A dart singleton class ensures you have only one instance of a class throughout your application’s lifecycle. This is crucial for managing resources, maintaining state, and preventing unintended side effects. This article will delve into the intricacies of creating and utilizing a dart singleton class, exploring different implementation techniques and best practices.
⚠️ Still Using Pen & Paper (or a Chalkboard)?! ⚠️
Step into the future! The Dart Counter App handles all the scoring, suggests checkouts, and tracks your stats automatically. It's easier than you think!
Try the Smart Dart Counter App FREE!Ready for an upgrade? Click above!
Let’s start by understanding the fundamental concept. A singleton pattern is a design pattern that restricts the instantiation of a class to one “single” instance. This is particularly useful when you need to control access to a shared resource, such as a database connection or a configuration file. In Dart, implementing this pattern efficiently is vital for building robust and scalable applications. We’ll cover various approaches to creating a dart singleton class, discussing their advantages and disadvantages.
The benefits of using a dart singleton class extend beyond simply managing resources; it also streamlines code by providing a single point of access to shared functionalities. Imagine managing a user’s profile information. Instead of having multiple instances of a `UserProfile` class scattered throughout your application, a singleton ensures a consistent and unified view of the user’s data. This makes your code easier to manage, understand, and debug.
This article will explore several implementation methods for a dart singleton class, compare their effectiveness, and offer insights into choosing the best approach for different scenarios. You will learn how to write clean, efficient, and testable singleton classes, and you’ll discover best practices to avoid common pitfalls. We will also touch upon more advanced concepts and how to handle potential issues that can arise from improper singleton implementation. Let’s get started!

Understanding the Dart Singleton Class
Before diving into the code, let’s solidify our understanding of what constitutes a dart singleton class. At its core, it’s a class that restricts the instantiation of new objects to just one instance. This instance is typically accessed through a static method or getter. This prevents multiple instances from accidentally interfering with each other, often crucial when dealing with resources that should only be accessed and managed in a singular fashion.
Why Use a Singleton?
- Controlled Resource Access: Singletons ensure only one instance accesses a shared resource, preventing conflicts and inconsistencies. Think of a database connection—multiple simultaneous connections can lead to performance issues or data corruption. A dart singleton class for the database connection elegantly solves this problem.
- Global State Management: Managing application-wide state is simplified. A singleton can hold and manage state that needs to be accessible from anywhere in the application.
- Reduced Complexity: By providing a single point of access, singletons can reduce code complexity and improve maintainability.
- Improved Testability: While often debated, well-designed singletons can be easily tested using dependency injection techniques.
However, overusing singletons can lead to tight coupling and make testing difficult. Therefore, careful consideration of whether a singleton is truly necessary for a given task is essential. Using a darts how to guide may provide similar results. Remember that other design patterns may be more appropriate in certain situations.
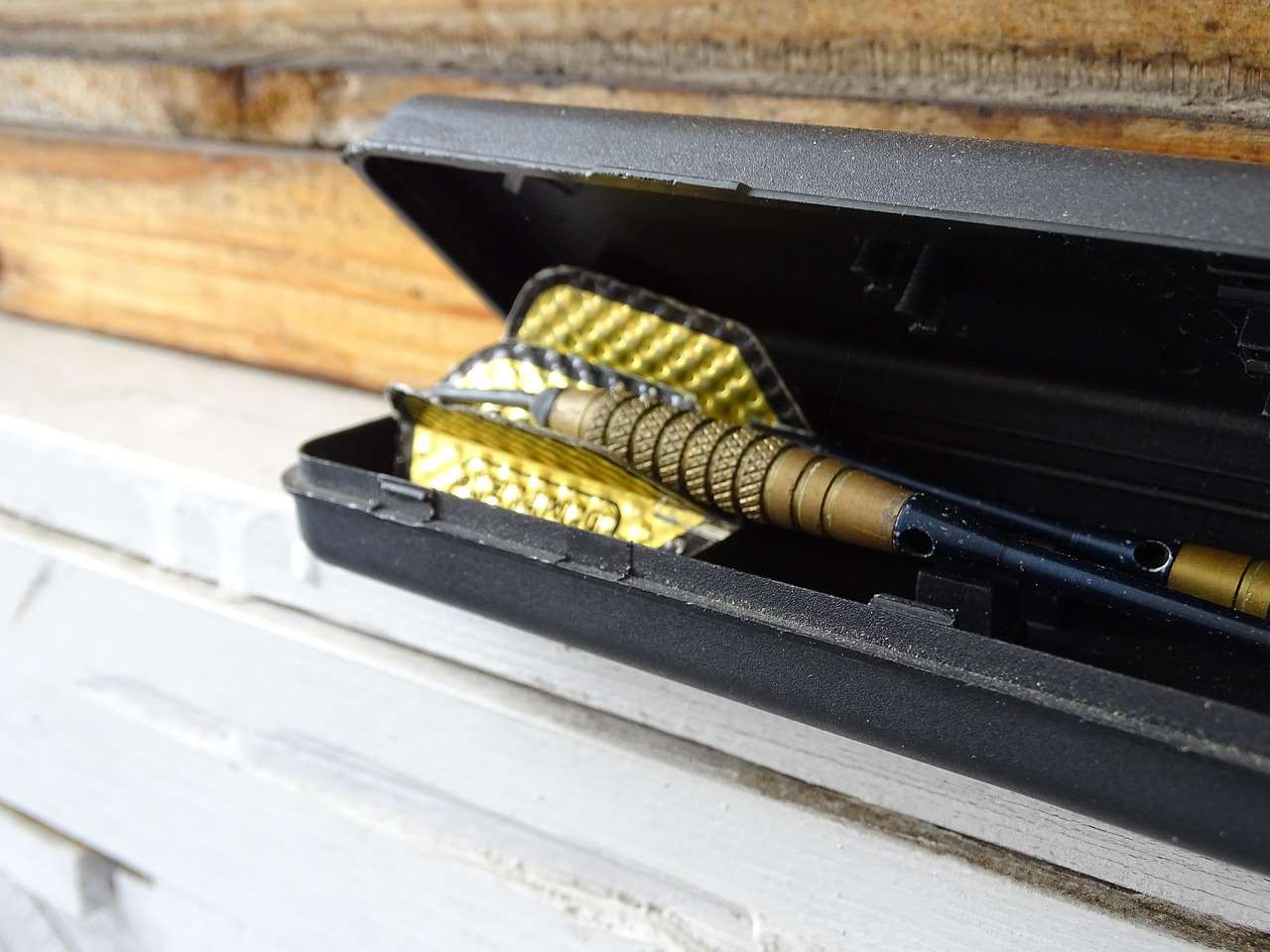
Implementing a Dart Singleton Class: Different Approaches
There are several ways to implement a dart singleton class in Dart. We’ll explore a few common approaches, highlighting their strengths and weaknesses.
Method 1: Static Instance and Private Constructor
This is a very common and straightforward approach. We create a private constructor to prevent direct instantiation and a static getter to access the single instance:
class DatabaseConnection {
static final DatabaseConnection _instance = DatabaseConnection._internal();
factory DatabaseConnection() {
return _instance;
}
DatabaseConnection._internal();
void connect() {
// Database connection logic
print('Connected to the database');
}
}
Here, the _internal
constructor is private, preventing external instantiation. The factory
constructor ensures that only the static instance _instance
is returned. This is a clean and efficient way to implement a dart singleton class.
Method 2: Using a Static Method
This approach uses a static method to return the singleton instance:
class Logger {
static Logger? _instance;
Logger._private(); // Private constructor
static Logger getInstance() {
_instance ??= Logger._private();
return _instance!;
}
void log(String message) {
print('Log: $message');
}
}
The getInstance()
method lazily creates the instance only when it’s needed. This approach is similar to the previous one, but it explicitly uses a static method for instantiation. This could be considered more transparent in terms of how the singleton is created and managed. It’s important to use the null-aware operator (`??=`) to avoid creating multiple instances.
Remember that consistency is key when selecting your approach. Sticking to one method throughout your application will help maintain consistency and reduce complexity. darts point length is a common concern, but a well-managed singleton pattern can help manage this type of data within the application.
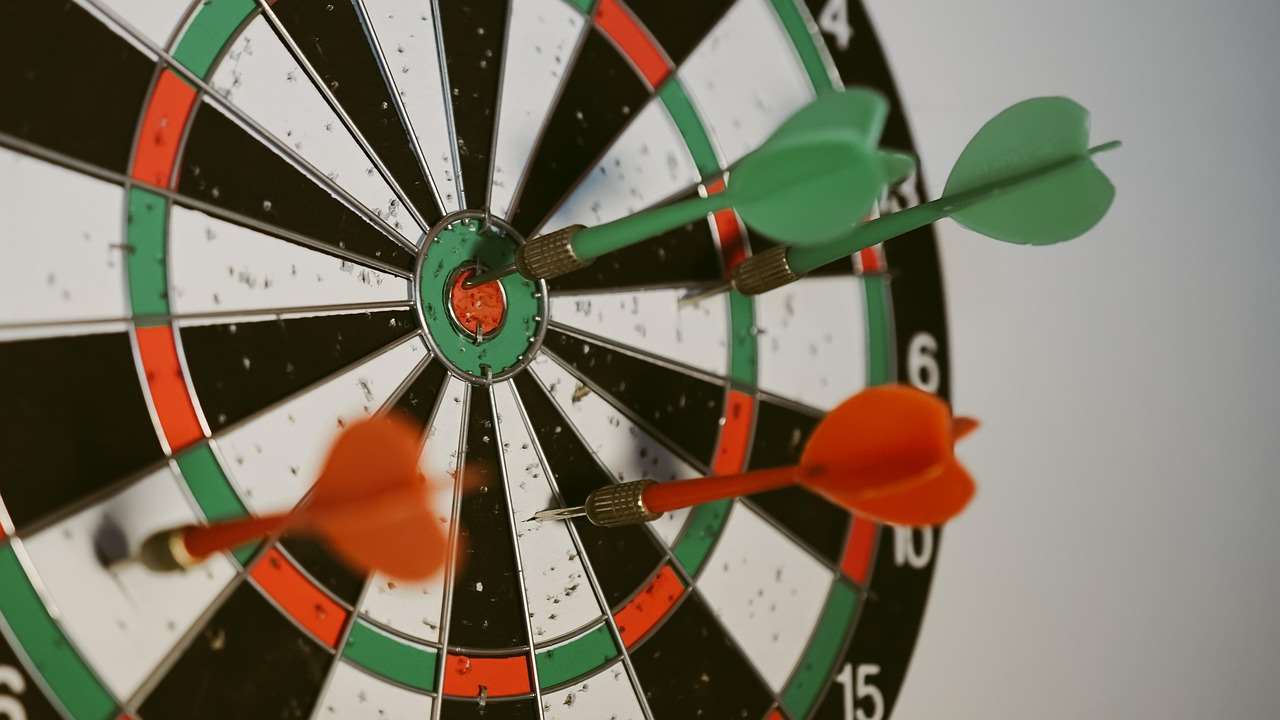
Best Practices for Dart Singleton Classes
While the examples above demonstrate functional singleton implementations, following best practices ensures maintainability and prevents potential issues:
- Keep it Simple: Avoid adding too much functionality to your singleton class. Singletons should focus on a specific, well-defined purpose.
- Consider Dependency Injection: For improved testability, consider using dependency injection to provide dependencies to your singleton instead of hardcoding them.
- Lazy Initialization: If possible, initialize the singleton instance lazily (only when needed) to improve performance.
- Thread Safety: If your application uses multiple threads, make sure your singleton implementation is thread-safe. This might involve using synchronization primitives like mutexes.
- Testing: Test your singleton implementation thoroughly to ensure it behaves as expected in various scenarios.
The choice between different methods depends on context and developer preference. For example, using Practice darts app with scoring could help you to understand the importance of proper data management techniques.
For instance, if you are working with a complex application that requires multiple threads interacting with a database, using a dart singleton class with careful thread safety measures is a better approach than using a simpler method. If you’re working on a smaller application with less complex data handling, a simpler implementation might suffice. Always weigh the pros and cons based on the specific needs of your project.
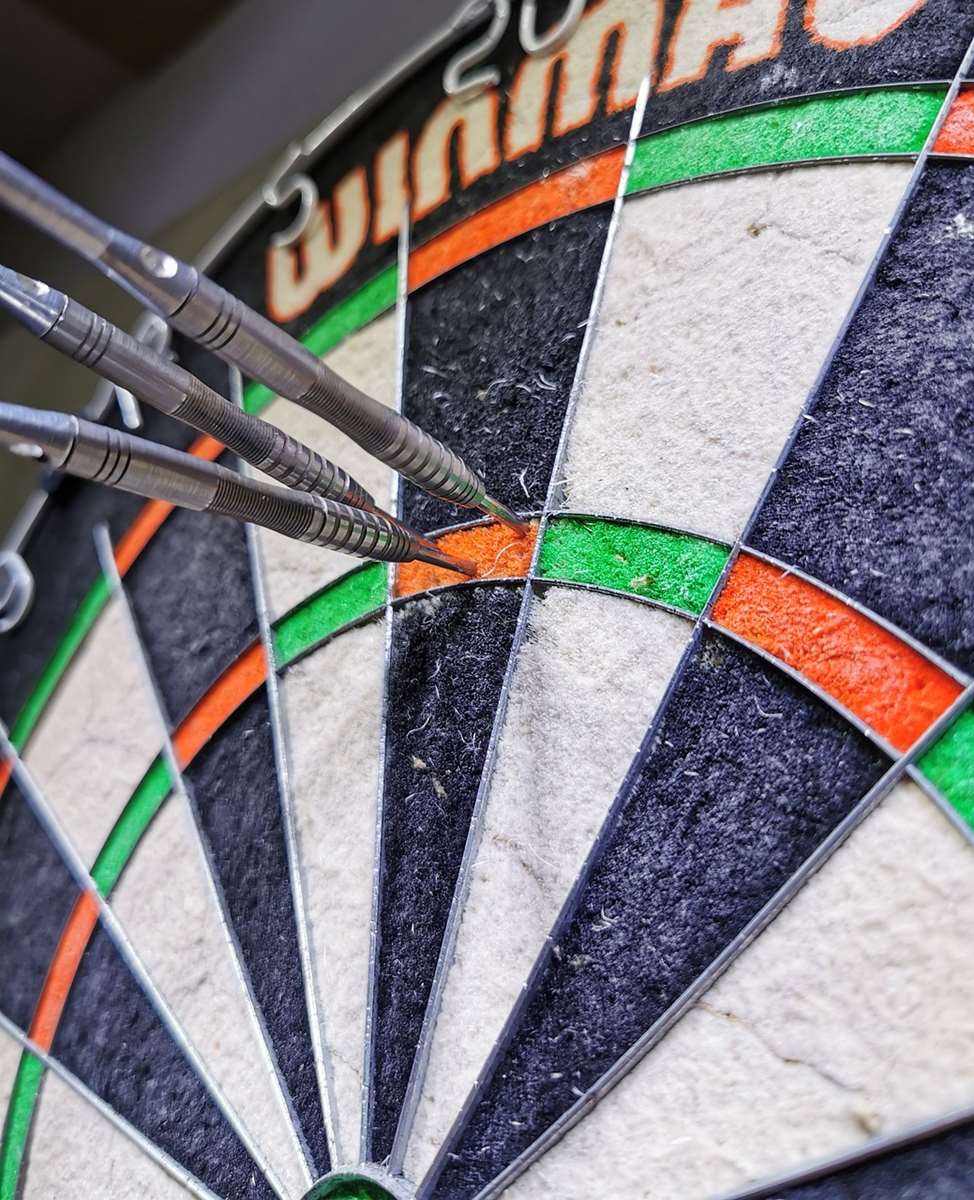
Advanced Concepts and Potential Issues
While singletons offer numerous benefits, they can present challenges if not implemented correctly:
- Testability: Singletons can make unit testing more difficult if not designed carefully. Dependency injection can mitigate this problem.
- Global State: Over-reliance on singletons can lead to tightly coupled code and make it harder to understand the flow of data within the application.
- Concurrency Issues: In multi-threaded environments, unsynchronized access to a singleton’s internal state can lead to race conditions and unexpected behavior.
Careful planning and implementation are crucial to avoid these potential drawbacks. Consider alternative design patterns such as factories or repositories if the singleton pattern introduces unnecessary complexity or tightly coupled code. Using long barrel darts might seem unrelated, but considering analogous situations in other domains helps improve your problem-solving approach to software architecture.
For example, if you are designing a game, it’s advisable to use a singleton to manage the game state. However, using a singleton for each game object is not recommended. This would result in a more tightly coupled code, causing issues during testing and maintaining your code base. Therefore, carefully considering where to use a singleton pattern is as important as properly implementing it.
Understanding the tradeoffs between convenience and maintainability is paramount. A dart singleton class offers a powerful tool, but it’s essential to use it judiciously.
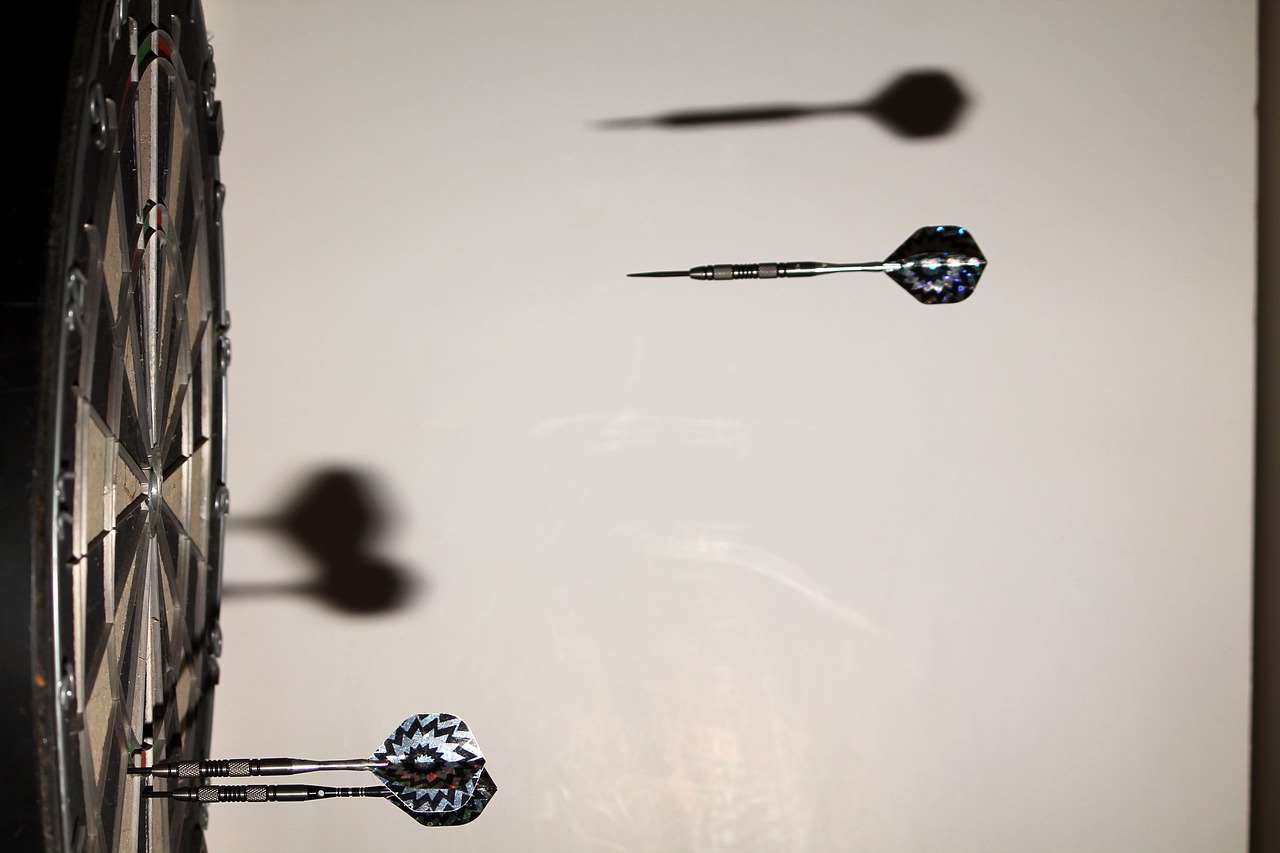
Conclusion
In conclusion, the dart singleton class provides a robust solution for managing single instances of a class, proving useful for resource management, state control, and simplifying code. We’ve explored several implementation methods, emphasizing best practices and highlighting potential pitfalls. By understanding these techniques and adhering to the guidelines presented, you can effectively leverage the power of singletons in your Dart projects while mitigating potential problems. Remember to consider alternative patterns when appropriate, ensuring your code remains clean, testable, and easily maintainable. Now go forth and create elegant, efficient, and well-structured Dart applications!
Remember to check out our other articles on Dart development for more insights and best practices. Happy coding!
Hi, I’m Dieter, and I created Dartcounter (Dartcounterapp.com). My motivation wasn’t being a darts expert – quite the opposite! When I first started playing, I loved the game but found keeping accurate scores and tracking stats difficult and distracting.
I figured I couldn’t be the only one struggling with this. So, I decided to build a solution: an easy-to-use application that everyone, no matter their experience level, could use to manage scoring effortlessly.
My goal for Dartcounter was simple: let the app handle the numbers – the scoring, the averages, the stats, even checkout suggestions – so players could focus purely on their throw and enjoying the game. It began as a way to solve my own beginner’s problem, and I’m thrilled it has grown into a helpful tool for the wider darts community.