Adapting your Dart testing strategy after a technique change is crucial to ensure your code still works as expected and that the new approach doesn’t introduce regressions; the key is to understand the impact of your changes on existing tests and adjust them accordingly. This article explores how to effectively approach Dart Testing After Technique Change, covering adaptation strategies, refactoring considerations, and best practices for maintaining robust test suites.
⚠️ Still Using Pen & Paper (or a Chalkboard)?! ⚠️
Step into the future! The Dart Counter App handles all the scoring, suggests checkouts, and tracks your stats automatically. It's easier than you think!
Try the Smart Dart Counter App FREE!Ready for an upgrade? Click above!
Understanding the Impact of Technique Change on Your Dart Tests
When you introduce a technique change in your Dart codebase, it’s essential to understand the potential impact on your existing tests. This involves analyzing how the change affects different parts of your application and identifying which tests might need modification or even complete rewriting. A key part of Dart Testing After Technique Change is identifying which tests should change and how much they need to be modified.
Start by creating a list of all modules and components that are touched or affected by the technique change. Then, trace back to the unit and integration tests that cover those components. Ask yourself these questions:
- Does the change affect the public API of the component?
- Does the change alter the internal behavior or data structures of the component?
- Does the change introduce new dependencies or remove existing ones?
The answers to these questions will help you prioritize which tests to focus on and determine the extent of the necessary modifications. This is a critical step in Dart Testing After Technique Change.

Adapting Your Dart Tests After a Technique Change
Once you’ve identified the affected tests, the next step is to adapt them to the new technique. This might involve updating assertions, mocking different dependencies, or even rewriting entire test cases. Consider these strategies:
Updating Assertions
If the technique change alters the expected output or behavior of a function or component, you’ll need to update the assertions in your tests to reflect the new reality. This can be as simple as changing the expected value in an expect
statement, or as complex as rewriting the entire assertion logic.
Refactoring Test Code
Sometimes, the technique change might require you to refactor your test code to make it more maintainable or to better reflect the new structure of your application. This could involve extracting common test logic into helper functions, or reorganizing your test files to better align with the new component structure. Effective refactoring ensures that your tests remain clean and easy to understand, even after significant changes. Remember, maintainable tests are crucial. You can also consider the Choose Best Dart Equipment to help maintain your focus.
Mocking New Dependencies
If the technique change introduces new dependencies, you’ll need to mock those dependencies in your tests to isolate the component under test. This can be done using mocking frameworks like mockito
or mocktail
. Proper mocking ensures that your tests are focused on the behavior of the component itself, rather than the behavior of its dependencies.
Refactoring Considerations for Dart Testing After Technique Change
When adapting your Dart tests after a technique change, it’s important to consider the impact on your existing test suite. Here are some key refactoring considerations:
Maintaining Test Coverage
Ensure that your test suite still provides adequate coverage of your code after the technique change. Use code coverage tools to identify any gaps in your test coverage and write new tests to fill those gaps. Aim for high test coverage to minimize the risk of introducing regressions.
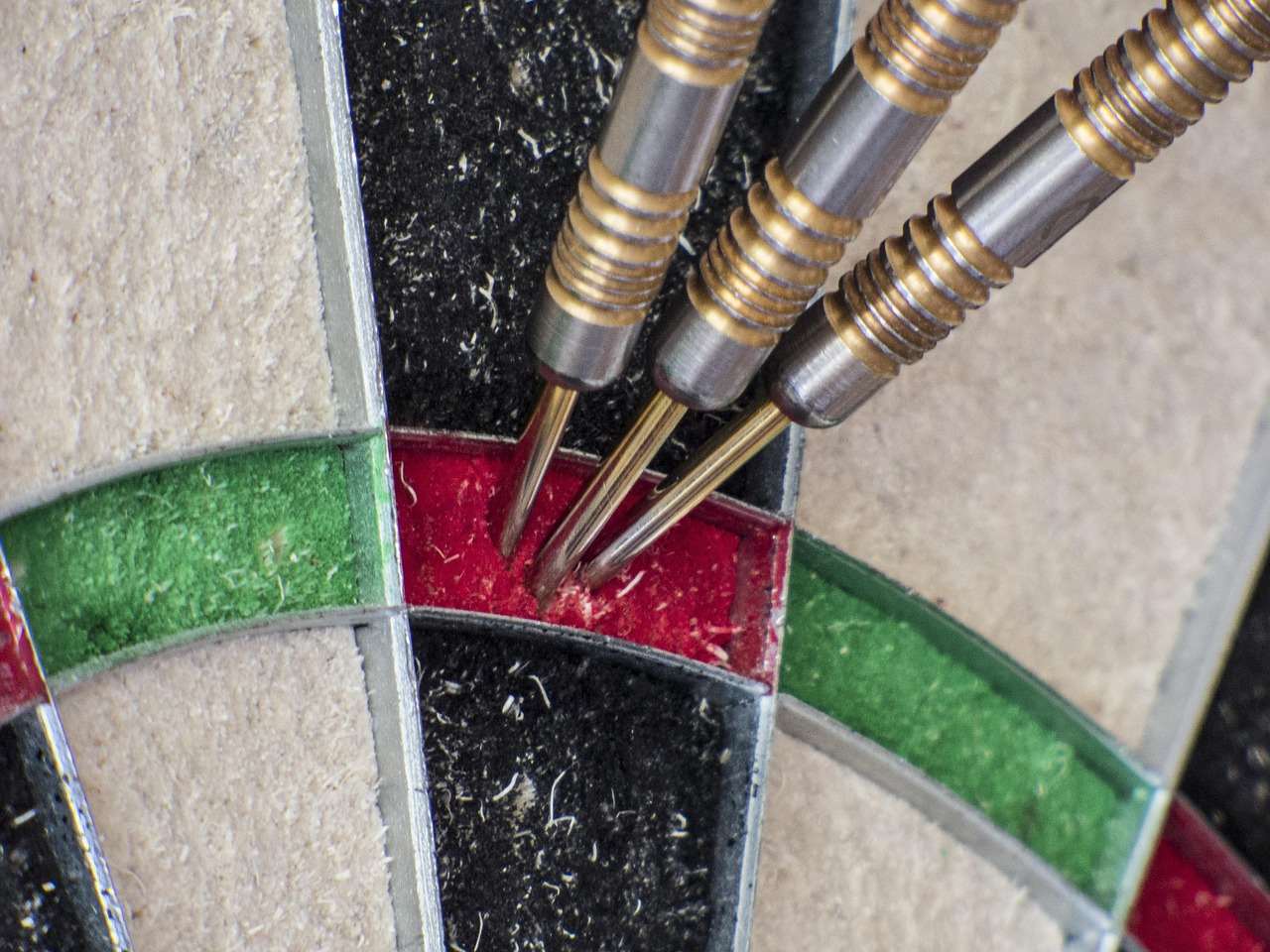
Avoiding Over-Specification
Be careful not to over-specify your tests by asserting on implementation details that are likely to change in the future. Instead, focus on asserting on the public API and the overall behavior of your components. This will make your tests more resilient to future changes and reduce the need for frequent updates.
Using Parameterized Tests
Parameterized tests allow you to run the same test case with multiple different inputs, which can be useful for testing different scenarios or edge cases. This can help you reduce code duplication and improve the maintainability of your test suite. By using parameterized tests you are able to provide a robust testing environment that will help identify any errors that the change has introduced.
Integration and End-to-End Testing
While unit tests are great for isolating individual components, it’s also important to have integration and end-to-end tests to verify that your application works correctly as a whole. These tests can help you catch issues that might not be apparent from unit tests alone. Integration testing is extremely important when doing Dart Testing After Technique Change because it ensures that your application still works with the new technology.
Best Practices for Robust Dart Tests After Code Modification
To ensure your Dart tests remain robust and effective after a technique change, adhere to these best practices:
Write Testable Code
Design your code with testability in mind. This means writing code that is modular, loosely coupled, and easy to mock. Use dependency injection to make it easier to swap out dependencies in your tests. Code that is hard to test often indicates a design flaw. Think about how How To Light Your Dartboard can lead to a clearer view of the target.
Follow the Arrange-Act-Assert Pattern
Organize your test cases using the Arrange-Act-Assert pattern. This makes your tests more readable and easier to understand. The “Arrange” section sets up the test environment, the “Act” section executes the code under test, and the “Assert” section verifies the expected results.
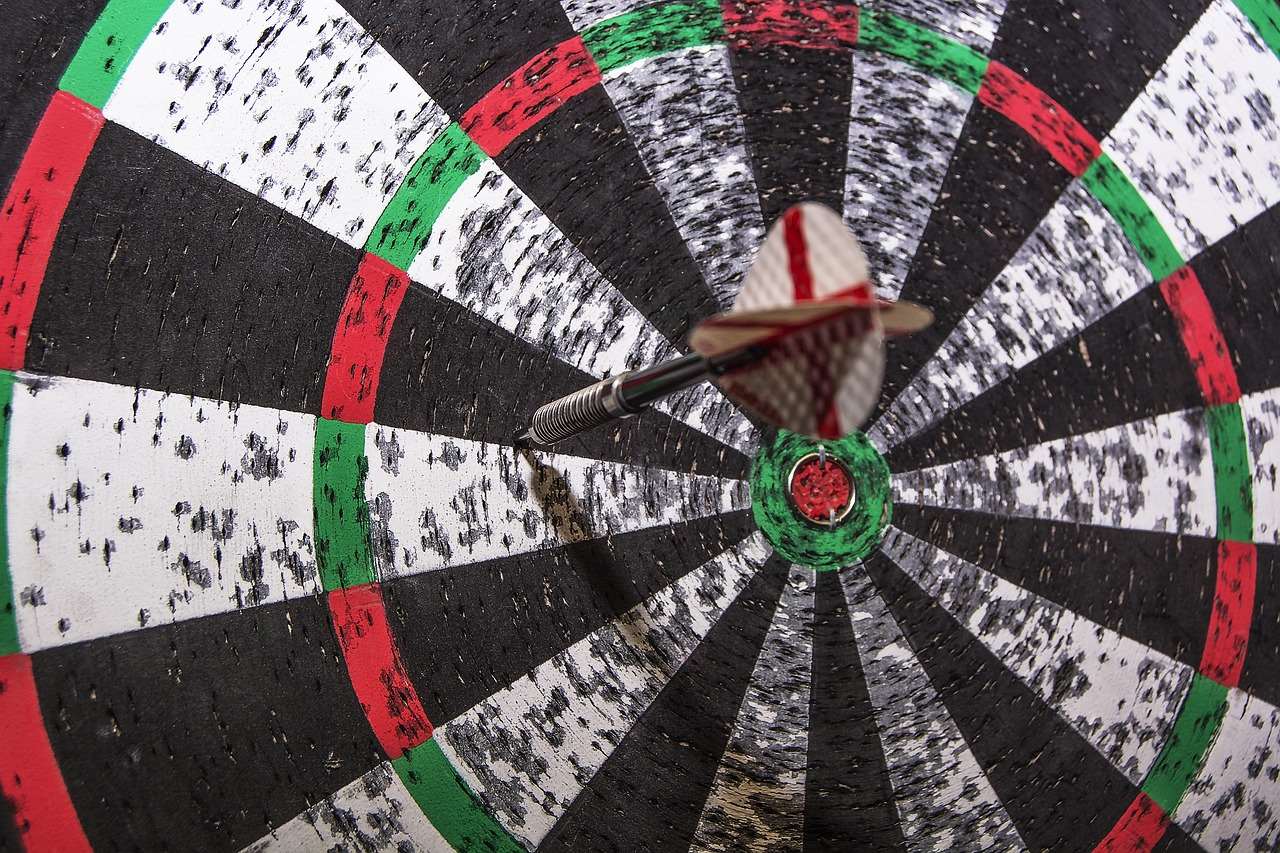
Keep Tests Independent
Ensure that your tests are independent of each other and do not rely on shared state. This will prevent tests from interfering with each other and make it easier to run your tests in parallel. You want each test to act in isolation so that they don’t cause any unforeseen problems. When you’re in the middle of Dart Testing After Technique Change, you don’t want problems from other tests confusing you.
Use Meaningful Test Names
Give your test cases descriptive and meaningful names that clearly indicate what they are testing. This will make it easier to understand the purpose of each test and to identify failures. Good test names serve as documentation for your code.
Automate Your Tests
Integrate your tests into your continuous integration (CI) pipeline so that they are run automatically every time you commit code. This will help you catch regressions early and prevent them from making their way into production. Automation is vital. A great start to a CI pipeline is the Types Optimal Dartboard Lighting.
Regularly Review Your Tests
Make time to regularly review your test suite and identify any tests that are obsolete, redundant, or no longer relevant. Remove these tests to keep your test suite lean and maintainable. Tests, like code, need maintenance. In Dart Testing After Technique Change, your tests will need to be constantly updated.
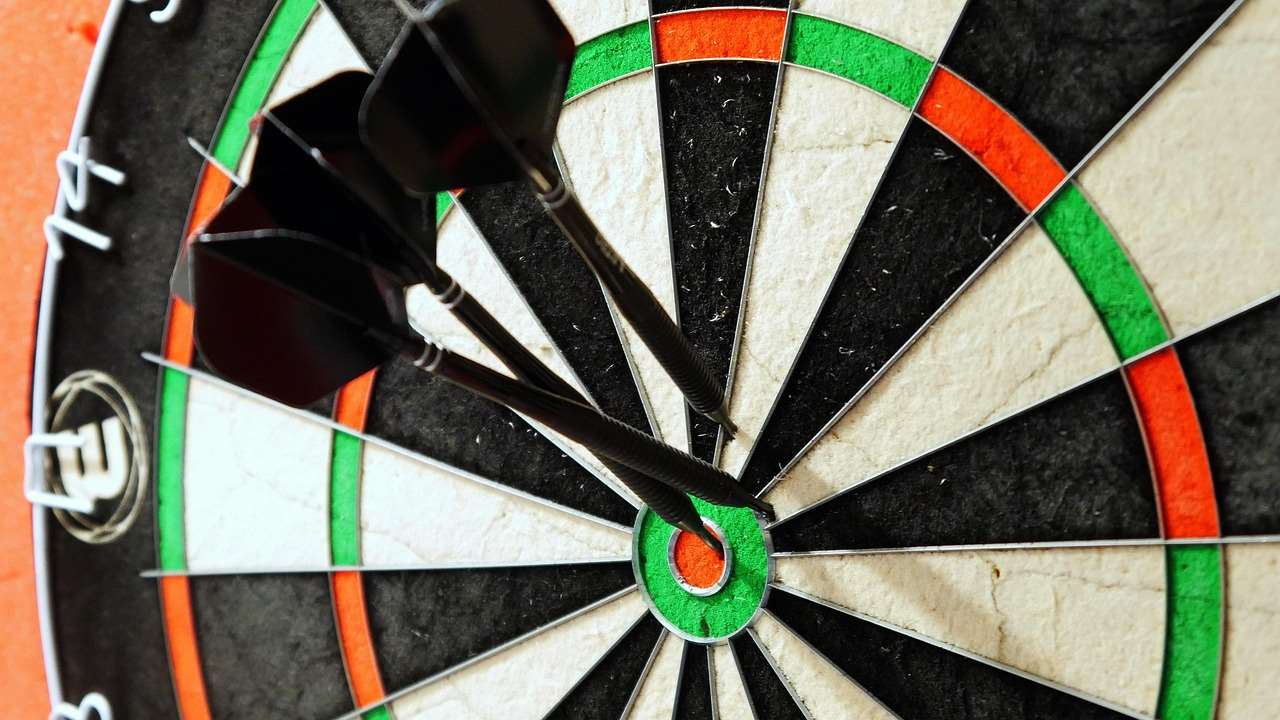
Tools and Frameworks for Dart Testing
Dart provides a rich ecosystem of testing tools and frameworks to help you write effective tests:
test
package: The official testing framework for Dart, providing a simple and intuitive API for writing unit tests, integration tests, and end-to-end tests.mockito
package: A powerful mocking framework that allows you to create mock objects and verify interactions with dependencies.mocktail
package: Another popular mocking framework with a focus on simplicity and ease of use.coverage
package: A tool for measuring code coverage and identifying gaps in your test suite.flutter_test
package: Provides testing support for Flutter applications, including widget testing and integration testing.
Choosing the right tools and frameworks can significantly improve your testing workflow and help you write more effective tests. When doing Dart Testing After Technique Change, you need tools to help you identify problems within your code.
Real-World Examples of Adapting Dart Tests
Let’s look at some real-world examples of how to adapt Dart tests after a technique change:
Example 1: Replacing a REST API with a GraphQL API
If you’re replacing a REST API with a GraphQL API, you’ll need to update your tests to reflect the new way of fetching data. This might involve changing your mock responses to return GraphQL data instead of REST data, and updating your assertions to verify the structure and content of the GraphQL responses. You’ll also need to mock the GraphQL client instead of the HTTP client. Keep in mind Best Dartboard Lighting Systems, as the clearer the target, the more accurate the results.
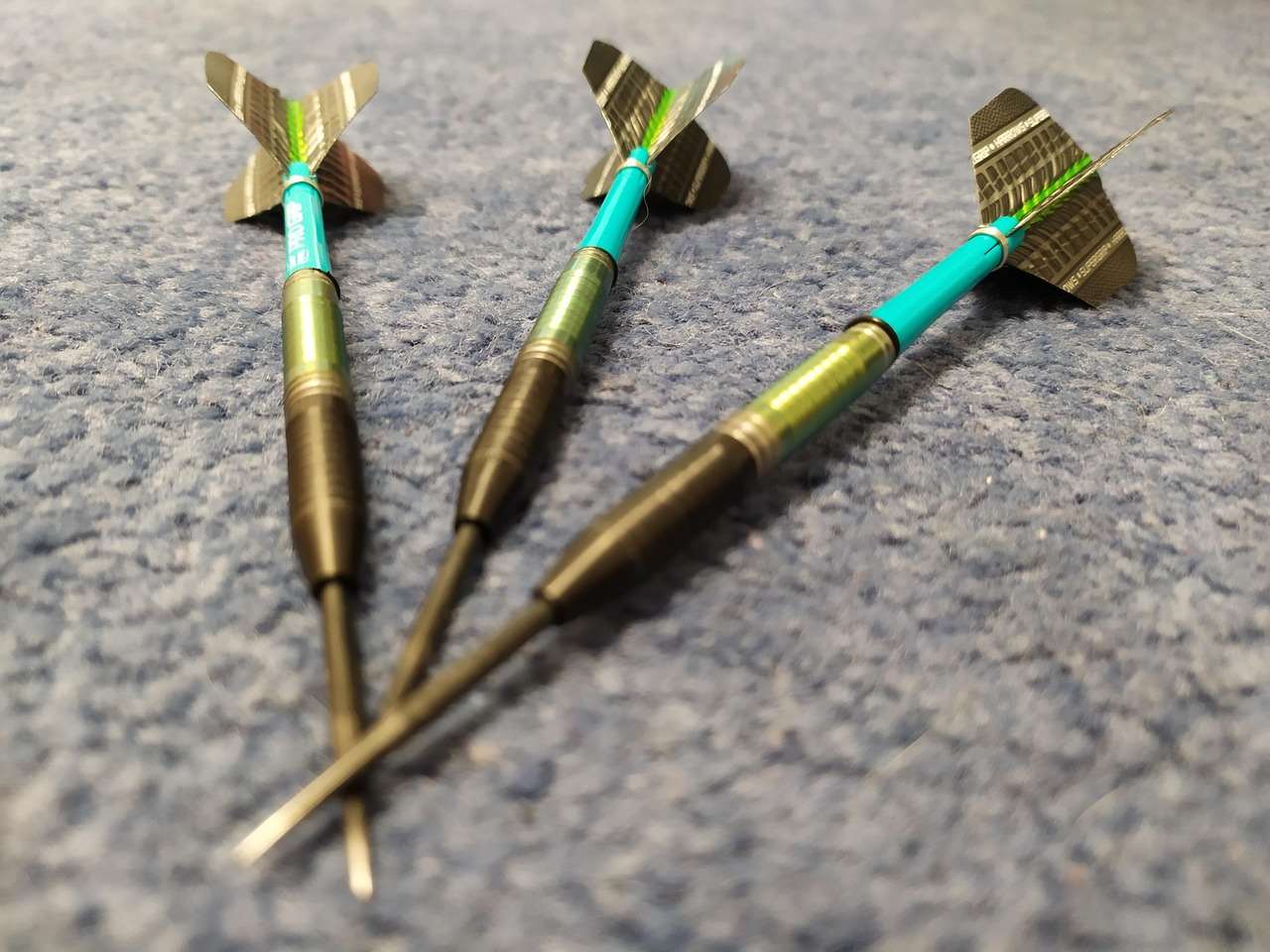
Example 2: Migrating from Streams to Async/Await
If you’re migrating from Streams to Async/Await, you’ll need to update your tests to use await
instead of listening to Stream events. This might involve changing your test code to call await
on asynchronous functions and verifying the returned values. You’ll also need to handle any exceptions that might be thrown by the asynchronous functions.
These examples illustrate the types of changes you might need to make to your Dart tests after a technique change. The key is to carefully analyze the impact of the change on your existing code and update your tests accordingly.
Conclusion
Dart Testing After Technique Change is a critical aspect of software development. By understanding the impact of your changes, adapting your tests accordingly, and following best practices, you can ensure that your Dart applications remain robust and reliable. Remember to prioritize test coverage, avoid over-specification, and regularly review your test suite. Embrace automation and leverage the available tools and frameworks to streamline your testing workflow. By making testing an integral part of your development process, you can build high-quality Dart applications that meet the needs of your users. Now that you understand how to test after a technique change, go forth and build great Dart applications!
Hi, I’m Dieter, and I created Dartcounter (Dartcounterapp.com). My motivation wasn’t being a darts expert – quite the opposite! When I first started playing, I loved the game but found keeping accurate scores and tracking stats difficult and distracting.
I figured I couldn’t be the only one struggling with this. So, I decided to build a solution: an easy-to-use application that everyone, no matter their experience level, could use to manage scoring effortlessly.
My goal for Dartcounter was simple: let the app handle the numbers – the scoring, the averages, the stats, even checkout suggestions – so players could focus purely on their throw and enjoying the game. It began as a way to solve my own beginner’s problem, and I’m thrilled it has grown into a helpful tool for the wider darts community.