Converting an integer to a double in Dart is straightforward: simply use the toDouble()
method. This provides a concise and efficient way to perform the dart double from int conversion, ensuring smooth data handling in your applications. This article will delve deeper into this process, exploring various scenarios, potential pitfalls, and best practices for handling dart double from int conversions effectively.
Still Using Pen & Paper (or a Chalkboard)?! 
Step into the future! The Dart Counter App handles all the scoring, suggests checkouts, and tracks your stats automatically. It's easier than you think!
Try the Smart Dart Counter App FREE!Ready for an upgrade? Click above!
Understanding the nuances of converting between integer and double data types is crucial for any Dart developer. While the basic conversion using toDouble()
is simple, this article will equip you with the knowledge to handle more complex situations and avoid common errors. We’ll cover different approaches, explore potential loss of precision, and offer practical examples to solidify your understanding.
Converting Dart Int to Double: The toDouble()
Method
The most direct and efficient method for achieving a dart double from int conversion is by utilizing the built-in toDouble()
method. This method is part of the core Dart language and is designed for this specific purpose. It’s incredibly simple to use; you just call the method on your integer variable.
int myInt = 10;
double myDouble = myInt.toDouble();
print(myDouble); // Output: 10.0
As you can see, the code snippet above clearly demonstrates the ease of this conversion. The toDouble()
method seamlessly transforms the integer myInt
into its double equivalent, myDouble
. This is a crucial technique for many programming tasks, particularly when dealing with calculations requiring floating-point precision, like those in financial applications or scientific simulations. Remember, understanding how dart double from int conversions work is vital for writing clean and efficient Dart code.
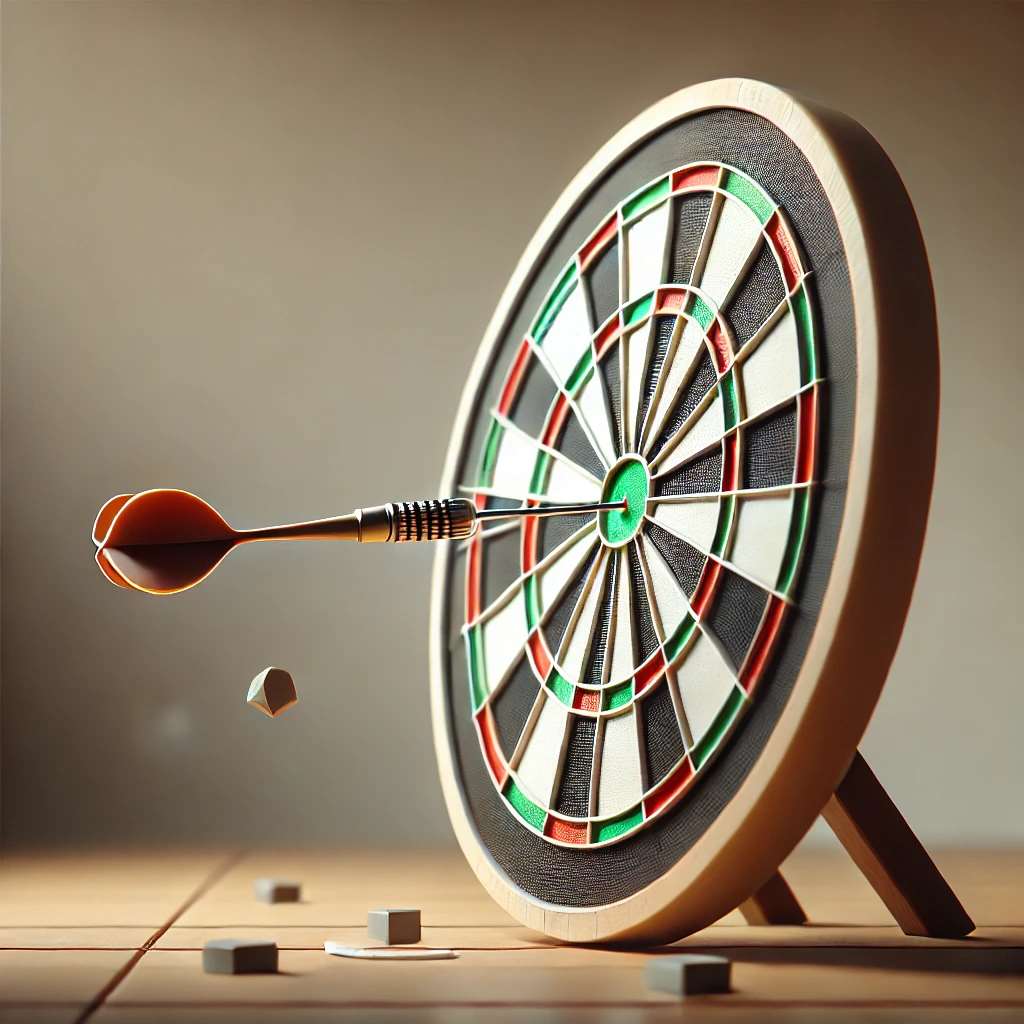
Implicit Type Conversion
In certain contexts, Dart might perform an implicit type conversion from int
to double
without requiring you to explicitly call toDouble()
. This usually happens when an integer value is used in an operation where a double is expected. For instance, if you divide an integer by another integer and assign the result to a double variable, Dart will automatically perform the conversion for you.
int num1 = 5;
int num2 = 2;
double result = num1 / num2; // Implicit conversion to double
print(result); // Output: 2.5
However, it’s generally good practice to be explicit with your type conversions. Using toDouble()
makes your code more readable and easier to understand, reducing the potential for confusion or unexpected behavior. Explicit type conversions help to promote code maintainability and avoid subtle bugs, especially when working on larger projects. This becomes even more vital when dealing with complex data structures and algorithms.
Handling Potential Precision Issues
While converting from dart double from int usually doesn’t introduce precision problems (since integers can be exactly represented as doubles), it’s always worth considering the broader context of floating-point arithmetic. Doubles, being approximations of real numbers, might exhibit subtle inaccuracies in certain computations. This can be particularly relevant when performing repeated calculations or dealing with very large or very small numbers.
For instance, if you’re working with financial applications where precise values are critical, you might want to explore using specialized libraries designed for arbitrary-precision arithmetic. These libraries provide greater accuracy but often come with a performance trade-off. It’s important to balance the accuracy requirements of your application with the overall performance constraints.
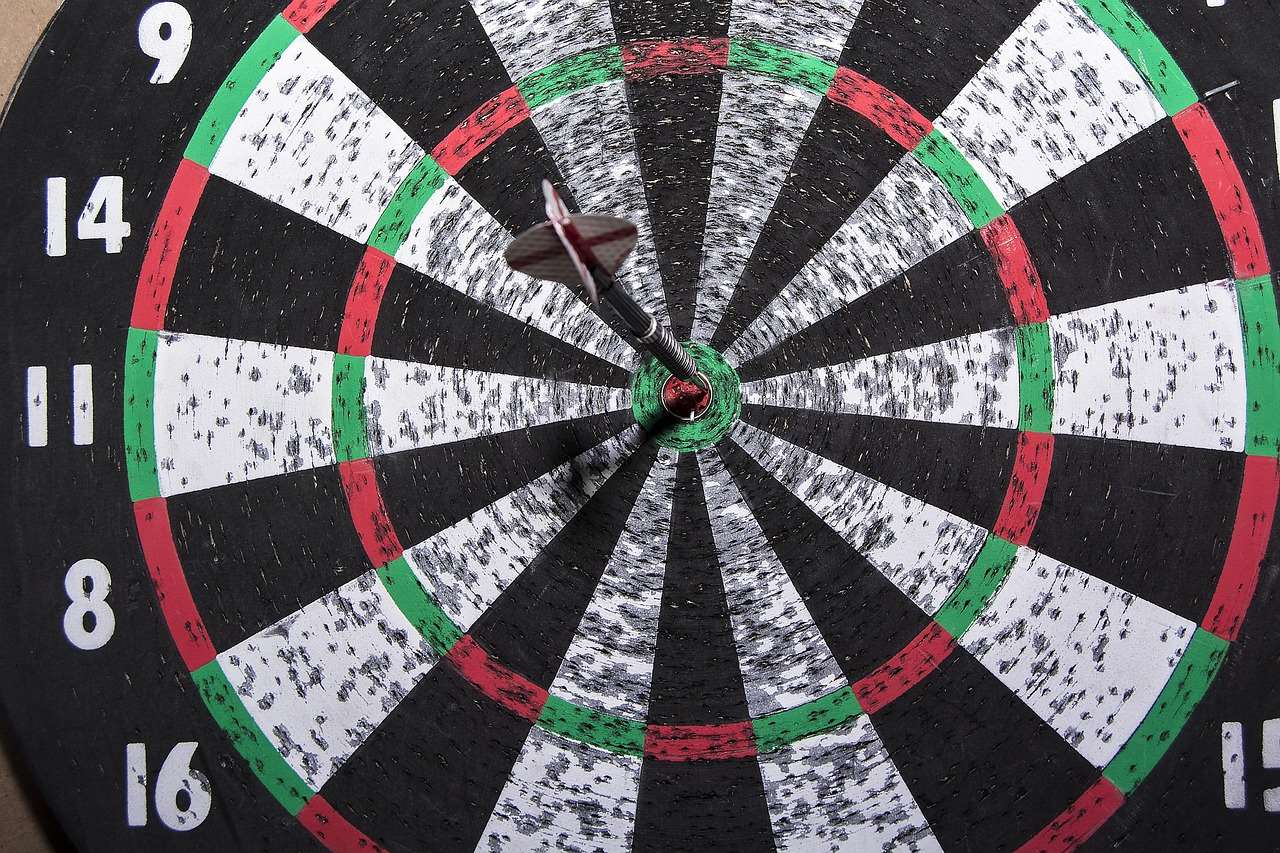
Comparing Doubles for Equality
Due to the inherent imprecision of floating-point numbers, directly comparing doubles for equality using the ==
operator can lead to unexpected results. Instead of checking for strict equality, you might need to introduce a tolerance value to determine if two doubles are “close enough” to be considered equal.
double a = 0.1 + 0.2;
double b = 0.3;
const double tolerance = 1e-9; // a small tolerance value
if ((a - b).abs() < tolerance) {
print("a and b are approximately equal");
}
This approach is crucial when you’re comparing doubles calculated in different ways or coming from different sources. Remember, floating-point representation errors can accumulate, so the choice of tolerance becomes significant, especially in iterative processes. For more information on effective use of floating-point numbers in calculations, consult resources on numerical analysis. Remember that precise handling of dart double from int is not only about the initial conversion but about how the resulting double values are further manipulated and compared within your program.
Advanced Scenarios and Best Practices
Beyond the simple toDouble()
method, there are more sophisticated scenarios where you might need a more nuanced approach to converting from dart double from int. Consider situations involving complex data structures, parsing external data, or integrating with other systems.
- JSON Parsing: When working with JSON data, you’ll often encounter numbers represented as strings. In such cases, you’ll need to parse these strings into numbers, potentially converting them from integers to doubles using
double.parse()
ordouble.tryParse()
(for error handling). - Database Interactions: Interactions with databases might require careful consideration of data types. Depending on your database system and how you’re accessing it, you might need to explicitly handle type conversions between
int
anddouble
. Ensure consistency between how you define your data and how it is handled by the database. - Working with Libraries: Some external Dart libraries might have specific requirements for input data types. Make sure to check the library documentation to see how they handle dart double from int conversions and conform to their expectations.
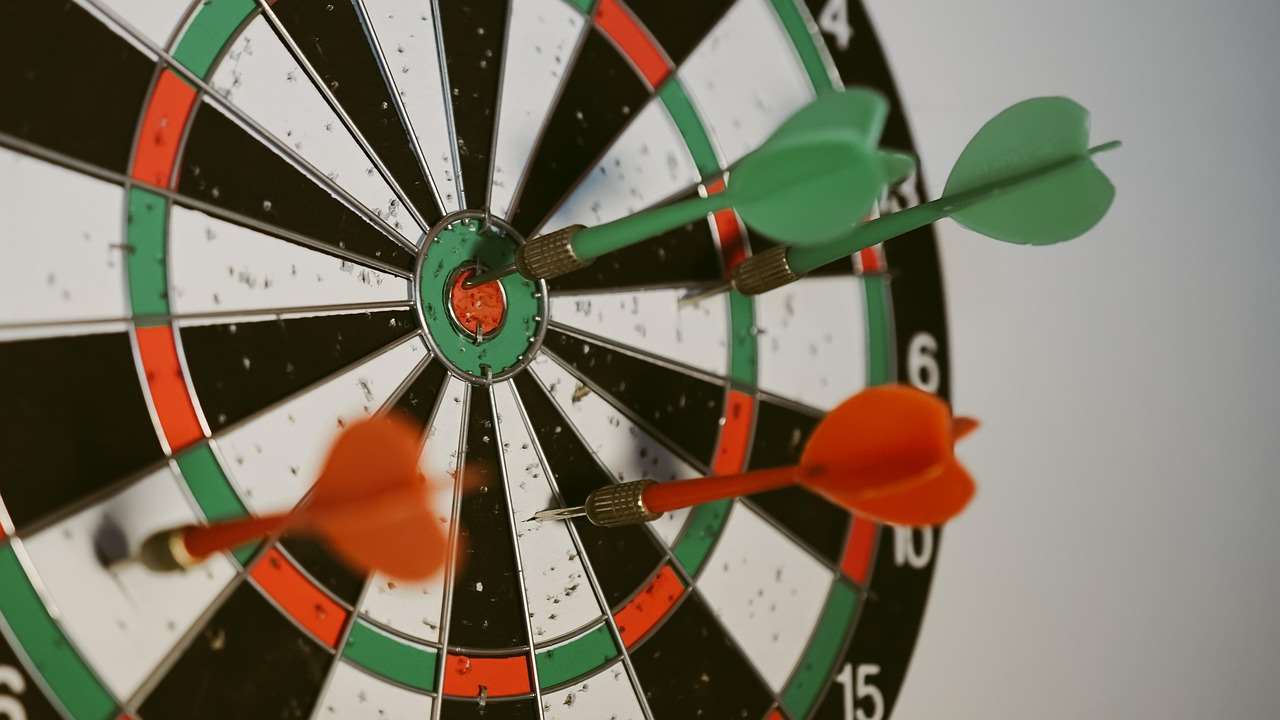
In many instances, choosing to use a double
even when starting with an int
can make your code more flexible and robust. It avoids needing to convert later when working with algorithms or libraries that inherently utilize floating-point arithmetic. Choosing the right data type in the first place is a proactive step toward cleaner and more maintainable code. Always remember to document your design decisions related to data types to help future developers understand the rationale behind your choices.
Example: Calculating Averages
Let's consider a practical example: calculating the average of a list of integers. Since averages can result in fractional values, it's necessary to convert the integers to doubles to avoid integer truncation.
List numbers = [1, 2, 3, 4, 5];
double sum = numbers.fold(0.0, (sum, number) => sum + number.toDouble());
double average = sum / numbers.length;
print(average); // Output: 3.0
In this example, we explicitly convert each integer in the list to a double before summing them. This ensures the accuracy of the average calculation, preventing potential loss of precision resulting from integer division. Using this method for your dart double from int operations guarantees proper handling of fractional results.
For more advanced scenarios, you might want to explore using streams for more efficient handling of large datasets, particularly in performance-critical applications. The methods described here form the foundation for effective data handling in various situations. Understanding the fundamentals of dart double from int conversions is crucial regardless of the complexity of your project.

Integrating with External Systems
When interacting with external systems or APIs, you might encounter data type mismatches. For instance, an API might return an integer value, but your application requires a double. Always carefully check the documentation for the external system to understand its data types and handle any necessary conversions appropriately. Consider using well-tested libraries or utility functions for handling conversions between data types when dealing with external APIs or data sources.
For instance, if you are using a Digital dart score app and retrieving scores, you may need to perform dart double from int conversion if the API returns integer scores but your application needs to display averages as floating-point numbers. Appropriate error handling is also critical, especially when integrating with external systems. Consider what happens if the external system returns unexpected or invalid data. Always employ robust error handling mechanisms to prevent application crashes or unexpected behavior.
Conclusion
Converting from a dart double from int is a fundamental operation in Dart programming. The simple toDouble()
method provides a convenient and efficient way to perform this conversion. However, understanding the nuances of floating-point arithmetic, potential precision issues, and handling diverse data formats are crucial for writing robust and reliable applications. By following the best practices outlined in this article, you can avoid common pitfalls and ensure your code handles dart double from int conversions effectively, regardless of the complexity of your project. Remember to choose the appropriate data type for your variables to ensure clarity and prevent unexpected behavior. For precise computations, consider using libraries suited for arbitrary precision arithmetic. Always thoroughly test your code, considering edge cases and various inputs to ensure robustness and reliability.
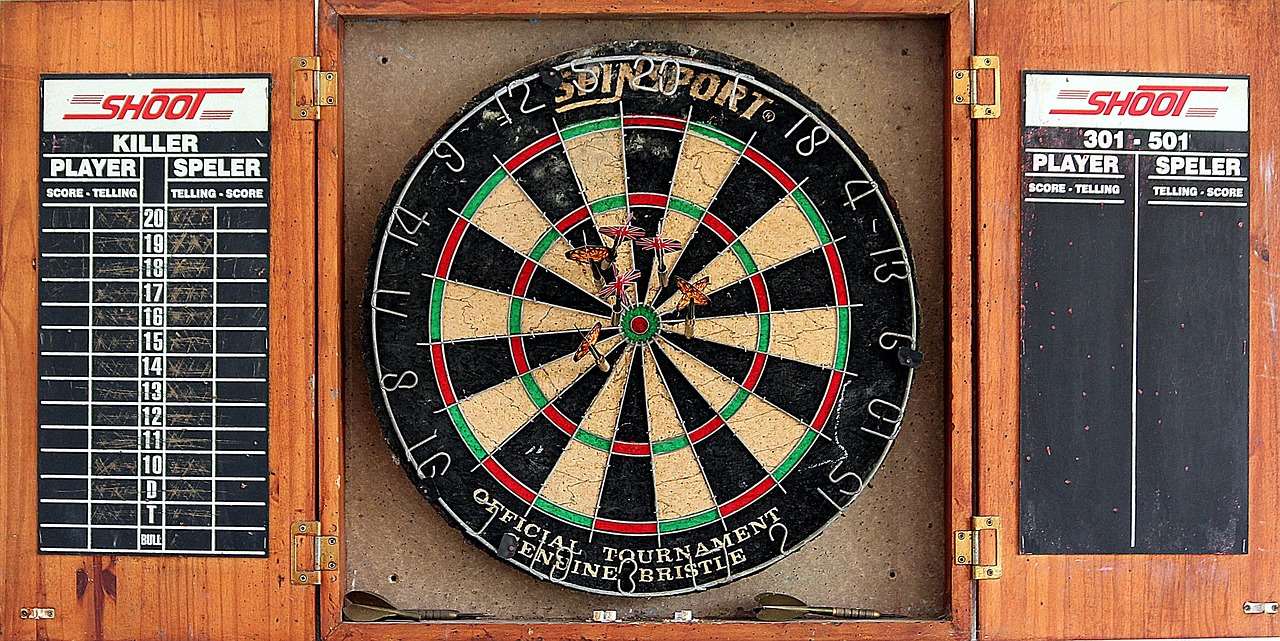
Start optimizing your Dart code today by applying these techniques for efficient and accurate dart double from int conversions! Remember to consult the Dart language documentation for more detailed information and explore advanced topics relevant to your specific project needs. Happy coding!
Consider exploring other related topics such as mission precision darts, baseball darts, dartbord afmetingen, unicorn barrel darts, and unicorn dart board to further enhance your Dart development skills.
Hi, I’m Dieter, and I created Dartcounter (Dartcounterapp.com). My motivation wasn’t being a darts expert – quite the opposite! When I first started playing, I loved the game but found keeping accurate scores and tracking stats difficult and distracting.
I figured I couldn’t be the only one struggling with this. So, I decided to build a solution: an easy-to-use application that everyone, no matter their experience level, could use to manage scoring effortlessly.
My goal for Dartcounter was simple: let the app handle the numbers – the scoring, the averages, the stats, even checkout suggestions – so players could focus purely on their throw and enjoying the game. It began as a way to solve my own beginner’s problem, and I’m thrilled it has grown into a helpful tool for the wider darts community.