Converting a dart double to int is a common task in Dart programming. The simplest way is using the toInt()
method. This article will delve deeper into this conversion, exploring different approaches, handling potential issues, and providing practical examples to solidify your understanding.
⚠️ Still Using Pen & Paper (or a Chalkboard)?! ⚠️
Step into the future! The Dart Counter App handles all the scoring, suggests checkouts, and tracks your stats automatically. It's easier than you think!
Try the Smart Dart Counter App FREE!Ready for an upgrade? Click above!
Before we jump into the nitty-gritty of dart double to int conversion, let’s consider why you might need to perform such an operation. Often, you’ll find yourself working with floating-point numbers (doubles) which represent continuous values, but your application requires integer values for certain calculations, data storage, or display purposes. Understanding the nuances of this conversion is crucial for writing robust and efficient Dart code.
This isn’t simply about chopping off the decimal part; we’ll examine how to handle rounding and truncation correctly to achieve the desired outcome. We’ll also consider the implications of potential data loss when converting from a double to an int. By the end of this article, you will be confident in selecting the most appropriate method for your specific needs.
Understanding the toInt()
Method for Dart Double to Int Conversion
The most straightforward approach to converting a dart double to int is employing the built-in toInt()
method. This method performs truncation, meaning it simply discards the fractional part of the double, leaving only the integer portion. Let’s illustrate this with an example:
double myDouble = 3.75;
int myInt = myDouble.toInt(); // myInt will be 3
print(myInt); // Output: 3
double anotherDouble = -2.2;
int anotherInt = anotherDouble.toInt(); // anotherInt will be -2
print(anotherInt); // Output: -2
As you can see, the toInt()
method effectively removes the decimal component without any rounding. While simple, this approach might not always be ideal, especially when dealing with situations that require rounding to the nearest integer. For more advanced scenarios, other techniques provide greater flexibility.
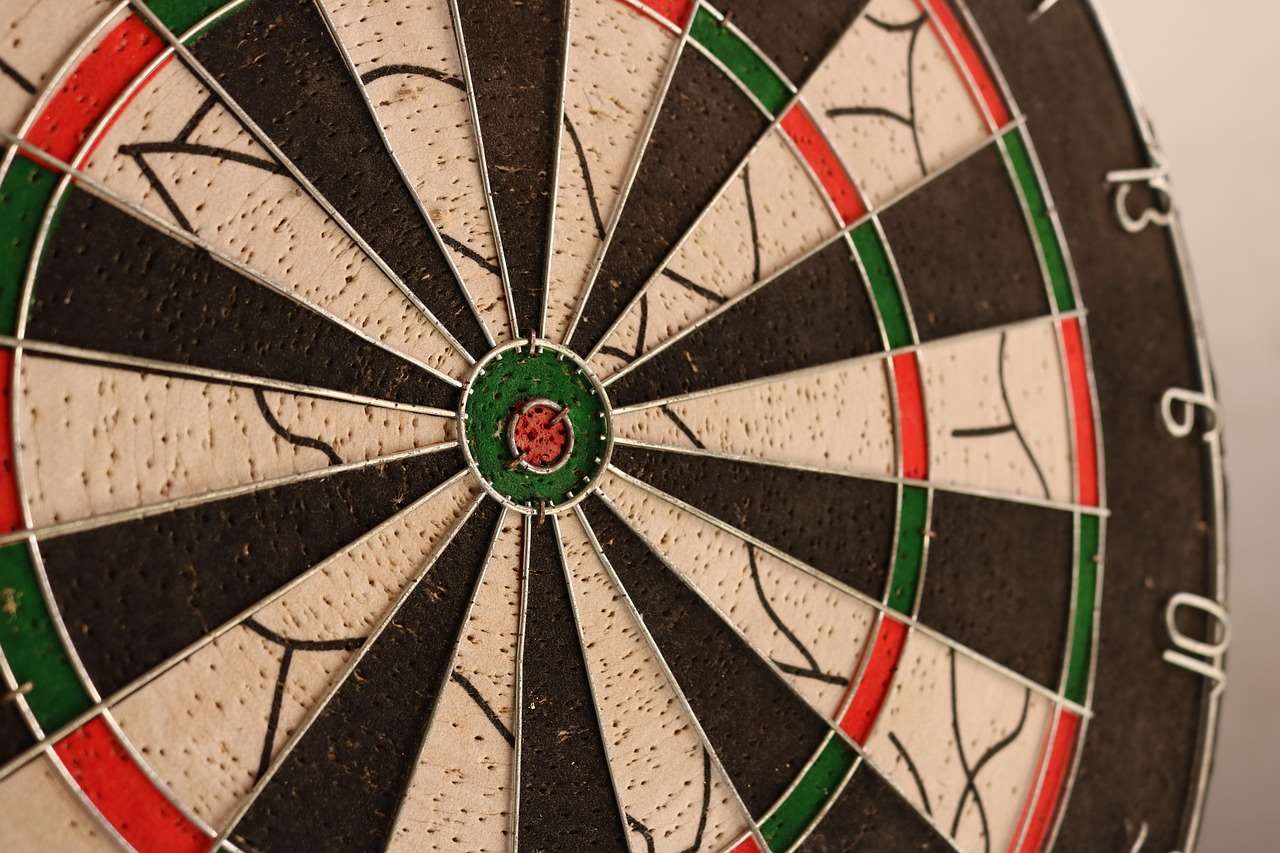
Alternative Approaches for Dart Double to Int Conversions
Rounding to the Nearest Integer
In scenarios where you need to round a dart double to int instead of simply truncating, you can use the round()
method. This method rounds the double to the nearest integer, using standard rounding rules (0.5 rounds up):
double myDouble = 3.75;
int myInt = myDouble.round(); // myInt will be 4
print(myInt); // Output: 4
double anotherDouble = 3.2;
int anotherInt = anotherDouble.round(); // anotherInt will be 3
print(anotherInt); // Output: 3
double negativeDouble = -2.7;
int negativeInt = negativeDouble.round();//negativeInt will be -3
print(negativeInt); // Output: -3
The round()
method provides a more accurate representation in cases where the fractional part is close to 0.5. Consider its use when precision is paramount.
Floor and Ceiling Functions
Dart also offers floor()
and ceil()
methods for rounding down and up, respectively. These are useful for specific rounding needs beyond simple nearest-integer rounding. The floor()
method always rounds down to the nearest integer, while ceil()
always rounds up. This is helpful when you need to ensure you are always above or below a certain value.
double myDouble = 3.75;
int myIntFloor = myDouble.floor(); // myIntFloor will be 3
int myIntCeil = myDouble.ceil(); // myIntCeil will be 4
print(myIntFloor); // Output: 3
print(myIntCeil); // Output: 4
The choice between floor()
, ceil()
, and round()
depends entirely on the specific requirements of your application. Understanding their differences is key to selecting the correct method for your dart double to int conversion.
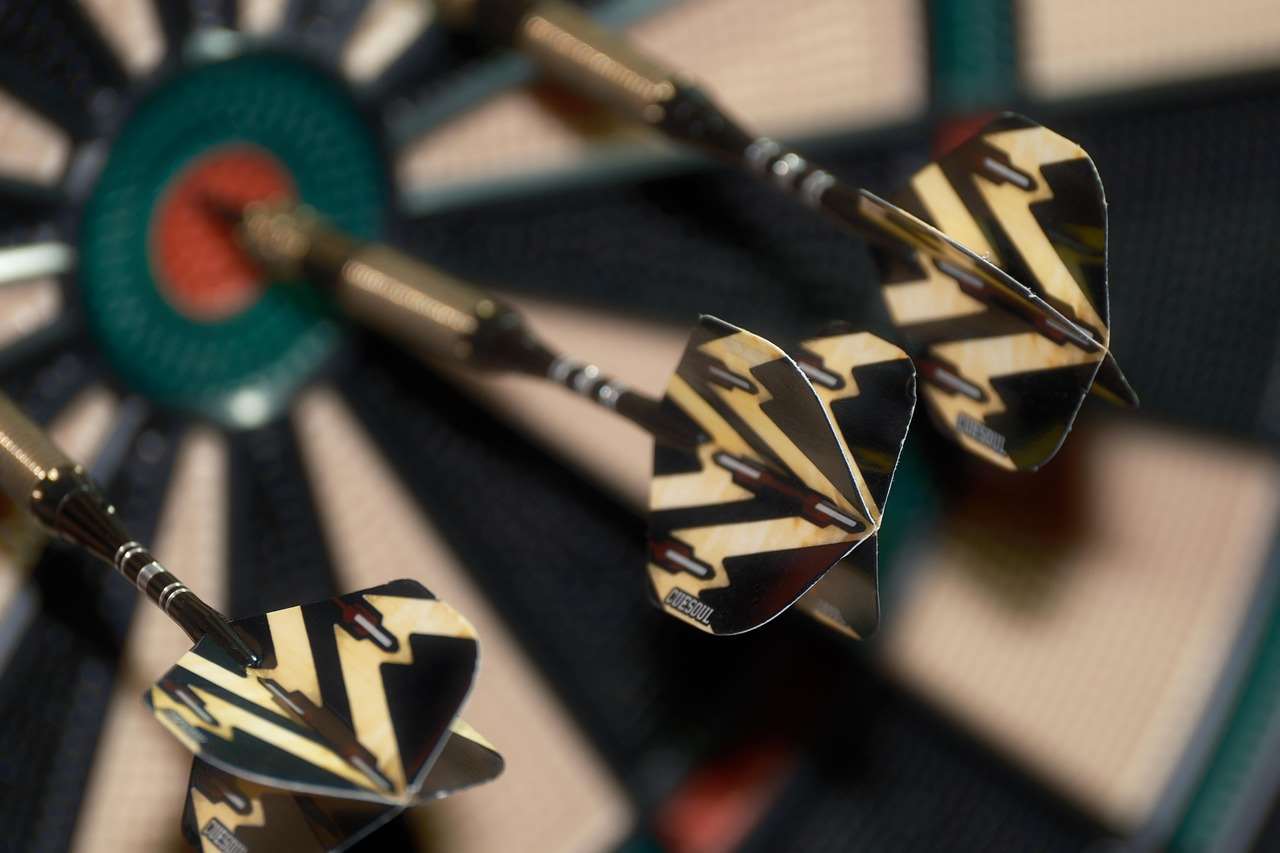
Error Handling and Data Loss
When converting from a double to an int, it’s important to be aware of the potential for data loss. Since integers cannot represent fractional values, any decimal portion will be discarded during the conversion. This isn’t necessarily a problem, but it’s crucial to understand the implications and handle them appropriately. In some cases, it might be necessary to preserve precision by keeping the value as a double, or utilizing different data types, but sometimes this cannot be avoided.
Consider scenarios where even minor inaccuracies could significantly impact the result. In such cases, you might need to implement error handling or choose a different approach altogether. For instance, if dealing with financial calculations, losing even a fraction of a cent could accumulate into substantial errors over time.
For applications involving financial data, it is crucial to select appropriate precision for your values, even if it means using more complex data types. You might consider using a library specializing in precise decimal arithmetic, instead of directly converting your dart double to int.
Best Practices for Dart Double to Int Conversions
- Choose the right rounding method: Select the rounding technique (
toInt()
,round()
,floor()
, orceil()
) that best suits your application’s needs and accuracy requirements. - Handle potential errors: Be aware of the potential for data loss and implement appropriate error handling mechanisms if necessary.
- Consider alternative data types: If precision is critical, explore using data types that maintain fractional values, such as
double
or specialized libraries for decimal arithmetic. - Document your choices: Clearly document the chosen method and its implications in your code comments.
By following these best practices, you can ensure your dart double to int conversions are both accurate and reliable.
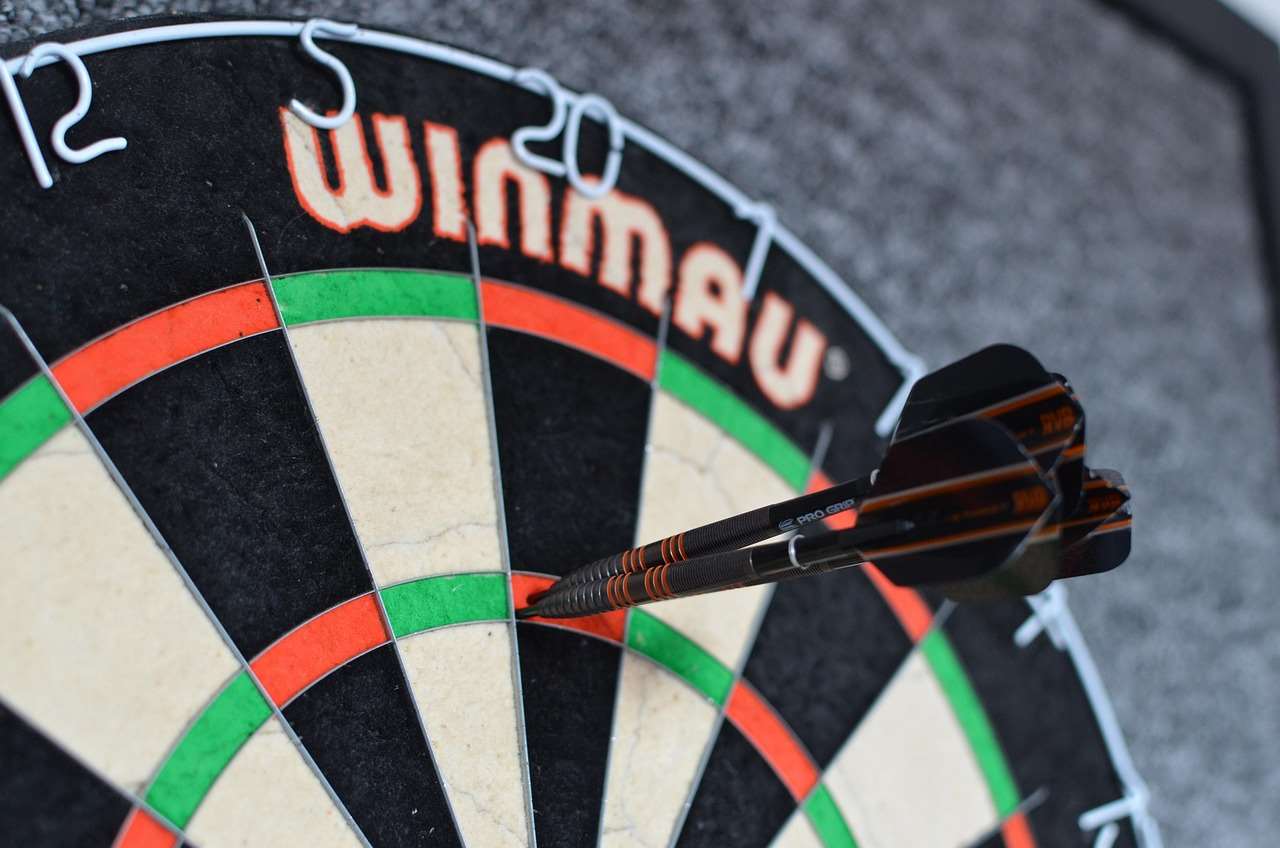
Advanced Scenarios and Considerations
While the basic methods cover most common dart double to int conversions, some scenarios might require more sophisticated techniques. For example, you might need to handle extremely large doubles or perform conversions within specific ranges. These situations often necessitate careful consideration of potential overflow or underflow issues. Understanding these potential pitfalls is crucial for writing reliable and efficient code.
Furthermore, certain applications might require conversions to conform to particular standards or formats. For instance, you might need to format an integer output in a specific way, such as adding leading zeros or padding for consistency.
Remember to always test your conversion logic thoroughly to ensure accuracy across different input values. Testing with edge cases, such as very large or very small numbers, near-zero values, and negative numbers, is vital to revealing potential issues in your dart double to int conversion process. Comprehensive testing is crucial for avoiding unexpected runtime errors.
Consider using automated testing frameworks to systematically test your conversion routines, ensuring that the results are always as expected under various conditions. Automated testing greatly simplifies the process of identifying and correcting any flaws in your conversion logic.
There are many online resources available that offer a wealth of information on Dart programming, including detailed explanations and examples of dart double to int conversions and other related topics.
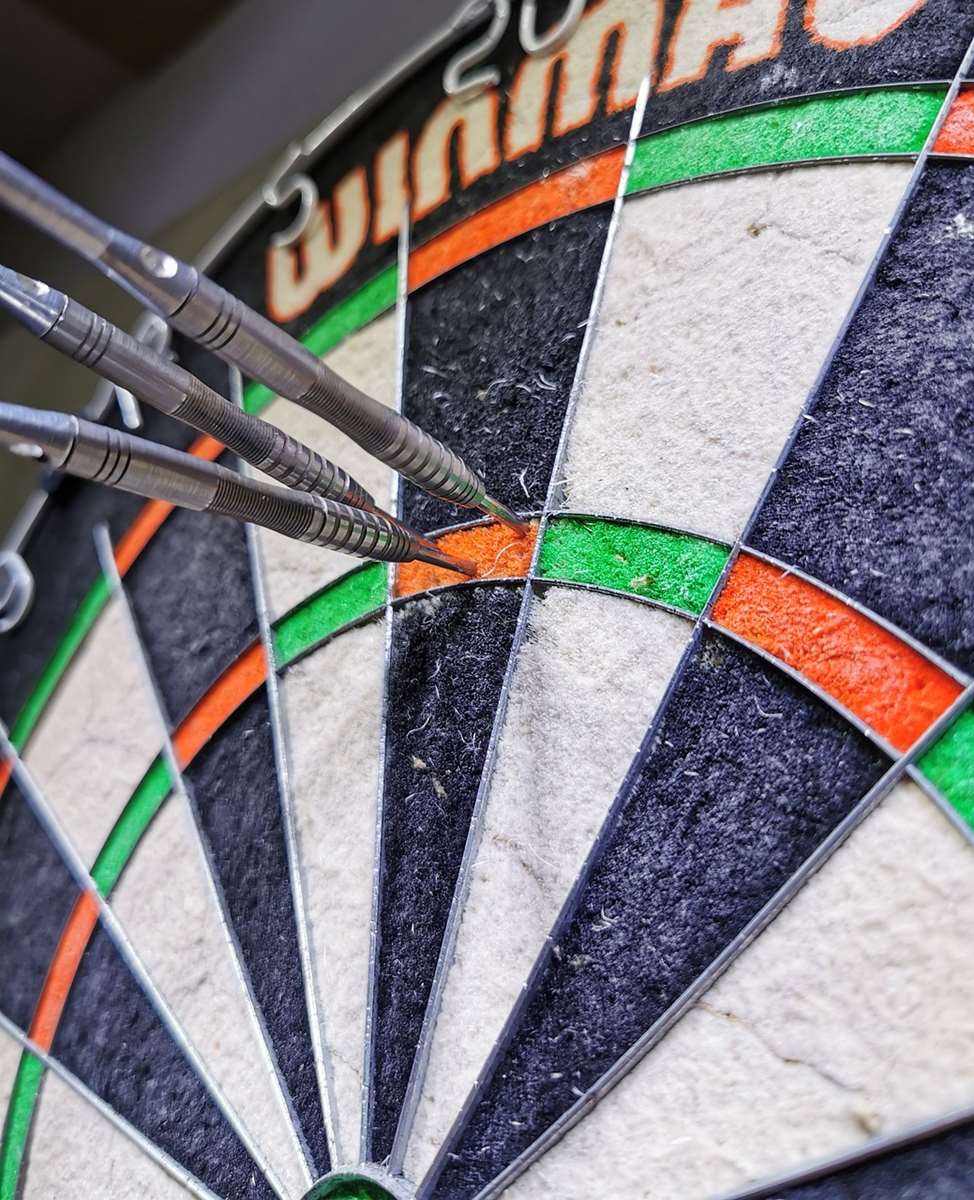
Using Dart Double to Int in Real-World Applications
The ability to convert a dart double to int finds application across a broad spectrum of software development tasks. In game development, for example, you might use it to represent the number of points scored, or the number of lives remaining. The darts game no download could use this type of conversion for example.
In data analysis applications, the ability to convert doubles to integers is often necessary when working with datasets that need to be processed and stored efficiently. In scenarios where precision is less critical, converting to an integer type can lead to noticeable performance gains, especially when working with large datasets. The same principle applies to other fields, including financial modeling, where careful rounding and data management are necessary.
The process of converting a dart double to int is fundamental in countless applications, and mastering this process is essential for Dart developers across numerous domains. Understanding the nuances of different rounding techniques is crucial for ensuring data integrity and achieving optimal performance in your applications. Moreover, remember the importance of thorough testing to catch potential problems before they impact your users. This detailed examination helps in developing robust and reliable applications.
When working with any target darts related application, you will find yourself using dart double to int frequently.
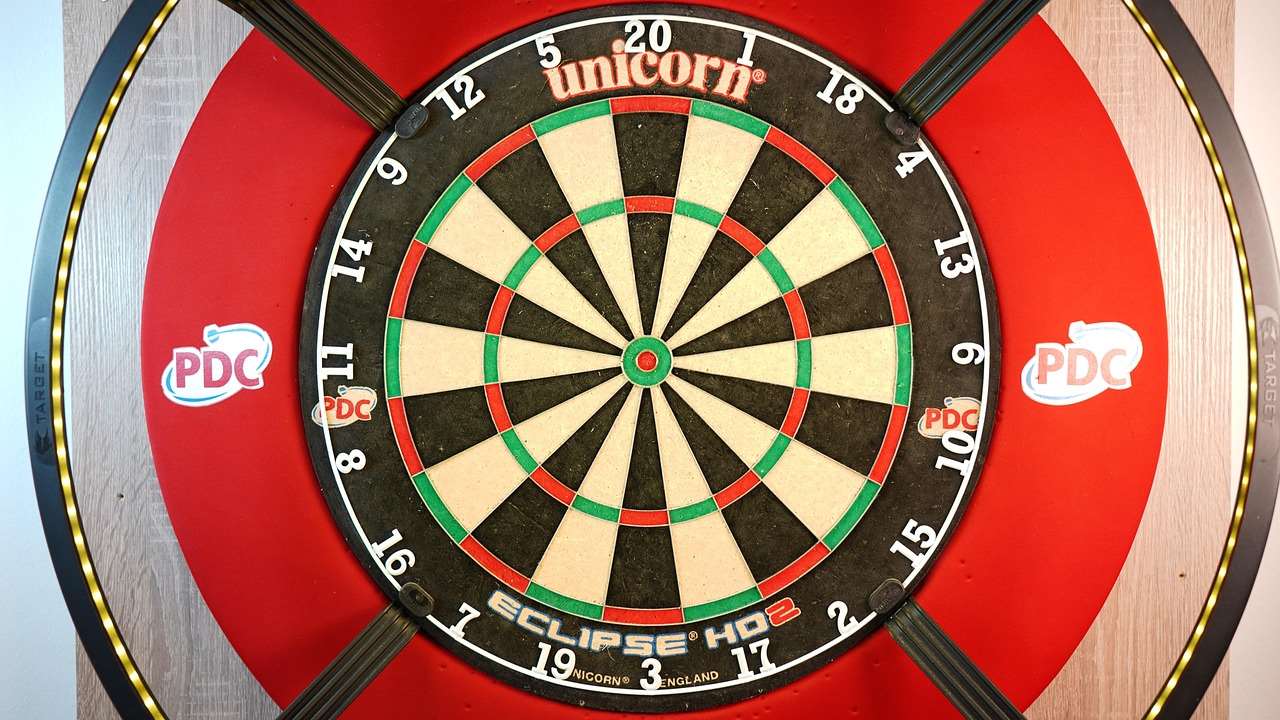
Conclusion
Converting a dart double to int in Dart is a fundamental task that requires careful consideration of the available methods and their implications. While the toInt()
method provides a straightforward approach for truncation, the round()
, floor()
, and ceil()
methods offer more nuanced control over rounding. Understanding the potential for data loss and implementing appropriate error handling are crucial for creating reliable and accurate applications. By carefully selecting the appropriate method and following best practices, you can ensure your dart double to int conversions are both efficient and reliable. Remember to consult the official Dart documentation for the most up-to-date information and best practices. Happy coding!
To learn more about Dart and its features, explore the official Dart documentation and online resources. You might also be interested in learning more about dart x flutter development.
For accurate and reliable electronic scoring in your darts games, consider using an Electronic dart score counter.
Hi, I’m Dieter, and I created Dartcounter (Dartcounterapp.com). My motivation wasn’t being a darts expert – quite the opposite! When I first started playing, I loved the game but found keeping accurate scores and tracking stats difficult and distracting.
I figured I couldn’t be the only one struggling with this. So, I decided to build a solution: an easy-to-use application that everyone, no matter their experience level, could use to manage scoring effortlessly.
My goal for Dartcounter was simple: let the app handle the numbers – the scoring, the averages, the stats, even checkout suggestions – so players could focus purely on their throw and enjoying the game. It began as a way to solve my own beginner’s problem, and I’m thrilled it has grown into a helpful tool for the wider darts community.