Understanding a dart match enum is crucial for anyone serious about programming applications related to darts scoring and match management. This article will provide a clear explanation of what a dart match enum is and how it’s implemented, along with practical examples and applications. We’ll also explore related concepts and best practices.
⚠️ Still Using Pen & Paper (or a Chalkboard)?! ⚠️
Step into the future! The Dart Counter App handles all the scoring, suggests checkouts, and tracks your stats automatically. It's easier than you think!
Try the Smart Dart Counter App FREE!Ready for an upgrade? Click above!
Before diving into the intricacies of a dart match enum, let’s establish a foundational understanding. A dart match involves players, scores, and a series of rounds or legs. To efficiently manage these elements within a program, using an enumerated type, or enum, offers a robust and organized structure. It enhances readability and maintainability significantly.
This approach provides a well-defined set of possible match types, improving code clarity and preventing errors that might arise from using arbitrary string values to represent the different match formats. For example, an enum can represent the standard game types like 301, 501, or even more specialized variants. A well-structured dart match enum acts as a cornerstone for a well-designed darts scoring application.
Understanding the Dart Match Enum
A dart match enum, in essence, is a data type that defines a set of named constants. These constants represent the various types of dart matches possible. This structured approach prevents errors associated with typos or inconsistencies in representing match types using strings. Instead of using strings like “301” or “501”, the dart match enum provides a safer and more readable alternative.
Defining a Dart Match Enum
The way you define a dart match enum will vary depending on the programming language you are using. However, the core concept remains consistent. You define a set of named constants representing different match types. These constants will frequently include a numerical representation that can be used for calculating game states or scores. For example, an enum could look like this (using C# syntax):
public enum DartMatchType {
Unknown = 0,
ThreeHundredOne = 301,
FiveHundredOne = 501,
Cricket = 1000,
AroundTheClock = 2000
}
This example shows a dart match enum defining common match types. Each type has an associated integer value for internal representation. Adding new match types is as simple as adding new members to the enum. This clear representation is a key advantage of using a dart match enum, which also makes it incredibly beneficial for maintaining code cleanliness and long-term project scalability.
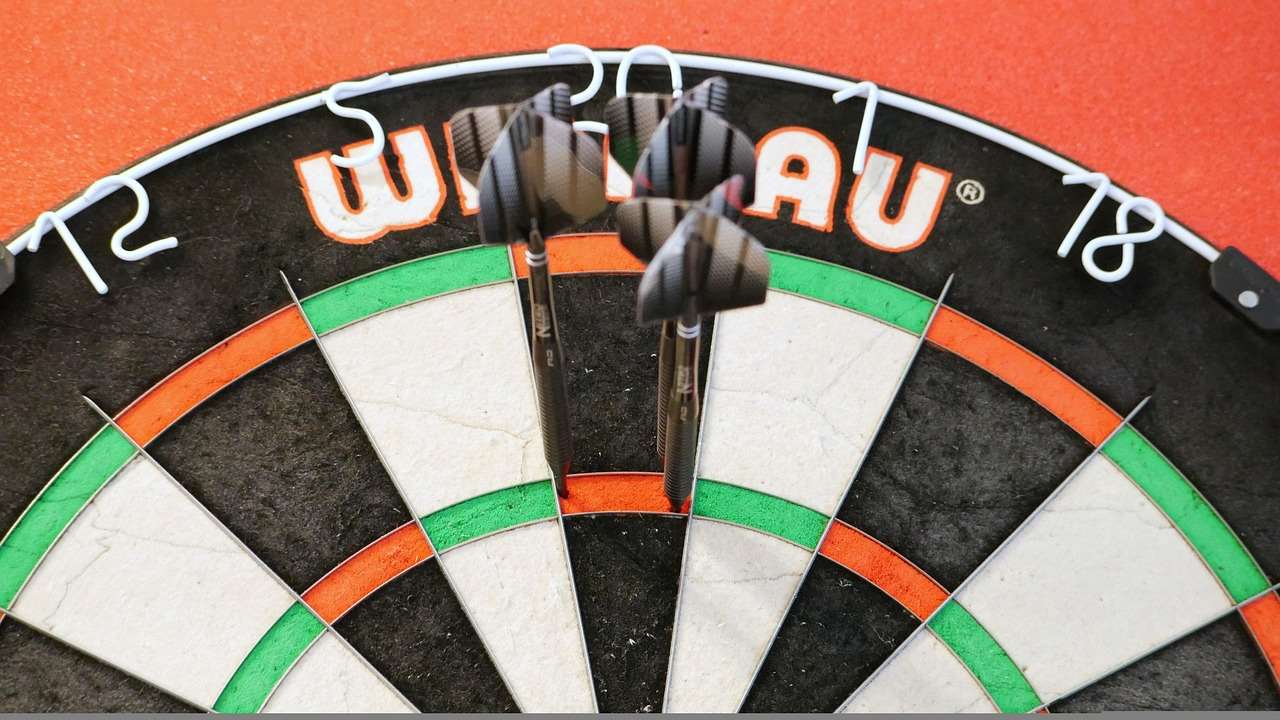
Advantages of Using a Dart Match Enum
Using a dart match enum in your darts application offers several key advantages over using strings or integers directly:
- Improved Readability:
DartMatchType.FiveHundredOne
is significantly clearer than the integer 501 or the string “501”. - Type Safety: The compiler or interpreter can help prevent errors by ensuring that only valid match types are used.
- Maintainability: Adding new match types is easy, and changes are contained within the enum definition.
- Extensibility: You can easily expand your dart match enum to accommodate future match variations.
- Refactoring: Changes to match type names or values only need to be made in one central location—the enum definition itself.
These advantages translate to cleaner, more maintainable code that’s less prone to errors. When working on a complex darts scoring system, especially one intended for wider use or potential expansion, the benefits of a dart match enum become even more pronounced. The improved type safety alone can save you significant debugging time down the line. Consider the possibilities of including more advanced match types like “Shanghai” or even customizable match settings. A well-designed dart match enum allows for such expansions with minimal effort.
Practical Applications of a Dart Match Enum
A well-defined dart match enum is indispensable for building robust applications involving darts scoring and match management. Here are several ways you can leverage it in practice:
- Game Initialization: Use the enum to select the type of match when initiating a new game.
- Score Calculation: Implement logic that uses the enum to determine how to calculate the score based on the match type.
- Game State Management: Maintain the current state of the game (e.g., player’s turn, score, number of rounds played) using the dart match enum.
- Data Persistence: Store the match type along with other game data for later retrieval and replay.
- User Interface: Use the enum values to display the match type in the user interface.
Beyond these fundamental applications, a dart match enum provides a flexible framework for implementing more advanced features, allowing your application to seamlessly handle various game rules and scenarios. It’s particularly useful for building a system that can manage and display the results of multiple concurrent matches, ensuring that all match data remains logically organized and easily accessible.
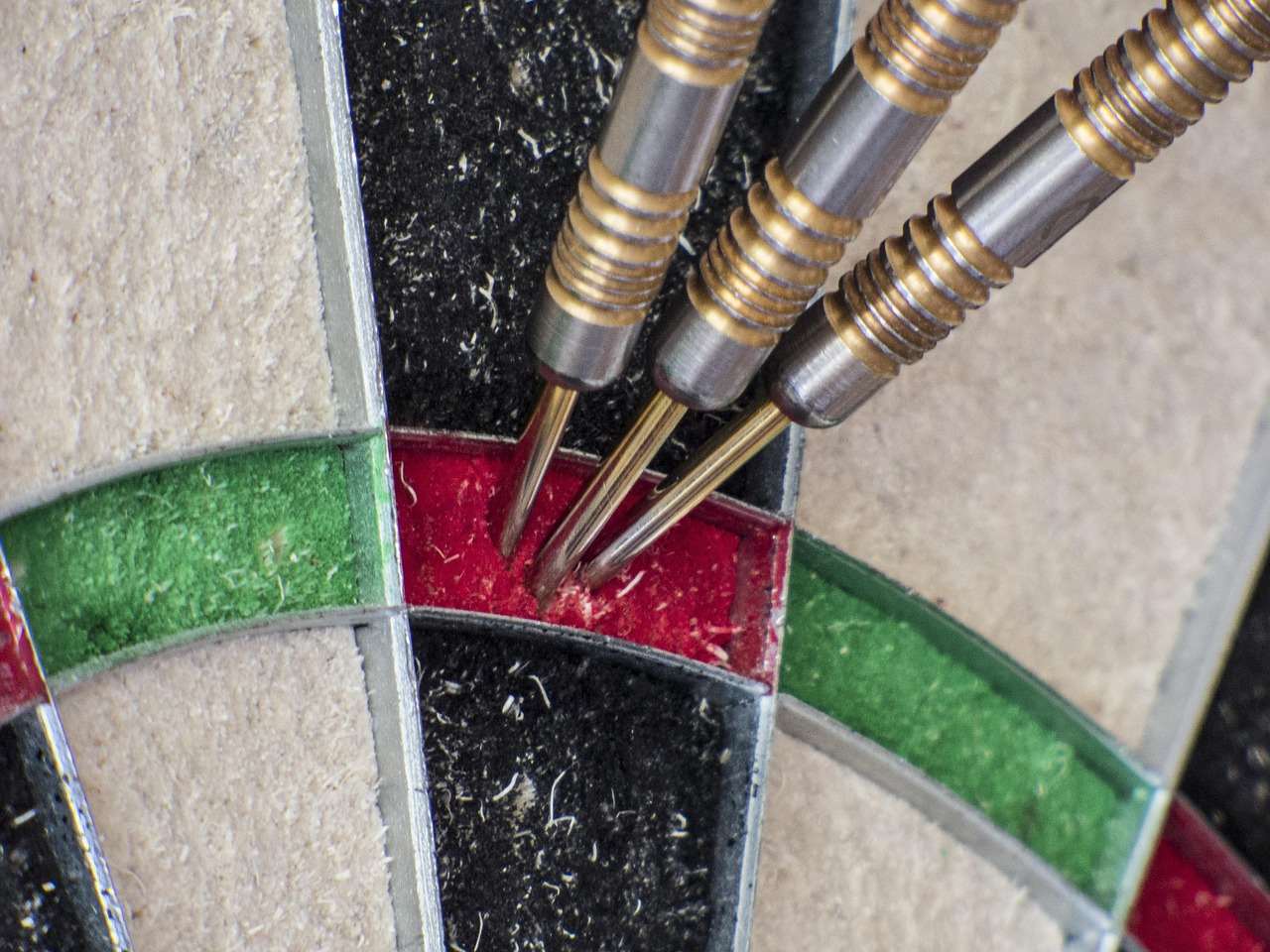
Beyond the Basics: Expanding Your Dart Match Enum
While a simple dart match enum covers the most common match types, you can expand its functionality to include more detailed information about each match. Consider adding properties or methods to the enum members. For instance, you could include properties specifying the number of legs, sets, or specific scoring rules. This approach enhances the versatility and extensibility of your dart match enum.
Adding Metadata to Your Enum
Let’s explore how to add metadata to enrich the information contained within your enum. In some languages, this might involve using associated data structures or helper classes. By adding metadata, you can effectively associate information like the number of legs required to win a match. This is particularly helpful for customizing the scoring logic, creating more dynamic and flexible dart applications.
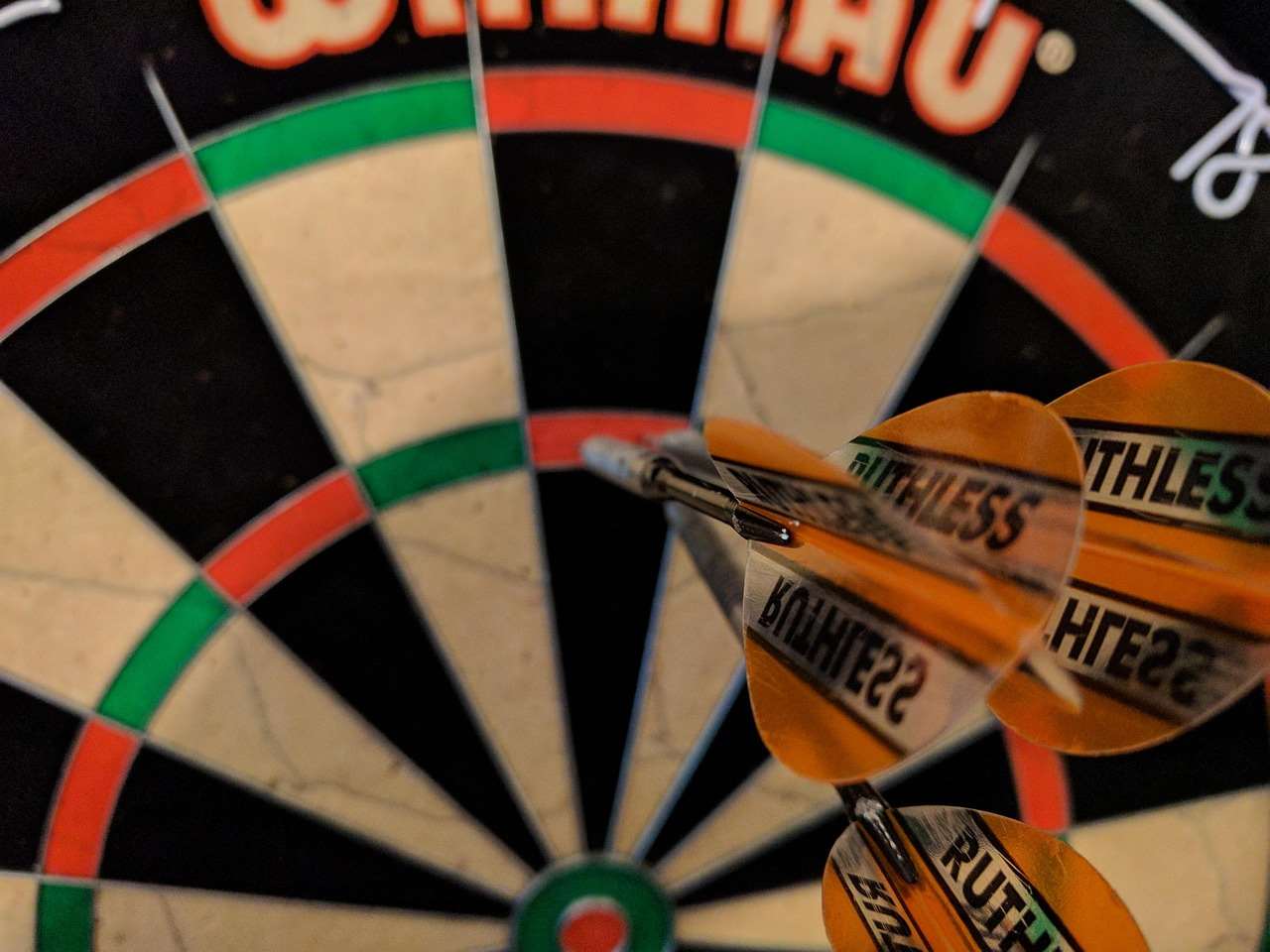
For example, if you are building a darts power point presentation, you might incorporate the dart match enum to show the various match types dynamically in a slide show. You could add another field to the enum to specify the appropriate visual representation for each match type, enriching the user experience and providing more detail in your presentation.
Error Handling and Robustness
Robust error handling is crucial in any application. When working with a dart match enum, consider incorporating checks to handle invalid or unexpected match types. This could involve using default values or throwing exceptions to prevent unexpected behavior. For instance, if you receive an unknown match type from an external source, your application should gracefully handle this scenario rather than crashing or producing erroneous results. This improves the overall reliability and user experience.
Incorporating strategies for handling unexpected inputs and error conditions is a vital part of developing robust and reliable software, including any application involving a dart match enum. Remember to consistently test your application against different scenarios, including those that might involve invalid or incomplete data, to ensure it can handle unexpected situations efficiently.
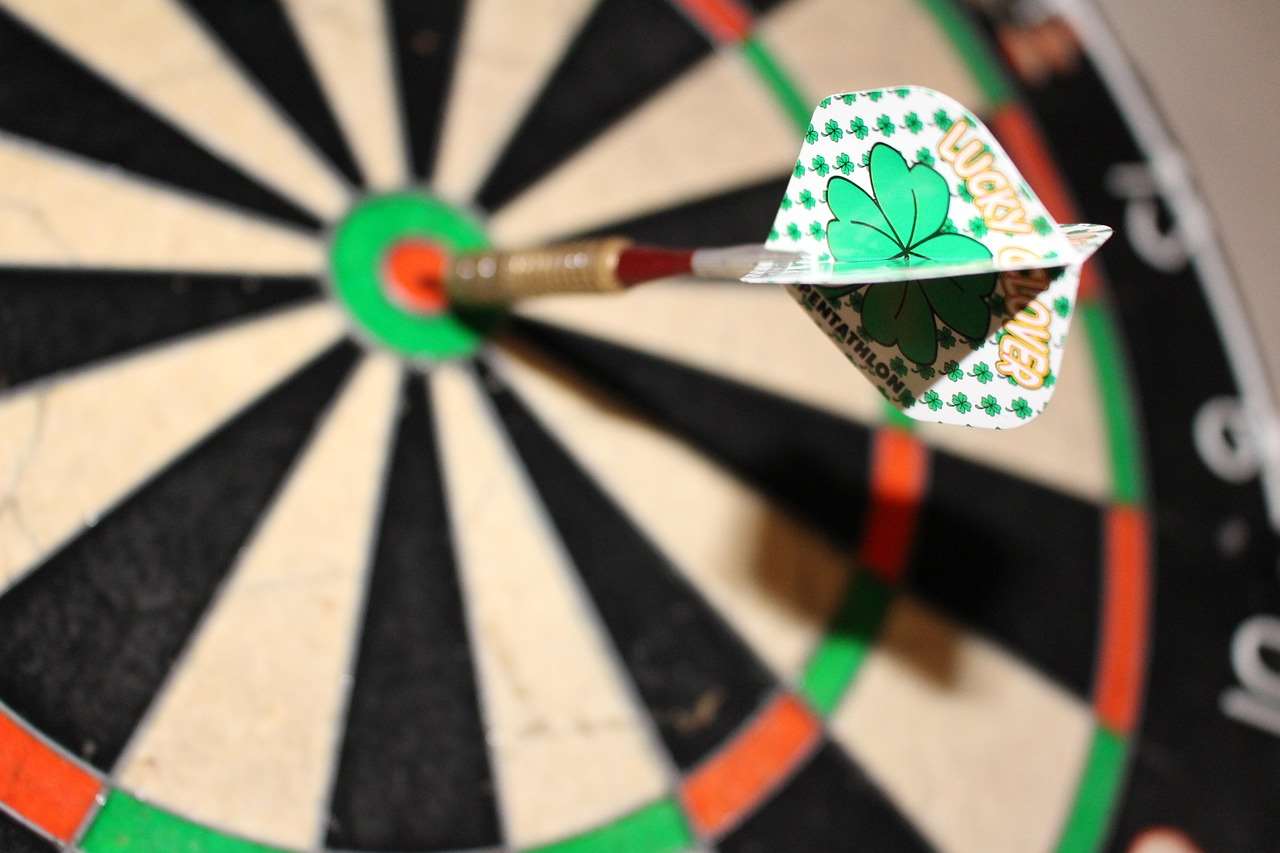
Integrating with Other Components
Your dart match enum should seamlessly integrate with other components of your application. For example, it should interact correctly with your scoring system, database, and user interface. Proper integration ensures smooth data flow and prevents inconsistencies. For example, ensuring your user interface correctly displays the chosen match type based on the enum value. If you’re developing a mobile application, ensure that the dart match enum is appropriately handled across different screen sizes and device orientations.
Consider also the potential for integrating with external services such as online databases or third-party APIs. If your application allows users to create and manage their own dart matches, a well-structured dart match enum is key for data validation and consistency across your entire system. Remember to design your system with scalability in mind, especially if you expect a large user base or a wide variety of match formats.
Building a comprehensive darts application requires careful attention to detail and robust architecture. A well-structured dart match enum is a critical component of this architecture, ensuring that your application remains maintainable, reliable, and easy to extend as your project evolves.
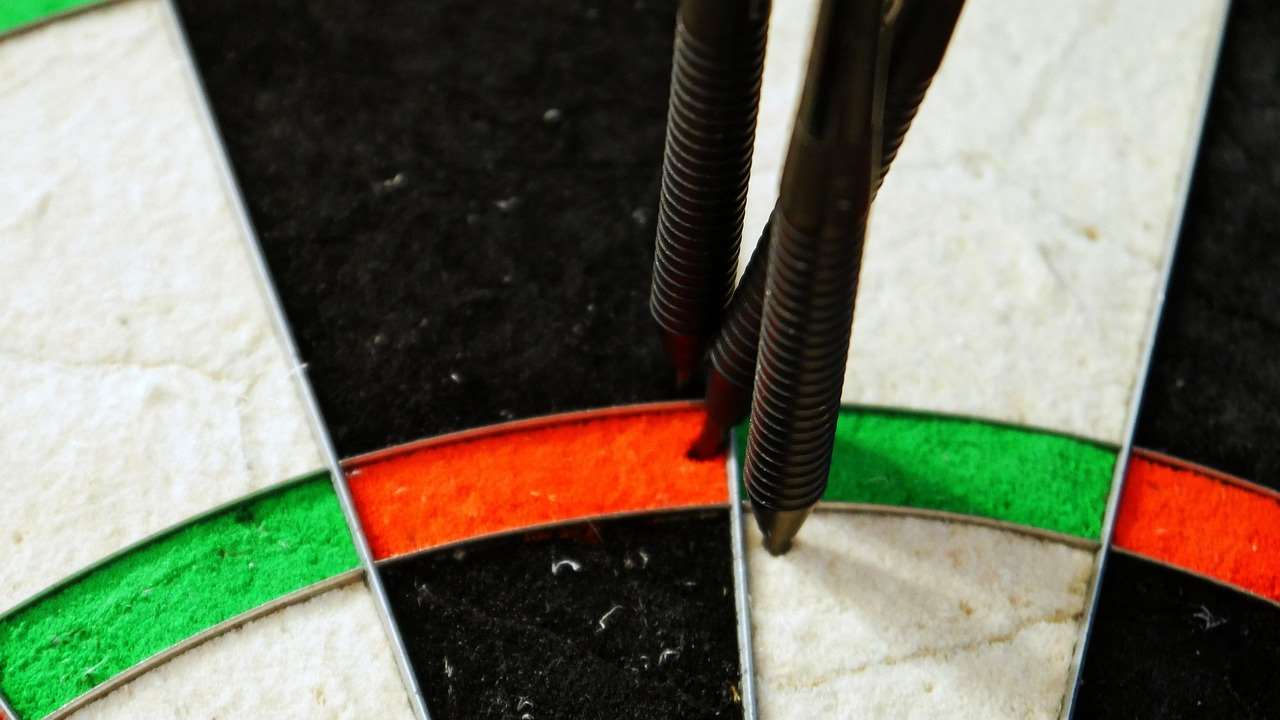
Conclusion: Mastering the Dart Match Enum
The dart match enum is a powerful tool for managing and organizing different match types in your darts application. By leveraging its benefits—improved readability, type safety, and maintainability—you can create a far more robust and user-friendly experience. Remember to consider error handling, integration with other components, and the potential for expansion as your project grows. Start building your application with a well-designed dart match enum today, and experience the improvements in your code’s clarity, efficiency, and maintainability.
For more information on developing darts applications, you may find resources like Dart Counter App helpful. Remember to explore advanced concepts such as associating metadata with your enum and implementing robust error handling to ensure your application is as polished and efficient as possible. Start building your next great darts application now!
Choosing the right dart match enum is crucial to developing a robust and maintainable darts application. Do you have questions about dart match enum implementation in a specific programming language or integration with specific tools? Let us know in the comments below!
Hi, I’m Dieter, and I created Dartcounter (Dartcounterapp.com). My motivation wasn’t being a darts expert – quite the opposite! When I first started playing, I loved the game but found keeping accurate scores and tracking stats difficult and distracting.
I figured I couldn’t be the only one struggling with this. So, I decided to build a solution: an easy-to-use application that everyone, no matter their experience level, could use to manage scoring effortlessly.
My goal for Dartcounter was simple: let the app handle the numbers – the scoring, the averages, the stats, even checkout suggestions – so players could focus purely on their throw and enjoying the game. It began as a way to solve my own beginner’s problem, and I’m thrilled it has grown into a helpful tool for the wider darts community.