Achieving Dart Testing For Consistency is crucial for building robust and reliable Dart applications; by implementing comprehensive tests, you ensure your code behaves as expected across different scenarios and future modifications. This article will explore various Dart testing techniques, tools, and best practices to help you maintain code quality and prevent unexpected errors, covering everything from unit testing to integration testing and end-to-end testing.
⚠️ Still Using Pen & Paper (or a Chalkboard)?! ⚠️
Step into the future! The Dart Counter App handles all the scoring, suggests checkouts, and tracks your stats automatically. It's easier than you think!
Try the Smart Dart Counter App FREE!Ready for an upgrade? Click above!
Why is Dart Testing For Consistency Important?
Consistency in software is paramount, and in the Dart ecosystem, achieving this through rigorous testing is non-negotiable. Without thorough testing, you risk introducing bugs and inconsistencies that can lead to unpredictable application behavior, data corruption, and ultimately, a poor user experience. Imagine building a complex Flutter application without adequate testing – you could face crashes, UI glitches, or incorrect data displays, leading to user frustration and negative reviews.
The benefits of Dart Testing For Consistency extend beyond simply finding bugs. Here are a few key advantages:
- Improved Code Quality: Writing tests forces you to think about your code’s design and functionality from a different perspective, leading to cleaner, more maintainable code.
- Reduced Debugging Time: By catching errors early in the development cycle, you significantly reduce the time spent debugging later on.
- Increased Confidence in Code Changes: When you have a comprehensive suite of tests, you can confidently make changes to your code knowing that you’ll be alerted if you introduce any regressions.
- Better Collaboration: Well-written tests serve as documentation for your code, making it easier for other developers to understand and contribute.
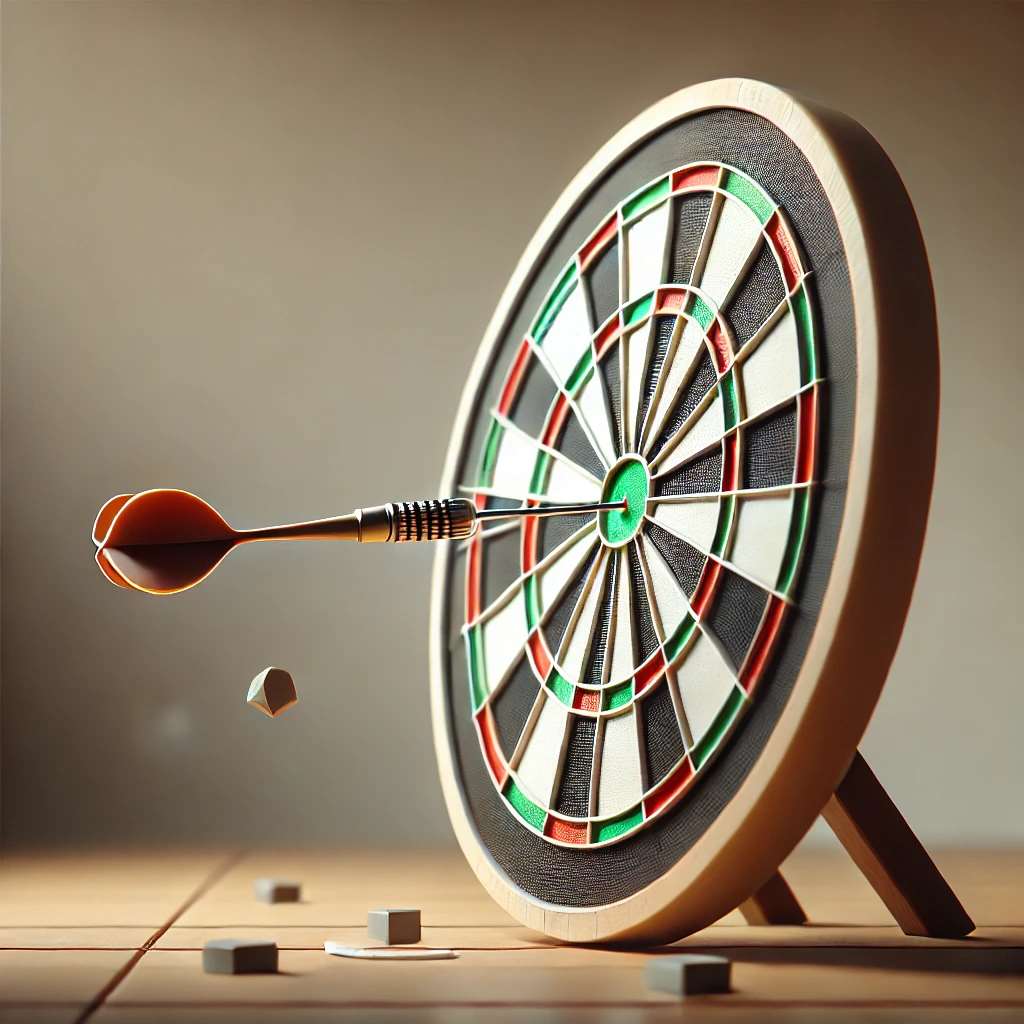
Types of Dart Tests
Dart offers a variety of testing options to suit different needs. Understanding the different types of tests is essential for creating a comprehensive testing strategy.
Unit Tests
Unit tests focus on testing individual components or functions in isolation. The goal is to verify that each unit of code performs its intended function correctly, independent of other parts of the application. These are typically small, fast, and easy to write.
For example, consider a simple function that calculates the sum of two numbers. A unit test would verify that the function returns the correct sum for various input values, including positive, negative, and zero values.
The `test` package in Dart provides a convenient way to write unit tests. Here’s a basic example:
import 'package:test/test.dart';
int add(int a, int b) {
return a + b;
}
void main() {
test('Test add function', () {
expect(add(2, 3), equals(5));
expect(add(-1, 1), equals(0));
expect(add(0, 0), equals(0));
});
}
Widget Tests (Flutter)
In Flutter, widget tests are used to verify the behavior of individual UI widgets. These tests allow you to simulate user interactions, such as tapping buttons or entering text, and verify that the widget responds correctly. They are essential for ensuring that your UI components render correctly and behave as expected. Using proper dart equipment can ensure consistent and reliable testing outcomes.
Here’s an example of a widget test:
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('MyWidget displays a message', (WidgetTester tester) async {
// Build our widget and trigger a frame.
await tester.pumpWidget(MaterialApp(home: Text('Hello, World!')));
// Verify that our widget displays the correct message.
expect(find.text('Hello, World!'), findsOneWidget);
});
}
Integration Tests
Integration tests verify the interaction between different parts of your application or between your application and external services. These tests are more comprehensive than unit tests and help to ensure that your application functions correctly as a whole.
For example, you might write an integration test to verify that your application can successfully connect to a database, retrieve data, and display it in the UI.
End-to-End Tests
End-to-end (E2E) tests simulate real user scenarios and verify that your application functions correctly from start to finish. These tests typically involve automating user interactions with the application’s UI and verifying that the application behaves as expected. E2E tests are often used to test critical user flows, such as user registration, login, and checkout.
Tools like Flutter Driver or third-party solutions like Selenium can be used for E2E testing in Flutter applications.
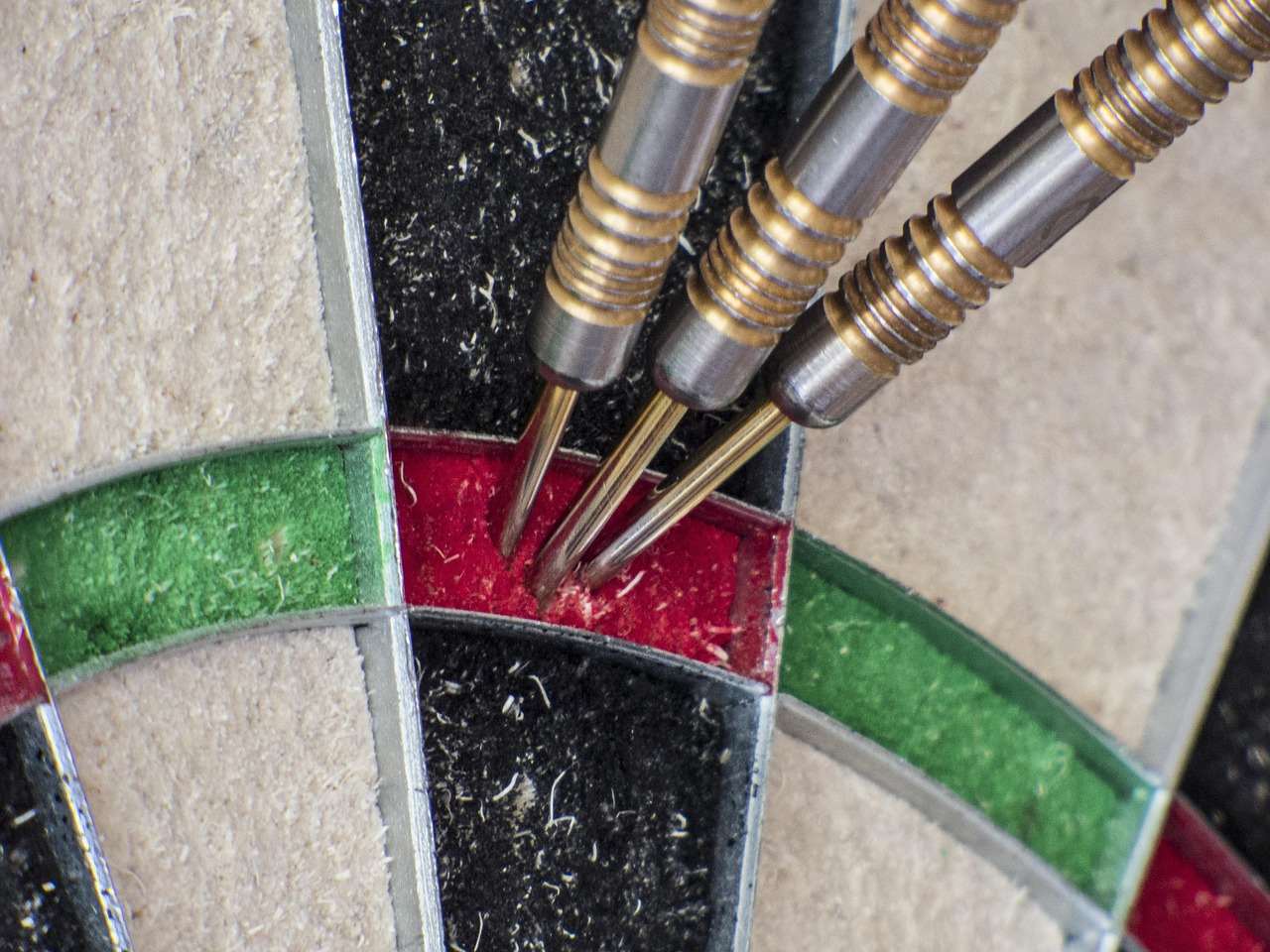
Best Practices for Dart Testing For Consistency
To maximize the benefits of Dart Testing For Consistency, it’s important to follow some best practices:
- Write tests early and often: Don’t wait until the end of the development cycle to write tests. Write tests as you write your code, and run them frequently to catch errors early.
- Follow the AAA pattern (Arrange, Act, Assert): Structure your tests clearly by following the AAA pattern. Arrange your test data, act by executing the code you want to test, and assert that the results are as expected.
- Use descriptive test names: Give your tests meaningful names that clearly describe what they are testing. This makes it easier to understand the purpose of each test and to identify failing tests quickly.
- Keep your tests small and focused: Each test should focus on testing a single aspect of your code. This makes it easier to identify the root cause of failures and to maintain your tests over time.
- Use mocks and stubs to isolate your tests: When testing complex components, use mocks and stubs to isolate your tests from external dependencies. This makes your tests more reliable and easier to maintain.
- Strive for high test coverage: Aim to achieve high test coverage for your codebase. This means writing tests for as much of your code as possible, including edge cases and error conditions.
Consider the Best Dartboard Lighting Systems as an analogy – just as consistent lighting is essential for a fair game, consistent testing is crucial for reliable software.
Tools and Libraries for Dart Testing
Dart has a rich ecosystem of tools and libraries to support testing. Here are some of the most popular ones:
- test: The core testing library for Dart, providing the foundation for writing unit tests, integration tests, and widget tests.
- flutter_test: A library specifically for testing Flutter widgets. It provides a rich set of tools for simulating user interactions and verifying widget behavior.
- mocktail and Mockito: Libraries for creating mocks and stubs, which are essential for isolating your tests from external dependencies.
- coverage: A tool for measuring code coverage, helping you identify areas of your code that are not adequately tested.
- Flutter Driver: A tool for writing end-to-end tests for Flutter applications.
Leveraging these tools effectively is vital for Dart Testing For Consistency.
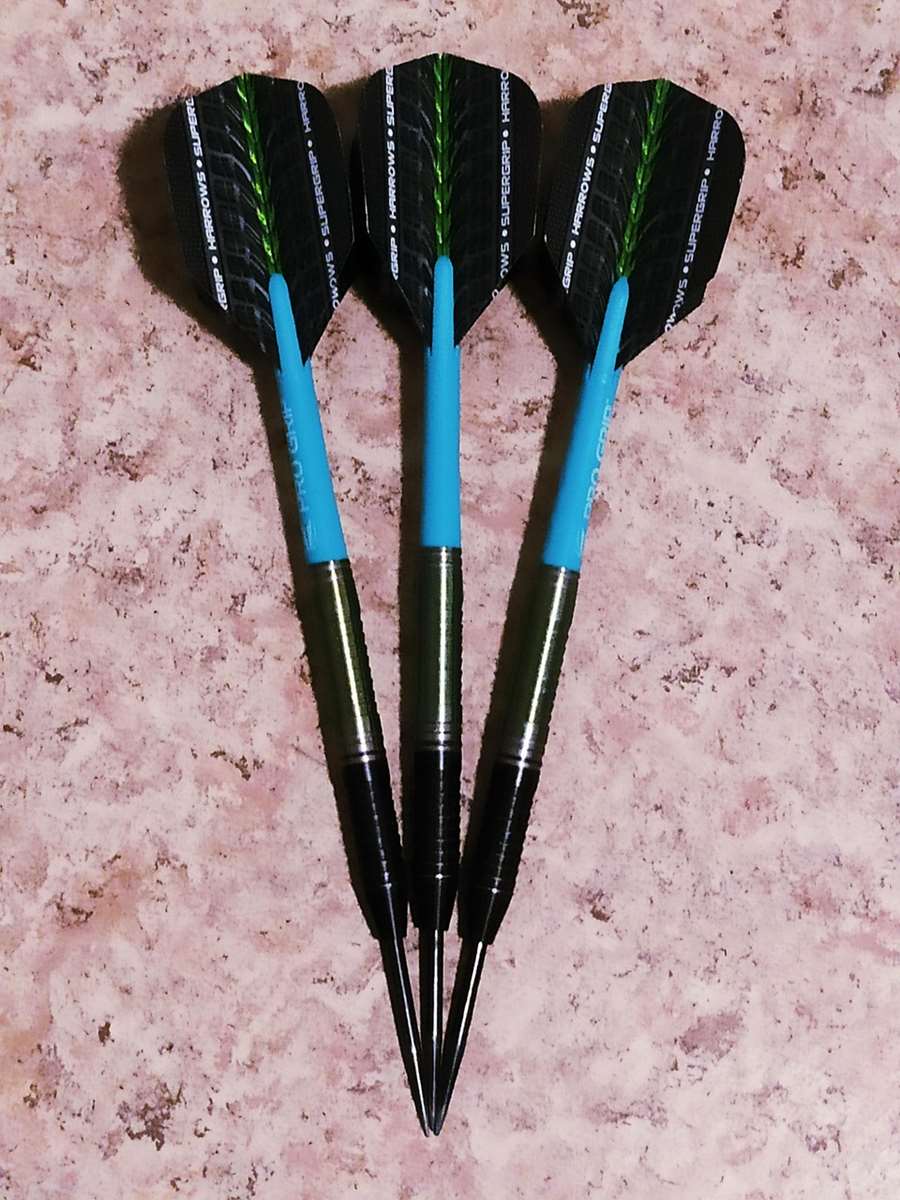
Implementing Mocking in Dart
Mocking is a powerful technique used in testing to isolate the unit being tested from its dependencies. This is especially useful when dealing with external resources like databases, APIs, or complex objects. By creating mock objects that mimic the behavior of these dependencies, you can ensure that your tests are predictable, reliable, and fast.
Libraries like `mocktail` and `Mockito` simplify the process of creating and using mocks in Dart. Here’s a basic example using `mocktail`:
import 'package:mocktail/mocktail.dart';
import 'package:test/test.dart';
class MyDependency {
String fetchData() {
return 'Real data';
}
}
class MockMyDependency extends Mock implements MyDependency {}
class MyClass {
final MyDependency dependency;
MyClass(this.dependency);
String getData() {
return dependency.fetchData();
}
}
void main() {
test('MyClass getData uses mocked dependency', () {
final mockDependency = MockMyDependency();
when(() => mockDependency.fetchData()).thenReturn('Mocked data');
final myClass = MyClass(mockDependency);
expect(myClass.getData(), equals('Mocked data'));
});
}
In this example, `MockMyDependency` is a mock object that replaces the real `MyDependency`. The `when` function is used to define the behavior of the mock object, ensuring that it returns ‘Mocked data’ when `fetchData` is called. This allows us to test `MyClass` in isolation, without relying on the actual implementation of `MyDependency`.
Dart Testing For Consistency and Continuous Integration (CI)
Integrating Dart Testing For Consistency into your CI/CD pipeline is critical for maintaining code quality throughout the development lifecycle. CI systems like GitHub Actions, GitLab CI, and Travis CI can be configured to automatically run your tests whenever changes are pushed to your repository. This ensures that any regressions are detected early, before they make their way into production.
To integrate tests into your CI/CD pipeline, you typically need to define a configuration file that specifies the steps required to build your application and run your tests. This file will vary depending on the CI system you are using. However, the basic steps are usually the same:
- Check out the code from the repository.
- Install the necessary dependencies.
- Run the tests.
- Report the test results.
By automating your testing process, you can ensure that your code is always tested thoroughly, regardless of who is making changes or how frequently changes are being made.
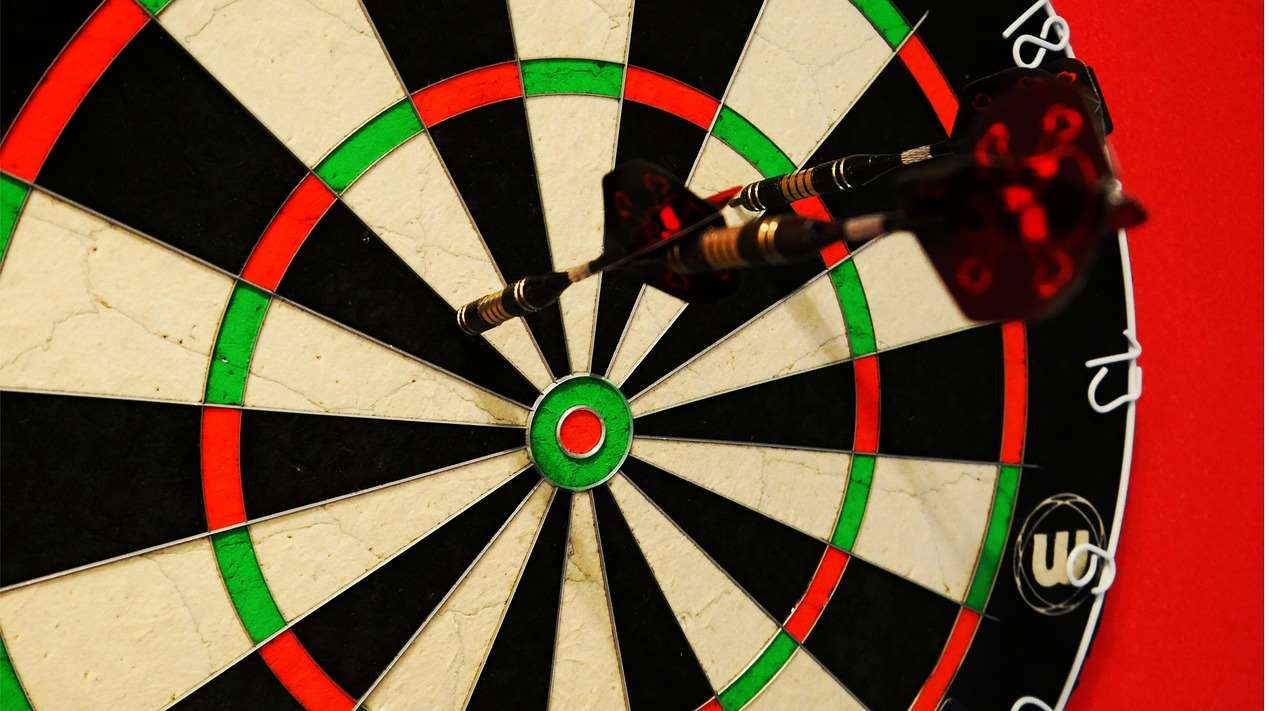
Advanced Testing Techniques
Beyond the basics, several advanced testing techniques can further enhance the quality and reliability of your Dart applications.
Property-Based Testing
Property-based testing involves defining properties or invariants that should always hold true for your code, and then automatically generating a large number of test cases to verify that these properties are indeed satisfied. This approach can be particularly effective for testing complex algorithms or data structures.
Mutation Testing
Mutation testing involves introducing small changes (mutations) to your code and then running your tests to see if they catch these mutations. If your tests are effective, they should fail when a mutation is introduced. If your tests don’t fail, it indicates that your tests are not comprehensive enough and need to be improved. This process ensures you catch errors and prevent potential issues with code maintainability.
Golden Tests
Golden tests, also known as snapshot tests, involve comparing the output of your code against a known “golden” output. This is particularly useful for testing UI components, where it can be difficult to write assertions that accurately capture the expected rendering. Golden tests can help you detect subtle changes in your UI that might otherwise go unnoticed.
Common Pitfalls to Avoid
Even with the best tools and techniques, it’s easy to fall into common testing pitfalls. Here are a few to watch out for:
- Writing tests that are too tightly coupled to the implementation: This makes your tests brittle and prone to breaking whenever the implementation changes, even if the functionality remains the same.
- Ignoring edge cases and error conditions: Make sure your tests cover all possible scenarios, including edge cases and error conditions.
- Writing tests that are too slow: Slow tests can discourage developers from running them frequently, which can lead to regressions going undetected for longer.
- Failing to maintain your tests: Tests need to be updated as your code changes. Failing to do so can lead to tests that are no longer relevant or that fail incorrectly.
Avoiding these pitfalls ensures the continued effectiveness of Dart Testing For Consistency efforts.
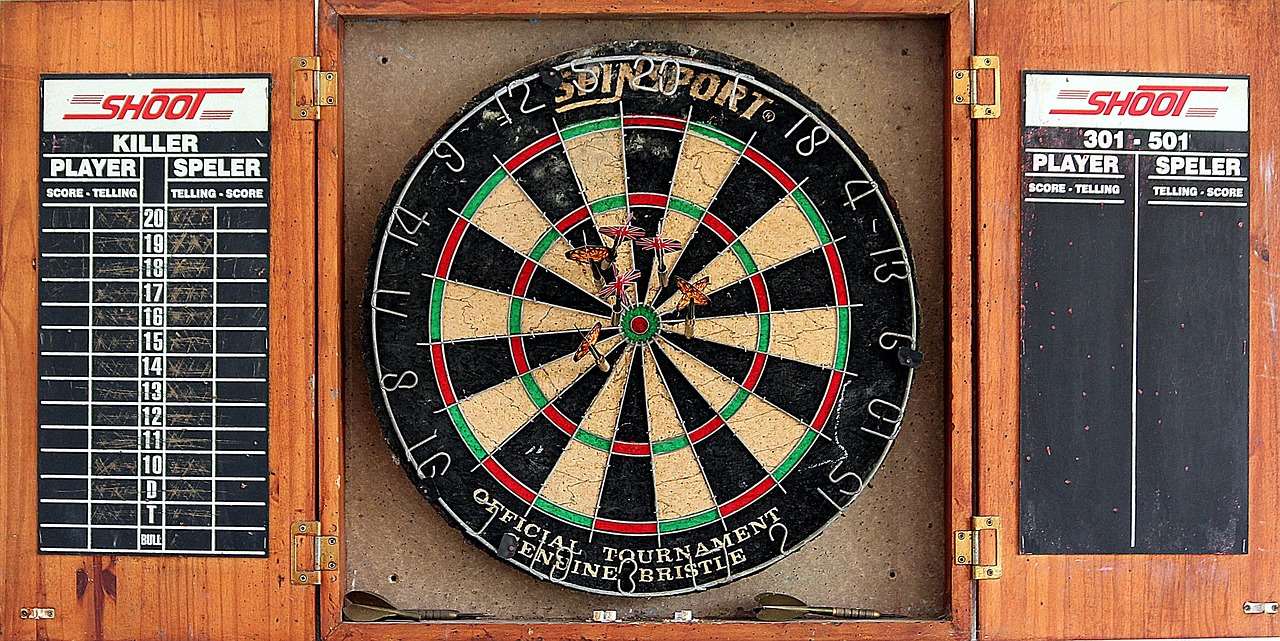
Conclusion
Dart Testing For Consistency is not merely an optional step in software development; it’s an indispensable practice for creating robust, reliable, and maintainable applications. By implementing a comprehensive testing strategy that includes unit tests, widget tests, integration tests, and end-to-end tests, you can significantly reduce the risk of bugs, improve code quality, and increase your confidence in code changes. Embracing best practices, leveraging available tools and libraries, and integrating testing into your CI/CD pipeline are key to achieving consistent and predictable application behavior. Remember that a strong testing foundation directly correlates with a positive user experience and a successful product. Start implementing or refining your testing strategy today – your future self (and your users) will thank you for it. Now that you have explored the depths of Dart Testing For Consistency, consider expanding your expertise and how to light your dartboard and also get the dart equipment.
Hi, I’m Dieter, and I created Dartcounter (Dartcounterapp.com). My motivation wasn’t being a darts expert – quite the opposite! When I first started playing, I loved the game but found keeping accurate scores and tracking stats difficult and distracting.
I figured I couldn’t be the only one struggling with this. So, I decided to build a solution: an easy-to-use application that everyone, no matter their experience level, could use to manage scoring effortlessly.
My goal for Dartcounter was simple: let the app handle the numbers – the scoring, the averages, the stats, even checkout suggestions – so players could focus purely on their throw and enjoying the game. It began as a way to solve my own beginner’s problem, and I’m thrilled it has grown into a helpful tool for the wider darts community.