Understanding the relationship between Dart and JavaScript is crucial for any developer working with modern web technologies. While they aren’t directly interchangeable, Dart JavaScript interoperability is increasingly important, especially with frameworks like Flutter that compile to JavaScript for web deployment. This article will explore this relationship, detailing how Dart and JavaScript work together and providing practical tips for developers working in both environments.
⚠️ Still Using Pen & Paper (or a Chalkboard)?! ⚠️
Step into the future! The Dart Counter App handles all the scoring, suggests checkouts, and tracks your stats automatically. It's easier than you think!
Try the Smart Dart Counter App FREE!Ready for an upgrade? Click above!
Dart, with its strong typing and object-oriented features, offers a different development experience compared to JavaScript’s dynamic nature. However, the ability to bridge the gap between these two languages is a significant advantage. We’ll cover how to leverage this interoperability, handle data exchange, and effectively utilize the strengths of both languages in your projects. We’ll also look at practical examples, common challenges, and best practices.
Understanding Dart and Its JavaScript Compilation
Dart is a client-optimized programming language developed by Google. It’s known for its fast performance, which is especially valuable in building user interfaces. One of its key features is its ability to compile to JavaScript. This means you can write your application logic in Dart and then have it automatically translated into JavaScript code that can run in any web browser. This capability is a cornerstone of the Dart JavaScript interaction we will discuss further.
This compilation process allows developers to enjoy the benefits of Dart’s structured programming model while still targeting the vast reach of the web, thanks to JavaScript’s ubiquitous support. The compilation effectively acts as a bridge, allowing Dart applications to function seamlessly within the JavaScript ecosystem. This is especially beneficial when integrating with existing JavaScript libraries or APIs. However, understanding the nuances of this compilation is critical to avoid performance bottlenecks or unexpected behavior. The compiled JavaScript code might not always be as performant as hand-written JavaScript, depending on the complexity of the original Dart code.
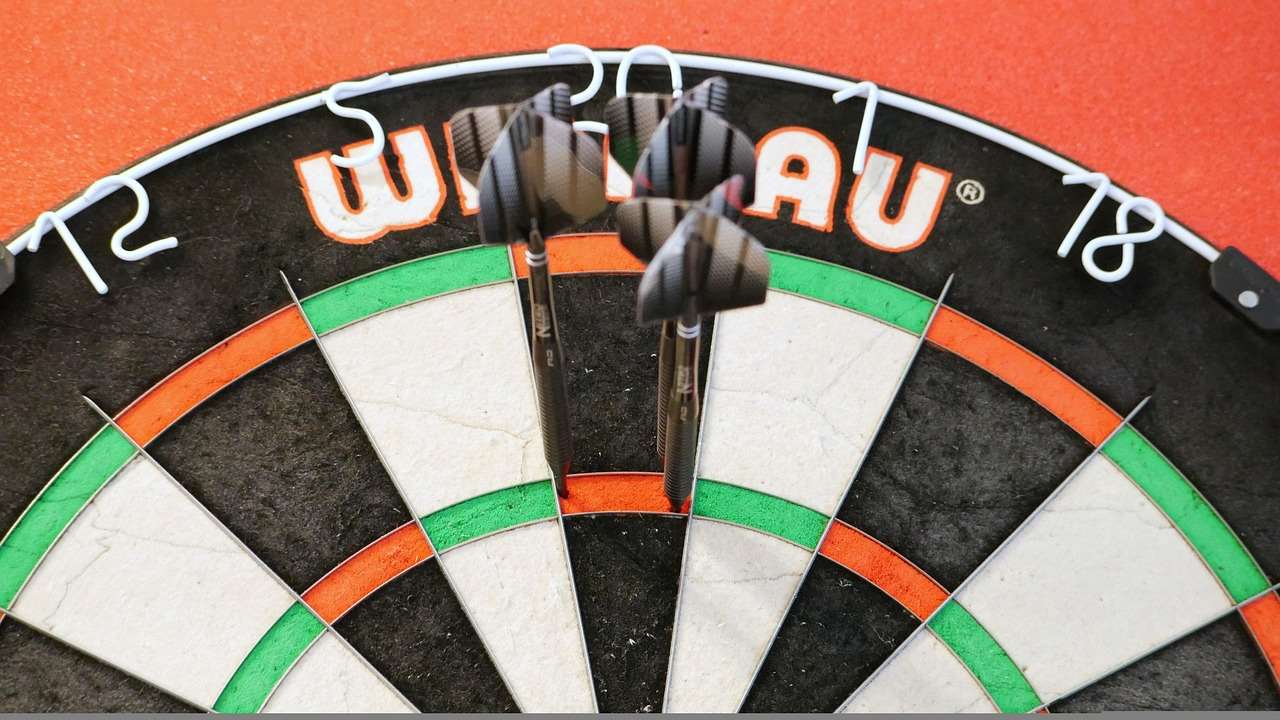
A key advantage of using Dart compiled to JavaScript is the improved developer experience. Dart’s static typing helps catch errors early in the development process, leading to more robust and maintainable code. This contrasts sharply with JavaScript’s dynamic typing, which can lead to runtime errors if not carefully managed. Using Dart for the core logic, and then letting it compile to JavaScript for the web, combines the best of both worlds.
Interacting with JavaScript from Dart
The ability to call JavaScript code from Dart is a significant aspect of Dart JavaScript integration. This is commonly achieved using the `js` package, a powerful tool that allows seamless communication between the two languages. The `js` package provides the necessary mechanisms to access existing JavaScript libraries and APIs directly from within your Dart code. This feature opens up a world of possibilities, enabling developers to leverage the extensive ecosystem of JavaScript libraries and frameworks.
Utilizing the `js` Package
The `js` package simplifies the process of interacting with JavaScript. Let’s explore a simple example. To use the package, first add `js: ^0.6.3` (or the latest version) to your `pubspec.yaml` file. Then, in your Dart code, you can use the `js` library to call JavaScript functions and access variables:
import 'dart:js';
void main() {
// Access a JavaScript function
context['myJavascriptFunction']?.call([]);
// Access a JavaScript variable
final myJavascriptVariable = context['myJavascriptVariable'];
}
Remember to replace `myJavascriptFunction` and `myJavascriptVariable` with the actual names of your JavaScript functions and variables. This simple example illustrates how straightforward it is to interact with JavaScript functions and variables using Dart and the js
package. Proper error handling and type checking are crucial to ensure the stability of your application.
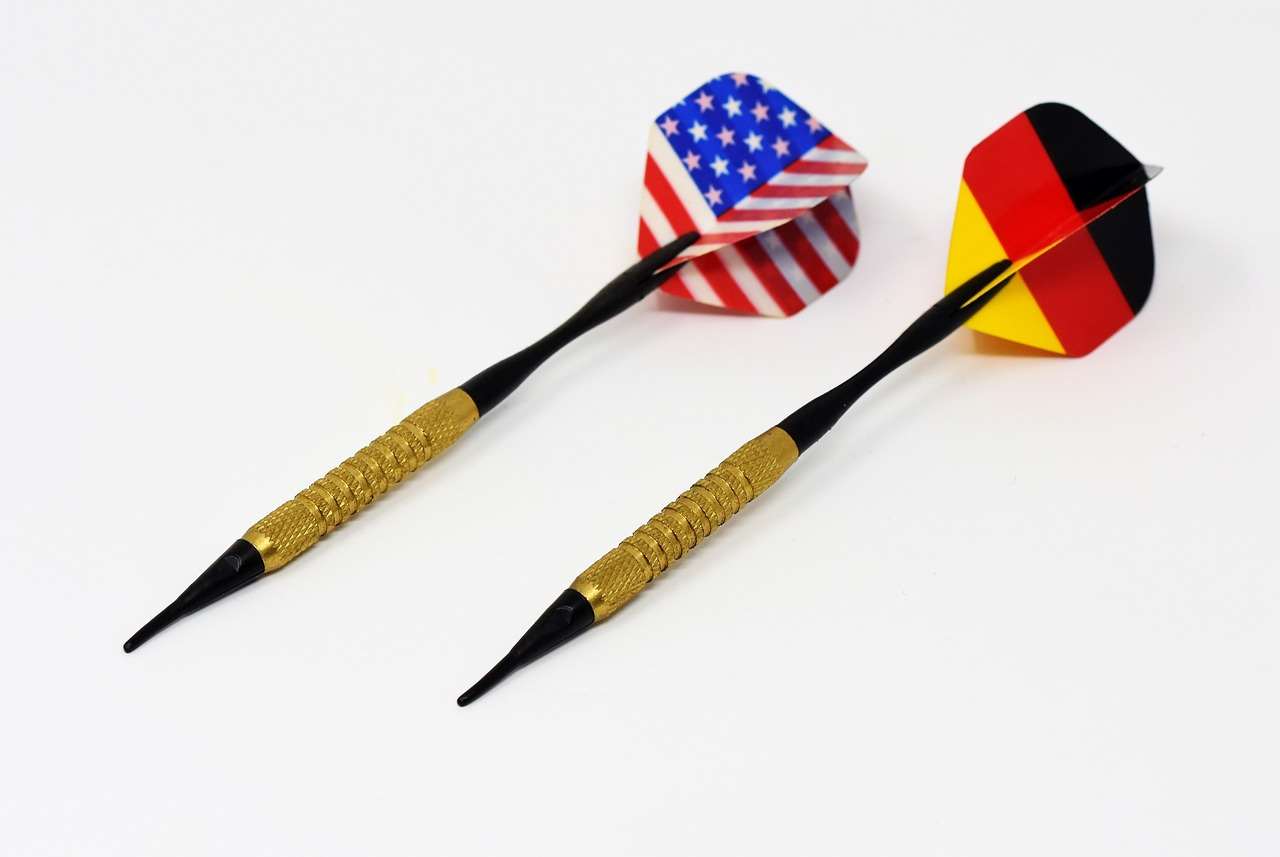
For instance, let’s imagine you have a JavaScript library that handles complex mathematical computations. You can call this library directly from your Dart code, utilizing its specialized functions without rewriting them in Dart. This significantly reduces development time and leverages existing, well-tested JavaScript codebases. This interoperability is one of the most powerful aspects of Dart JavaScript development.
Passing Data Between Dart and JavaScript
Effective data exchange between Dart and JavaScript is key to successful interoperability. Because Dart and JavaScript have different type systems, careful consideration is required. Dart’s strong type system helps prevent errors, but when interacting with JavaScript’s dynamically typed environment, you need to be mindful of potential type mismatches. The `js` package provides mechanisms for converting data between Dart and JavaScript types to mitigate these issues.
Data Type Conversion
Understanding how data types are converted during the Dart JavaScript interaction is vital. Simple data types like numbers and strings usually convert seamlessly. However, complex objects require more careful handling. When passing complex objects, you might need to convert them into a simpler format (like JSON) to ensure compatibility between the two languages. This process might involve serialization and deserialization, which the `js` package can assist with.
Using JSON for data exchange is a common best practice. JSON’s lightweight format and broad compatibility make it an ideal medium for inter-language communication. By converting Dart objects to JSON before passing them to JavaScript, and vice versa, you can significantly reduce the risk of type-related errors.
It’s essential to meticulously test your data exchange mechanisms. Debugging issues related to data type mismatches can be time-consuming, so preventative measures such as thorough type checking and JSON serialization are crucial for a smooth and reliable development experience.
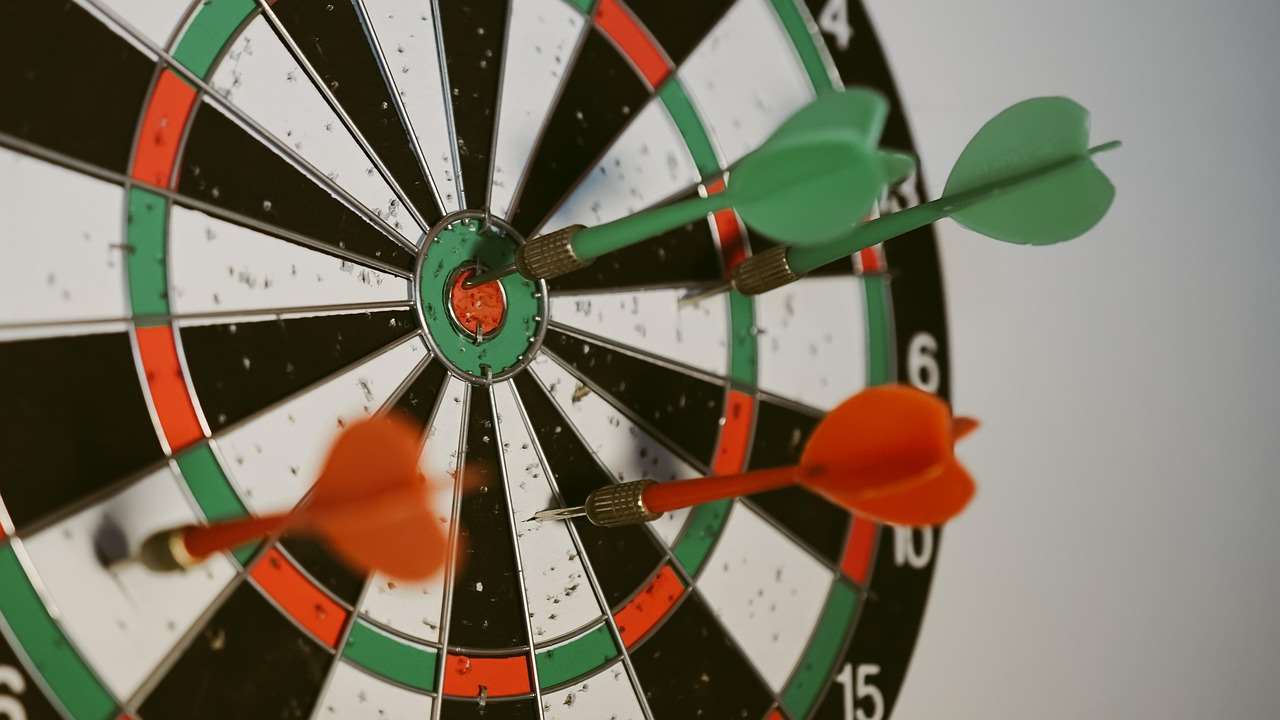
Best Practices for Dart JavaScript Development
To maximize the effectiveness of your Dart JavaScript projects, adhere to these best practices:
- Use the `js` package efficiently: Understand the nuances of its functionalities to interact seamlessly with JavaScript libraries and APIs.
- Employ JSON for data exchange: This robust method minimizes type-related errors and enhances interoperability.
- Implement thorough error handling: Carefully manage potential exceptions arising from the interoperability of the two languages.
- Leverage Dart’s strong typing: This feature significantly improves the quality of your Dart code, despite the dynamic nature of JavaScript.
- Thoroughly test your code: Rigorous testing is essential to uncover and resolve potential issues with data exchange and integration.
By following these best practices, you can build more robust, maintainable, and efficient applications that leverage the strengths of both Dart and JavaScript. This combined approach allows you to enjoy the improved developer experience of Dart while maintaining the broad reach of JavaScript.
For example, you might use Dart to build the core logic and UI of your application, relying on its performance and structured approach. Then, you can utilize existing JavaScript libraries for specialized tasks, such as map integrations or complex animations. This modular approach is key to effectively using Dart JavaScript.
Troubleshooting Common Issues
Despite the power of Dart’s JavaScript compilation, you may encounter challenges. Understanding common issues and troubleshooting techniques is essential. Issues often relate to data type mismatches, improper use of the `js` package, or unexpected behavior during the compilation process. Careful logging and debugging are crucial for diagnosing and resolving these issues.
Remember to consult the official Dart and Flutter documentation for the most up-to-date information and solutions to potential problems. The online community is also a valuable resource for finding answers to specific queries and sharing best practices.
Proper error handling in your Dart code, coupled with logging mechanisms, can significantly aid in debugging interactions with JavaScript. When encountering errors, examine the type and origin of the error message to pinpoint the source of the problem. Often, a simple typo or a mismatch in data types can be the root cause.
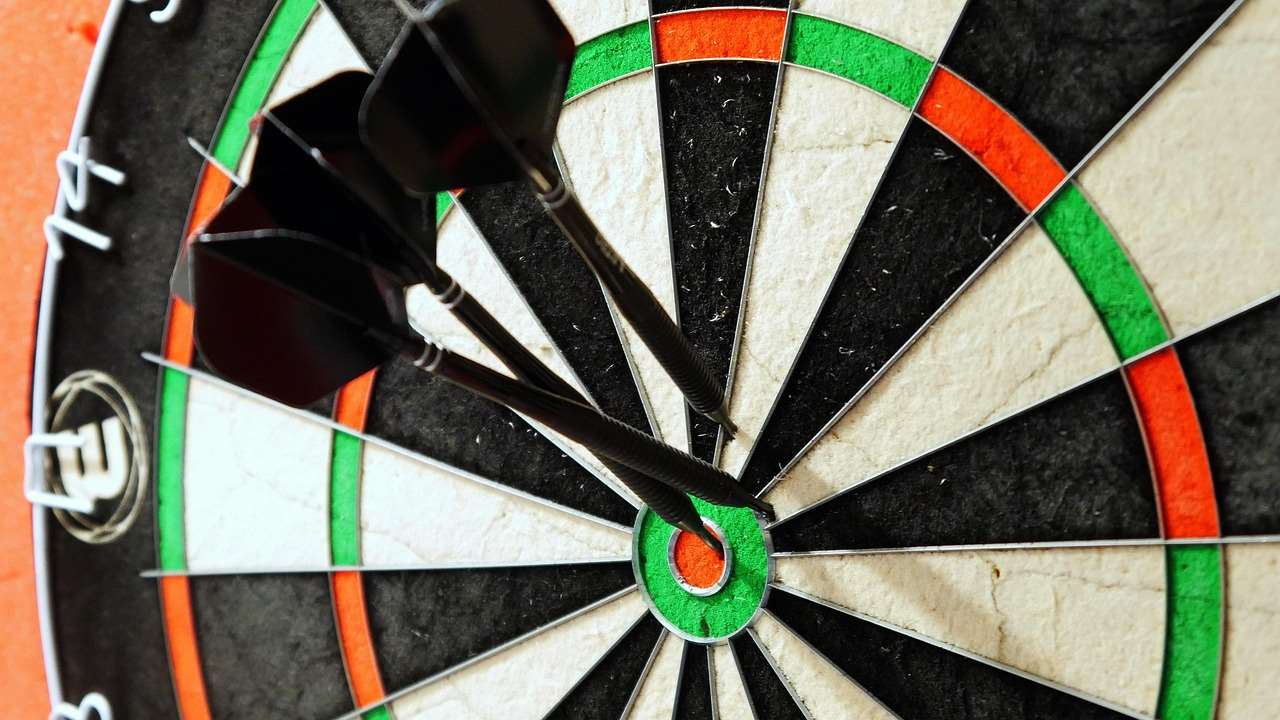
Effective use of debugging tools, both in your IDE and your browser’s developer tools, can be invaluable in identifying and resolving issues during the development process. The ability to step through your code and inspect the state of variables is essential for understanding the flow of execution.
Conclusion: Embracing the Power of Dart JavaScript
Dart JavaScript interoperability offers a powerful combination for web development. By understanding the mechanics of compilation, data exchange, and the `js` package, developers can build robust applications that leverage the best of both worlds: the structured development experience of Dart and the vast reach of JavaScript. Adopting best practices and effective troubleshooting techniques are key to maximizing the potential of this synergistic approach.
Remember to explore the extensive resources available online, including official documentation and community forums, to deepen your understanding and solve any challenges you might encounter. Embrace the flexibility and power of Dart JavaScript to create innovative and efficient web applications. Start experimenting with the Best darts scoring app to see the power of Dart in action.
To further enhance your understanding, consider exploring resources on darts bull rules, darts counter man, and even dart flights michael van gerwen – the seemingly unrelated topics can illustrate the versatility of web technologies built using Dart and JavaScript. Learn more about granny darts bullseye and target agora to see further practical applications.
Don’t forget to check out our other resources on darts wax, darts second hand, dart rankings, darts game line, and what is darts checkout for further exploration into the world of darts and related technology.
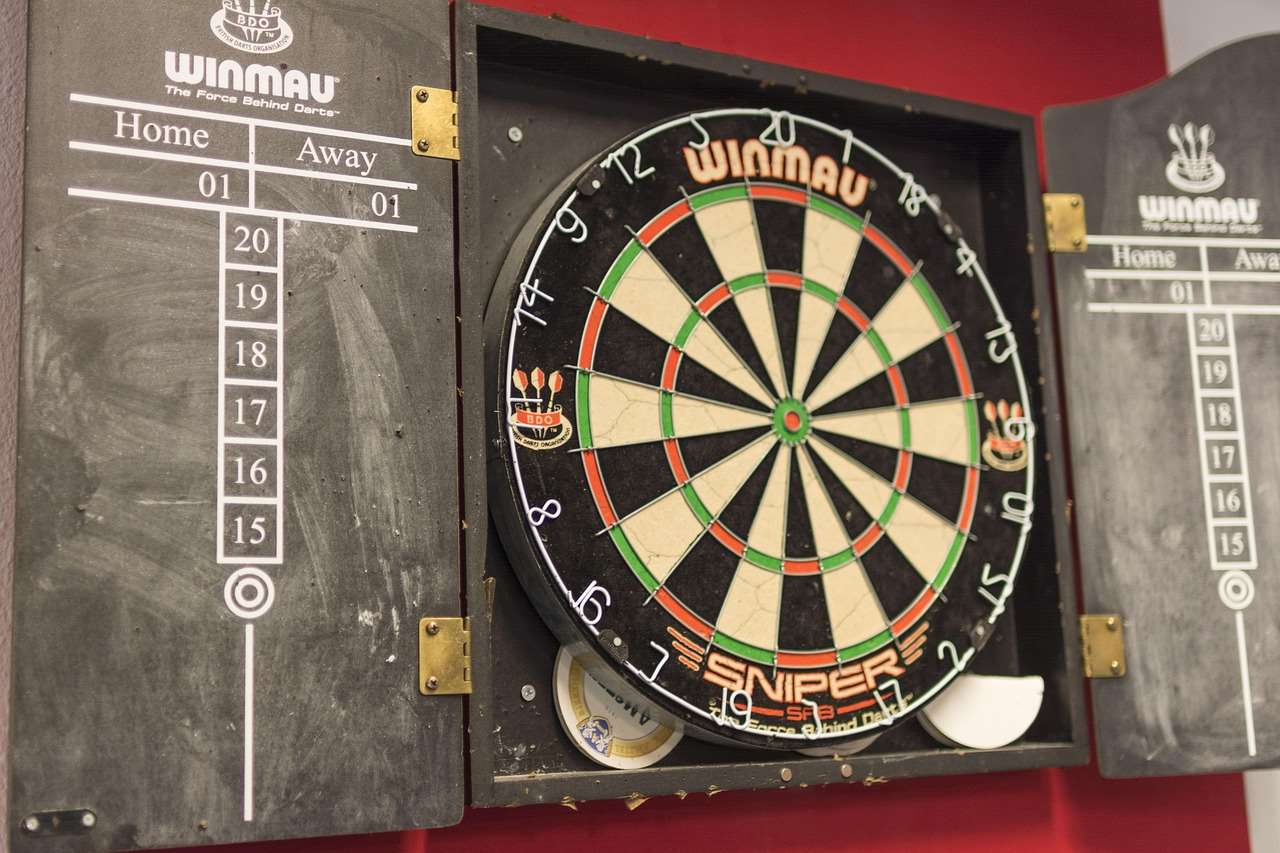
Hi, I’m Dieter, and I created Dartcounter (Dartcounterapp.com). My motivation wasn’t being a darts expert – quite the opposite! When I first started playing, I loved the game but found keeping accurate scores and tracking stats difficult and distracting.
I figured I couldn’t be the only one struggling with this. So, I decided to build a solution: an easy-to-use application that everyone, no matter their experience level, could use to manage scoring effortlessly.
My goal for Dartcounter was simple: let the app handle the numbers – the scoring, the averages, the stats, even checkout suggestions – so players could focus purely on their throw and enjoying the game. It began as a way to solve my own beginner’s problem, and I’m thrilled it has grown into a helpful tool for the wider darts community.