The dart double exclamation mark in Dart programming signifies a null-aware assertion operator. It’s a concise way to check if a variable is not null before accessing its members, avoiding potential NullPointerExceptions
. This article will delve into its functionality, providing practical examples and exploring related concepts in Dart development.
Still Using Pen & Paper (or a Chalkboard)?! 
Step into the future! The Dart Counter App handles all the scoring, suggests checkouts, and tracks your stats automatically. It's easier than you think!
Try the Smart Dart Counter App FREE!Ready for an upgrade? Click above!
Understanding the dart double exclamation mark is crucial for writing robust and error-free Dart code. It’s a powerful tool that helps prevent runtime crashes caused by null values, a common issue in object-oriented programming. This comprehensive guide will equip you with the knowledge to confidently use this operator and improve the reliability of your applications.
Understanding the Dart Double Exclamation Mark (!!)
The dart double exclamation mark, or the null-aware assertion operator (!!), is a concise and efficient way to handle potential null values in Dart. It asserts that an expression is not null; if it is, it throws a NullPointerException
. This allows for more direct code without the need for extensive null checks using conditional statements like if
and else
. This direct approach can make your code easier to read and maintain, especially when dealing with deeply nested objects or complex data structures.
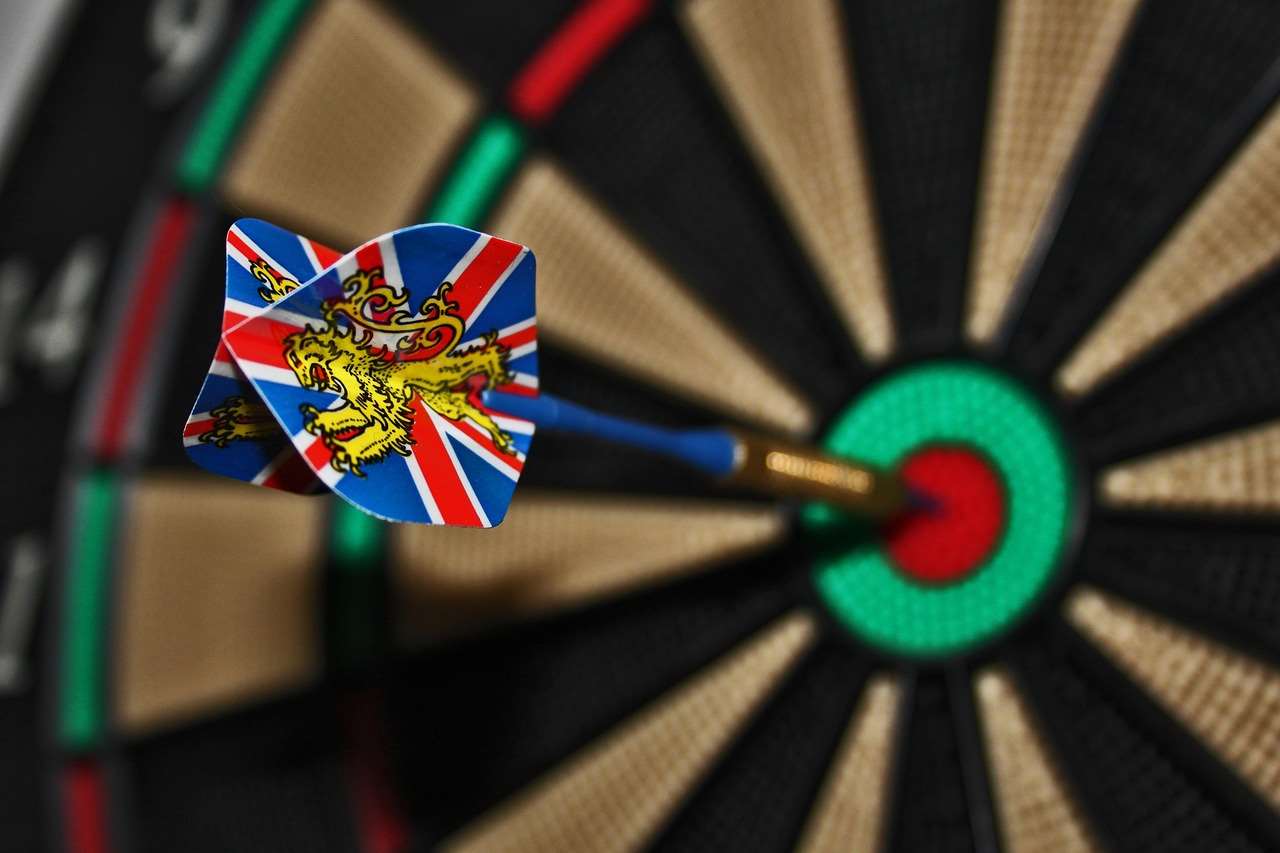
For instance, consider a scenario where you have a user object that might be null: User? user;
. If you want to access the user’s name, you could write:
String userName = user!.name; // Using the null-aware assertion operator
This code will execute without issue if user
is not null. However, if user
is null, a NullPointerException
will be thrown at runtime. This is different from the null-aware operator (?.
), which would return null in the case of a null value. The dart double exclamation mark makes it absolutely clear that you’re confident that the value isn’t null – and the runtime will confirm it.
When to Use the !! Operator
The dart double exclamation mark should be used judiciously. It is best employed when you are absolutely certain that a variable cannot be null at a given point in your code. Overusing it can mask underlying null-handling issues and lead to difficult-to-debug errors. Consider situations where rigorous null checks have already been performed upstream, making it highly improbable that the variable will be null here. A good example is immediately after a successful database query where a non-null object is expected.
Alternatives to the Dart Double Exclamation Mark
While the dart double exclamation mark offers a compact syntax, it’s important to understand its limitations and explore alternative approaches. The null-aware operator (?.
) provides a safer alternative in many cases, particularly when the possibility of a null value exists. This operator allows you to safely access members of an object only if the object is not null, gracefully returning null
if the object is null. This helps prevent runtime crashes.
For example, using the null-aware operator:
String? userName = user?.name; // Safe access: returns null if user is null
This approach is generally preferred unless you’re absolutely sure the variable is non-null. It’s often better practice to handle potential null values explicitly rather than relying on exceptions to reveal them.
The Null-Aware Operator (?.)
The null-aware operator (?.
) is crucial for handling potential null
values. Unlike the dart double exclamation mark which throws an exception if the value is null, ?.
gracefully returns null
, allowing you to handle the situation safely within your code. This prevents unexpected crashes and provides more predictable program behavior.
Consider using the null-aware operator (`?.`) combined with the null-coalescing operator (`??`) to provide default values when a variable might be null. This provides a more elegant and robust way to handle null values than relying solely on the dart double exclamation mark.
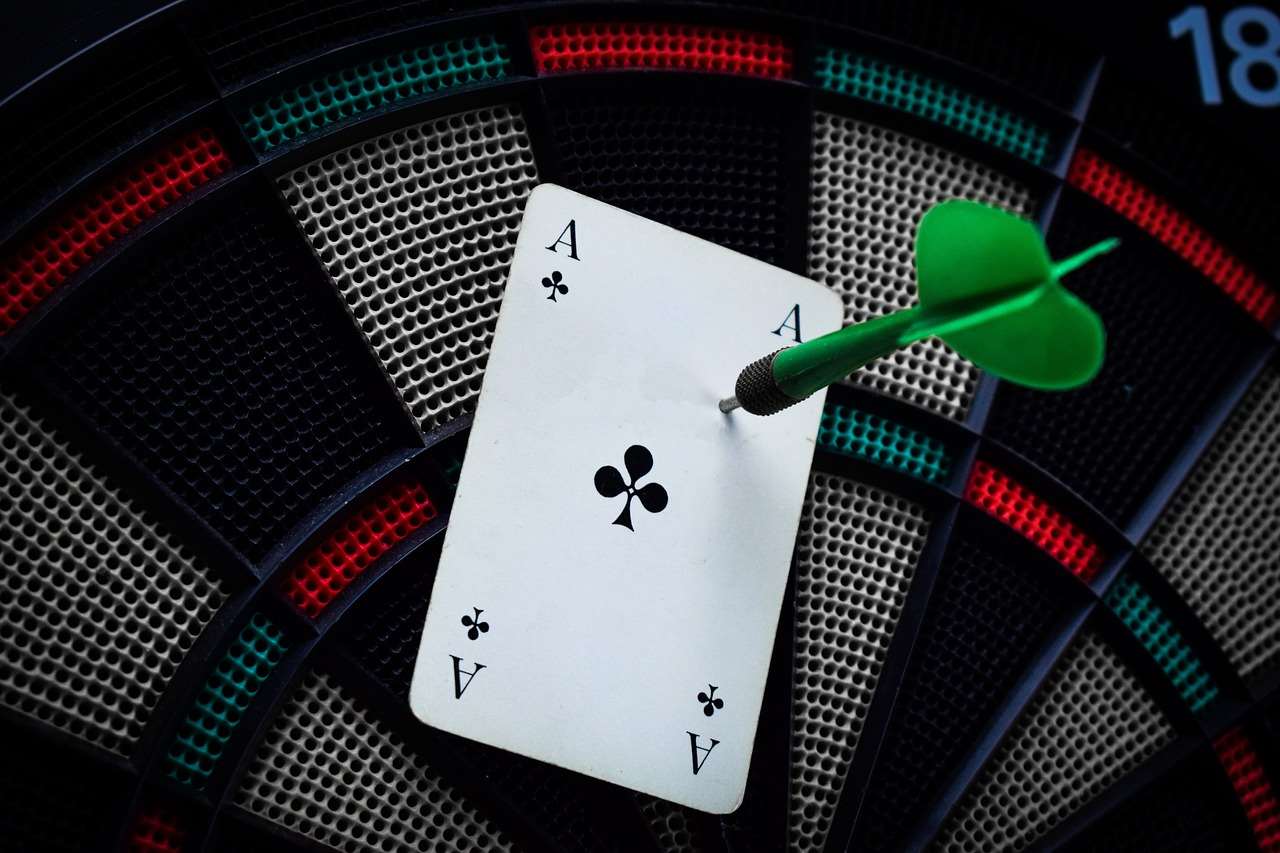
Practical Examples and Best Practices
Let’s examine a few scenarios to illustrate the proper and improper use of the dart double exclamation mark. Consider a function that retrieves user data from a database. If you’re certain that the database query will always return a user, you can use the !!
operator:
String getUserName(int userId) {
final user = getUserFromDatabase(userId); // Assume this always returns a non-null user
return user!!.name;
}
However, if there’s even a remote chance that getUserFromDatabase
might return null (e.g., due to a network error or the user not existing), then you should employ safer null-handling techniques, such as:
String? getUserName(int userId) {
final user = getUserFromDatabase(userId);
return user?.name;
}
Or a more comprehensive approach:
String getUserName(int userId) {
final user = getUserFromDatabase(userId);
return user?.name ?? 'Unknown User'; // Provides a default value if user is null
}
This handles the null case gracefully and provides a meaningful default. Remember, the dart double exclamation mark should be reserved for situations where you are unequivocally certain that a null value is impossible. In most other situations, the null-aware operator and the null-coalescing operator provide a far more robust and error-resistant approach to null-safety.
Using the dart double exclamation mark improves code conciseness where absolutely necessary, but always prioritize defensive programming practices. Consider using static analysis tools to identify potential NullPointerExceptions
. These tools are invaluable in improving code reliability and mitigating potential runtime errors. They’ll often flag areas where a dart double exclamation mark might lead to unexpected behavior.
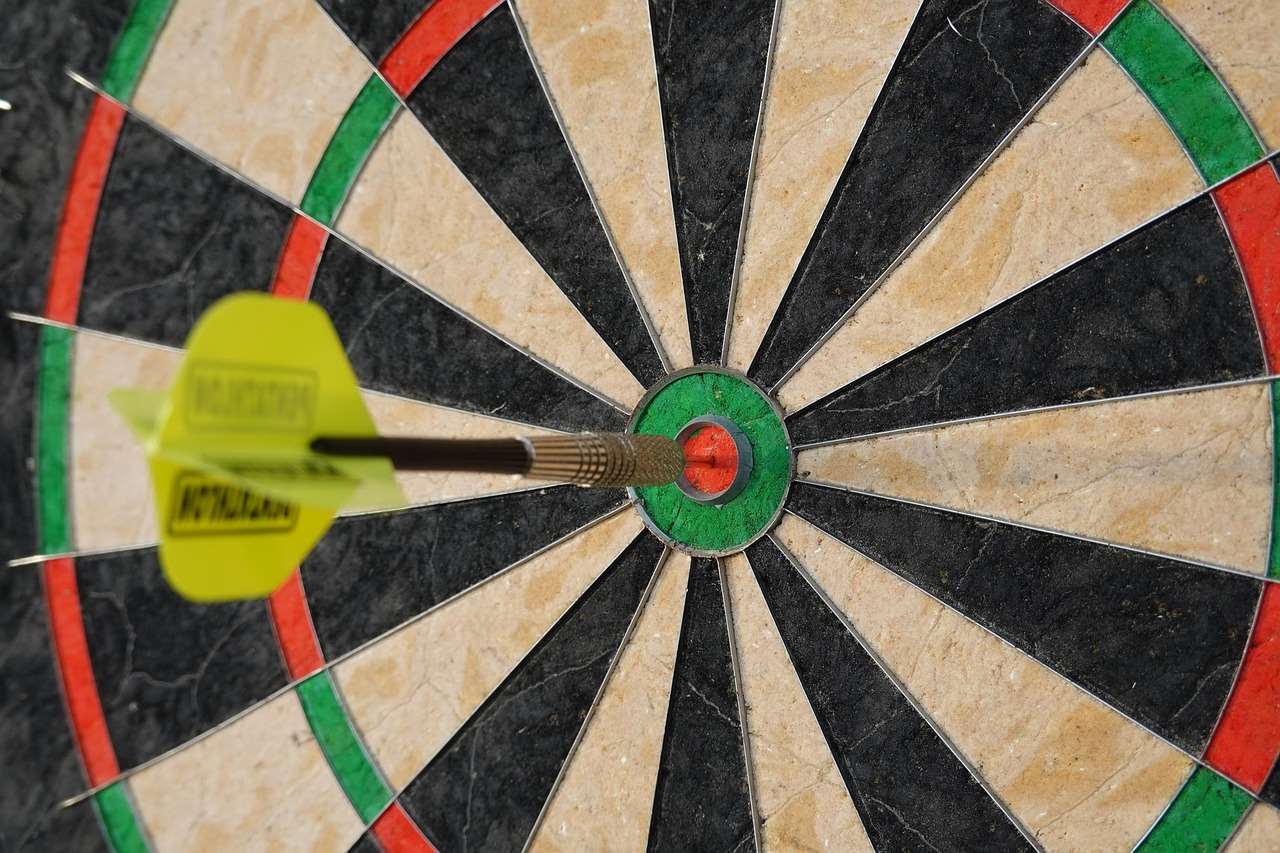
Null Safety in Dart
Dart’s null safety features, introduced in Dart 2.12, significantly enhance the language’s ability to prevent null pointer exceptions. Understanding null safety is essential to effectively utilize the dart double exclamation mark. The core concept is that by default, variables cannot be null unless explicitly declared as nullable (using a ?
after the type). This helps catch many potential null-related issues during compile time rather than at runtime.
However, there will be instances where you might need to bypass this safety net, which is where the dart double exclamation mark comes into play. Remember, the appropriate use is limited to those cases where you’re completely sure the value will never be null at the specific point in your code. If there’s any doubt whatsoever, it’s far safer to use alternative null-handling strategies.
Advanced Use Cases and Considerations
While often used with simple objects, the dart double exclamation mark can also be applied within more complex scenarios. When dealing with nested objects, using !!
can lead to cleaner code, provided you are confident in the absence of null values at each stage of the nested access. Remember, however, that the responsibility for ensuring non-null values lies entirely with the developer. The compiler does not actively prevent the use of the !!
operator even in cases where it may be inappropriate.

One area to exercise particular caution is within asynchronous operations. If you are retrieving data from an API or a database asynchronously, you must ensure that your code handles the potential for null values appropriately. Even if you are sure the API should return data, temporary network issues or server errors could lead to a null response. In these situations, using the !!
operator can result in runtime exceptions, causing your application to crash or behave unpredictably. Always prefer asynchronous error handling techniques when dealing with asynchronous data retrieval.
For example, consider a scenario that retrieves user information from an online database. Let’s say you have a function fetchUser(userId)
which returns a Future
. Because it’s asynchronous, there is always the possibility that an error occurs, or the user simply doesn’t exist. Therefore, relying on !!
here would be inappropriate. You should instead use either the ?.
operator or handle possible errors correctly within the asynchronous operation.
Consider using dart set replace when working with collections.
Conclusion
The dart double exclamation mark provides a concise syntax for asserting non-null values in Dart. However, its use should be carefully considered. While it can improve code readability in certain situations, it’s crucial to prioritize null safety and defensive programming practices. Overusing the dart double exclamation mark can lead to less robust and harder-to-maintain code. The null-aware operator (?.
) and null-coalescing operator (??
) generally provide safer and more reliable alternatives. By understanding these concepts and employing them judiciously, you can create more resilient and less error-prone Dart applications. Remember to leverage the strengths of Dart’s null safety features to minimize the need for this operator. By using best practices, you can create cleaner and more robust applications. Check out Dart Counter App for a practical application of Dart principles. Also, for further reading on Dart’s null safety features, consider exploring other resources on this topic.
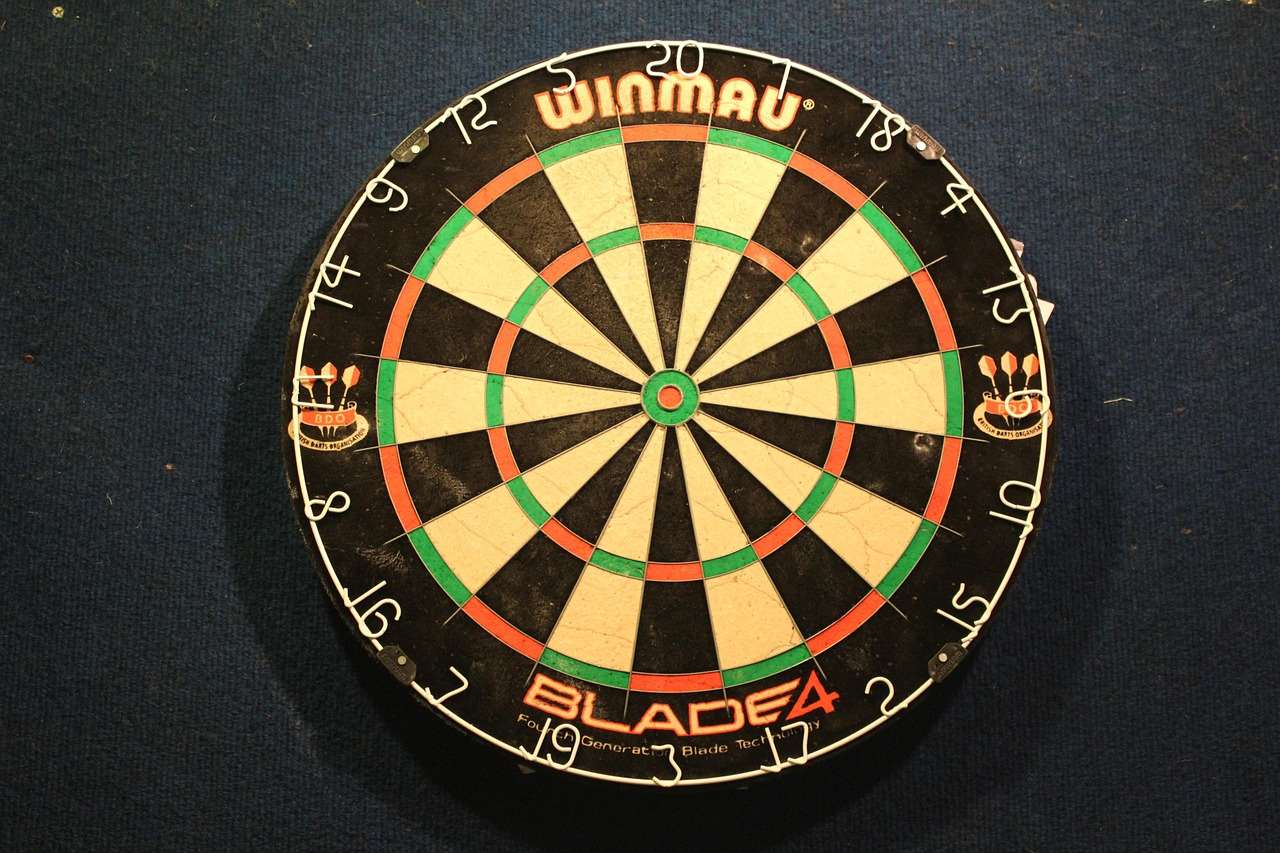
Always remember to thoroughly test your code to ensure the absence of null values at the points where you use the dart double exclamation mark. Failing to do so can lead to unexpected crashes and difficult-to-debug issues. The careful and informed application of the dart double exclamation mark, combined with sound error-handling and appropriate use of the null-aware operator, will allow you to write clean, efficient, and robust Dart code.
Explore our other resources on darts champion league, mdarts.fr, darts no score, dart point won’t stay in, darts 180 call, bedlington darts players, dart board online, dartboard cabinet light, and darts cases for more information on the world of darts.
Hi, I’m Dieter, and I created Dartcounter (Dartcounterapp.com). My motivation wasn’t being a darts expert – quite the opposite! When I first started playing, I loved the game but found keeping accurate scores and tracking stats difficult and distracting.
I figured I couldn’t be the only one struggling with this. So, I decided to build a solution: an easy-to-use application that everyone, no matter their experience level, could use to manage scoring effortlessly.
My goal for Dartcounter was simple: let the app handle the numbers – the scoring, the averages, the stats, even checkout suggestions – so players could focus purely on their throw and enjoying the game. It began as a way to solve my own beginner’s problem, and I’m thrilled it has grown into a helpful tool for the wider darts community.