Determining the size of an iterable is a fundamental task in Dart programming. The most straightforward way to find the dart iterable count is by using the .length
property. This article will not only explain this core method but also delve into alternative approaches, handling edge cases, and optimizing for performance when dealing with large datasets or potentially infinite iterables.
⚠️ Still Using Pen & Paper (Of een schoolbord)?! ⚠️
Stap in de toekomst! De Dart Teller -app behandelt alle scoren, stelt kassa voor, en volgt uw statistieken automatisch. It's easier than you think!
Probeer de Smart Dart Teller -app gratis!Klaar voor een upgrade? Klik hierboven!
Understanding how to efficiently determine the dart iterable count is crucial for many programming tasks. Bijvoorbeeld, you might need to know the number of items in a list before processing them, or you may want to check if a collection is empty. This knowledge is essential for writing robust and efficient Dart applications. This guide will equip you with various techniques and best practices for efficiently counting iterable elements, including strategies for handling potentially very large collections or cases where directly getting the .length
might not be feasible.
Let’s start with the simplest and most common method: using the .length
property, which is particularly efficient for lists, which have a predefined length. For iterables such as sets or maps where length is often computed dynamically, it’s still a quick and dependable option.
The Simplest Approach: Using the .length
Property for Dart Iterable Count
The most efficient way to get the dart iterable count for lists is by using the .length
property. This property directly returns the number of elements in the list. This method is straightforward and computationally inexpensive.
Here’s a simple example:
List
int count = names.length; // count will be 4
print(count);
This code snippet showcases how easily you can obtain the dart iterable count for a list. De .length
property provides a direct and efficient method for determining the size of your list. Remember that this approach is most efficient for lists because they maintain an internal count of their elements.
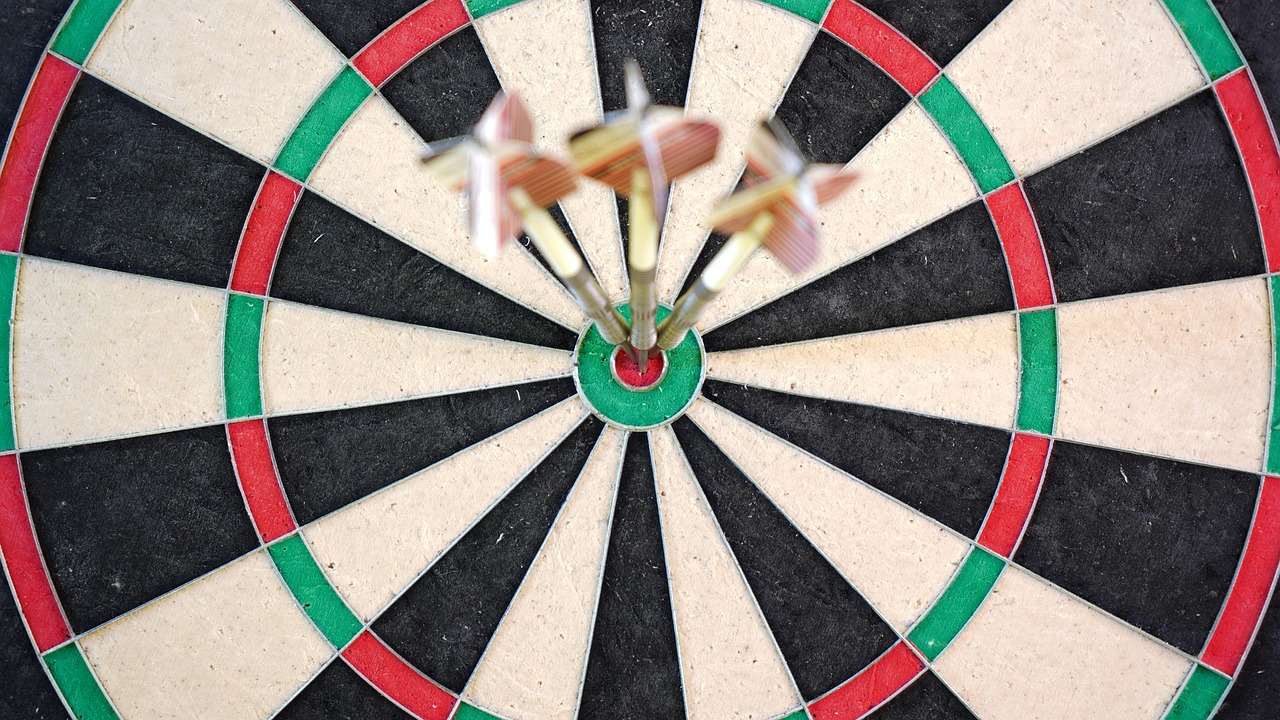
Echter, keep in mind that .length
might not be the ideal solution for all iterable types. For iterables that are generated on demand or are potentially infinite, obtaining the .length
might be computationally expensive, or even impossible. In such cases, we need alternative techniques.
Working with Other Iterables: Beyond Lists in Dart Iterable Count
While lists offer convenient access to the dart iterable count via .length
, other iterables like Sets and Maps require different approaches. Sets, for example, don’t maintain a readily accessible element count like lists do. Therefore, while you can still use .length
, it’s important to understand that the calculation involves an internal operation to determine the size.
Here’s an example illustrating this with a Set:
Set
int count = numbers.length; // count will be 5
print(count);
For Maps, the same principle applies; .length
gives you the number of key-value pairs. For both Sets and Maps, de .length
property remains the most efficient approach, despite internal computations, as it leverages the data structures’ optimized internal mechanisms.
Iterables without a .length
Property
It’s important to note that not all iterables in Dart explicitly have a .length
property. For such cases, you will need to adopt a different strategy to determine the dart iterable count. This often involves iterating through the elements and maintaining a counter.
Consider this example where we use a for
loop to count elements from a stream:
Stream
int count = 0;
numberStream.listen((event) {
count++;
}); // You need to await completion of stream or use async/await
print(count); // this will print 5 eventually
In this scenario, we explicitly use a counter variable and increment it for each item received. This emphasizes the importance of understanding the nature of your iterable before attempting to count its elements. For iterables that produce elements on demand, like Streams, using .length
is not possible; instead, you need to iterate.

Handling Large Datasets and Infinite Iterables for Dart Iterable Count
When dealing with extremely large datasets or infinite iterables, directly utilizing .length
or even a simple for
loop can be computationally expensive or even impossible. Efficiently determining the dart iterable count under these conditions requires more sophisticated techniques. Bijvoorbeeld, you might sample the dataset to estimate the count or use specialized data structures that provide better performance for large data sets.
For extremely large datasets, consider strategies like:
- Sampling: Take a statistically significant sample of the dataset and extrapolate the total count based on the sample’s size and proportion. This is particularly useful when dealing with a dataset whose size is unknown or too large to traverse completely.
- Chunking: Process the data in smaller chunks to minimize memory usage. Each chunk’s size can be counted individually, and the counts aggregated for a total. This approach reduces the demand on your system’s memory.
- Parallel processing: Utilize Dart’s concurrency features to divide the counting task into smaller subtasks, processed concurrently. This can significantly reduce overall processing time. Libraries like `dart:async` can help facilitate this.
Herinneren, the best approach is often determined by the specifics of your data and the constraints of your application. Understanding the size and characteristics of your iterable is paramount in selecting the most efficient method.
Error Handling and Edge Cases in Dart Iterable Count
Terwijl .length
is generally straightforward, it’s important to consider potential edge cases. Bijvoorbeeld, if you’re working with asynchronous iterables or those that might throw exceptions, you’ll need to implement proper error handling. Failing to account for potential issues can lead to unexpected crashes or incorrect results.
Here’s how to handle potential errors when obtaining the dart iterable count:
try {
int count = myIterable.length;
print('Iterable length: $count');
} catch (e) {
print('Error obtaining iterable length: $e');
}
Dit try-catch
block ensures that even if an error arises during the attempt to retrieve the length, your application doesn’t crash unexpectedly. This robust approach protects against potential exceptions, enhancing the reliability of your code.
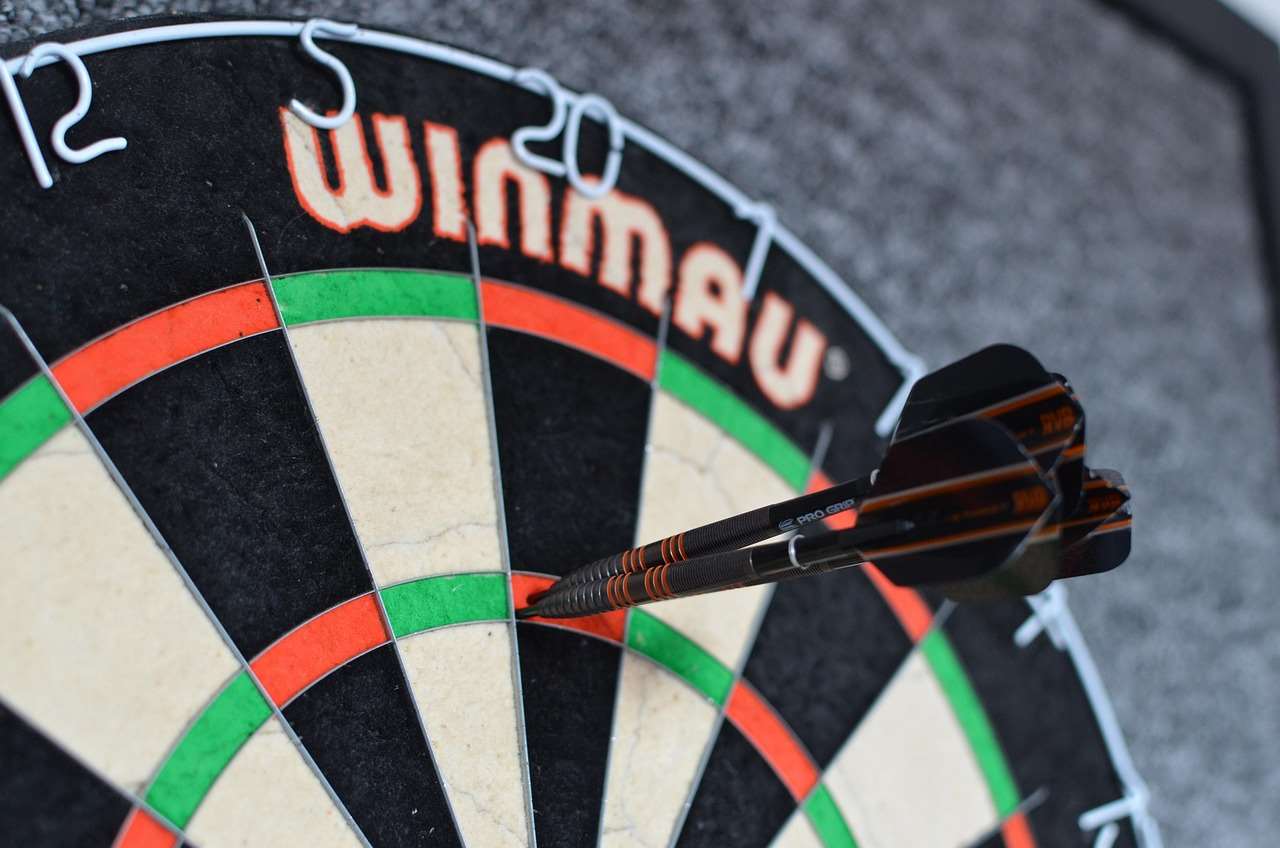
Optimization and Performance Considerations
For smaller iterables, the performance difference between different counting methods is negligible. Echter, for larger datasets, the choice of method can significantly impact performance. Understanding the underlying data structure and choosing the appropriate approach is crucial. Prefer using the .length
property whenever possible, as it’s generally the fastest and most efficient method, especially for lists. Echter, for situations involving streams or other iterables without a .length
property, employing appropriate techniques like chunking or parallel processing can be crucial.
Always profile your code to identify performance bottlenecks. Dart’s profiling tools can help you pinpoint areas where optimizations are needed. Measuring execution time with different approaches can guide your selection of the most suitable counting method. When working with large datasets, remember to also consider memory management to prevent potential out-of-memory exceptions.
Practical Applications of Dart Iterable Count
Determining the dart iterable count is a fundamental task with a wide range of applications in Dart development. Whether you’re working with user data, processing sensor readings, or handling network responses, knowing the number of items in an iterable is often the first step in various operations.
- Data validation: Checking if a form submission has the expected number of fields.
- Progress indicators: Displaying the progress of a long-running operation by tracking the number of processed items.
- Conditional logic: Determining whether to proceed with a certain operation based on the size of a dataset.
- Pagination: Breaking down large datasets into smaller, manageable pages for better user experience. A darts scoreboard electronic app, bijvoorbeeld, might use pagination to display numerous game results.
- Resource allocation: Determining the amount of memory or processing power required to handle a certain task based on the data size.
These are just a few examples, showcasing how ubiquitous the need for determining dart iterable count is in various Dart programming scenarios.
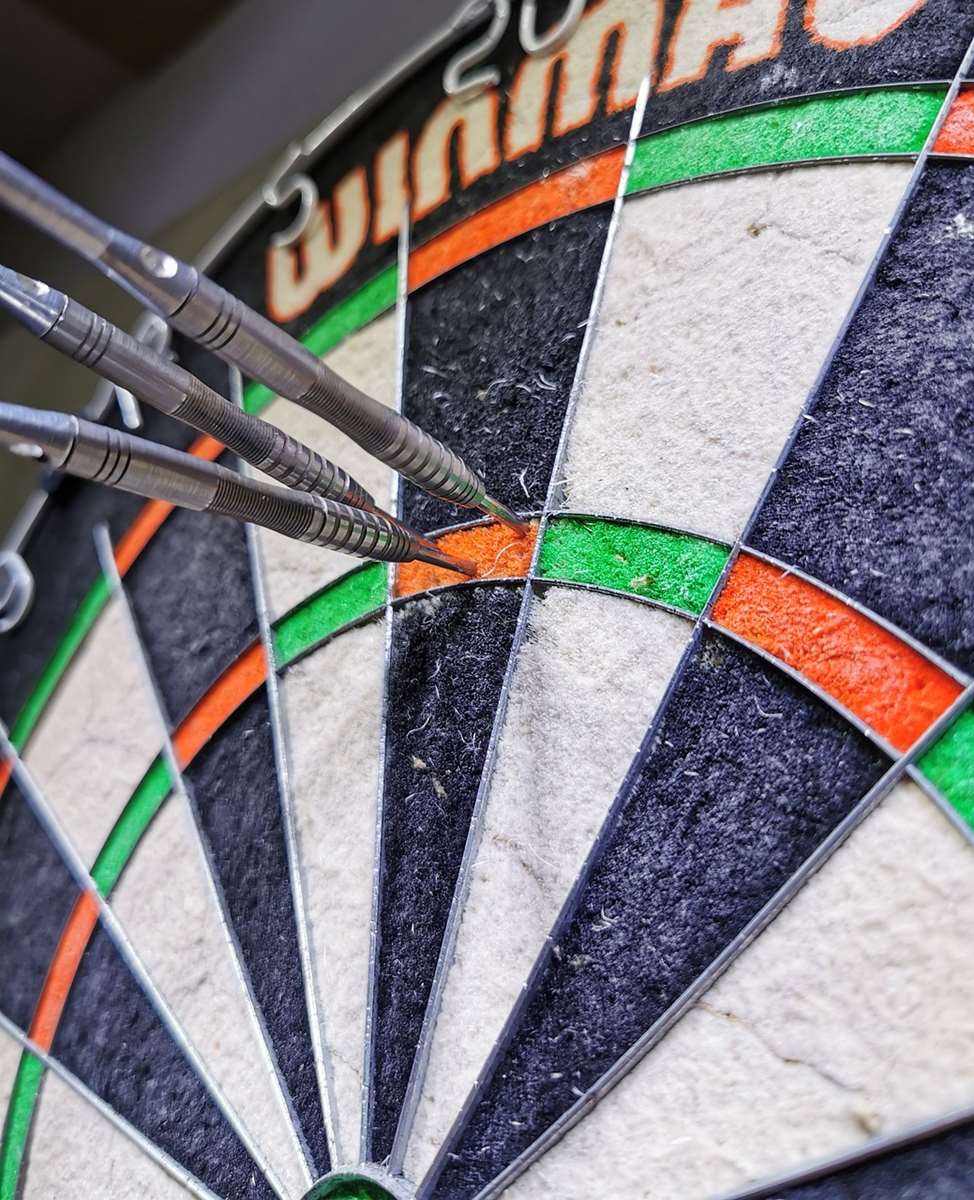
Advanced Techniques and Libraries
While the core methods already discussed suffice for many situations, you might encounter scenarios where specialized libraries or more advanced techniques provide further optimization or streamlined workflows. Exploring options like the collection libraries or external packages tailored for data processing can broaden your toolset and enhance your efficiency when dealing with complex iterable structures.
Consider exploring the various capabilities of collections available in Dart. Libraries such as the collection package offer advanced data structures and algorithms. Investigating what these libraries offer could open up new possibilities, potentially simplifying your tasks or enhancing performance.
For particularly large or complex data sets, consider leveraging packages designed for large-scale data processing. Such packages can provide substantial performance benefits over conventional methods.
Conclusie
Efficiently determining the dart iterable count is a vital skill for any Dart developer. While using the .length
property offers the simplest and often fastest approach for lists, understanding alternative methods for handling other iterables, such as Sets, Maps, and Streams, is equally important. This understanding extends to managing large datasets and infinite iterables, where techniques like sampling, chunking, and parallel processing become vital. By mastering these methods and incorporating robust error handling, you’ll write more efficient and reliable Dart code. Remember to choose the optimal method based on the specific characteristics of your data and application needs. So start exploring the various techniques, practice what you’ve learned, and watch your Dart development efficiency soar. Happy coding!
To further enhance your Dart skills, check out our comprehensive guide on dart flight path and explore the possibilities of a Darts scoreboard app.
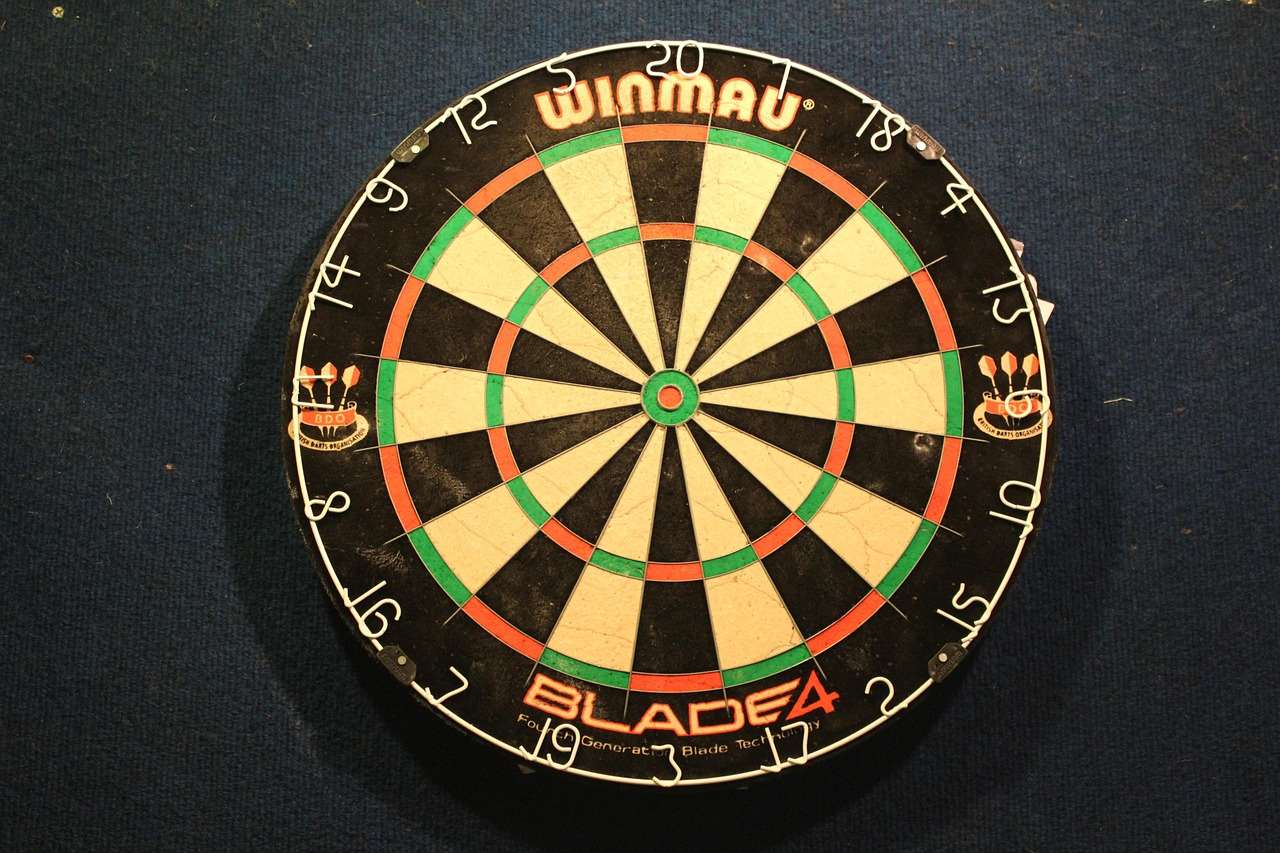
Hoi, Ik ben Dieter, En ik heb Dartcounter gemaakt (Dartcounterapp.com). Mijn motivatie was geen darts -expert - helemaal tegenovergestelde! Toen ik voor het eerst begon te spelen, Ik hield van het spel, maar vond het moeilijk en afleidend om nauwkeurige scores te houden en statistieken te volgen.
Ik dacht dat ik niet de enige kon zijn die hiermee worstelde. Dus, Ik besloot om een oplossing te bouwen: een eenvoudig te gebruiken applicatie die iedereen, Ongeacht hun ervaringsniveau, zou kunnen gebruiken om moeiteloos te scoren.
Mijn doel voor Dartcounter was eenvoudig: Laat de app de nummers afhandelen - het scoren, de gemiddelden, de statistieken, Zelfs checkout suggesties - zodat spelers puur kunnen richten op hun worp en genieten van het spel. Het begon als een manier om het probleem van mijn eigen beginners op te lossen, En ik ben heel blij dat het is uitgegroeid tot een nuttig hulpmiddel voor de bredere darts -community.