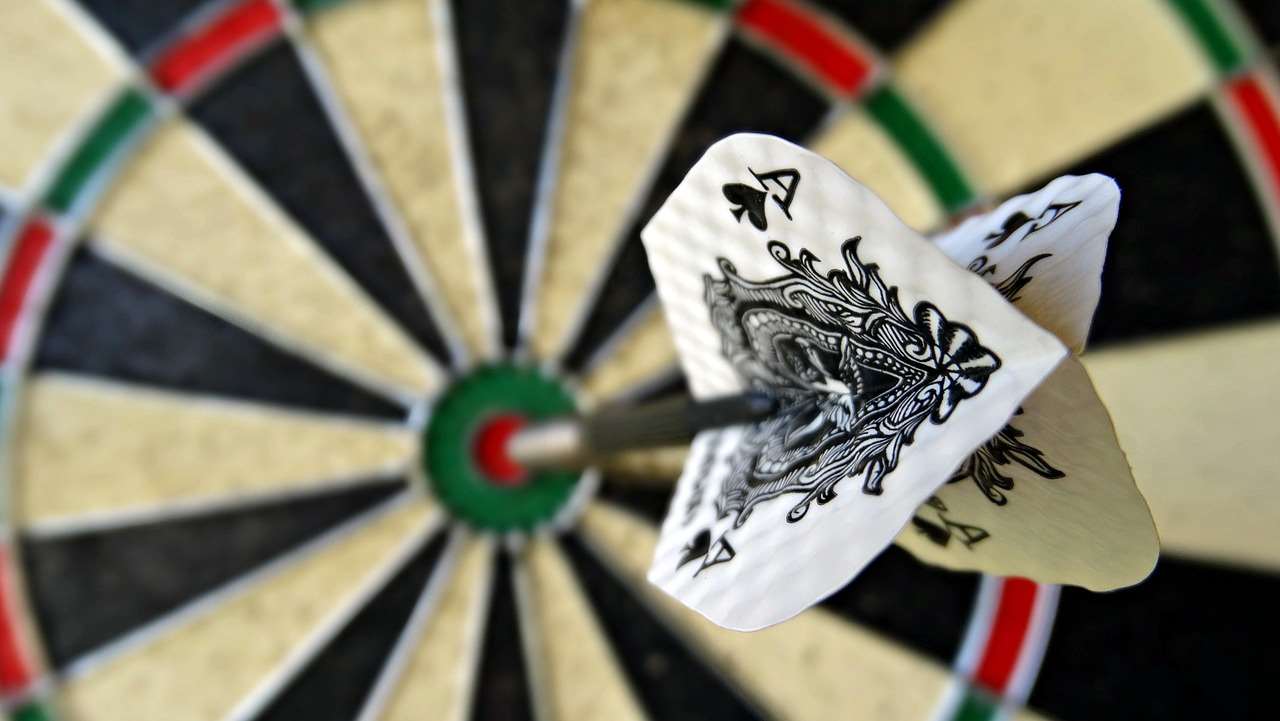
The primary benefit of using groups is improved test organization. When dealing with complex applications, a flat structure of tests can become unwieldy quickly. Groups provide a hierarchical structure that mirrors the architecture of your code, making it easier to find and understand related tests. This also aids in debugging, as you can quickly isolate issues to specific parts of your application.
Basic Group Definition
The most basic way to define a test group in Dart is using the group()
function. This function takes two arguments: a description (a string) and a callback function containing the tests that belong to the group. Here’s a simple example:
import 'package:test/test.dart';
void main() {
group('String manipulation tests', () {
test('String should be uppercased', () {
expect('hello'.toUpperCase(), equals('HELLO'));
});
test('String should be contain a substring', () {
expect('hello world'.contains('world'), isTrue);
});
});
}
In this example, we’ve created a group called “String manipulation tests” that contains two individual tests. The test()
function defines each test case, and the expect()
function asserts that the expected outcome is achieved.
Advanced Grouping Techniques
While basic grouping is useful, Dart’s testing framework offers more advanced features to further refine your test organization. These include nested groups, conditional test execution, and shared setup/teardown logic.
Nesting Test Groups
Nesting groups allows you to create a hierarchical structure that reflects the complexity of your application. You can have groups within groups, enabling fine-grained organization. For example:
import 'package:test/test.dart';
void main() {
group('User Authentication', () {
group('Login', () {
test('Successful login', () {
// Test successful login scenario
});
test('Failed login with invalid credentials', () {
// Test failed login scenario
});
});
group('Logout', () {
test('Successful logout', () {
// Test successful logout scenario
});
});
});
}
This example demonstrates a nested structure where “User Authentication” is the top-level group, and “Login” and “Logout” are subgroups. This allows for a very clear and organized representation of your tests.
Conditional Test Execution
Sometimes, you might want to run certain tests only under specific conditions. Dart’s testing framework allows you to conditionally execute tests based on various factors. For example, you might want to skip a test if it relies on a feature that is not yet implemented or if it’s known to fail in a particular environment. Dart testing supports skipping tests that are marked with a `skip` annotation. See more on how to optimize dartboard lighting after testing your visual acuity.
import 'package:test/test.dart';
void main() {
group('Database tests', () {
test('Connect to database', () {
// Test database connection
}, skip: 'Database connection not yet implemented');
test('Query database', () {
// Test database query
});
});
}
In this example, the “Connect to database” test is marked as skipped with a descriptive message. When the tests are run, this test will be skipped, and the message will be displayed in the test results.
Setup and Teardown
Often, tests within a group share common setup and teardown logic. Dart provides the setUp
and tearDown
functions to handle these tasks. These functions are executed before and after each test within the group, respectively. Similarly, setUpAll
and tearDownAll
are executed once before the first test and once after the last test in the group, respectively.
import 'package:test/test.dart';
void main() {
group('File processing tests', () {
setUpAll(() {
// Create a temporary file
print('Setting up file for tests');
});
tearDownAll(() {
// Delete the temporary file
print('Tearing down file after tests');
});
test('Read file content', () {
// Test reading file content
});
test('Write to file', () {
// Test writing to file
});
});
}
In this example, setUpAll
creates a temporary file before any tests are run, and tearDownAll
deletes the file after all tests have completed. This ensures that each test runs in a clean environment.
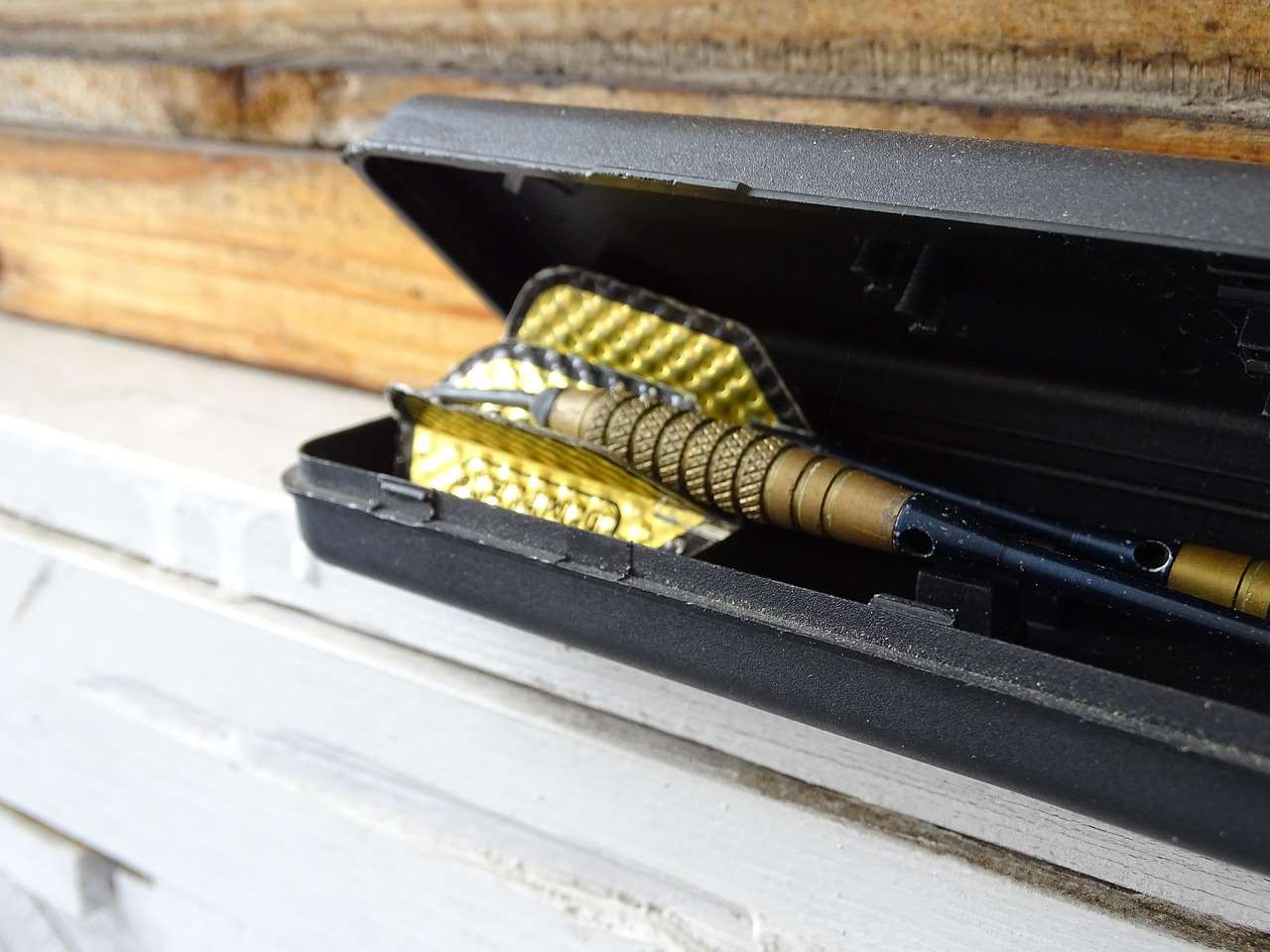
Best Practices for Effective Dart Testing For Grouping
To maximize the benefits of Dart Testing For Grouping, consider these best practices:
- Keep groups focused: Each group should focus on a specific area of functionality or a particular module.
- Use descriptive names: Group names should clearly indicate the purpose of the tests within the group.
- Avoid excessive nesting: While nesting can be useful, too much nesting can make your test suite difficult to navigate. Find a balance that works for your project.
- Use setup and teardown effectively: Leverage
setUp
,tearDown
,setUpAll
, andtearDownAll
to avoid code duplication and ensure a consistent testing environment. - Maintain test independence: Ensure that tests within a group do not depend on each other’s execution order or state.
By following these best practices, you can create a well-organized, maintainable, and efficient test suite using Dart’s grouping capabilities. For better visual acuity, check out the Optimal Dartboard Lighting Solutions Guide.
Example: Testing a Simple Class with Groups
Let’s consider a simple example of testing a Calculator
class using groups. The Calculator
class has methods for addition, subtraction, multiplication, and division. We can create a group for each operation to organize our tests.
class Calculator {
int add(int a, int b) => a + b;
int subtract(int a, int b) => a - b;
int multiply(int a, int b) => a * b;
double divide(int a, int b) => a / b;
}
import 'package:test/test.dart';
void main() {
final calculator = Calculator();
group('Calculator', () {
group('Addition', () {
test('2 + 2 should equal 4', () {
expect(calculator.add(2, 2), equals(4));
});
test('-1 + 1 should equal 0', () {
expect(calculator.add(-1, 1), equals(0));
});
});
group('Subtraction', () {
test('5 - 3 should equal 2', () {
expect(calculator.subtract(5, 3), equals(2));
});
test('10 - 5 should equal 5', () {
expect(calculator.subtract(10, 5), equals(5));
});
});
group('Multiplication', () {
test('3 * 4 should equal 12', () {
expect(calculator.multiply(3, 4), equals(12));
});
test('0 * 5 should equal 0', () {
expect(calculator.multiply(0, 5), equals(0));
});
});
group('Division', () {
test('10 / 2 should equal 5', () {
expect(calculator.divide(10, 2), equals(5));
});
test('6 / 3 should equal 2', () {
expect(calculator.divide(6, 3), equals(2));
});
});
});
}
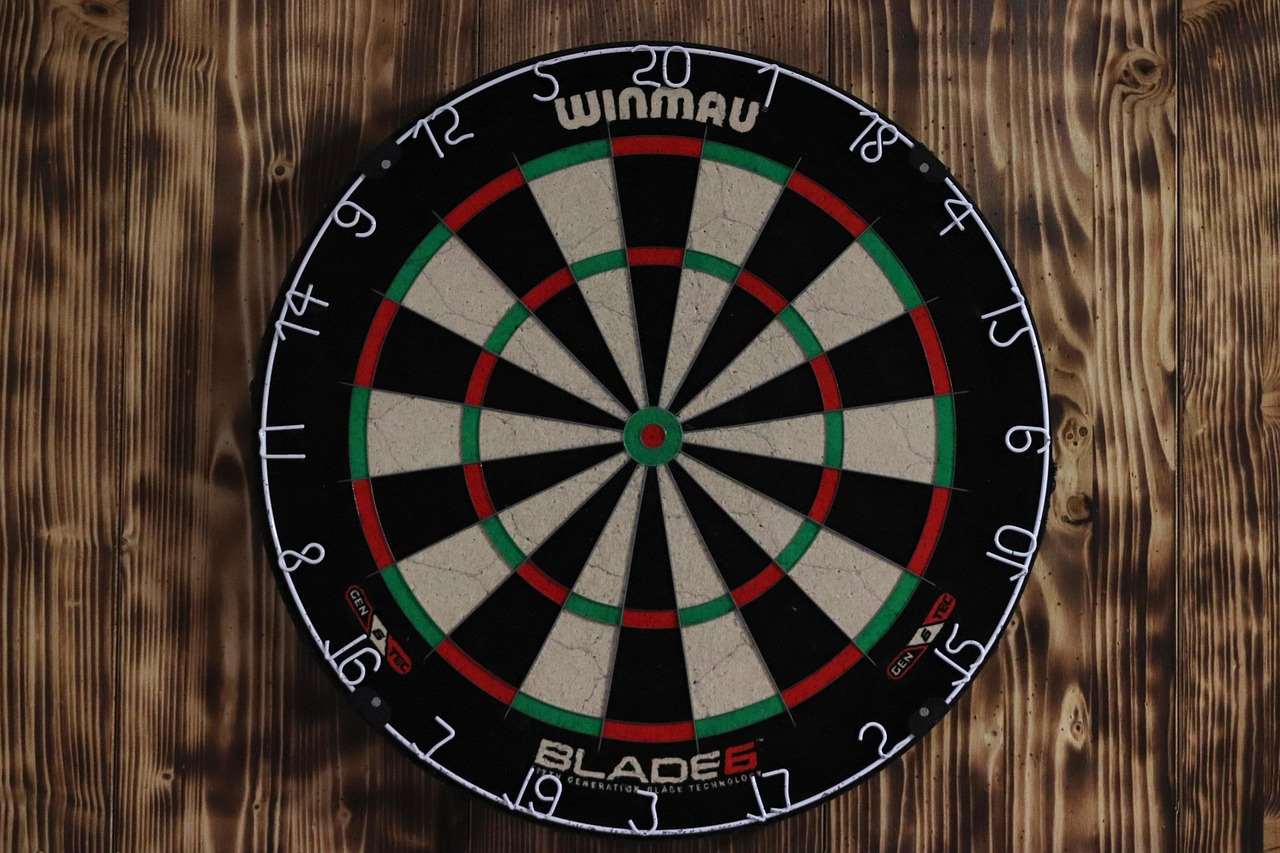
This example demonstrates how you can structure your tests to mirror the structure of your code. Each group focuses on a specific method of the Calculator
class, making it easy to find and maintain the tests. Test-driven development (TDD) benefits greatly from this type of structure.
Integrating Mocking with Test Groups
Mocking is a crucial technique in unit testing, allowing you to isolate the code under test by replacing its dependencies with controlled substitutes. When used in conjunction with test groups, mocking becomes even more powerful. You can create specific groups for tests that require mocking and set up the necessary mocks within the group’s setUp
function. It’s similar to how you light your dartboard–creating ideal conditions for success.
Consider a scenario where you’re testing a service that depends on an external API. You can use mocking to simulate the API’s behavior and avoid making real API calls during your tests. This makes your tests faster, more reliable, and less dependent on external factors.
The Future of Dart Testing
The Dart testing ecosystem is constantly evolving, with new tools and features being added regularly. As Dart continues to grow in popularity, we can expect to see even more sophisticated testing techniques and frameworks emerge. Staying up-to-date with the latest developments in Dart testing will help you write more effective and maintainable tests. Consider the Best Dartboard Lighting Systems as an analogy – continuous improvement ensures optimal performance.
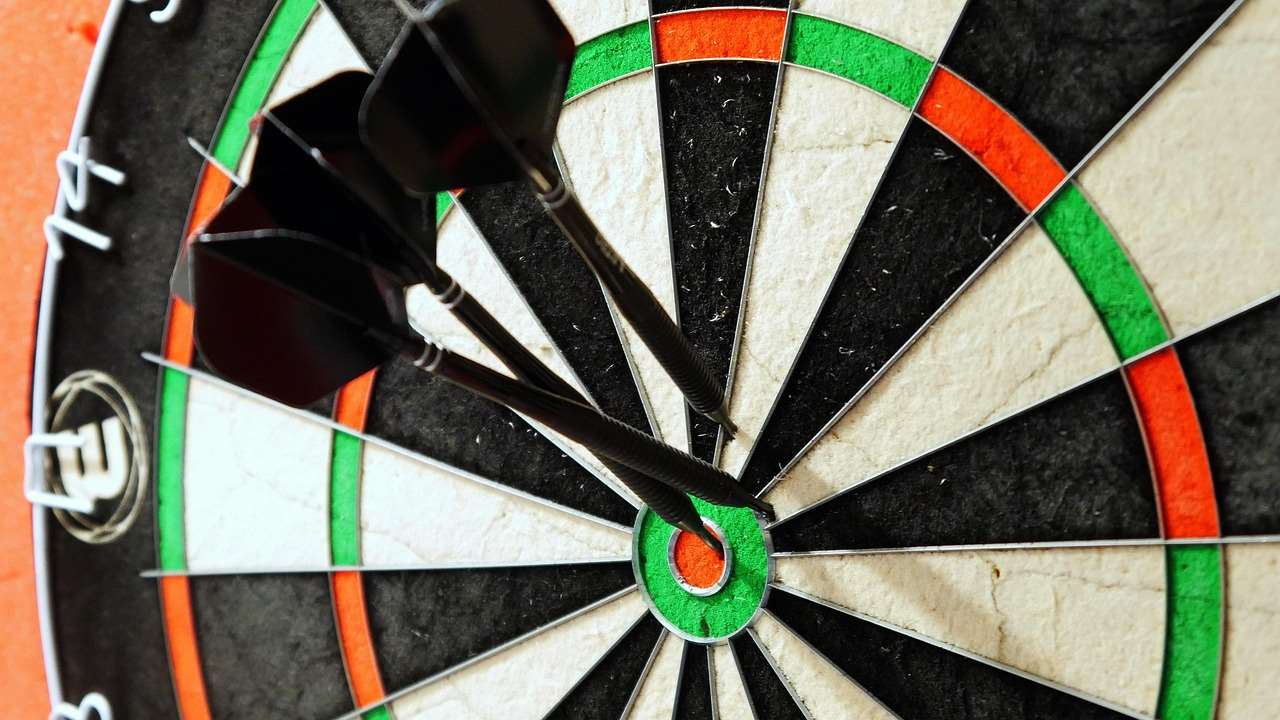
Troubleshooting Common Grouping Issues
While Dart Testing For Grouping is generally straightforward, you might encounter some common issues. One common problem is forgetting to import the test
package, which can lead to errors when using the group
, test
, and expect
functions. Another issue is incorrect setup or teardown logic, which can cause tests to fail intermittently. Carefully review your setUp
, tearDown
, setUpAll
, and tearDownAll
functions to ensure that they are performing the expected actions.
Another potential issue is overly complex group structures. While nesting groups can be useful, too much nesting can make your test suite difficult to navigate and understand. Strive for a balance between organization and simplicity. Don’t over complicate the groups.
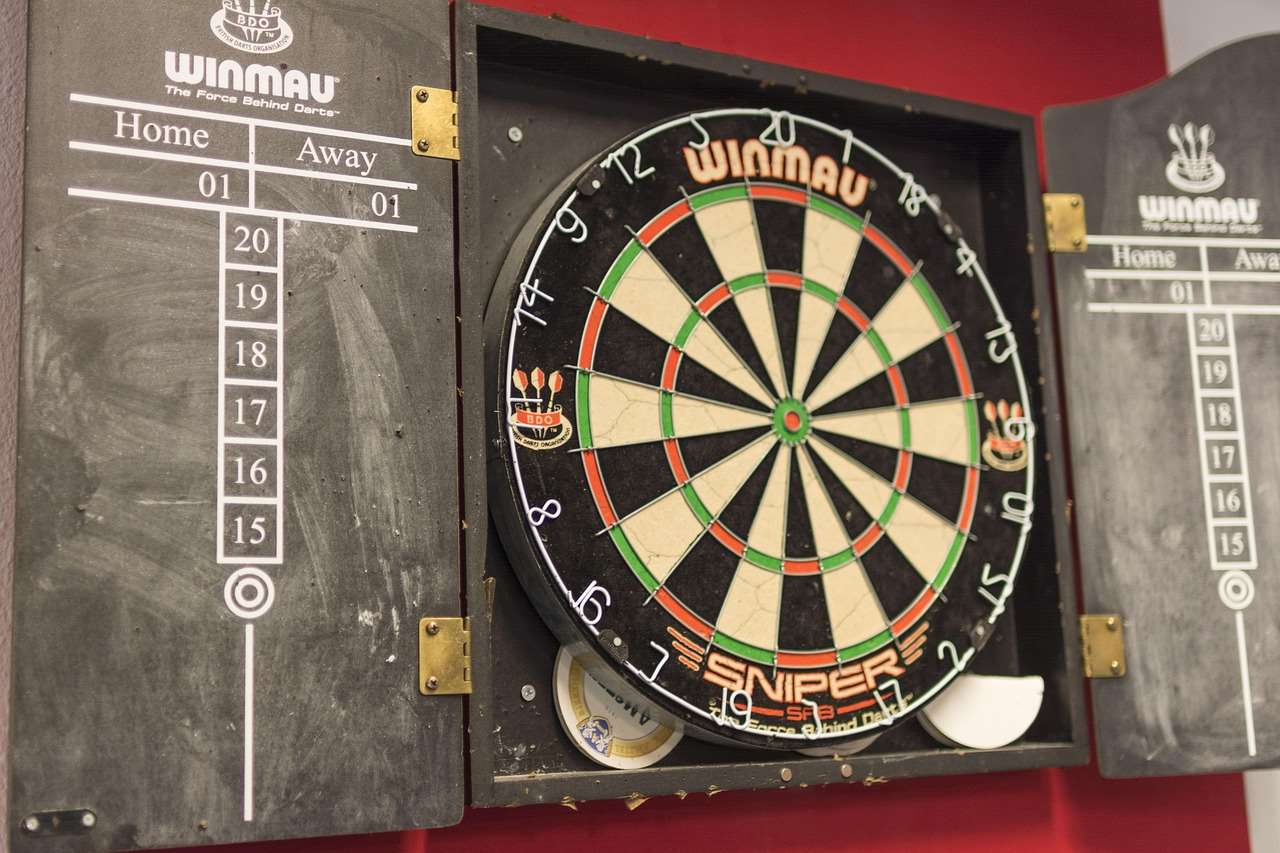
Conclusion
Dart Testing For Grouping is an essential technique for organizing and managing your test suite. By using groups effectively, you can improve the readability, maintainability, and efficiency of your tests. Remember to keep your groups focused, use descriptive names, avoid excessive nesting, and leverage setup and teardown logic. As you continue to develop your Dart applications, invest time in creating a well-structured and comprehensive test suite to ensure the quality and reliability of your code. Now that you have a solid understanding of how to group your tests effectively, why not explore the best dart equipment to complement your testing efforts? Happy testing!
Hi, I’m Dieter, and I created Dartcounter (Dartcounterapp.com). My motivation wasn’t being a darts expert – quite the opposite! When I first started playing, I loved the game but found keeping accurate scores and tracking stats difficult and distracting.
I figured I couldn’t be the only one struggling with this. So, I decided to build a solution: an easy-to-use application that everyone, no matter their experience level, could use to manage scoring effortlessly.
My goal for Dartcounter was simple: let the app handle the numbers – the scoring, the averages, the stats, even checkout suggestions – so players could focus purely on their throw and enjoying the game. It began as a way to solve my own beginner’s problem, and I’m thrilled it has grown into a helpful tool for the wider darts community.