The core answer to the question, ‘dart is single threaded or multi threaded,’ is that Dart is primarily single-threaded. However, it offers powerful mechanisms to leverage multiple cores through isolates, providing a form of concurrency without true multithreading. This article will delve into the intricacies of Dart’s concurrency model, explore isolates in detail, and discuss the implications for your application development.
⚠️ Still Using Pen & Paper (or a Chalkboard)?! ⚠️
Step into the future! The Dart Counter App handles all the scoring, suggests checkouts, and tracks your stats automatically. It's easier than you think!
Try the Smart Dart Counter App FREE!Ready for an upgrade? Click above!
Understanding how Dart handles concurrency is crucial for building high-performance and responsive applications. While the underlying execution model is primarily single-threaded, leveraging isolates allows for efficient parallelization of tasks, improving the responsiveness of your UI and the overall efficiency of your application. The core language itself remains fundamentally single-threaded, making it easier to reason about and debug, but this doesn’t limit its ability to handle complex, multi-faceted processes.
Let’s explore the implications of this single-threaded nature for different aspects of your Dart projects. For example, you might have multiple animations running on the screen, each seeming to execute concurrently but in fact are carefully managed within the confines of the single thread, thanks to the event loop and its ability to rapidly switch between tasks.
Dart is Single Threaded or Multi Threaded: Understanding the Single-Threaded Model
At its heart, Dart employs a single-threaded event loop. This means that a single thread handles all the events and code execution within a Dart program. This approach simplifies development by minimizing race conditions and the complexities associated with shared memory access inherent to true multi-threading. This eliminates the need for complicated synchronization mechanisms that can be prone to errors in multi-threaded environments. This makes debugging far easier since there is only a single thread of execution to trace and analyze.
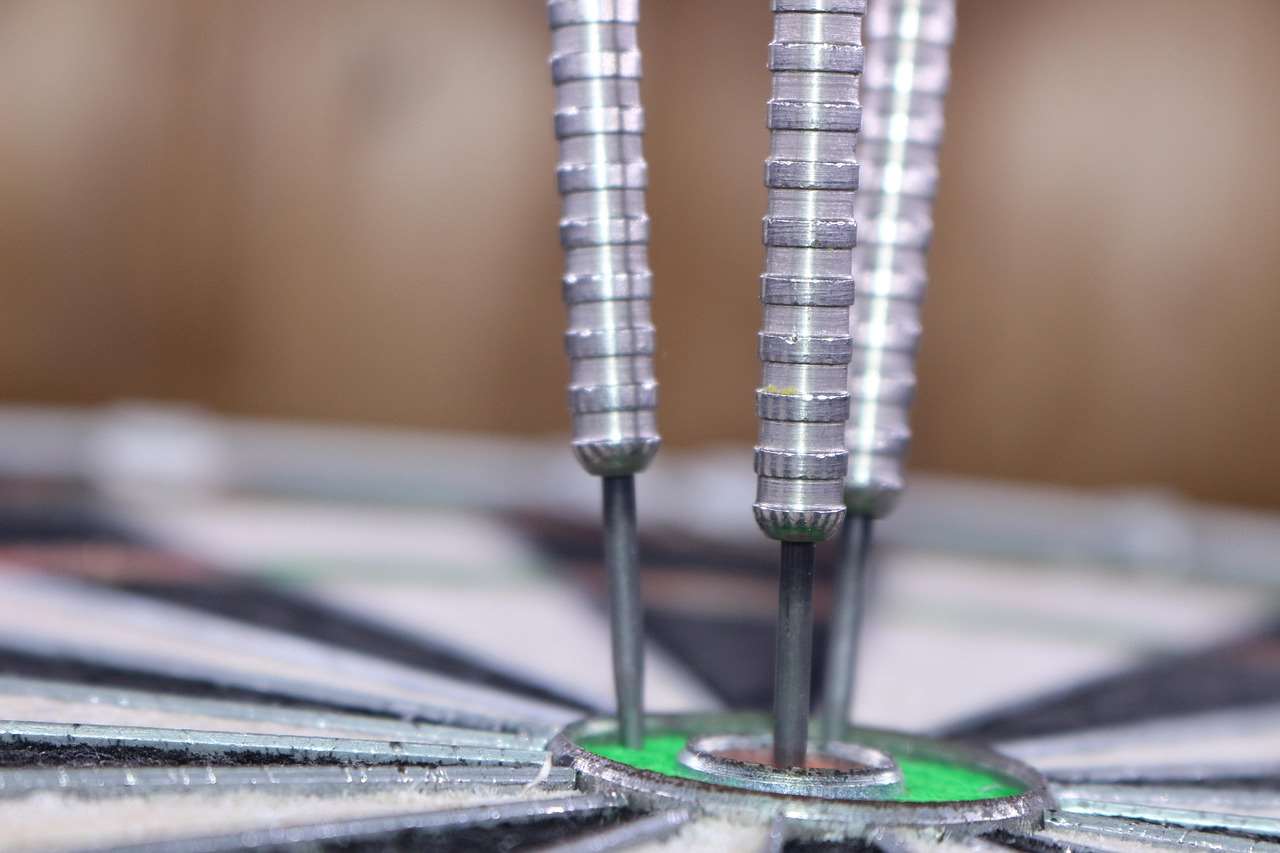
Consider a simple example: a user interface updating in response to user interactions. In a truly multithreaded environment, multiple threads might simultaneously attempt to update the same UI elements, causing conflicts and unexpected behavior. Dart’s single-threaded approach elegantly prevents this by ensuring that UI updates occur one after another, in a predictable and consistent manner. While this might seem limiting, Dart’s robust event loop and asynchronous programming models more than compensate for this apparent limitation.
The benefits of Dart’s single-threaded model are significant: it’s much easier to write correct, concurrent code in a single-threaded environment than it is in a multi-threaded one. The simplicity inherent in this approach promotes code readability, understandability, and maintainability. Moreover, it often results in faster execution times for simpler tasks, due to the absence of the overhead associated with managing multiple threads.
Advantages of Dart’s Single-Threaded Architecture
- Simplified Debugging: Easier to trace execution flow and identify issues.
- Reduced Complexity: Less overhead in managing threads and synchronization.
- Improved Code Readability: Cleaner, simpler code structure.
Dart is Single Threaded or Multi Threaded: The Power of Isolates
While Dart is fundamentally single-threaded, it provides a powerful mechanism for achieving concurrency: isolates. Isolates are independent workers that run in parallel, each possessing its own memory space. This is key; isolates do not share memory. This isolation prevents race conditions and simplifies concurrency management. Communication between isolates is handled through message passing, which adds a layer of safety and predictability. The need for complex synchronization mechanisms, a common source of bugs in multi-threaded programming, is avoided. This is where Dart cleverly sidesteps some of the usual challenges associated with true multi-threading.
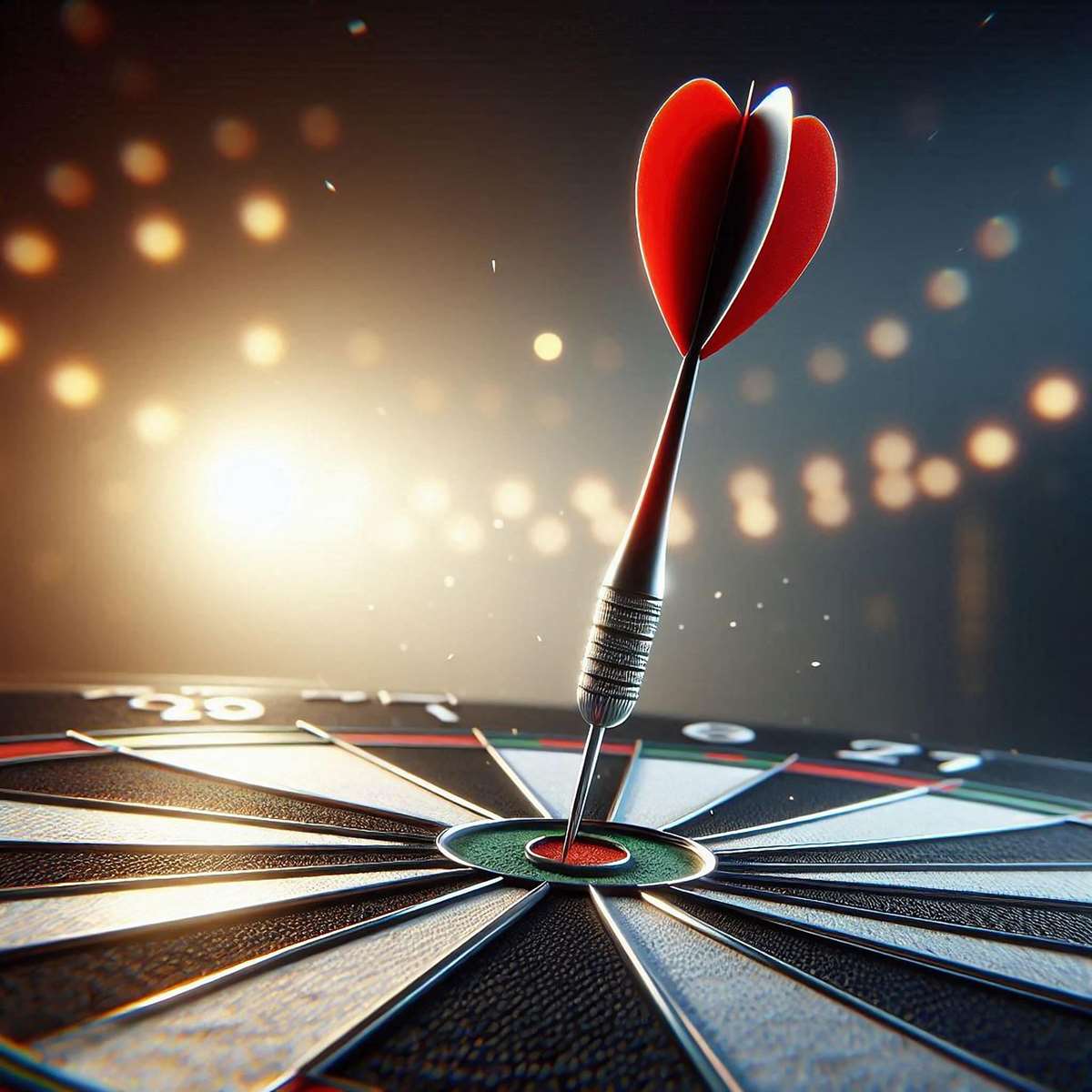
Think of isolates as separate Dart programs running concurrently on different cores (if available). They communicate through a channel similar to sending messages, preventing the complexities and risks of shared memory access. While not directly multi-threaded in the traditional sense, this mechanism enables a level of parallel processing that is both powerful and safe.
This approach contrasts sharply with true multi-threading, where threads share memory. In multi-threaded programming, careful synchronization is crucial to avoid data corruption. Dart’s use of isolates avoids this need, providing a safer and simpler way to achieve concurrency. This makes Dart well-suited for applications requiring high performance and responsiveness, such as those with complex UI updates or those performing long-running background tasks, like network requests or intensive computations. It’s a very important consideration when choosing which language and environment to use for a project.
Practical Applications of Isolates
Isolates are particularly beneficial when dealing with CPU-intensive tasks. Instead of blocking the main thread, which could freeze the UI, you can offload such tasks to a separate isolate. For instance, you could use an isolate to process large datasets or perform complex calculations without impacting the user interface responsiveness. This leads to a much smoother and more enjoyable user experience, even when dealing with resource-intensive operations.
Another excellent use case for isolates is handling long-running operations like network requests. By offloading these requests to an isolate, you prevent the main thread from becoming blocked, allowing the application to remain responsive to user interactions. When the request completes, the result is passed back to the main isolate, ensuring data consistency and predictability.
Consider the following scenario: you are building a mobile application that needs to download and process a large image from the internet. If you attempt to do this directly on the main thread, it will block the UI, causing a frozen user experience. Instead, by using an isolate to handle the download and processing, your UI stays responsive and the user has a seamless experience. This aspect is crucial for developing modern, user-friendly applications.
To use isolates in Dart, you’ll use the Isolate.spawn
function, which creates and starts a new isolate. Communication between the isolates happens using message passing using ports for sending and receiving data.
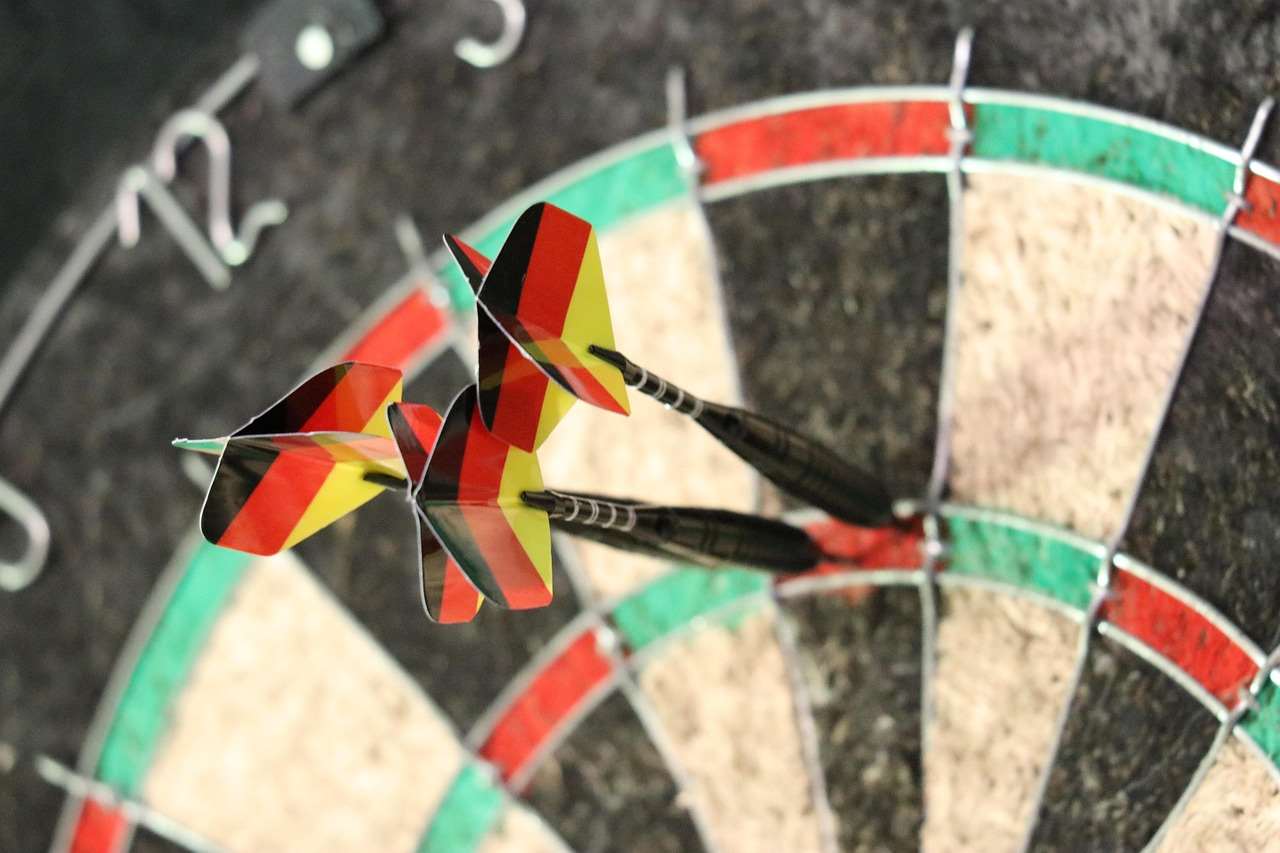
Example of Using Isolates in Dart
A simple example would be to run a computationally expensive function in a separate isolate:
import 'dart:isolate';
void main() async {
final receivePort = ReceivePort();
await Isolate.spawn(heavyComputation, receivePort.sendPort);
final result = await receivePort.first;
print('Result from isolate: $result');
receivePort.close();
}
void heavyComputation(SendPort sendPort) {
final result = performHeavyComputation();
sendPort.send(result);
}
int performHeavyComputation() {
// Simulate a time-consuming computation
var sum = 0;
for (var i = 0; i < 100000000; i++) {
sum += i;
}
return sum;
}
This example demonstrates how to separate computationally expensive operations into a different isolate to maintain a responsive UI. This pattern is extremely useful in improving performance and user experience within Dart applications.
Choosing the Right Approach: Single-Threaded vs. Multi-Threaded Concepts
The question, "dart is single threaded or multi threaded," is best answered with nuance. Dart's single-threaded nature makes it easier to write and maintain code. However, its powerful isolates provide the necessary mechanisms for achieving concurrent execution, harnessing the capabilities of multi-core processors and enhancing application performance. Choosing the right approach will depend on the specific needs of your application.
For simple applications with minimal concurrency needs, the simplicity of the single-threaded model might suffice. However, for complex applications involving long-running background tasks, extensive UI updates, or CPU-intensive operations, leveraging isolates will significantly improve responsiveness and performance. It's important to appreciate the trade-offs between the simplicity of a single-threaded approach and the scalability offered by isolates. You might want to explore how isolates can improve your application's performance, if possible. This ensures you are using the most suitable strategy for your projects.
Remembering that Dart's event loop is highly efficient at managing tasks within a single thread helps clarify this. For many common scenarios, efficient event loop management negates the need for more complex concurrency approaches. It is only when truly parallel processing is required that Isolates become the efficient solution. You may find this information to be quite useful when working on your next project.
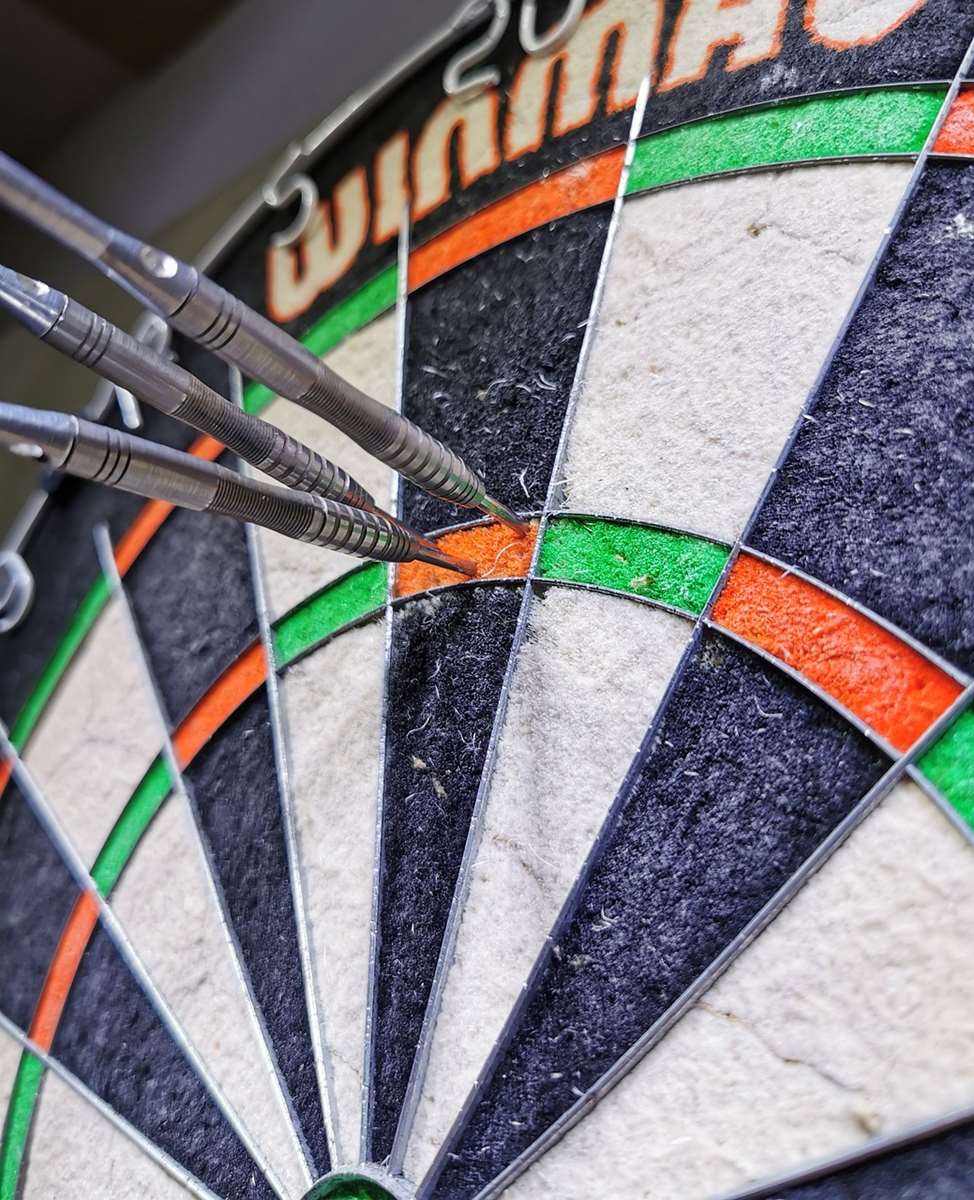
Understanding this fundamental aspect of Dart – that it's single-threaded but provides concurrency through isolates – allows developers to build applications that are both efficient and easy to maintain. The choice between utilizing the built-in single-threaded approach or leveraging Isolates for more intensive tasks is a key decision when designing modern Dart applications. Choosing between these options will depend on your project's needs and complexities.
Efficiently utilizing the Dart language requires a good understanding of when to embrace the simplicity of single-threaded programming and when to utilize the power of concurrency offered by isolates. Many resources are available online to help you better understand these concepts. Mastering these principles leads to well-structured, high-performance Dart applications.
Consider exploring using an App to score darts to help manage the scores within your dart games. Efficient score tracking can be quite helpful and improve the overall user experience.
Furthermore, you can utilize resources such as tutorials and documentation to guide your journey. This helps ensure you build well-structured, efficient applications. Effective use of Dart's capabilities directly contributes to the success of your projects.
Conclusion
The question, "dart is single threaded or multi threaded," highlights a key characteristic of the language: while fundamentally single-threaded, Dart offers robust concurrency through isolates. This approach balances the simplicity of a single-threaded model with the performance benefits of parallel processing. Understanding this duality is crucial for building high-performance, responsive Dart applications. By leveraging isolates strategically for CPU-intensive tasks and long-running operations, developers can create applications that are both efficient and easy to maintain. Remember to carefully evaluate your application's requirements to determine the optimal balance between single-threaded simplicity and isolate-based concurrency. Happy coding!
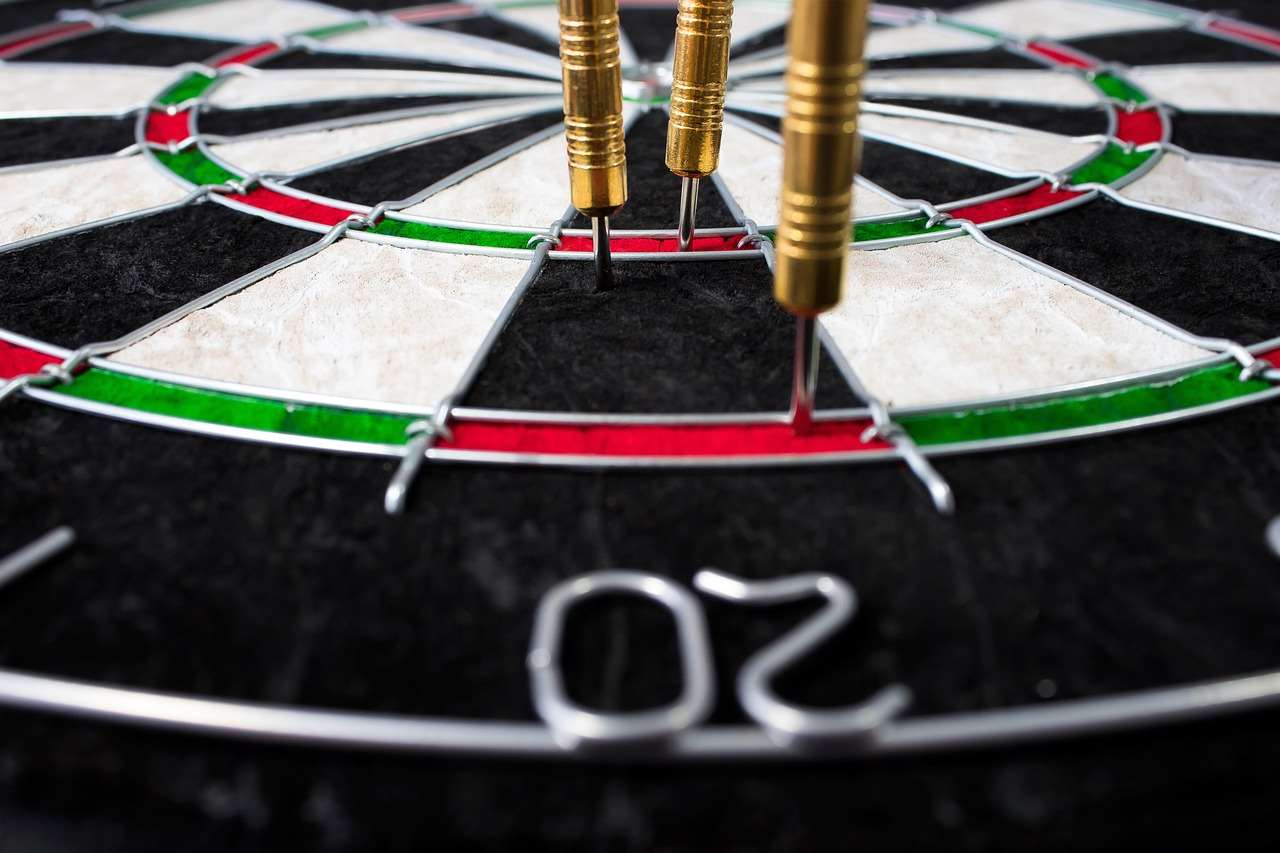
Remember to check out our other articles, like darts how to count points, dartboard electronic scoreboard, and single player dart games for more helpful tips and tricks!
Hi, I’m Dieter, and I created Dartcounter (Dartcounterapp.com). My motivation wasn’t being a darts expert – quite the opposite! When I first started playing, I loved the game but found keeping accurate scores and tracking stats difficult and distracting.
I figured I couldn’t be the only one struggling with this. So, I decided to build a solution: an easy-to-use application that everyone, no matter their experience level, could use to manage scoring effortlessly.
My goal for Dartcounter was simple: let the app handle the numbers – the scoring, the averages, the stats, even checkout suggestions – so players could focus purely on their throw and enjoying the game. It began as a way to solve my own beginner’s problem, and I’m thrilled it has grown into a helpful tool for the wider darts community.