Understanding dart double ceil in Dart programming is crucial for handling floating-point numbers effectively. This means understanding how to round a double up to the nearest integer. This article will explain this concept thoroughly, providing examples and exploring related functionalities.
⚠️ Still Using Pen & Paper (or a Chalkboard)?! ⚠️
Step into the future! The Dart Counter App handles all the scoring, suggests checkouts, and tracks your stats automatically. It's easier than you think!
Try the Smart Dart Counter App FREE!Ready for an upgrade? Click above!
The simplest and most efficient way to achieve this is using the `ceil()` method. However, dart double ceil is often paired with other methods and concepts, which we’ll delve into later.
Let’s first establish a clear understanding of what dart double ceil actually does. This functionality is essential in various scenarios, from game development and financial calculations to data analysis. Mastering it will significantly improve the robustness of your Dart applications.
Understanding Dart Double Ceil
The core function of dart double ceil is to round a double-precision floating-point number up to the nearest integer. This means that if the fractional part of the number is greater than zero, the number will be rounded up. If the fractional part is zero, the number remains unchanged (as it’s already an integer). For example, `ceil(3.14)` returns `4`, while `ceil(5.0)` returns `5`.
This seemingly simple operation finds applications in various aspects of programming. Consider a scenario where you’re calculating the number of buses needed to transport a group of people. If each bus can carry 50 people and you have 253 people, simply dividing (253/50) and using dart double ceil will give you the required number of buses, accurately rounding up to avoid any passenger being left behind.
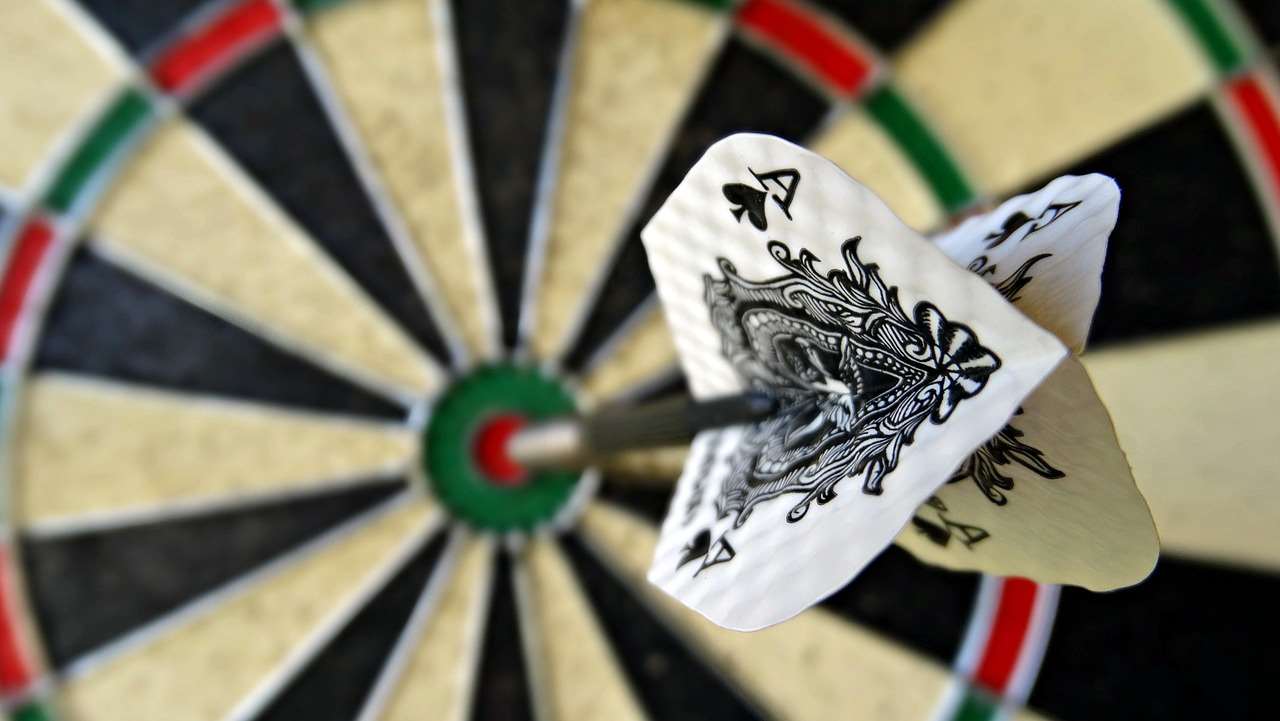
In contrast to `ceil()`, the `floor()` method rounds down to the nearest integer. Understanding the difference between `ceil()` and `floor()` is crucial for selecting the appropriate rounding method for your specific requirements. Choosing the wrong method can lead to incorrect results, especially in applications requiring precise calculations. Remember, using the correct rounding function directly impacts the accuracy of your calculations and the overall reliability of your Dart programs.
Practical Application of Dart Double Ceil
Let’s explore some practical examples to solidify your understanding of dart double ceil. We’ll examine scenarios where using `ceil()` leads to more accurate and efficient results compared to simply truncating the decimal portion of a number.
- Pricing Calculations: Imagine you’re building an e-commerce application. When calculating the total cost including sales tax, using `ceil()` ensures you’re charging the correct amount. If the calculated total is $12.345, rounding up to $12.35 avoids undercharging and ensures correct transaction processing.
- Resource Allocation: When allocating resources like memory or CPU time, rounding up using dart double ceil is often necessary to prevent errors. For example, if a process requires 2.7 megabytes of memory, you must allocate at least 3 megabytes to avoid resource conflicts.
- Game Development: In game development, using dart double ceil can aid in tasks such as determining the number of tiles needed for a game map or calculating the number of units required for a particular scenario.
Moreover, consider using this function for calculations where inaccuracies may cause problems down the line. For instance, when dealing with financial transactions or data analysis involving large datasets, the accuracy of your calculations will depend heavily on correct rounding strategies. Neglecting details as small as dart double ceil can lead to significant errors.
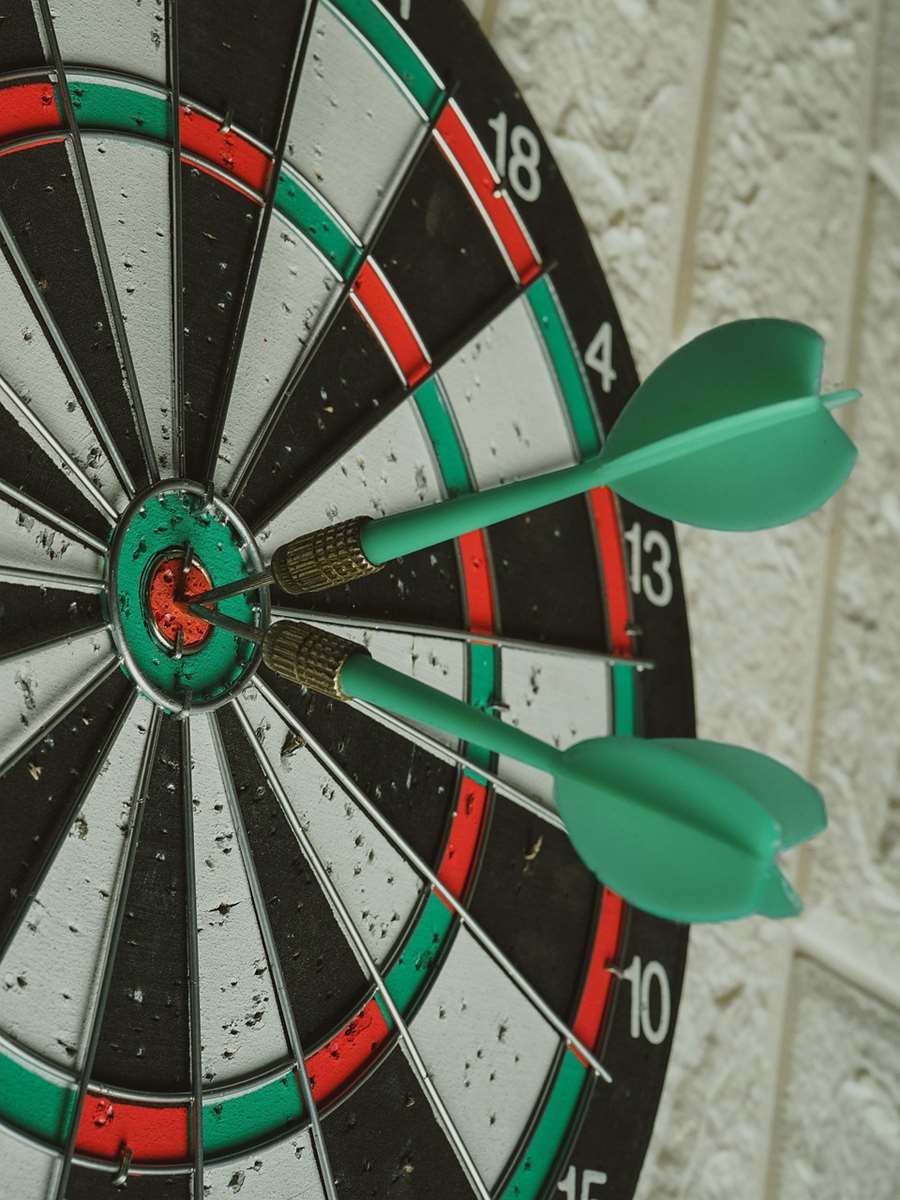
Beyond Dart Double Ceil: Related Functions
While dart double ceil is incredibly useful, the Dart language provides additional functions that are often used in conjunction with it. These include `floor()`, `round()`, and `truncate()`. Understanding these functions helps you choose the most appropriate method for various scenarios.
Floor(): Rounding Down
The `floor()` method is the opposite of `ceil()`; it rounds a double down to the nearest integer. If the fractional part is greater than zero, the number is rounded down. If the fractional part is zero, the number remains unchanged. For example, `floor(3.14)` returns `3`, while `floor(5.0)` returns `5`.
Using `floor()` is useful when you want to obtain the largest integer that’s less than or equal to the given double. For example, if you’re calculating the number of full boxes needed given a certain number of items and items per box, `floor()` will give you the most accurate figure.
Round(): Rounding to the Nearest Integer
The `round()` method rounds a double to the nearest integer. If the fractional part is 0.5 or greater, the number is rounded up; otherwise, it’s rounded down. For example, `round(3.14)` returns `3`, and `round(3.5)` returns `4`. This method is commonly used for general rounding purposes, particularly when you need a value close to the original double without any bias towards rounding up or down.
In contrast to dart double ceil, `round()` provides a more balanced approach to rounding, making it suitable for scenarios where an even distribution of rounding up and down is important. Consider `round()` for scenarios like calculating averages or reporting data where even distribution of rounding is important.
Truncate(): Removing the Fractional Part
The `truncate()` method removes the fractional part of a double, leaving only the integer part. It does not round the number up or down. For example, `truncate(3.14)` returns `3`, and `truncate(5.99)` also returns `5`.
This method is different from rounding and is useful when you need only the whole number portion of a double, regardless of the fractional part. This differs from dart double ceil which focuses specifically on rounding up.
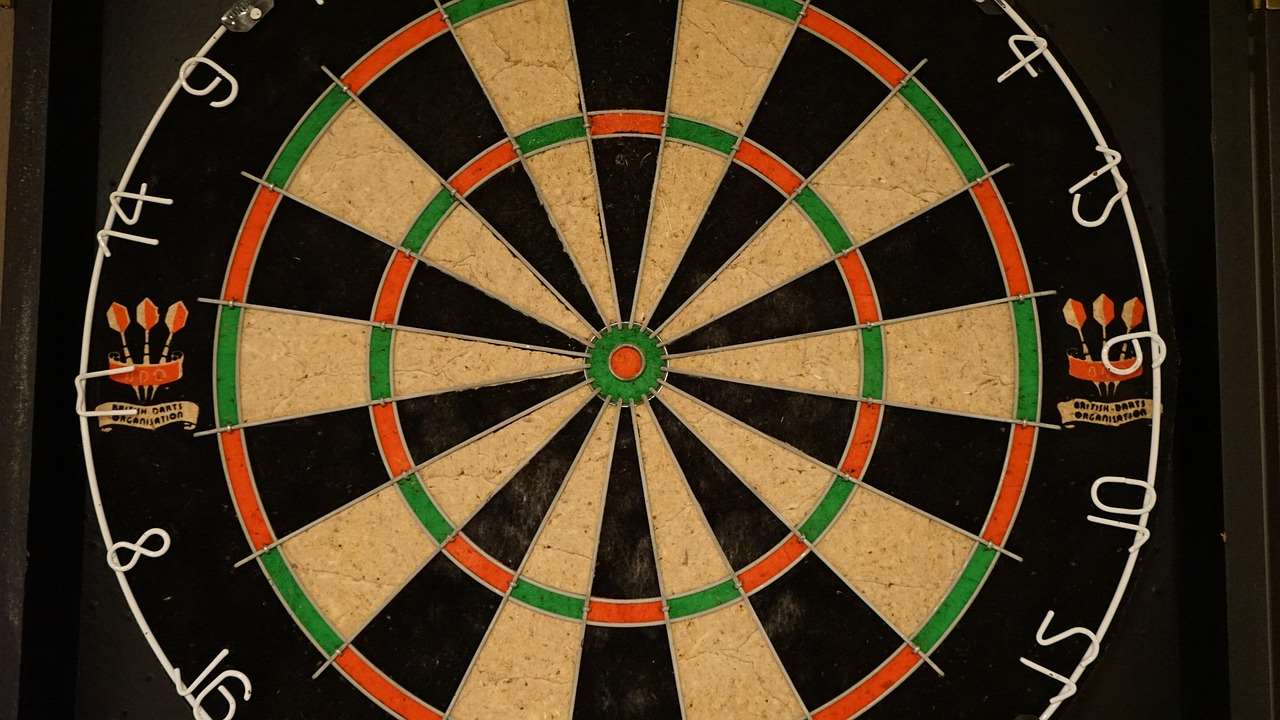
Error Handling and Best Practices
While using dart double ceil and other rounding functions is generally straightforward, it’s crucial to consider potential errors and adopt best practices to ensure the accuracy and reliability of your code. Always carefully consider the context in which you are applying these methods.
One common issue is dealing with extremely large or small numbers. In some cases, rounding might introduce significant inaccuracies when dealing with numbers outside the normal range of representation. Be mindful of potential overflow errors, and consider the limitations of your data types and the precision offered by Dart’s floating-point representation.
Additionally, when working with financial applications or other situations that demand extreme precision, consider using specialized libraries or data types specifically designed for high-precision calculations. While dart double ceil is useful in most cases, alternative methods may be necessary for extremely demanding applications.
Always test your code thoroughly and validate your results, especially when using rounding methods in critical parts of your application. Consider edge cases and ensure that the results are consistent and accurate under different conditions. This approach mitigates the risk of errors caused by incorrect rounding and ensures the reliability of your software.
Furthermore, document your code clearly, including explanations of the rounding methods used and the rationale behind those choices. This will significantly improve the readability and maintainability of your code, and make it easier for others (or your future self!) to understand your reasoning and identify potential issues. Well-documented code is crucial for preventing future problems and simplifying maintenance tasks.
Remember to use a Dart game scoring app for any practice you might need.
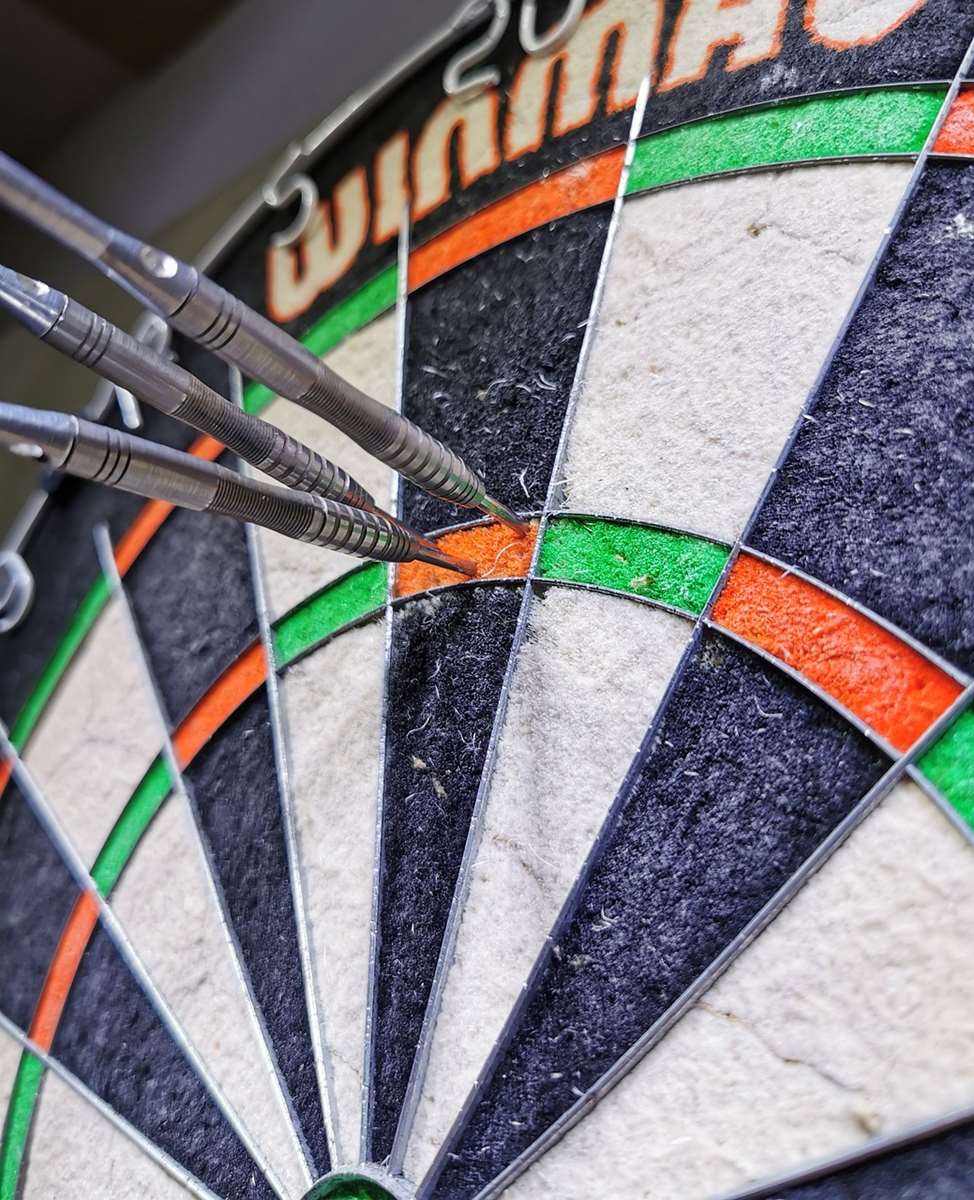
Advanced Usage and Optimization
While simple direct use of dart double ceil is effective in most scenarios, understanding advanced usage and optimization techniques will refine your Dart applications and enhance efficiency. You can combine `ceil()` with other mathematical functions and conditional statements to create more complex logic tailored to your specific needs.
For instance, combining `ceil()` with conditional checks allows for nuanced control over the rounding behavior. You might choose to round up only under specific circumstances, or round down in others, based on additional conditions within your application’s logic. Remember that the flexibility of Dart allows for creating extremely tailored and precise calculations.
For performance-critical applications, using bitwise operations or other low-level techniques for integer manipulation can help optimize rounding calculations. While these techniques can lead to performance gains, it’s crucial to weigh the complexity against the potential benefits. Often the simpler approach with dart double ceil is perfectly sufficient.
Consider carefully whether the added complexity and potential for error introduction outweighs the performance gains. Always prioritize code clarity and readability, unless performance is a truly critical constraint.
Remember to optimize for readability too; while performance matters, easily understood and maintainable code is equally important, especially in team development environments.
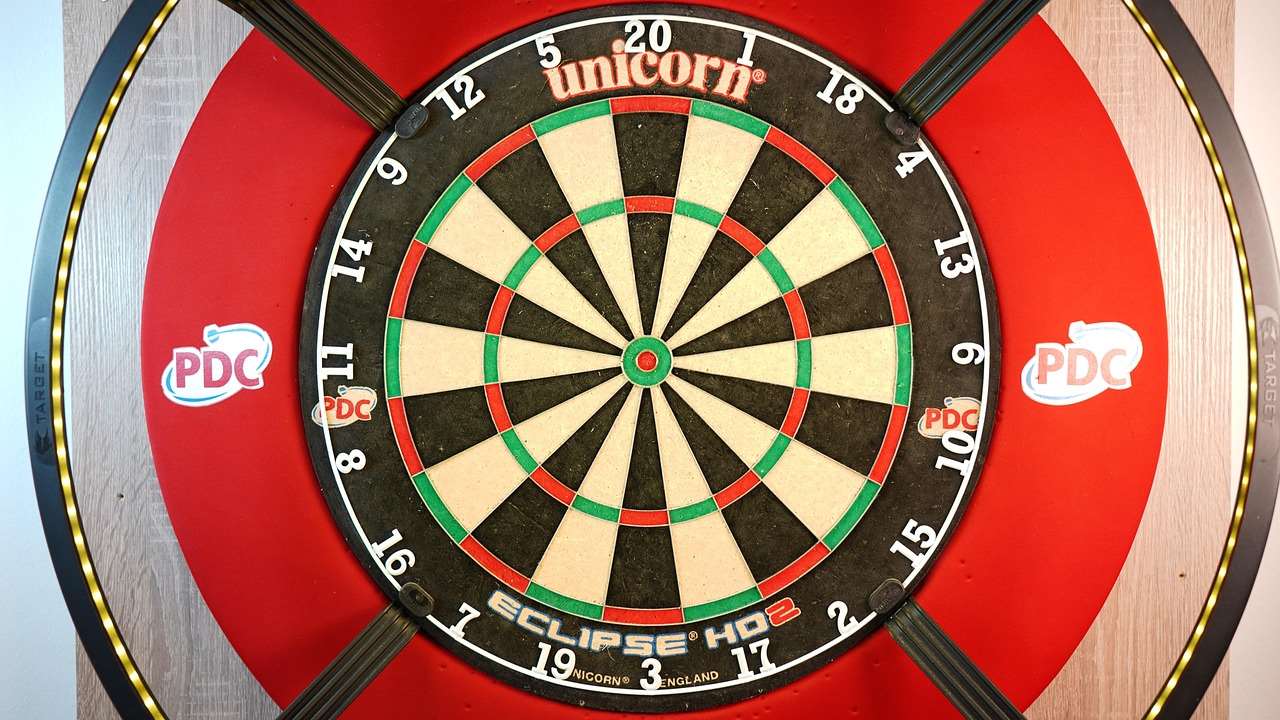
Conclusion
Mastering dart double ceil is a fundamental skill for any Dart developer. Understanding its function, applications, and how it interacts with other related methods like `floor()`, `round()`, and `truncate()` is essential for writing robust, accurate, and efficient code. Remember to consider error handling, best practices, and optimization techniques to create high-quality applications. By understanding these concepts, you can confidently tackle various programming challenges and produce reliable and accurate results in your Dart projects. Start implementing these techniques today and experience the difference in your code quality! Don’t forget to explore further into related Dart functionalities for an even deeper understanding of numerical operations. Perhaps check out our article on darts count down number for an exciting new perspective.
Hi, I’m Dieter, and I created Dartcounter (Dartcounterapp.com). My motivation wasn’t being a darts expert – quite the opposite! When I first started playing, I loved the game but found keeping accurate scores and tracking stats difficult and distracting.
I figured I couldn’t be the only one struggling with this. So, I decided to build a solution: an easy-to-use application that everyone, no matter their experience level, could use to manage scoring effortlessly.
My goal for Dartcounter was simple: let the app handle the numbers – the scoring, the averages, the stats, even checkout suggestions – so players could focus purely on their throw and enjoying the game. It began as a way to solve my own beginner’s problem, and I’m thrilled it has grown into a helpful tool for the wider darts community.