In Dart, to **dart get max int**, you’ll typically use `double.infinity` because Dart doesn’t have a true maximum integer like some other languages. `double.infinity` effectively represents a value larger than any other number you’d realistically work with. This article will explore how to use this concept, potential alternative approaches, and considerations for various scenarios when working with numbers in Dart.
⚠️ Still Using Pen & Paper (or a Chalkboard)?! ⚠️
Step into the future! The Dart Counter App handles all the scoring, suggests checkouts, and tracks your stats automatically. It's easier than you think!
Try the Smart Dart Counter App FREE!Ready for an upgrade? Click above!
Understanding Numeric Limits and `dart get max int` Alternatives
When dealing with numbers in programming, it’s often important to understand their limits. While Dart doesn’t offer a predefined constant representing the absolute maximum integer value directly, there are ways to achieve similar results or work around this limitation. Understanding the different numeric types in Dart is crucial for effective number handling.
Dart supports several numeric types, including:
- int: Represents integer values.
- double: Represents floating-point values.
While `int` has a bounded range, `double` includes special values like `double.infinity` and `double.negativeInfinity`, which can be useful for representing extremely large or small numbers. For scenarios requiring the functional equivalent of **dart get max int**, exploring these options is essential.
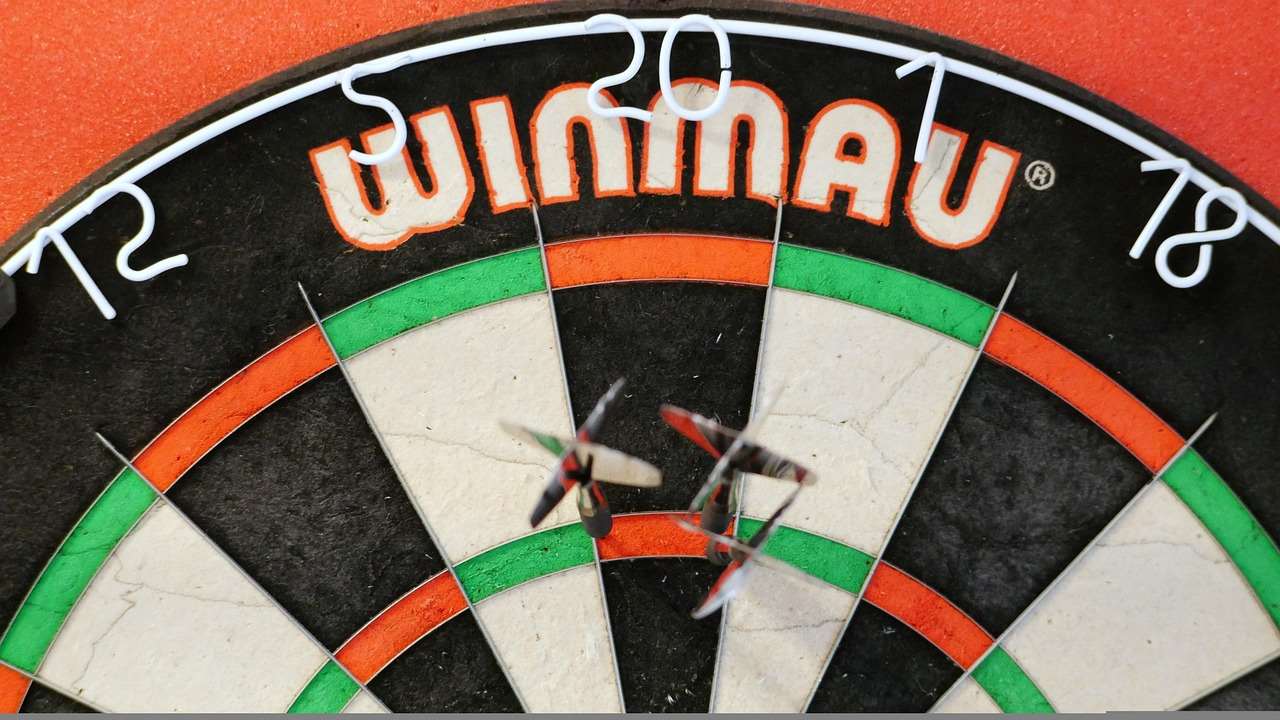
Using `double.infinity` as a Proxy for Maximum Value
The most common approach when seeking a representation of the “maximum” value in Dart is to use `double.infinity`. This value effectively serves as a marker for a number larger than any other finite `double` value. This is useful in scenarios such as:
- Initializing variables to track minimum values: You can initialize a variable to `double.infinity` and then compare it with other values to find the true minimum.
- Representing unbounded values: When a value could potentially grow indefinitely, `double.infinity` can accurately reflect this behavior.
Here’s a simple example:
void main() {
double maxValue = double.infinity;
print('Maximum value: $maxValue'); // Output: Maximum value: Infinity
}
Keep in mind that while `double.infinity` is a useful tool, it behaves differently than a true maximum integer. Arithmetic operations involving infinity can produce unexpected results, such as `double.infinity + 1` still being equal to `double.infinity`. Understanding these nuances is vital.
Alternatives and Considerations for Handling Large Numbers
While `double.infinity` is a good option, depending on your use case, alternative solutions could be more appropriate. If you are specifically concerned with the maximum **dart integer value**, the `int` data type may present challenges. Some alternatives for handing large numbers include:
- Using a library for arbitrary-precision arithmetic: Packages like `bignum` on Pub.dev offer the capability to work with numbers of virtually unlimited size. This can be useful for cryptographic applications or financial calculations where precision is paramount. Understanding which dart shafts are best can be useful in optimizing other aspects of your game.
- Custom implementation: In some cases, implementing a custom solution for handling large numbers might be necessary. This could involve representing numbers as strings or arrays of digits.
- Using `double` with caution: While `double` can represent very large numbers, it’s important to be aware of potential precision issues due to its floating-point representation.
Consider the following example using the `bignum` package:
import 'package:bignum/bignum.dart';
void main() {
BigInteger largeNumber = BigInteger.parse('123456789012345678901234567890');
print('Large number: $largeNumber');
}
This approach is particularly useful when the potential for exceeding the `int` range is a genuine concern, and accuracy is critical.
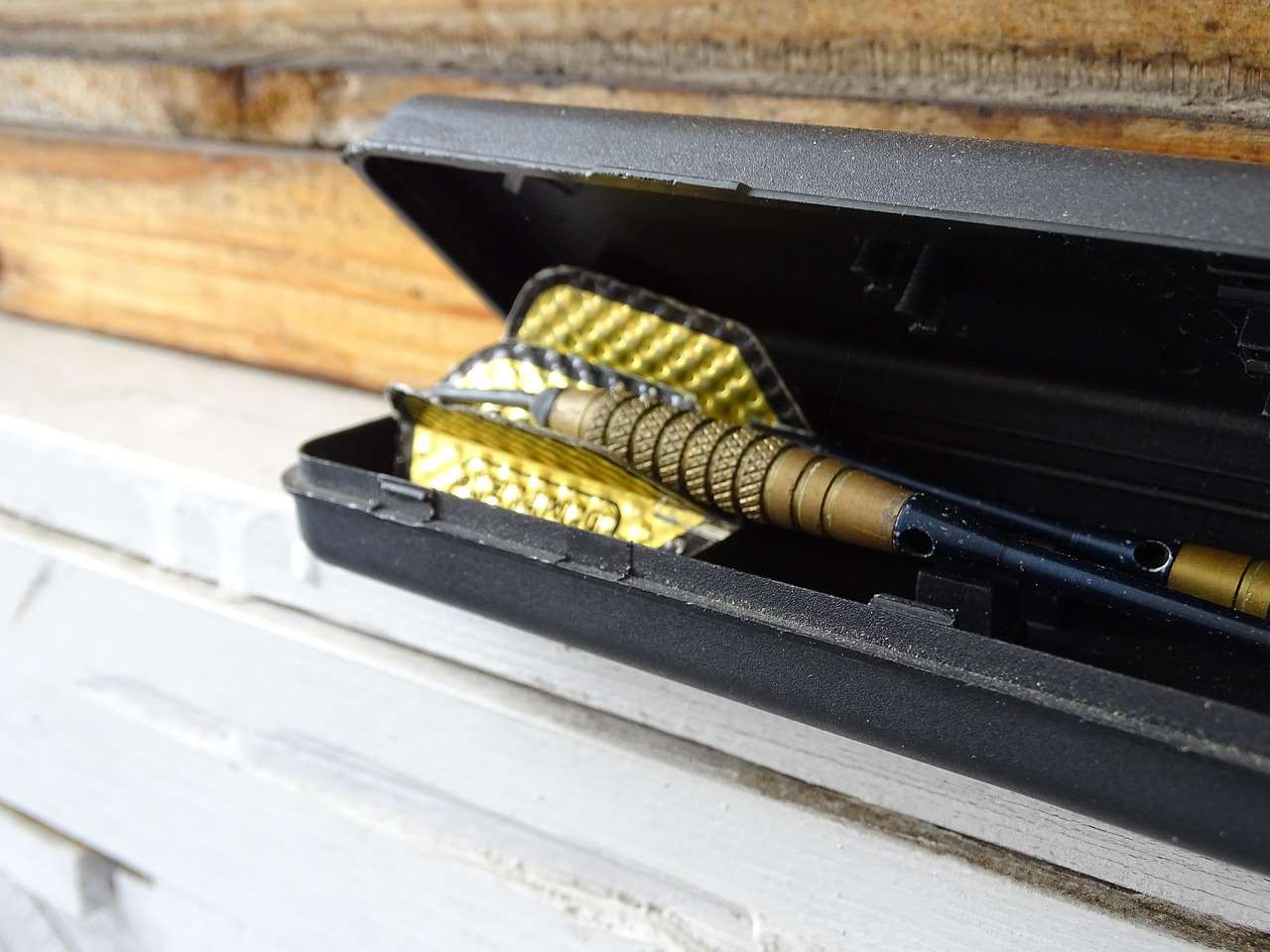
Practical Scenarios Where `dart get max int` Matters
Understanding how to represent or approximate the maximum value is important in several practical scenarios:
- Data Validation: Ensuring that input values are within acceptable ranges.
- Algorithm Design: Optimizing algorithms that depend on maximum value constraints.
- Handling Extreme Cases: Developing robust applications that can gracefully handle potentially unbounded values.
- Initializing Comparison Variables: When searching for minimum values.
For instance, in data validation, you might want to check if an integer is within a specific range. In this case, you could use `double.infinity` to represent an upper bound, albeit with the understanding that you’re working with `double` semantics.
Error Handling and Edge Cases
When working with numbers and potential maximum value approximations, thorough error handling is critical. Always consider edge cases where calculations might overflow or produce unexpected results.
- Overflow: Be mindful of potential integer overflows. Use `try-catch` blocks to handle exceptions if necessary.
- Precision Loss: Understand that `double` values have limited precision. Avoid relying on exact equality comparisons.
- Division by Zero: Always check for division by zero errors before performing division operations. Consider how to increase your chances for a world cup of darts nine darter by perfecting your technique.
The choice between using `double.infinity` and other approaches depends on the specific requirements of your application and the level of precision and robustness required.
Best Practices for Numeric Operations in Dart
To ensure that your Dart code is reliable and performs as expected when dealing with numeric operations, follow these best practices:
- Use appropriate data types: Choose the data type that best fits the range and precision requirements of your data. Use `int` when you need integers and `double` when you need floating-point numbers.
- Validate input data: Always validate input data to prevent errors and ensure that it’s within expected ranges.
- Handle potential errors: Use `try-catch` blocks to handle potential errors, such as overflow and division by zero.
- Consider using libraries: For complex numeric operations, consider using libraries that provide advanced functionality and error handling.
- Document your code: Clearly document your code to explain the assumptions and limitations of your numeric operations.
By adhering to these best practices, you can write more robust and maintainable Dart code that effectively handles numeric operations, including scenarios where approximating or representing maximum values becomes crucial.
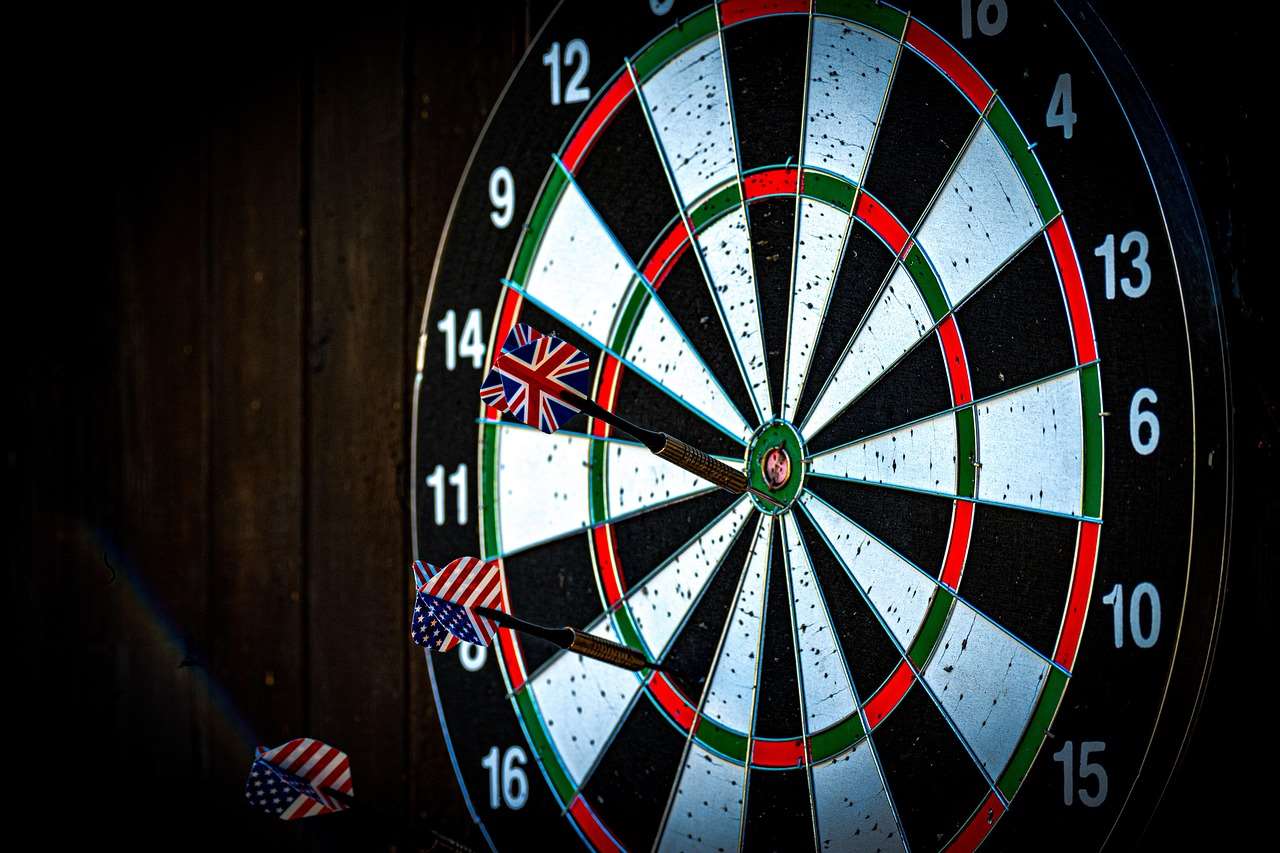
Leveraging Packages and Libraries
Dart’s ecosystem offers a variety of packages and libraries designed to simplify numeric operations and provide enhanced features. Consider exploring these options:
- `decimal` package: For precise decimal arithmetic. This is useful for financial calculations where accuracy is paramount.
- `fraction` package: For working with fractions and rational numbers.
- `complex` package: For handling complex numbers.
By leveraging these packages, you can avoid reinventing the wheel and benefit from well-tested and optimized solutions.
Comparing Dart’s Numeric Handling to Other Languages
Dart’s approach to numeric limits differs somewhat from other programming languages. For example:
- Java: Provides constants like `Integer.MAX_VALUE` and `Double.MAX_VALUE`.
- C++: Uses `INT_MAX` and `DBL_MAX` defined in the `
` header. - Python: Doesn’t have built-in maximum integer limits due to its arbitrary-precision integer implementation (up to system memory constraints).
Dart’s reliance on `double.infinity` as a proxy for the maximum value is a characteristic feature of its design. Looking for a new Best darts scoring app to keep track of all these calculations?
Testing Numeric Boundary Conditions
When working with numeric values in Dart, it’s important to write thorough tests to ensure your code behaves correctly under various conditions, including boundary conditions. Test cases should include:
- Maximum and minimum values: Test with `double.infinity` and `double.negativeInfinity`, as well as the largest and smallest possible `int` values.
- Edge cases: Test with values close to the boundaries, such as one less than or one greater than the maximum value.
- Zero: Test with zero values to ensure your code handles them correctly.
By writing comprehensive tests, you can identify and fix potential issues before they cause problems in production.
Debugging Numeric Issues
Debugging numeric issues can be challenging, especially when dealing with floating-point numbers and potential precision problems. Here are some tips for debugging numeric issues in Dart:
- Use the debugger: Step through your code and inspect the values of variables to identify where errors occur.
- Print values: Add `print` statements to your code to output the values of variables at various points.
- Use a numeric debugger: Some IDEs provide specialized debuggers for numeric code that can help you visualize and analyze numeric values.
Careful debugging practices can save a lot of time when facing unexpected numeric behavior.
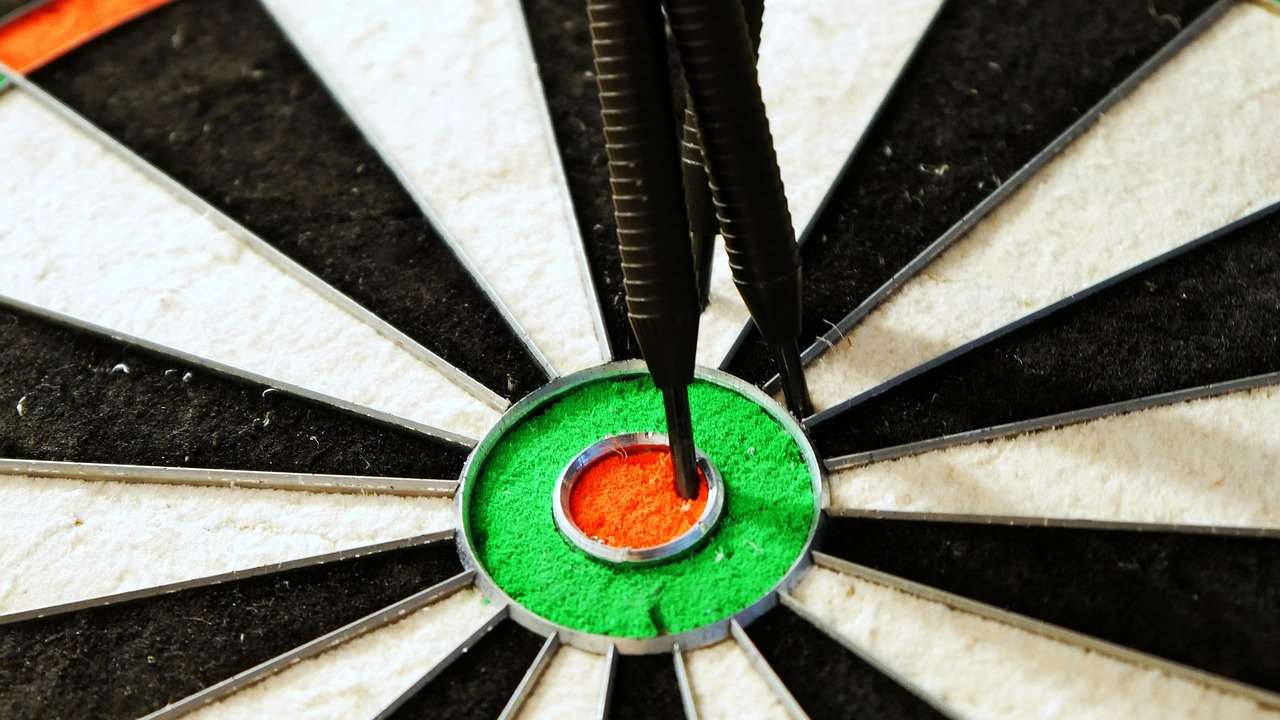
Advanced Numeric Techniques
For more advanced scenarios, consider these techniques:
- Bitwise operations: For manipulating individual bits in integers.
- Matrix operations: Using packages like `vector_math` for linear algebra.
- Statistical analysis: Using packages like `stats` for statistical calculations.
These advanced techniques can expand your capabilities when working with numbers in Dart, especially for specialized applications.
How `dart get max int` influences Algorithm Design
The representation of a “maximum value” significantly affects algorithm design, especially when initializing variables for finding minimum values or setting bounds for iterative processes. Using `double.infinity` allows developers to define an initial state that’s guaranteed to be larger than any encountered real value, streamlining comparison and optimization steps within algorithms.
Integrating `dart get max int` with other Dart features
`double.infinity` and other numeric handling techniques can be seamlessly integrated with other Dart features. For example:
- Streams and asynchronous programming: Use these numeric techniques when processing data from streams or performing asynchronous calculations.
- Flutter UI: Display numeric data in Flutter UI elements and handle user input of numeric values. The darts masters lineup is always a topic of interest, providing a good example for data to be rendered.
- Server-side Dart: Use numeric techniques in server-side Dart applications for data processing, calculations, and API development.
Dart’s flexibility allows you to apply these numeric techniques across various aspects of your applications.
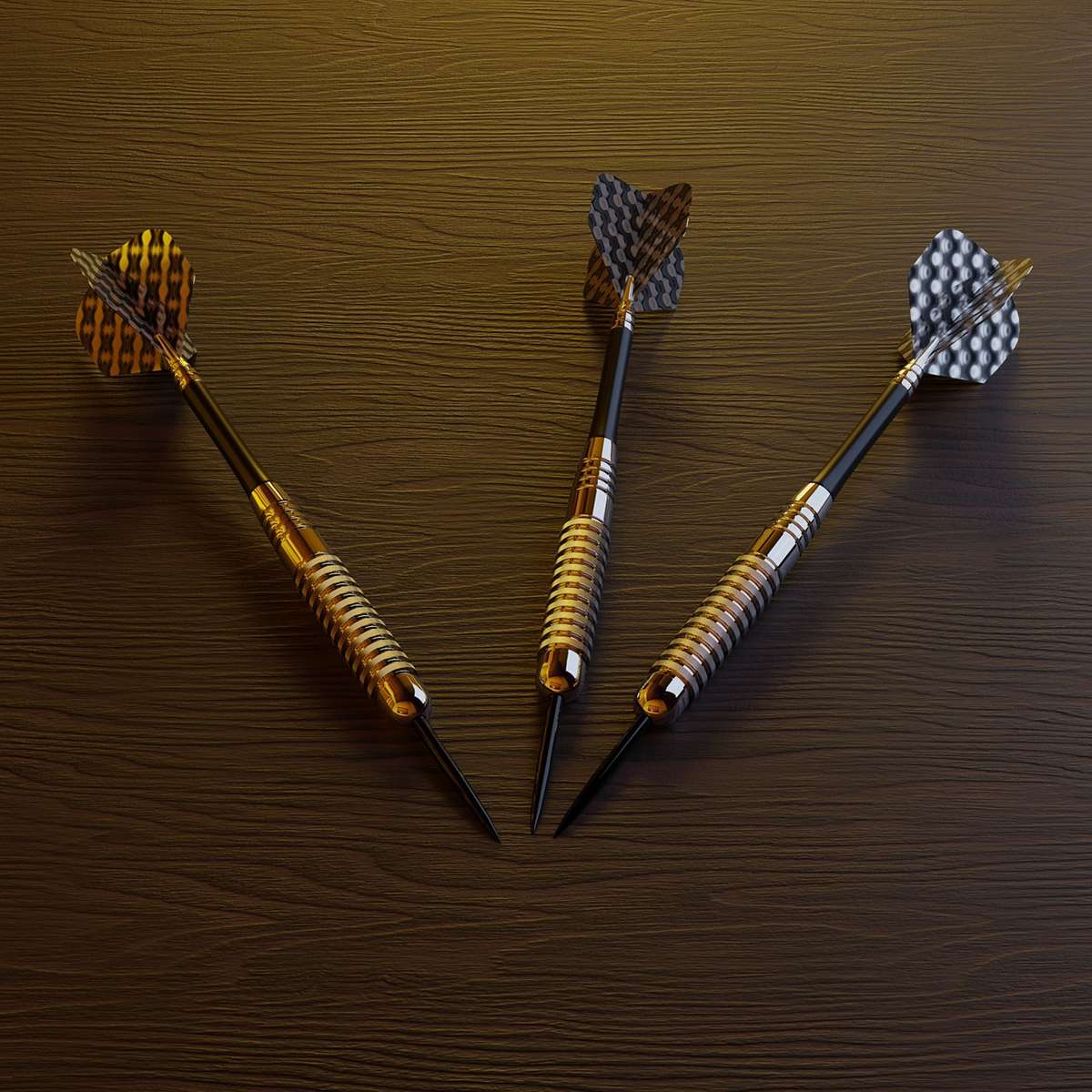
Conclusion
While Dart lacks a specific constant for a maximum integer value equivalent to `Integer.MAX_VALUE` in Java or `INT_MAX` in C++, the use of `double.infinity` provides a functional equivalent in most scenarios. Understanding how to effectively use this value, along with exploring alternatives such as arbitrary-precision arithmetic libraries, allows you to handle large numbers and numeric limits effectively in your Dart applications. Remember to consider potential precision issues and edge cases when working with floating-point numbers, and always validate your input data. Now that you know how to **dart get max int**, practice implementing these techniques in your own Dart projects! Consider exploring the darts set gary anderson for quality gear to enhance your game.
Hi, I’m Dieter, and I created Dartcounter (Dartcounterapp.com). My motivation wasn’t being a darts expert – quite the opposite! When I first started playing, I loved the game but found keeping accurate scores and tracking stats difficult and distracting.
I figured I couldn’t be the only one struggling with this. So, I decided to build a solution: an easy-to-use application that everyone, no matter their experience level, could use to manage scoring effortlessly.
My goal for Dartcounter was simple: let the app handle the numbers – the scoring, the averages, the stats, even checkout suggestions – so players could focus purely on their throw and enjoying the game. It began as a way to solve my own beginner’s problem, and I’m thrilled it has grown into a helpful tool for the wider darts community.