A Dart HashMap is a fundamental data structure in Dart, offering efficient key-value pair storage and retrieval. This article will delve into its intricacies, exploring its implementation, usage, and best practices, equipping you with the knowledge to leverage this powerful tool effectively in your Dart applications.
⚠️ Still Using Pen & Paper (or a Chalkboard)?! ⚠️
Step into the future! The Dart Counter App handles all the scoring, suggests checkouts, and tracks your stats automatically. It's easier than you think!
Try the Smart Dart Counter App FREE!Ready for an upgrade? Click above!
The Dart HashMap, unlike lists which use numerical indices, utilizes unique keys to access associated values. This makes it perfect for scenarios where you need quick lookups based on specific identifiers, rather than sequential positions. For instance, imagine storing user data where each user’s ID acts as the key, and their details as the value. This is where the efficiency of a Dart HashMap truly shines.
Understanding the Dart HashMap
At its core, a Dart HashMap is an implementation of a hash table. This means it uses a hash function to map keys to indices within an underlying array, allowing for (on average) constant-time O(1) complexity for operations like insertion, deletion, and retrieval. This is a significant advantage over linear data structures such as lists, which have O(n) complexity for these operations (where n is the number of elements).
The hash function transforms the key into a numerical index, determining where the key-value pair is stored. Efficient hash functions minimize collisions (multiple keys mapping to the same index), ensuring optimal performance. The Dart language provides built-in support for HashMaps through the `Map` class, making their integration into your projects straightforward. You can create a new Dart HashMap using the literal syntax or by using the `Map()` constructor.
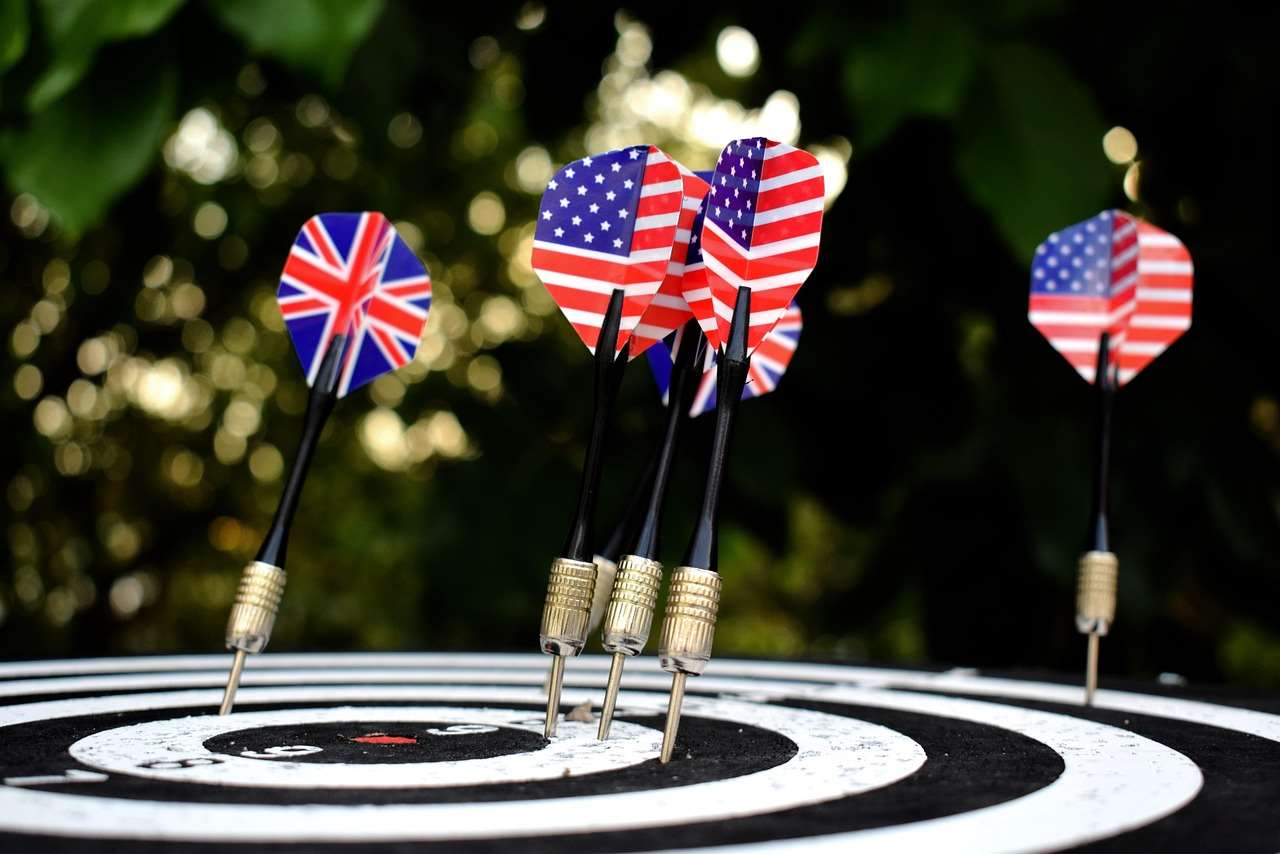
Here’s an example of creating and using a Dart HashMap:
void main() {
// Using literal syntax
Map ages = {
'Alice': 30,
'Bob': 25,
'Charlie': 35,
};
// Using the Map() constructor
Map capitals = Map();
capitals['France'] = 'Paris';
capitals['Germany'] = 'Berlin';
print(ages['Alice']); // Output: 30
print(capitals['France']); // Output: Paris
}
As you can see, accessing values is done using the key within square brackets. The example showcases how to initialize a Dart HashMap with string keys and integer/string values. You can adapt this to any data type you need. Remember that keys must be unique; attempting to assign a value to an existing key will simply overwrite the previous value.
Key Features and Methods of Dart HashMap
The Dart HashMap provides several useful methods beyond basic insertion and retrieval. Some crucial methods include:
containsKey(key)
: Checks if the map contains a specific key.containsValue(value)
: Checks if the map contains a specific value.remove(key)
: Removes the key-value pair associated with the given key.clear()
: Removes all entries from the map.length
: Returns the number of key-value pairs in the map.keys
: Returns an iterable of all keys in the map.values
: Returns an iterable of all values in the map.entries
: Returns an iterable of all key-value pairs (MapEntry).forEach(function)
: Iterates through each key-value pair and applies a given function.
Mastering these methods will significantly enhance your ability to manipulate and utilize Dart HashMaps effectively. Understanding the behavior of each method is crucial for writing robust and efficient Dart code.
Practical Applications of Dart HashMaps
Dart HashMaps are invaluable across many Dart programming tasks. Their ability to offer fast lookups makes them ideal for:
- Caching data: Store frequently accessed data for quick retrieval, improving performance.
- Representing graphs and trees: Nodes can be represented as keys, and their connections as values, enabling efficient graph traversal.
- Implementing counters: Track the frequency of occurrences of specific items using items as keys and their counts as values.
- Data serialization and deserialization: Convert data structures to and from JSON using keys as identifiers.
- Game development: Store game objects and their properties efficiently.
These are just a few examples; the versatility of the Dart HashMap makes it a cornerstone of many effective applications.
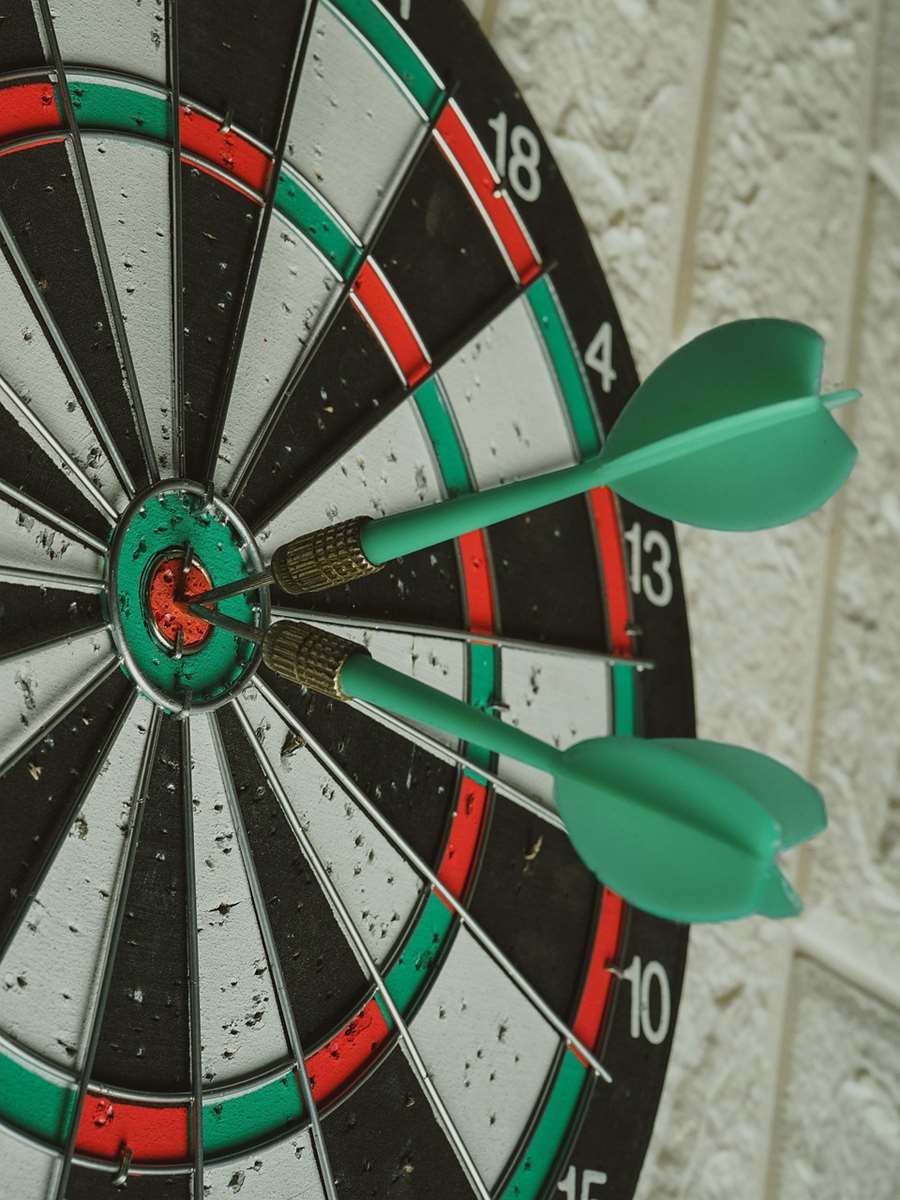
Consider scenarios where you need to track player scores in a game. A Dart HashMap would be perfect. The player’s name could be the key, and their score the value. Updating the score is a simple matter of accessing the key and modifying the associated value. This approach is far more efficient than searching through a list every time you need to update or retrieve a player’s score. Using a Dart HashMap in such situations ensures optimal performance even with a large number of players.
Advanced Techniques and Considerations
While Dart HashMaps are generally easy to use, there are some advanced techniques and considerations to keep in mind for optimal performance and code maintainability:
- Choosing the right key type: Keys must implement the `hashCode` and `==` operators correctly. This ensures that the hash function works effectively and that key comparisons are accurate.
- Handling collisions: Although Dart’s implementation manages collisions efficiently, understanding how they’re handled can help you write more robust code. While rare, extreme cases can lead to performance degradation.
- Memory management: Large HashMaps can consume significant memory. Efficient memory usage is particularly important in resource-constrained environments.
- Iterating efficiently: Use the `forEach` method for efficient iteration, avoiding unnecessary key lookups.
By understanding these advanced aspects, you’ll be able to build more efficient and scalable applications utilizing Dart HashMaps.
Working with Null Safety
Dart’s null safety features play an important role when dealing with Dart HashMaps. It’s crucial to handle potential null values correctly. Using the null-aware operator (`?.`) when accessing values will help prevent runtime errors if a key doesn’t exist. For example, instead of `print(myMap[‘missingKey’]);`, use `print(myMap[‘missingKey’]?.toString());` or leverage the `containsKey()` method before accessing the value. This practice is fundamental to creating reliable code within a null-safe context. Using a dartcounter op laptop might be useful in larger projects.
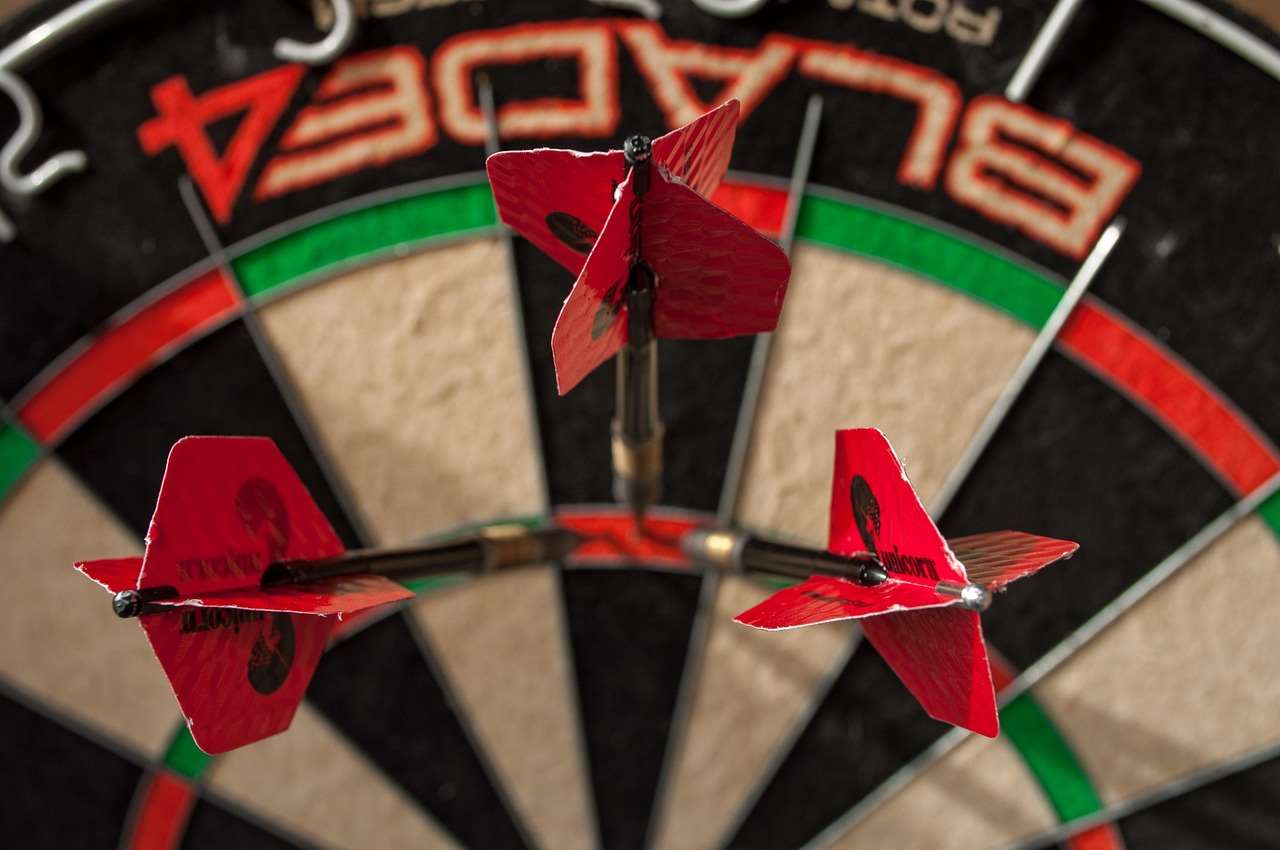
Comparing Dart HashMap with Other Data Structures
It’s helpful to compare Dart HashMaps with other data structures to understand their strengths and weaknesses:
- Lists: Lists provide sequential access but lack the speed of hash map lookups. They are more appropriate for ordered data where the index matters.
- Sets: Sets are unordered collections of unique elements. Use them when you only need to store unique values without associating them with other data.
The choice between these data structures depends heavily on the specific needs of your application. For quick key-based lookups, Dart HashMaps are undoubtedly the superior choice. In other cases, lists or sets might be more suitable, depending on the order and uniqueness requirements of your data.
Troubleshooting and Common Errors
When working with Dart HashMaps, common errors include:
- Incorrect key types: Ensure keys correctly implement `hashCode` and `==`.
- Null key errors: Avoid using null keys; they are not allowed in Dart HashMaps. Use null safety features to prevent runtime errors.
- Unhandled exceptions: Handle potential exceptions, such as `NoSuchMethodError`, when using HashMap methods.
Careful error handling and adherence to best practices will significantly improve the robustness and reliability of your applications. Using a Dart game scoring app can help with some of these debugging tasks.
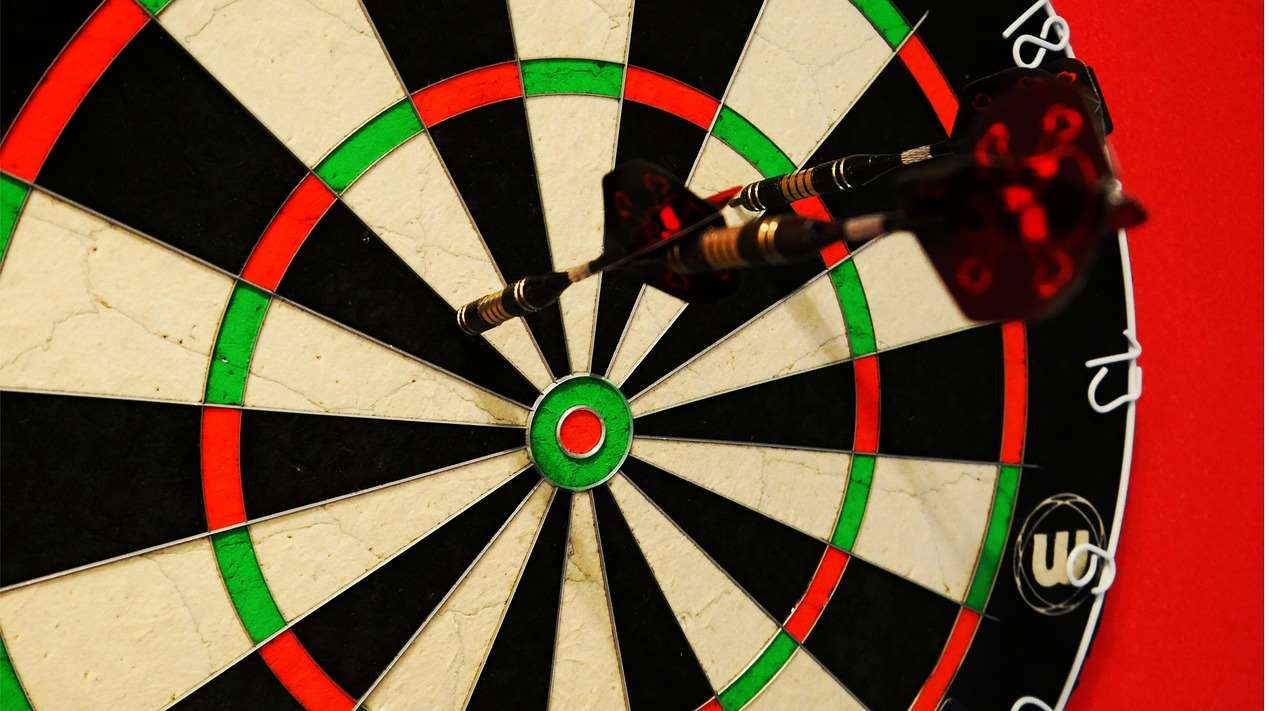
Remember that while Dart HashMaps are incredibly powerful and efficient, they may not always be the best choice for every situation. Carefully consider your data structure needs before selecting the optimal solution for your application. A well-chosen data structure can dramatically improve the efficiency and maintainability of your Dart projects. For instance, when dealing with large datasets, optimizing your Dart HashMap usage can reduce memory consumption and improve performance significantly. Proper understanding of how to work with these key-value pairs is fundamental for any serious Dart programmer. Furthermore, remember that efficient data structures are a cornerstone of effective software engineering, and mastering Dart HashMaps is a crucial step in improving your overall programming skills.
Conclusion
This comprehensive guide has explored the essential aspects of Dart HashMaps, covering their implementation, usage, common applications, and advanced techniques. We’ve highlighted the importance of selecting the correct data structures for different scenarios, and emphasized the benefits of utilizing Dart HashMaps for quick and efficient key-based access. You’ve learned about various techniques, including efficient iteration, handling null safety, and troubleshooting potential errors. By mastering these concepts, you’ll be well-equipped to effectively integrate Dart HashMaps into your projects and write high-performance Dart applications. Remember to leverage the built-in methods, such as `containsKey()` and `remove()`, to streamline your code and avoid common pitfalls. If you’re a dedicated darts player, you might find interesting resources on dart songs.
Now, it’s time to put your new knowledge into practice! Start experimenting with Dart HashMaps in your own projects and witness the performance boost they provide. Remember to check out the official Dart documentation for even more in-depth information. Start building those amazing apps leveraging the power of the Dart HashMap!
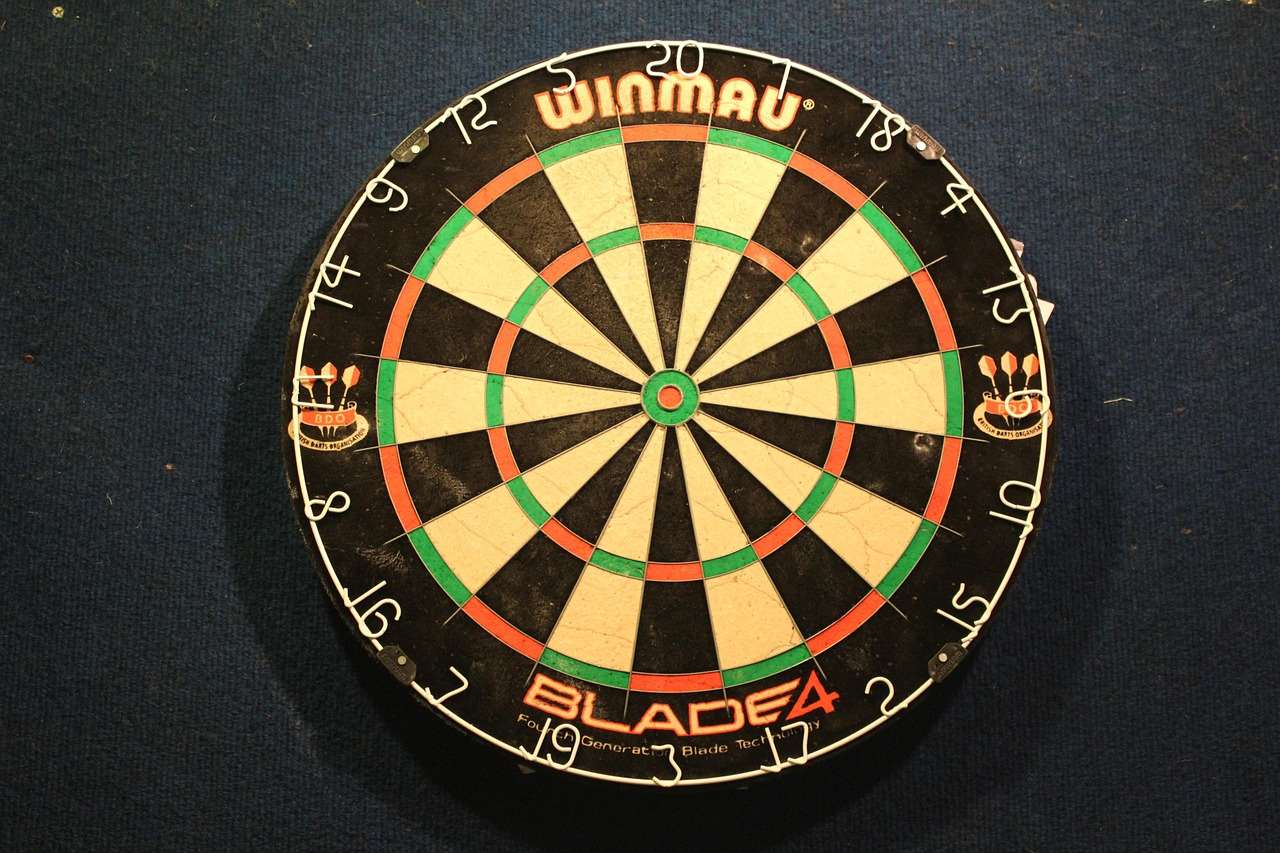
And for additional resources, you might find information on darts double knee, darts match treble, dart newark nj, or darts corner manchester interesting.
Hi, I’m Dieter, and I created Dartcounter (Dartcounterapp.com). My motivation wasn’t being a darts expert – quite the opposite! When I first started playing, I loved the game but found keeping accurate scores and tracking stats difficult and distracting.
I figured I couldn’t be the only one struggling with this. So, I decided to build a solution: an easy-to-use application that everyone, no matter their experience level, could use to manage scoring effortlessly.
My goal for Dartcounter was simple: let the app handle the numbers – the scoring, the averages, the stats, even checkout suggestions – so players could focus purely on their throw and enjoying the game. It began as a way to solve my own beginner’s problem, and I’m thrilled it has grown into a helpful tool for the wider darts community.