Understanding how to perform a dart regexp match is crucial for efficient string manipulation in Dart. This article will show you exactly how to achieve this, providing practical examples and explaining the nuances of regular expressions in the Dart programming language. We’ll cover various scenarios and best practices, ensuring you master dart regexp match operations.
⚠️ Still Using Pen & Paper (or a Chalkboard)?! ⚠️
Step into the future! The Dart Counter App handles all the scoring, suggests checkouts, and tracks your stats automatically. It's easier than you think!
Try the Smart Dart Counter App FREE!Ready for an upgrade? Click above!
Regular expressions, or regexes, are powerful tools for pattern matching within strings. In Dart, the `RegExp` class provides the functionality to work with these patterns. Mastering dart regexp match opens up possibilities for data validation, text processing, and more. Beyond the core dart regexp match, we’ll explore useful techniques and common pitfalls to avoid.
Understanding Dart RegExp Match
The foundation of any successful dart regexp match operation lies in understanding regular expressions themselves. A regular expression is essentially a pattern that describes a set of strings. The RegExp
class in Dart allows you to create these patterns and then use them to search for matches within a given string. Let’s look at a simple example:
import 'dart:convert';
void main() {
final regex = RegExp(r'\d+'); // Matches one or more digits
final string = 'There are 123 apples and 456 oranges.';
final matches = regex.allMatches(string);
for (final match in matches) {
print(match.group(0)); // Prints the matched substring
}
}
This code defines a regular expression that matches one or more digits (\d+
). It then searches for all occurrences of this pattern within the sample string. The output will print “123” and “456”, demonstrating a successful dart regexp match.
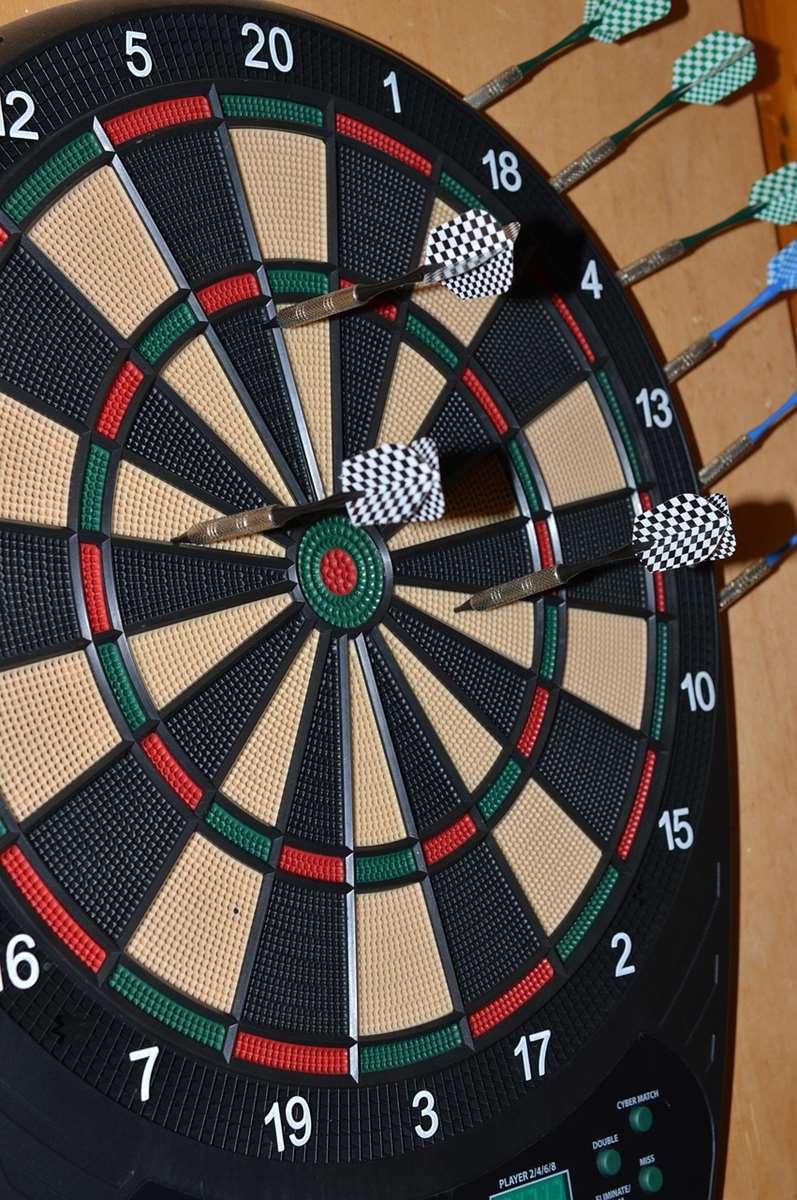
Key Components of a Dart RegExp
- Pattern String: This is the actual regular expression itself, enclosed in forward slashes (
/pattern/
). For instance,r'\b\w+\b'
matches whole words. - Flags: Optional flags modify the behavior of the regular expression. Common flags include
i
(case-insensitive) andm
(multiline). - Methods: The
RegExp
class offers various methods, such ashasMatch()
,allMatches()
, andfirstMatch()
, to find matches within a string.
Understanding these components is crucial for effective dart regexp match operations. Experimenting with different patterns and flags will help you grasp their power and versatility.
Advanced Dart RegExp Matching Techniques
While basic dart regexp match is straightforward, more complex scenarios demand advanced techniques. This section delves into those advanced techniques, enhancing your ability to handle intricate string manipulations.
Capturing Groups
Capturing groups allow you to extract specific parts of a matched string. Parentheses ()
are used to define capturing groups. For example:
final regex = RegExp(r'(\d+)\s+(\w+)'); // Matches digits followed by whitespace and a word
final string = 'There are 123 apples.';
final match = regex.firstMatch(string);
if (match != null) {
print('Number: ${match.group(1)}'); // Prints "Number: 123"
print('Word: ${match.group(2)}'); // Prints "Word: apples"
}
Here, the regular expression uses capturing groups to extract the number and the word separately. This functionality is incredibly useful for parsing structured data.
Lookarounds
Lookarounds are zero-width assertions that match a position in the string without consuming any characters. They are powerful tools for finding patterns based on their context. For example, a positive lookahead ((?=pattern)
) asserts that the pattern follows the current position without including it in the match.
Imagine you need to find all words followed by a period. A lookahead can elegantly solve this problem without including the period in the match itself.
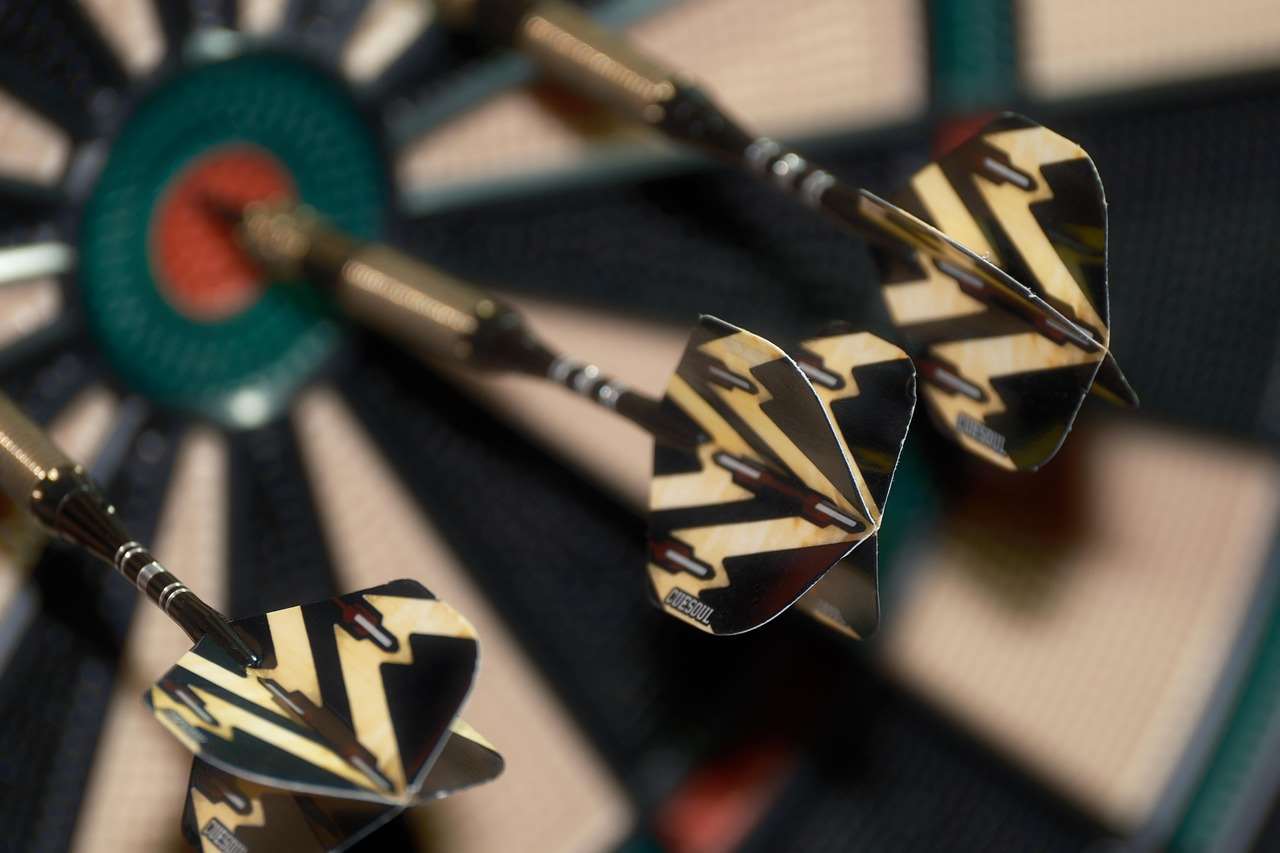
Character Classes
Character classes provide concise ways to define sets of characters. For instance, [aeiou]
matches any lowercase vowel. Combining character classes with other regular expression features enhances the expressiveness and efficiency of your dart regexp match operations. For example, you can easily match any alphanumeric character using \w
or any non-alphanumeric character using \W
, further simplifying your dart regexp match patterns.
Practical Applications of Dart RegExp Match
The practical applications of dart regexp match are vast and span various domains. This section highlights several key use cases, illustrating the power and versatility of regular expressions in Dart.
Data Validation
Regular expressions are invaluable for validating user inputs. You can ensure that email addresses, phone numbers, or other critical data meet specific formats, preventing invalid entries and enhancing the reliability of your applications. For instance, a simple regular expression can validate an email address, ensuring it contains the “@” symbol and a valid domain.
Text Processing
Regular expressions excel at simplifying text processing tasks. Whether you’re extracting keywords from a document, cleaning up messy data, or parsing log files, regular expressions offer powerful tools to streamline these operations. For example, you could use a regular expression to extract all hashtags from a social media post. Think about how much simpler this becomes than iterating manually through characters.
Web Scraping
Regular expressions are frequently employed in web scraping to extract specific data from web pages. By identifying patterns in the HTML source code, you can efficiently extract the desired information. You might use regular expressions to pull out product names, prices, or other crucial data from e-commerce websites. Consider how efficiently you can extract information from an unstructured HTML page using well-crafted regular expressions, a powerful tool for your dart regexp match toolkit.
Efficient web scraping often hinges on the effectiveness of your dart regexp match strategy. The right regular expression can drastically reduce processing time and enhance data accuracy.
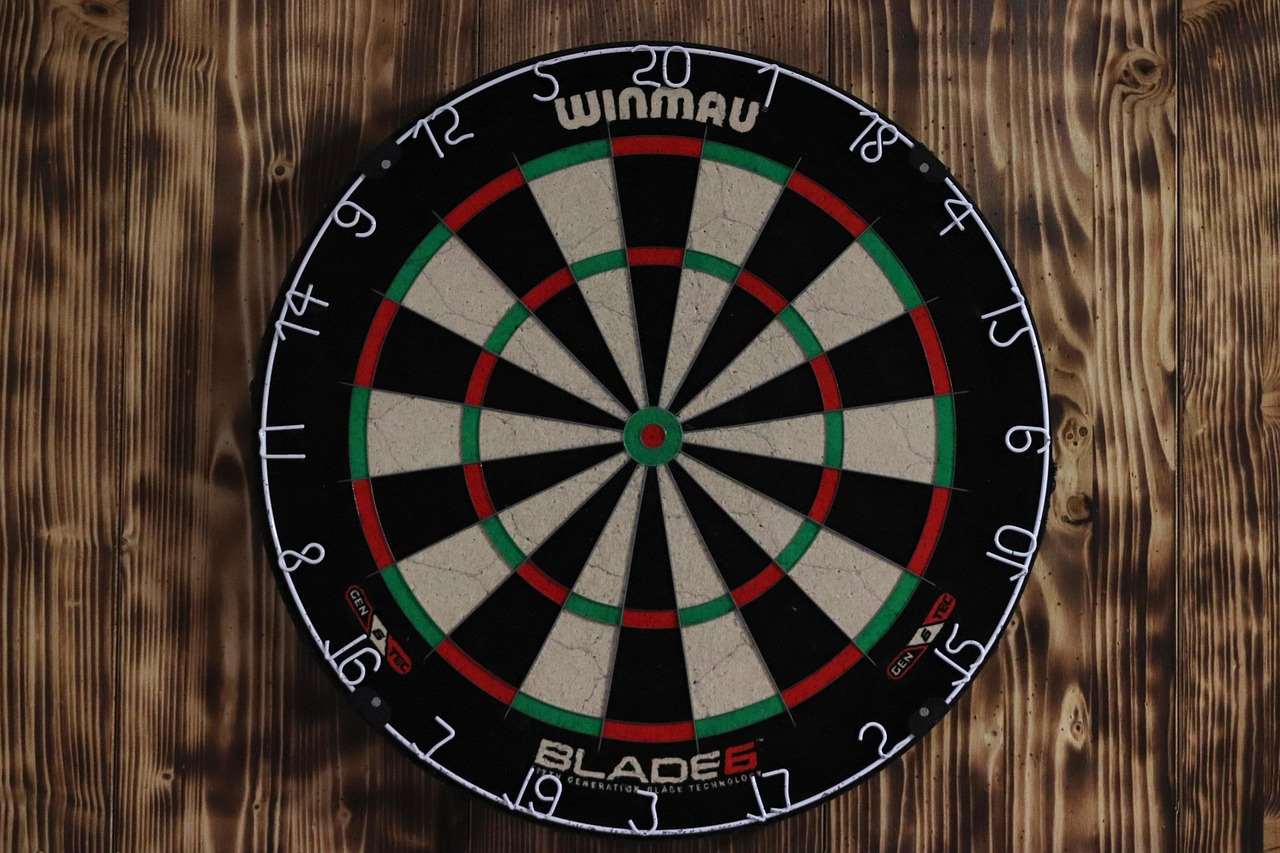
Troubleshooting Common Dart RegExp Issues
Even experienced developers occasionally encounter issues when working with regular expressions. This section addresses common problems and provides solutions to ensure smooth dart regexp match operations.
Escaping Special Characters
Regular expressions contain special characters (e.g., .
, *
, +
) that have specific meanings. If you need to match these characters literally, you must escape them using a backslash (\
). Failure to escape special characters can lead to unexpected results.
Quantifiers and Greedy Matching
Quantifiers (*
, +
, ?
, {n}
) specify how many times a preceding element should match. By default, quantifiers are greedy, meaning they match the longest possible string. If you need non-greedy matching, use a question mark (?
) after the quantifier (e.g., *?
). Understanding this behavior is critical for accurate dart regexp match.
Testing and Debugging
Testing and debugging your regular expressions is crucial. Many online tools allow you to test your expressions against sample strings and visualize the matches. This iterative process helps refine your patterns until they accurately capture your intended matches. Remember that debugging regular expressions can be challenging. Take advantage of available tools to refine your regex effectively.
Optimizing Dart RegExp Match Performance
For optimal performance, consider these tips to optimize your dart regexp match operations, especially when dealing with large amounts of text data.
Avoid Overly Complex Patterns
While complex patterns can achieve precise matches, they can significantly impact performance, especially when applied to large datasets. Opt for simpler, more efficient expressions whenever possible. Prioritize simplicity and clarity for better efficiency.
Use Appropriate Methods
The RegExp
class provides several methods (hasMatch()
, firstMatch()
, allMatches()
). Select the method best suited for your specific needs, as unnecessary iterations over all matches can degrade performance. Choose the most efficient matching method for your use case.
Precompile Regular Expressions
For repeated use of the same regular expression, consider precompiling it. This can yield notable performance improvements by avoiding repeated compilation overhead, especially in performance-critical sections of your code. This optimization strategy is particularly useful for repetitive dart regexp match scenarios.

Using a precompiled RegExp
object, rather than creating a new one for each matching operation, drastically improves performance when working with large datasets and repetitive dart regexp match tasks.
Integrating Dart RegExp Match with Other Libraries
The power of dart regexp match extends beyond its core functionality. Integrating it with other Dart libraries can unlock even greater potential.
For instance, you might combine it with libraries handling file input/output for processing large text files or with libraries for web interactions to facilitate web scraping.
The ability to seamlessly integrate dart regexp match within a larger application workflow unlocks enhanced functionality and streamlines data processing tasks.
Consider incorporating dart regexp match within a larger application context, leveraging its capabilities for efficient and reliable data manipulation.
Resources for Mastering Dart RegExp Match
Numerous resources are available to deepen your understanding of dart regexp match and regular expressions in general.
- The official Dart documentation provides comprehensive details on the
RegExp
class and its methods. Consult this invaluable resource for accurate and up-to-date information. - Online regex testers allow you to experiment with different patterns and quickly check their behavior. Experiment and learn through practical application and testing.
- Numerous tutorials and blog posts cover regular expressions in Dart, offering additional insights and practical examples.
Consistent practice and exploration of these resources will further enhance your mastery of dart regexp match.
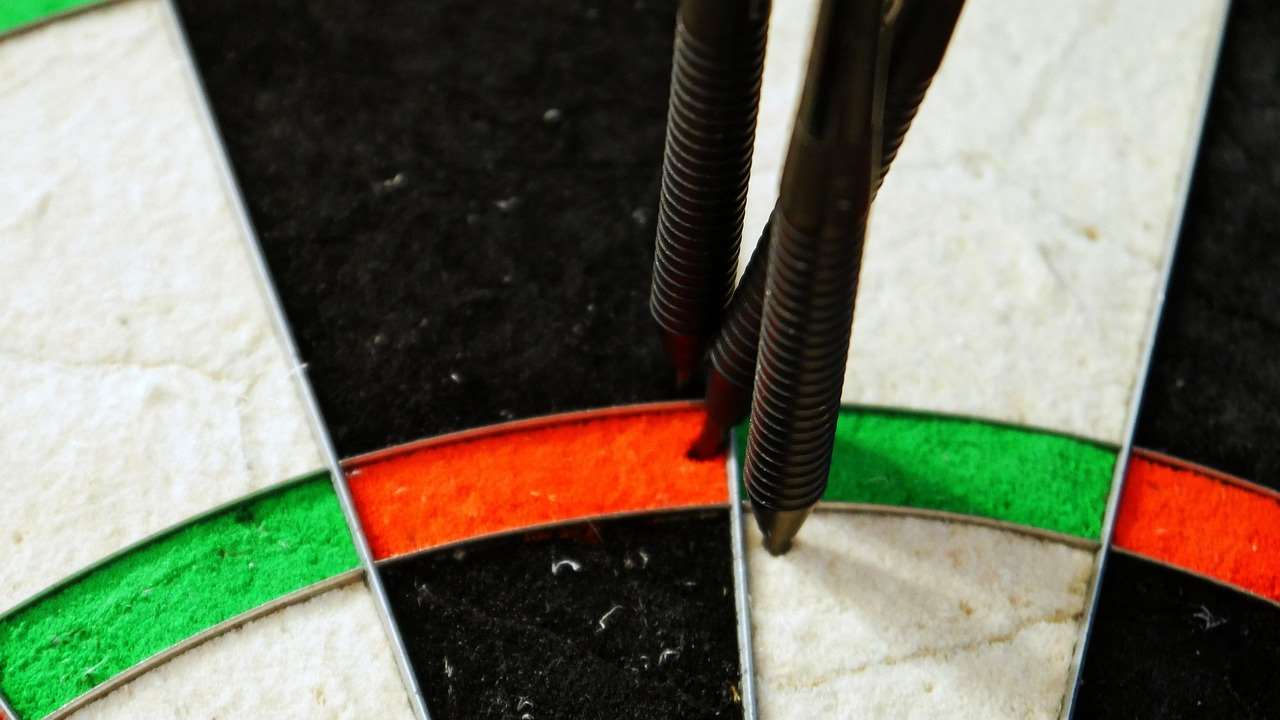
Conclusion
Mastering dart regexp match is essential for any Dart developer. This article has provided a comprehensive guide, covering fundamental concepts, advanced techniques, practical applications, and performance optimization strategies. Remember to use online tools to test and refine your regular expressions and consult the official documentation for accurate information. By applying the techniques and best practices discussed here, you can significantly improve your ability to manipulate and extract information from strings in your Dart projects. Start practicing today and leverage the full power of regular expressions in your projects. If you’re working on a project that needs efficient scorekeeping, check out our Darts scorekeeper app for a reliable solution. You can also learn more about improving your game by researching topics like dart board reviews, dartboard size, or even most 180s in a darts match. For those interested in customizing your darts, you might find resources on single dart hood construction or blade 6 winmau helpful. And if you’re looking for online retailers, you might find popdarts amazon useful. Remember to check out our guides on darts stand line, darts counter master in and dartcounter beta for further tips. Don’t forget to explore our guide on creating a dart flight template!
Hi, I’m Dieter, and I created Dartcounter (Dartcounterapp.com). My motivation wasn’t being a darts expert – quite the opposite! When I first started playing, I loved the game but found keeping accurate scores and tracking stats difficult and distracting.
I figured I couldn’t be the only one struggling with this. So, I decided to build a solution: an easy-to-use application that everyone, no matter their experience level, could use to manage scoring effortlessly.
My goal for Dartcounter was simple: let the app handle the numbers – the scoring, the averages, the stats, even checkout suggestions – so players could focus purely on their throw and enjoying the game. It began as a way to solve my own beginner’s problem, and I’m thrilled it has grown into a helpful tool for the wider darts community.