Determining the dart list count in Dart is straightforward using the length
property. This article will show you exactly how, along with exploring other useful list manipulation techniques and troubleshooting common issues. You’ll also learn how to efficiently handle lists of varying sizes and data types.
⚠️ Still Using Pen & Paper (Of een schoolbord)?! ⚠️
Stap in de toekomst! De Dart Teller -app behandelt alle scoren, stelt kassa voor, en volgt uw statistieken automatisch. It's easier than you think!
Probeer de Smart Dart Teller -app gratis!Klaar voor een upgrade? Klik hierboven!
Let’s begin by looking at the most basic method for getting the dart list count. De length
property is your best friend here; it directly provides the number of elements in your list. This is incredibly useful for tasks such as iteration, conditional logic based on list size, en meer. Understanding how to efficiently use this property is fundamental to effective Dart programming.
Beyond the basics of determining the dart list count, we’ll also cover advanced techniques, including how to handle nested lists, and the potential pitfalls to avoid when working with lists of dynamic sizes or types. We’ll further examine different approaches to ensure efficient code execution, particularly when dealing with large datasets. This ensures your code remains performant even with increasingly complex lists.
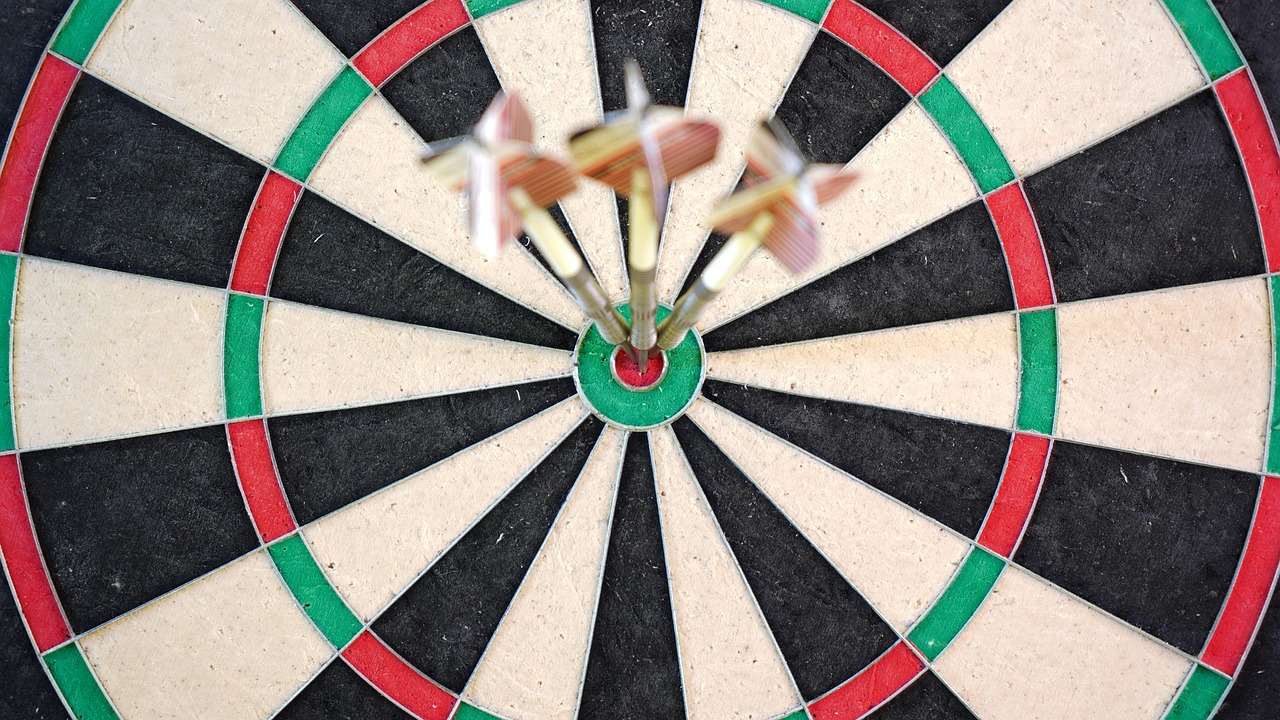
Finding the Dart List Count: The Basics
The simplest way to get your dart list count is using the built-in length
property. This property is directly accessible on any List
object in Dart. Bijvoorbeeld:
List<int> numbers = [1, 2, 3, 4, 5];
int count = numbers.length; // count will be 5
print("The list has $count elements.");
This concise snippet demonstrates the fundamental approach to obtaining the dart list count. De length
property returns an integer representing the number of items contained within the list. This approach works regardless of the data type held within the list (integers, strings, custom objects, etc.). It’s incredibly efficient and directly reflects the current size of the list.
Working with Different List Types
De length
property works consistently across various list types in Dart. Whether you’re working with a List<int>
, A List<String>
, of een List<dynamic>
(which can hold various data types), de length
property will always correctly return the number of elements. This consistent behavior makes it a reliable tool for any situation where you need to know your dart list count.
Bijvoorbeeld, if you have a list of strings:
List<String> names = ["Alice", "Bob", "Charlie"];
int nameCount = names.length; // nameCount will be 3
print("There are $nameCount names in the list.");
This shows how easily adaptable the length
property is. Its universal applicability across diverse data types is a significant advantage in Dart programming, simplifying list manipulation tasks considerably.
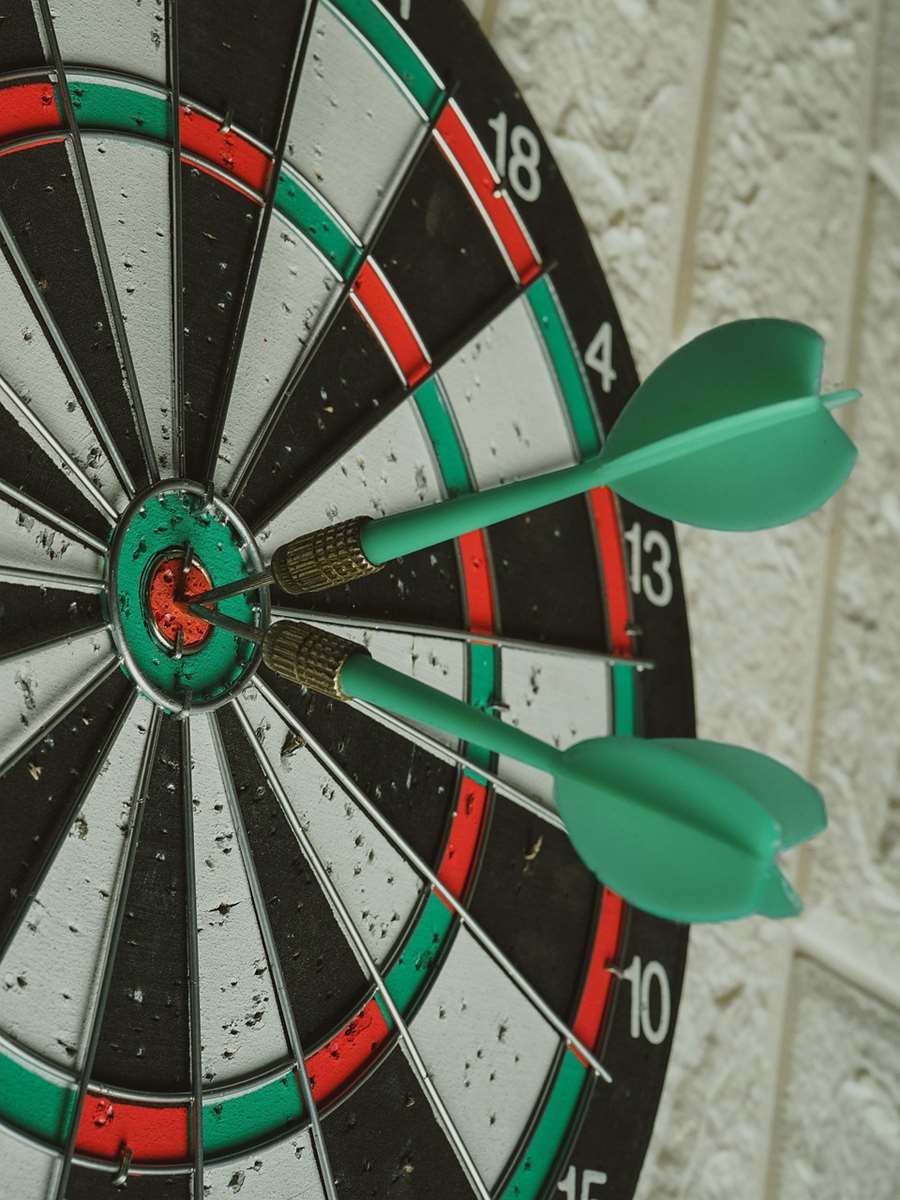
Advanced Techniques for Dart List Count Manipulation
Terwijl de length
property provides a direct dart list count, more complex scenarios might require additional techniques. Let’s explore some of them.
Handling Nested Lists
When dealing with nested lists (lists within lists), determining the total number of elements requires a slightly different approach. You’ll need to iterate through each nested list and sum up their individual lengths. Here’s an example:
List<List<int>> nestedList = [[1, 2], [3, 4, 5], [6]];
int totalcount = 0;
for (List<int> innerList in nestedList) {
totalcount += innerList.length;
}
print("The nested list contains $totalcount elements.");
This approach effectively handles nested structures. It iterates over the outer list, accessing each inner list, and then uses the length
property of each inner list to accumulate the total dart list count. This solution is crucial for handling data structures beyond simple linear lists.
Efficiently Counting Elements in Large Lists
For extremely large lists, consider optimizing your approach to avoid unnecessary overhead. Directly using the length
property remains the most efficient, but if you need to perform operations concurrently with counting, explore Dart’s asynchronous capabilities to prevent blocking the main thread and maintaining application responsiveness. For very large lists or performance-critical applications, consider using specialized data structures or libraries optimized for large datasets.
Herinneren, understanding the size of your list is crucial for optimizing algorithms and avoiding performance bottlenecks. Premature optimization can be detrimental, but knowing when to prioritize efficiency, especially when dealing with substantial data, is a skill every Dart developer should cultivate. Learn more about optimization strategies in our comprehensive guide.
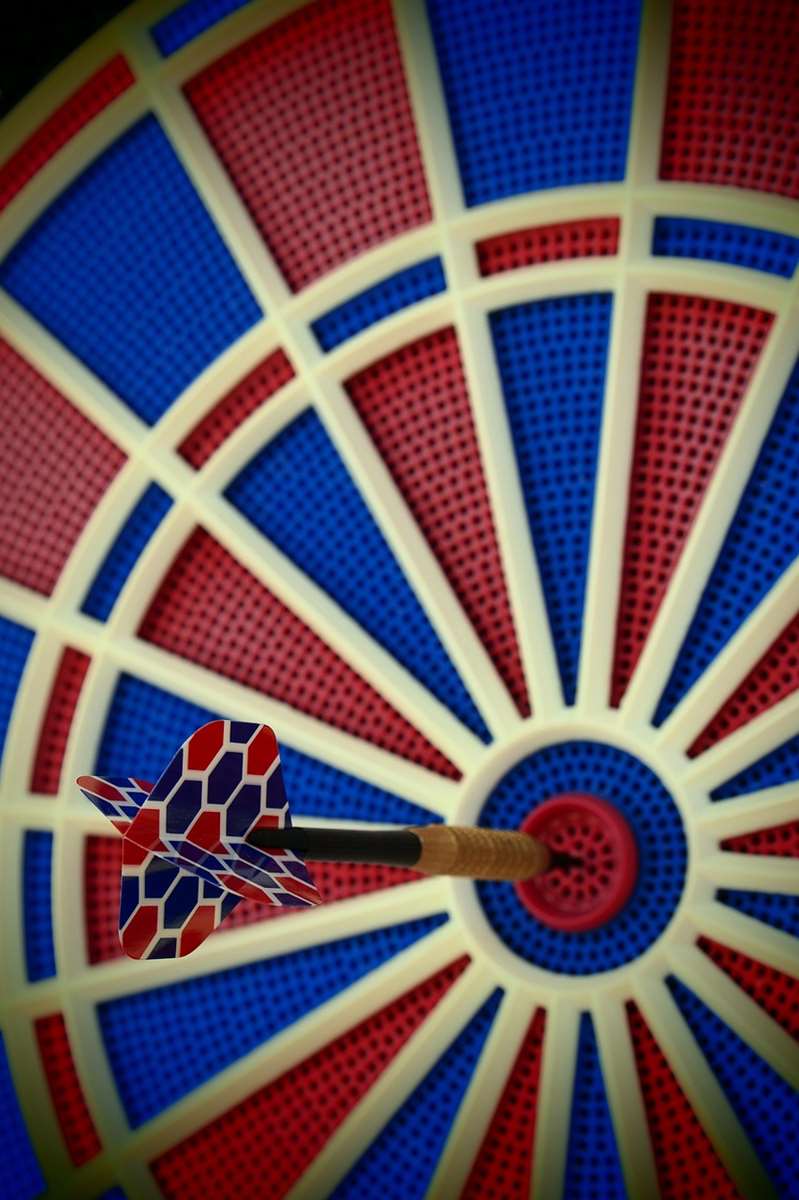
Troubleshooting Common Dart List Count Issues
Occasionally, you might encounter unexpected behavior when working with lists. Let’s address some common issues.
Null or Empty Lists
Attempting to access the length
property of a null
list will throw a NullPointerException
. Always check for null
values before accessing properties:
List<int> maybeNumbers; // This list is null
int count = maybeNumbers != null ? maybeNumbers.length : 0;
print("List length: $count");
This safe approach avoids runtime errors by handling the case of a null
list gracefully. It provides a default value (0) when the list is null, preventing exceptions and ensuring robust code.
Dynamically Changing Lists
If your list is modified frequently (adding or removing elements), de length
property will always accurately reflect the current number of elements. Echter, bear in mind that frequently modifying a list within a loop can be computationally expensive. Consider if alternate data structures would be more appropriate for your specific use case.
Bijvoorbeeld, if you are adding elements based on an external data source, you might need a mechanism to efficiently handle updates while preventing blocking or overly frequent re-counting. Consider using streams and asynchronous programming for more complex scenarios where the dart list count changes dynamically.
Remember that understanding how your list is modified and when the dart list count is critical is essential for writing efficient and reliable Dart code. This requires carefully considering the frequency of updates and potentially using more complex mechanisms for handling dynamic data.

Best Practices for Working with Dart Lists
Effective list management is crucial for efficient and maintainable Dart applications. Here are some best practices to follow.
- Use the correct list type: Choose between
List<T>
(for fixed-type lists) EnList<dynamic>
(for lists holding various types) based on your needs. Using the most specific type enhances type safety and code readability. - Initialize lists correctly: Avoid unexpected behavior by initializing your lists appropriately. Use the
[]
constructor or theList.filled()
constructor to create lists with appropriate initial sizes. - Leverage Dart’s collection methods: Utilize methods like
add()
,remove()
,addAll()
, EnremoveAt()
for efficient list manipulation. - Consider immutability: Indien mogelijk, create immutable lists to prevent unexpected changes, particularly in multi-threaded environments. Immutability ensures that the dart list count will remain constant unless a new list is explicitly created.
These simple best practices significantly impact the efficiency and maintainability of your Dart code and help prevent common list-related issues. They are crucial for developing scalable and robust applications.
By consistently applying these practices, you can ensure your code is cleaner, more efficient, and easier to maintain, especially in larger projects where managing lists efficiently is critical. These simple practices improve not just the dart list count but overall application performance.
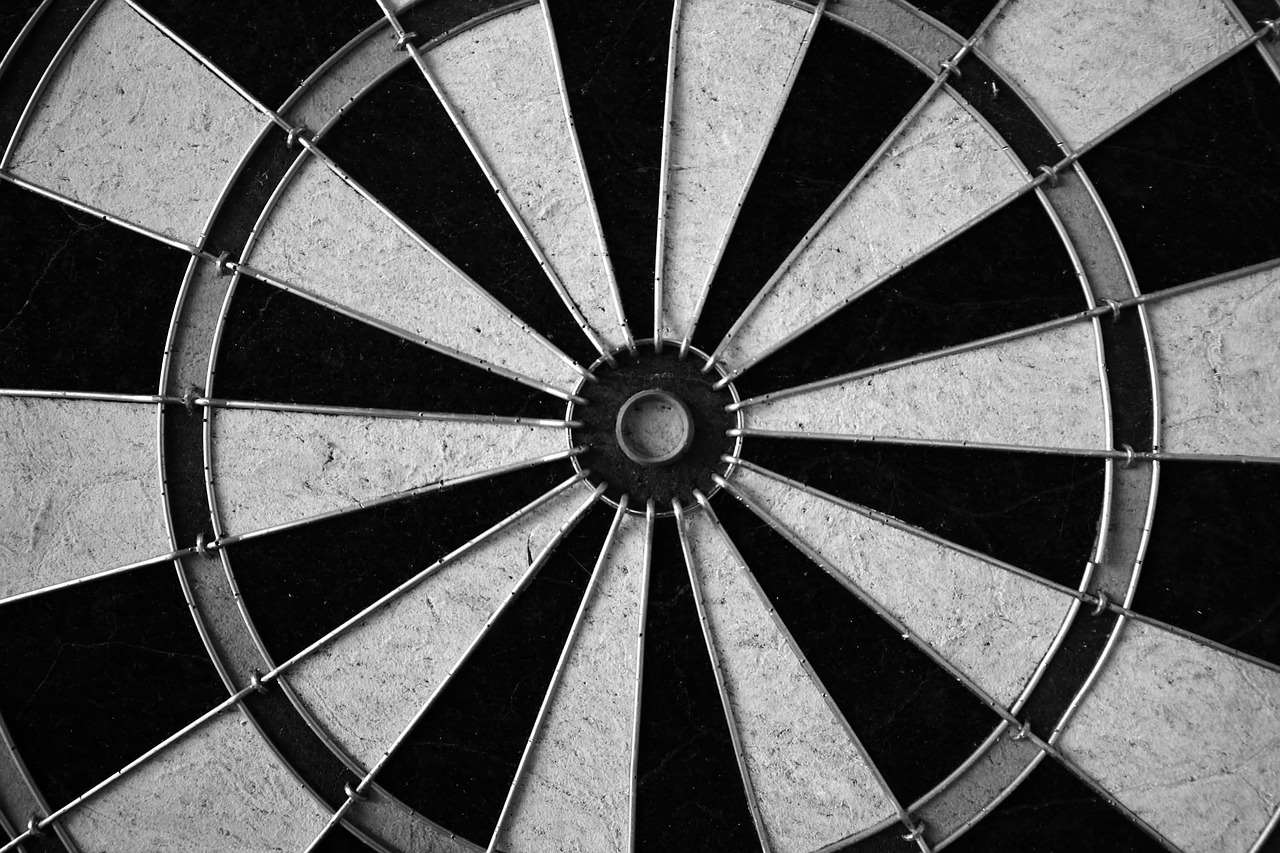
Conclusie
Determining the dart list count is a fundamental task in Dart programming. De length
property provides a straightforward and efficient way to achieve this. This article covered the basics, advanced techniques for handling nested lists and large datasets, and common troubleshooting scenarios. Remember to adopt best practices to ensure your code remains efficient, maintainable, en robuust. Mastering list manipulation is key to building powerful and scalable Dart applications. To further enhance your Dart skills, Practice darts app with scoring is an invaluable resource.
If you’re working with Dart and need to efficiently manage and manipulate lists, Inzicht in de dart list count is essential. This information allows you to write optimized code and avoid common pitfalls associated with working with lists of varying sizes and data types. By incorporating the techniques and best practices discussed in this article, you can build more efficient and reliable Dart applications.
Learn more about optimizing Dart list usage.
Hoi, Ik ben Dieter, En ik heb Dartcounter gemaakt (Dartcounterapp.com). Mijn motivatie was geen darts -expert - helemaal tegenovergestelde! Toen ik voor het eerst begon te spelen, Ik hield van het spel, maar vond het moeilijk en afleidend om nauwkeurige scores te houden en statistieken te volgen.
Ik dacht dat ik niet de enige kon zijn die hiermee worstelde. Dus, Ik besloot om een oplossing te bouwen: een eenvoudig te gebruiken applicatie die iedereen, Ongeacht hun ervaringsniveau, zou kunnen gebruiken om moeiteloos te scoren.
Mijn doel voor Dartcounter was eenvoudig: Laat de app de nummers afhandelen - het scoren, de gemiddelden, de statistieken, Zelfs checkout suggesties - zodat spelers puur kunnen richten op hun worp en genieten van het spel. Het begon als een manier om het probleem van mijn eigen beginners op te lossen, En ik ben heel blij dat het is uitgegroeid tot een nuttig hulpmiddel voor de bredere darts -community.