Understanding dart uri is crucial for any Dart developer working with network requests or file system operations. This article will provide a comprehensive guide to working with dart uri, explaining its core functionality and demonstrating its practical applications. You’ll learn how to parse, construct, and manipulate URIs effectively.
⚠️ Still Using Pen & Paper (or a Chalkboard)?! ⚠️
Step into the future! The Dart Counter App handles all the scoring, suggests checkouts, and tracks your stats automatically. It's easier than you think!
Try the Smart Dart Counter App FREE!Ready for an upgrade? Click above!
The Uri
class in Dart provides a powerful way to handle Uniform Resource Identifiers (URIs), which are essentially strings that identify a resource on the internet or within a file system. Whether you’re fetching data from a remote server or accessing local files, understanding how to properly use dart uri is essential for building robust and reliable applications. This understanding extends to parsing existing URIs, constructing new ones, and manipulating their various components, such as the scheme, host, path, and query parameters. Proper dart uri handling is crucial for secure and efficient data access and manipulation in Dart applications.
Understanding the Dart Uri Class
At its core, the dart uri class is a representation of a Uniform Resource Identifier (URI). It allows you to work with URIs in a structured and manageable way. Instead of manipulating raw strings, you can use the Uri
class’s methods to extract specific components, modify parts of the URI, or construct new ones from scratch. This approach ensures better code readability and reduces the risk of errors associated with manual string manipulation. One important thing to remember is that the dart uri class handles both URLs (Uniform Resource Locators) and URNs (Uniform Resource Names), both of which fall under the umbrella term of URI.
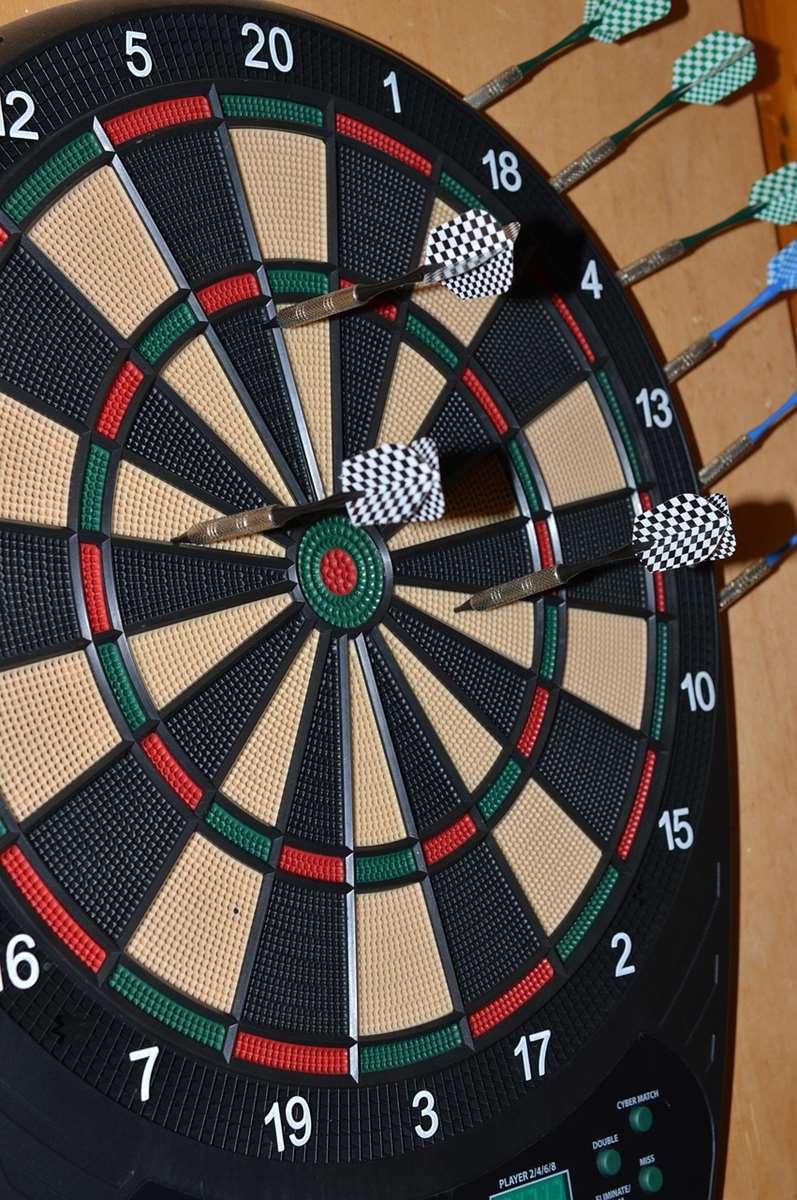
The Uri
class provides methods for accessing different parts of a URI, such as the scheme (e.g., “http”, “https”, “file”), the host, the path, the query parameters, and the fragment. For example, if you have a URI like https://www.example.com/path?query=value#fragment
, you can easily access the host (“www.example.com”), the path (“/path”), the query parameter (“query=value”), and the fragment (“fragment”) using the Uri
class’s methods. This makes it significantly easier to parse and interpret information from various sources, such as web servers or local file systems. This capability is particularly useful in client-server interactions and file system management within your Dart applications.
Key Properties of the Dart Uri Object
scheme
: The protocol of the URI (e.g., “http”, “https”, “ftp”).authority
: The authority part of the URI, including the host and port (e.g., “www.example.com:8080”).host
: The host part of the URI (e.g., “www.example.com”).port
: The port number (if specified) of the URI.path
: The path part of the URI (e.g., “/path/to/resource”).query
: The query parameters of the URI (e.g., “param1=value1¶m2=value2”).fragment
: The fragment identifier (e.g., “#section1”).
Constructing Dart URIs
You can construct dart uri objects using the Uri.parse()
method, which takes a URI string as input and returns a Uri
object. You can also build URIs from individual components using the Uri
constructor. This is particularly useful when dynamically generating URIs, as it allows for more precise control over the final URI string. By using the constructor, you avoid the potential pitfalls of string concatenation and ensure the validity of your URI.
Here’s an example of how to construct a dart uri using both methods:
// Using Uri.parse()
var uri1 = Uri.parse('https://www.example.com/path?query=value');
// Using the Uri constructor
var uri2 = Uri(
scheme: 'https',
host: 'www.example.com',
path: '/path',
queryParameters: {'query': 'value'}
);
print(uri1); // Output: https://www.example.com/path?query=value
print(uri2); // Output: https://www.example.com/path?query=value
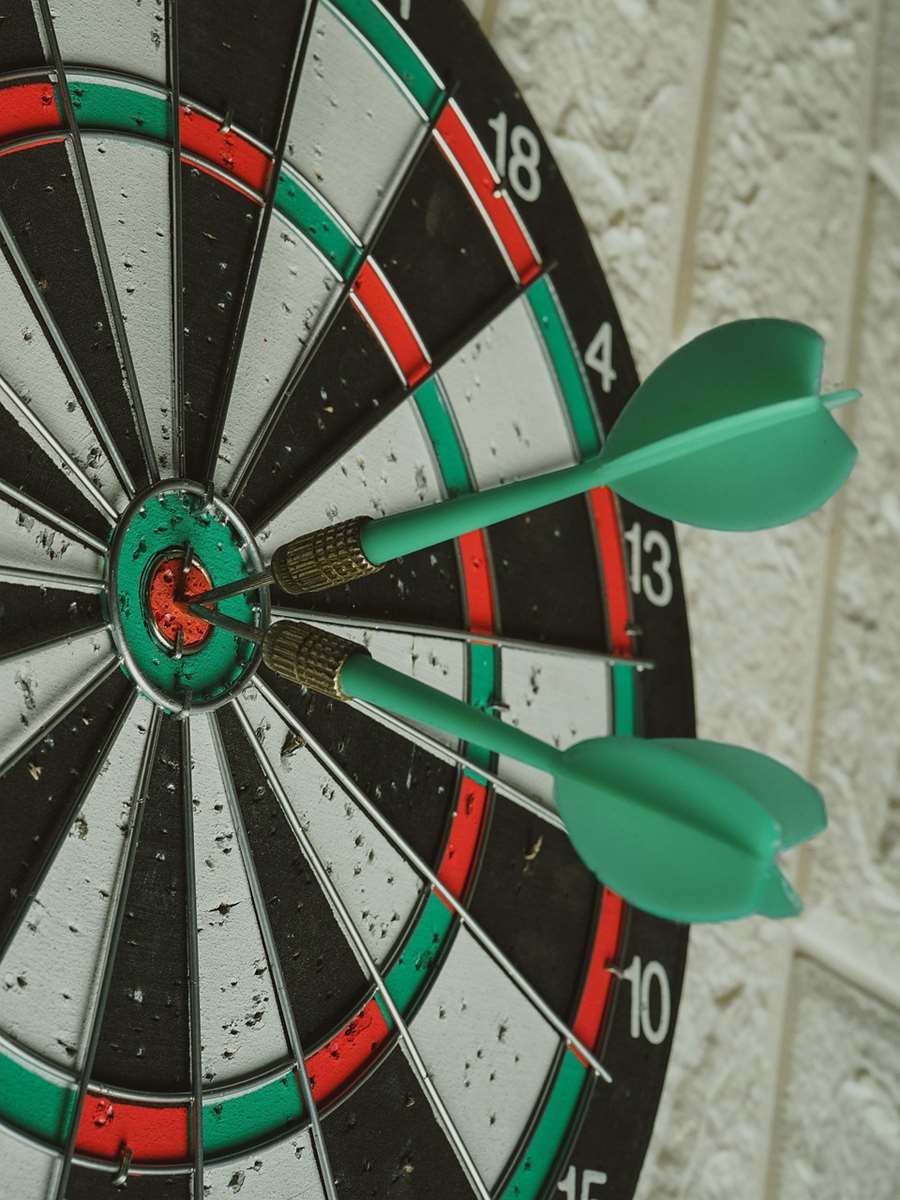
Manipulating Dart URIs
Once you have a Uri
object, you can easily manipulate its components. You can replace parts of the URI, add query parameters, or remove them entirely. The Uri
class offers methods for this, ensuring that changes are made in a consistent and reliable way. This is important for maintaining the integrity of the URI and preventing errors.
For example, to replace the path of a dart uri, you can use the replace
method:
var uri = Uri.parse('https://www.example.com/old/path');
var newUri = uri.replace(path: '/new/path');
print(newUri); // Output: https://www.example.com/new/path
To add or modify query parameters, you can use the queryParameters
property:
var uri = Uri.parse('https://www.example.com/path');
var newUri = uri.replace(queryParameters: {'param1': 'value1', 'param2': 'value2'});
print(newUri); // Output: https://www.example.com/path?param1=value1¶m2=value2
Handling Errors with Dart URIs
When working with dart uri, it’s crucial to handle potential errors gracefully. Invalid URI strings can lead to exceptions. The Uri.tryParse()
method is a safer alternative to Uri.parse()
, as it returns null
instead of throwing an exception if the input string is not a valid URI. This allows you to handle invalid URIs without causing your application to crash. Always validate user inputs and handle potential errors to create a robust application.
var uriString = 'invalid-uri';
var uri = Uri.tryParse(uriString);
if (uri != null) {
// Process the valid URI
print(uri);
} else {
// Handle the invalid URI
print('Invalid URI: $uriString');
}
Practical Applications of Dart URIs
The dart uri class finds extensive use in various Dart applications, particularly those dealing with network requests and file system access. In web applications, it’s integral to making HTTP requests to servers, constructing URLs for API calls, and processing responses. It’s equally vital in desktop or mobile apps using file I/O, helping construct file paths and manage file access.
Let’s look at some specific examples:
- HTTP Requests: When making HTTP requests using packages like
http
, you’ll use dart uri to specify the target URL. Properly formatted URIs are critical for successful communication with the server. - File System Access: In applications needing to read or write files, dart uri helps in constructing file paths correctly. Using the file system efficiently demands correct path structuring.
- API Interactions: Building APIs in Dart and interacting with external APIs heavily relies on correctly constructing and parsing URIs to ensure seamless data exchange.
- URL Shortening Services: Building a URL shortening service will involve extensive usage of the dart uri class for parsing and generating shortened URLs.
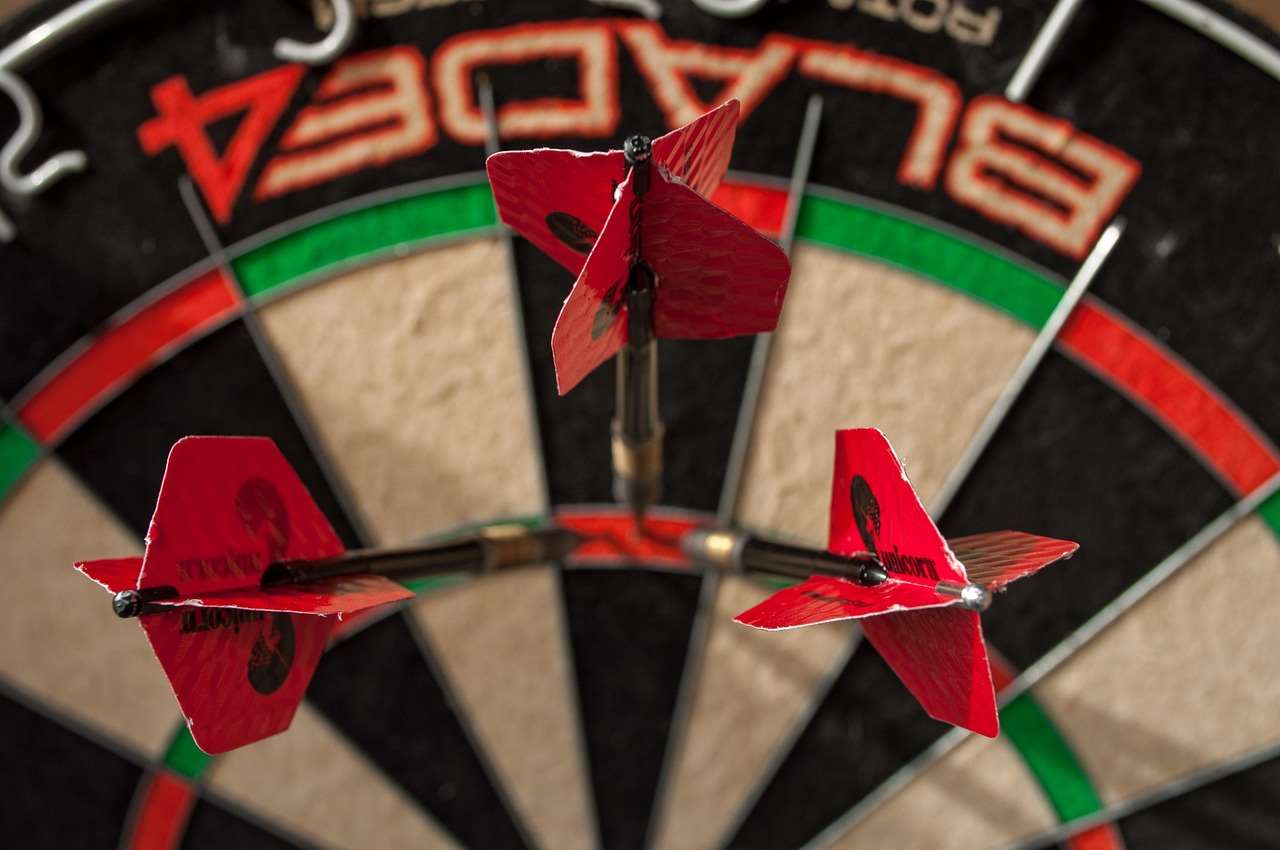
Advanced Dart URI Techniques
Beyond the basics, several advanced techniques can enhance your use of dart uri. These include:
- Query Parameter Encoding/Decoding: Understanding how to properly encode and decode query parameters is important for handling special characters and ensuring data integrity during URI construction and parsing.
- Path Manipulation: Learning more advanced path manipulation techniques, such as joining paths, normalizing paths, and resolving relative paths, allows for more robust file system operations.
- URI Component Validation: Implementing comprehensive validation of each URI component helps prevent errors and vulnerabilities.
- Custom URI Schemes: While less common, for specialized applications, Dart allows defining custom URI schemes.
Mastering these advanced techniques enables efficient and secure handling of URIs within your applications. This results in well-structured, robust code. Consider using Darts scorekeeper app for your darts scorekeeping needs.
Troubleshooting Common Dart URI Issues
While the Uri
class is designed to simplify URI handling, some problems might arise. Common issues include:
- Incorrectly formatted URI strings: Always use
Uri.tryParse()
to validate URIs before processing them. This prevents exceptions and improves robustness. - Encoding issues with query parameters: Ensure proper URL encoding of query parameters to handle special characters like spaces and symbols correctly. Failure to encode these characters can lead to errors.
- Path resolution problems: When dealing with relative paths, understand how path resolution works to avoid unexpected behavior. Be explicit about whether it’s a relative or absolute path.
Careful attention to these details during development will significantly reduce the likelihood of encountering these issues, leading to more stable and reliable code.
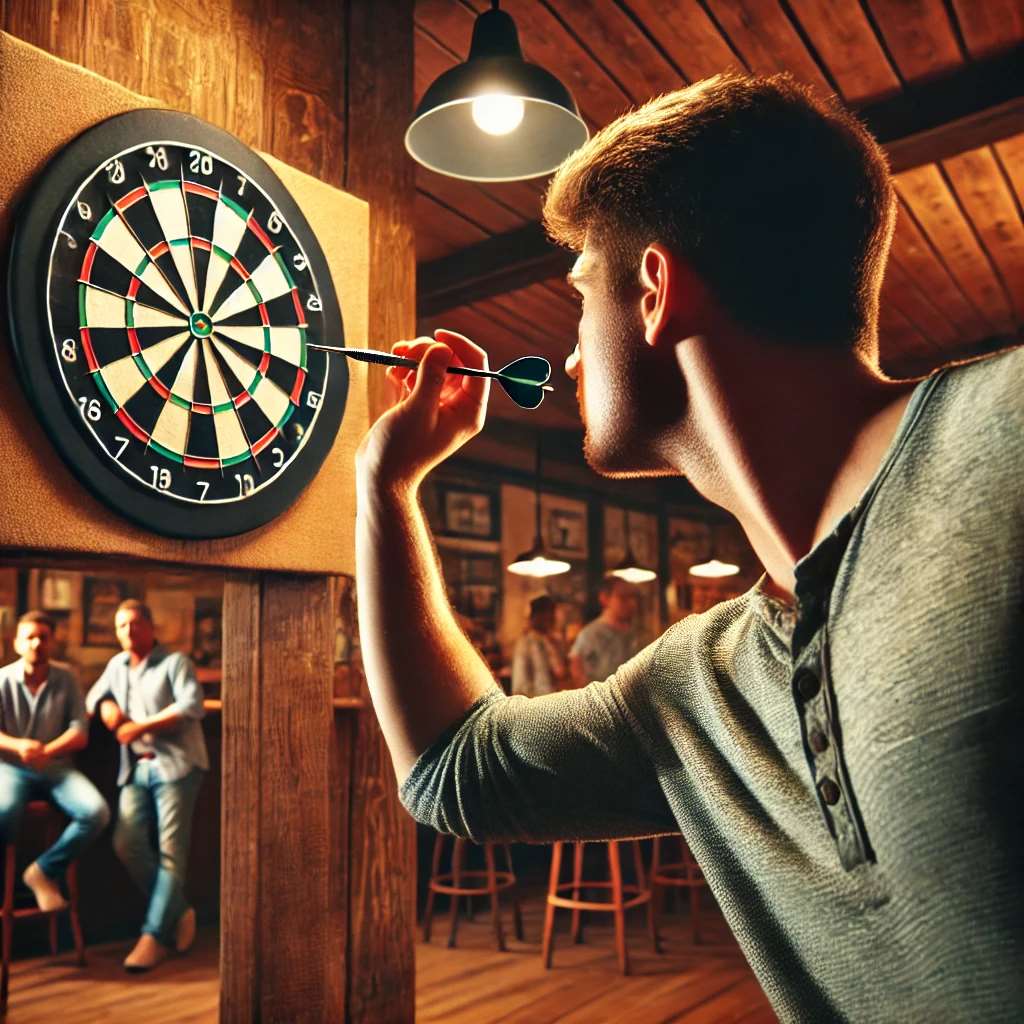
Best Practices for Using Dart URIs
Following best practices will ensure that your code is efficient, robust, and maintainable:
- Always use the
Uri
class: Avoid manual string manipulation of URIs whenever possible. TheUri
class provides a safer and more structured approach. - Validate URI inputs: Always validate any user-provided URI strings using
Uri.tryParse()
to prevent exceptions and handle invalid input gracefully. - Encode query parameters: Always encode query parameters to handle special characters and avoid errors.
- Use named constructors for clarity: When constructing URIs using the
Uri
constructor, use named parameters to improve code readability. - Handle errors effectively: Use
try-catch
blocks to handle potential exceptions, ensuring the application doesn’t crash if it encounters an invalid URI.
Adhering to these best practices will make your code more efficient, robust, and easier to maintain. Remember to use a darts at home pdc setup for a proper home dart experience.
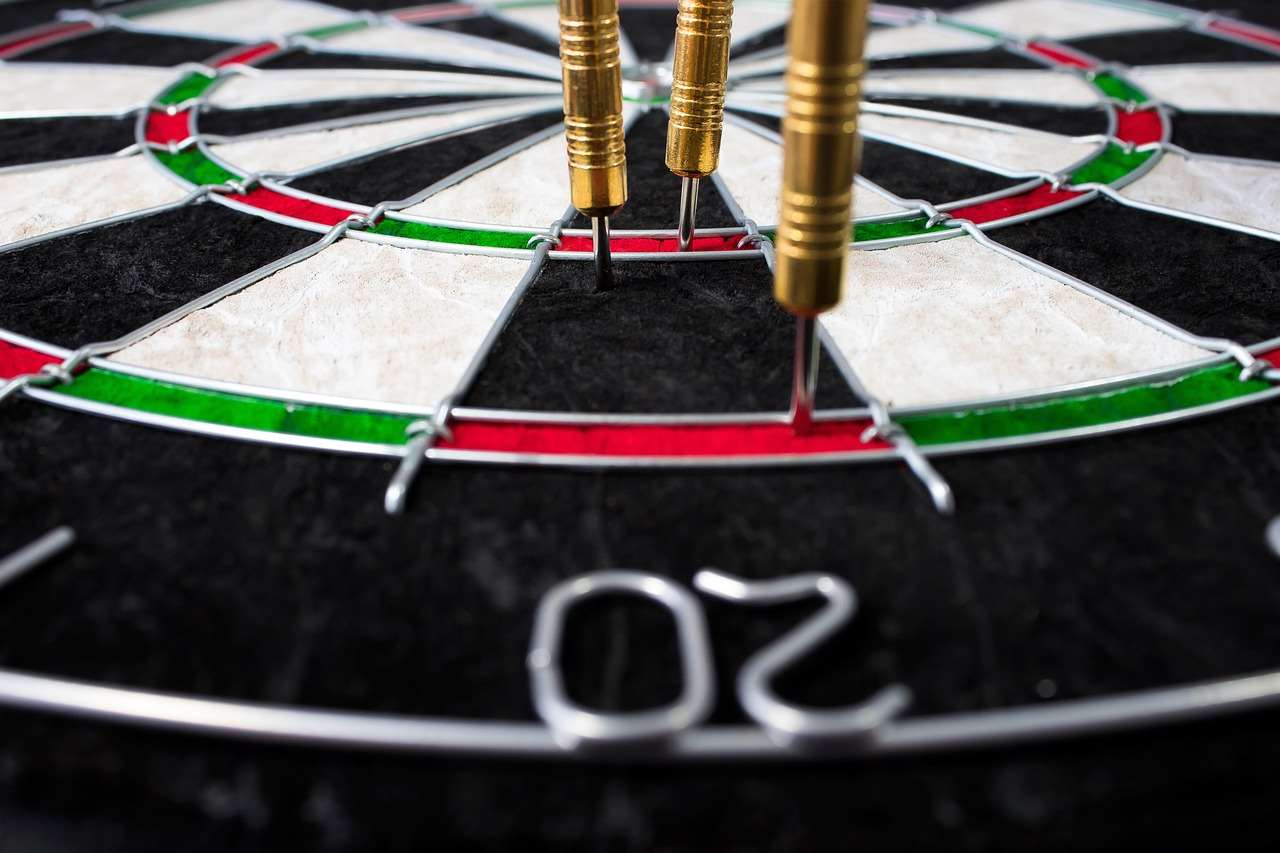
Conclusion
The dart uri class is a fundamental component for any Dart application dealing with network requests or file system interactions. By understanding its properties, methods, and best practices, you can write robust, efficient, and maintainable code. Remember to use Uri.tryParse()
for error handling and employ proper query parameter encoding. This comprehensive guide has provided the necessary tools for handling dart uri effectively in your Dart projects. Now, go forth and build amazing Dart applications!
Start practicing today and enhance your Dart development skills by implementing the best practices outlined in this article. Don’t forget to check out our other resources on dart flights kite harrows and dart finishers for more information about related topics.
For further exploration, consider reviewing the official Dart documentation on the Uri
class and exploring related packages that enhance URI handling. Happy coding!
We hope this article provided valuable insights into Dart URIs, helping you confidently handle them in your projects. Improve your game with information on how wide is darts oche, darts oche sticker, and dartboard outdoor options.
This article also touches on target darts december launch, which is helpful for those interested in specific dart products. Also learn about how to put dart together and dart m.
Don’t forget to explore more advanced techniques, such as custom URI schemes and advanced path manipulations, to further elevate your Dart skills. Happy coding!
Hi, I’m Dieter, and I created Dartcounter (Dartcounterapp.com). My motivation wasn’t being a darts expert – quite the opposite! When I first started playing, I loved the game but found keeping accurate scores and tracking stats difficult and distracting.
I figured I couldn’t be the only one struggling with this. So, I decided to build a solution: an easy-to-use application that everyone, no matter their experience level, could use to manage scoring effortlessly.
My goal for Dartcounter was simple: let the app handle the numbers – the scoring, the averages, the stats, even checkout suggestions – so players could focus purely on their throw and enjoying the game. It began as a way to solve my own beginner’s problem, and I’m thrilled it has grown into a helpful tool for the wider darts community.