Mastering dart json is crucial for any Dart developer building applications that interact with APIs or need to handle data efficiently. This article provides a comprehensive guide to working with dart json, covering encoding, decoding, and best practices. You’ll also learn about common pitfalls and how to avoid them.
⚠️ Still Using Pen & Paper (or a Chalkboard)?! ⚠️
Step into the future! The Dart Counter App handles all the scoring, suggests checkouts, and tracks your stats automatically. It's easier than you think!
Try the Smart Dart Counter App FREE!Ready for an upgrade? Click above!
Before diving into the specifics, let’s address a fundamental question: why is dart json so important? The answer lies in its role as the standard method for representing structured data in a human-readable format. This is vital for communication between your Dart application and external services. This makes understanding how to effectively utilize dart json a fundamental skill for any serious Dart developer.
We’ll delve into various aspects, from basic encoding and decoding to handling complex data structures and efficient error handling. You’ll also learn about relevant packages and libraries that can streamline your workflow, helping you write cleaner and more maintainable code. Get ready to level up your Dart skills!
Understanding Dart JSON: Encoding and Decoding
At its core, dart json processing revolves around two key operations: encoding and decoding. Encoding transforms Dart objects (like lists and maps) into JSON strings, while decoding does the opposite – converting JSON strings back into Dart objects. The core functionality for this lies within the `dart:convert` library, which provides the `jsonEncode` and `jsonDecode` functions. Let’s explore them further.
Encoding Dart Objects to JSON
Encoding a Dart object into JSON is straightforward using the `jsonEncode` function. This function takes a Dart object as input and returns a JSON string representation. For instance:
import 'dart:convert';
void main() {
final data = {'name': 'John Doe', 'age': 30, 'city': 'New York'};
final jsonString = jsonEncode(data);
print(jsonString); // Output: {"name":"John Doe","age":30,"city":"New York"}
}
This code snippet demonstrates how to encode a simple map into a JSON string. The output is a valid JSON string that can be easily sent to an API or stored in a file.
Decoding JSON to Dart Objects
Decoding JSON strings back into Dart objects is equally simple using the `jsonDecode` function. This function takes a JSON string as input and returns the corresponding Dart object.
import 'dart:convert';
void main() {
final jsonString = '{"name":"Jane Doe","age":25,"city":"London"}';
final data = jsonDecode(jsonString);
print(data); // Output: {name: Jane Doe, age: 25, city: London}
}
This example shows how to decode a JSON string into a Dart map. Note that the decoded object’s type will depend on the structure of the JSON string.
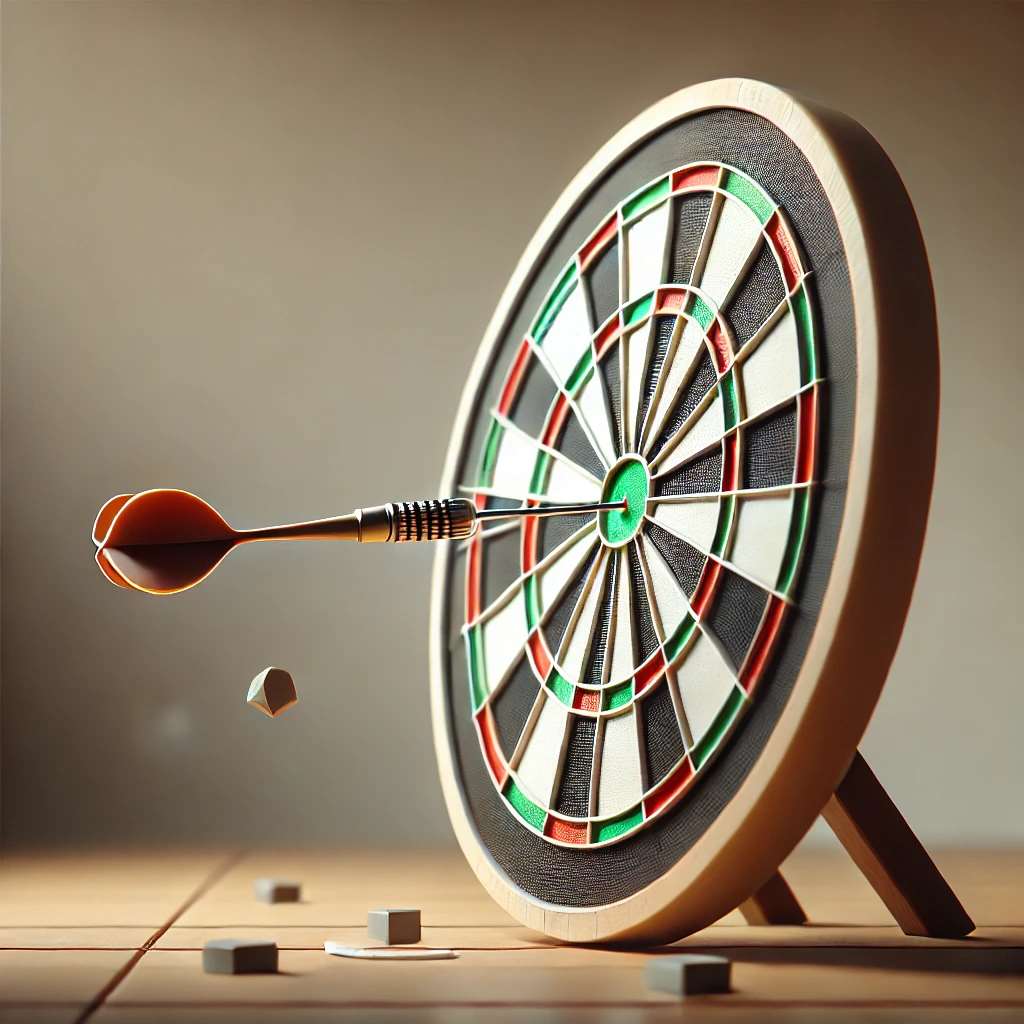
Handling Complex Dart JSON Structures
While simple maps and lists are easy to handle, real-world applications often involve more complex dart json structures. These might include nested maps, lists of maps, or custom Dart classes. Let’s look at how to manage such structures effectively.
Working with Nested JSON
Dealing with nested JSON requires a bit more attention. You’ll need to recursively traverse the JSON structure to access the data at different levels. Consider this example:
import 'dart:convert';
void main() {
final jsonString = '{"user": {"name": "Alice", "address": {"street": "123 Main St", "city": "Anytown"}}}';
final data = jsonDecode(jsonString);
print(data['user']['name']); // Output: Alice
print(data['user']['address']['city']); // Output: Anytown
}
Here, we access nested fields using chained indexing. This approach works well for simple nested structures but can become cumbersome for deeply nested JSON.
Custom Dart Classes and JSON Serialization
For more complex scenarios, creating custom Dart classes to represent your JSON data is highly recommended. This enhances code readability and maintainability. You can leverage packages like `json_serializable` to automate the process of generating code for converting between Dart classes and JSON. This simplifies handling complex structures significantly. The dart json processing becomes more organized and less error-prone.
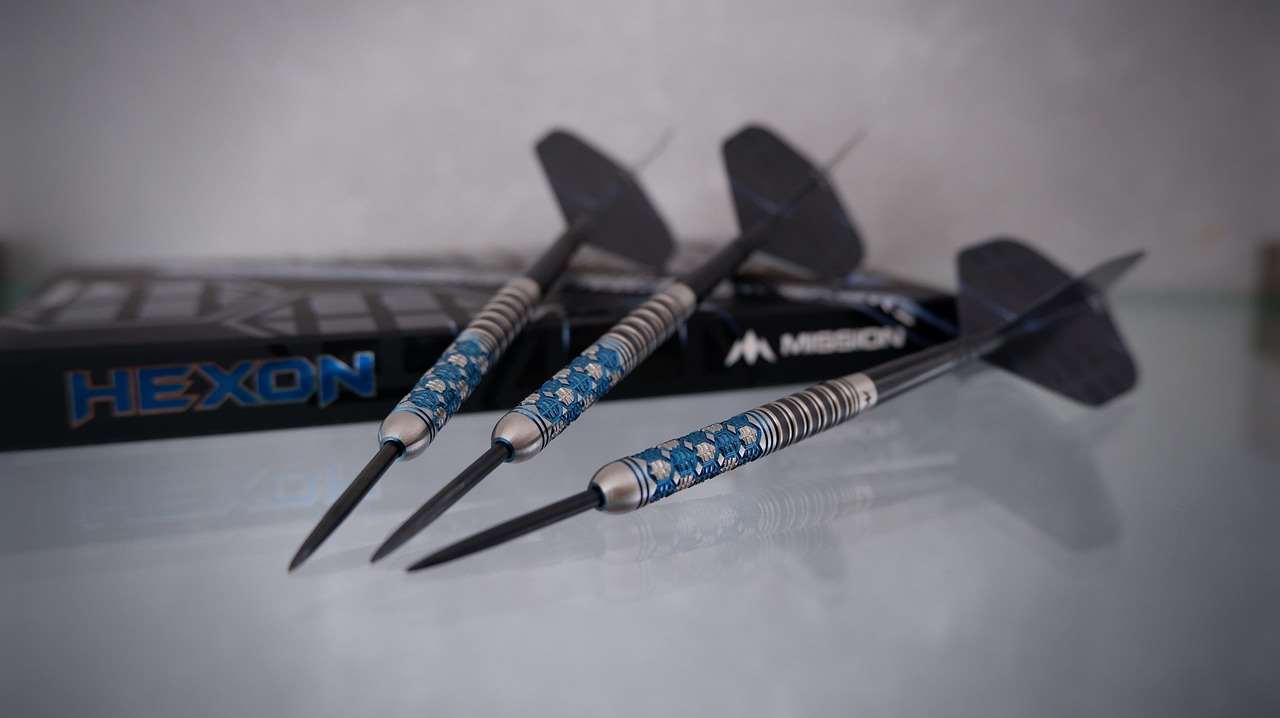
Error Handling in Dart JSON Processing
Robust error handling is crucial when dealing with dart json, as invalid or malformed JSON can easily crash your application. Always anticipate potential errors and incorporate appropriate error handling mechanisms into your code. The `jsonDecode` function can throw a `FormatException` if the input string is not valid JSON. It’s best practice to wrap calls to `jsonDecode` in a `try-catch` block.
import 'dart:convert';
void main() {
final jsonString = '{"name": "Bob", "age": "thirty"}'; //Invalid JSON - age should be a number
try {
final data = jsonDecode(jsonString);
print(data);
} catch (e) {
print('Error decoding JSON: $e');
}
}
This code demonstrates proper error handling, preventing application crashes due to malformed JSON. Always validate your JSON input before attempting to decode it to mitigate potential errors.
Working with HTTP Requests and Dart JSON
Many Dart applications interact with APIs over HTTP. This often involves sending and receiving JSON data. The `http` package is commonly used for making HTTP requests in Dart. Let’s illustrate how to combine HTTP requests with dart json processing.
Here is a simplified example of fetching JSON data from a remote API and parsing it using `jsonDecode`:
import 'dart:convert';
import 'package:http/http.dart' as http;
Future fetchData() async {
final response = await http.get(Uri.parse('https://jsonplaceholder.typicode.com/todos/1'));
if (response.statusCode == 200) {
final jsonResponse = jsonDecode(response.body);
print(jsonResponse);
} else {
print('Request failed with status: ${response.statusCode}.');
}
}
void main() async {
await fetchData();
}
This shows a basic structure, remembering that robust error handling and appropriate exception management should always be implemented. Remember to add the `http` package to your `pubspec.yaml` file: `dependencies: http: ^1.1.0`
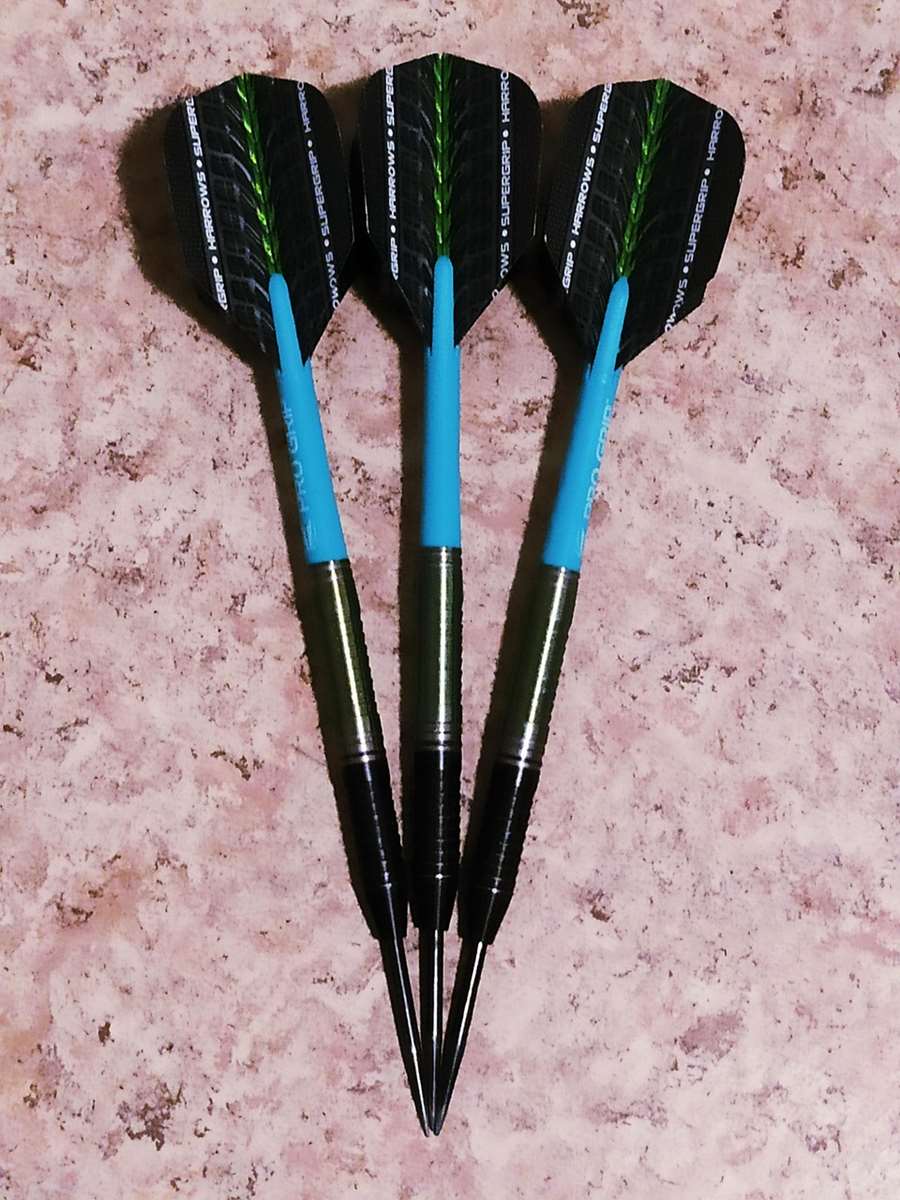
Best Practices for Dart JSON
Following best practices is crucial for writing maintainable and efficient code when working with dart json. Here are some key recommendations:
- Use custom Dart classes: Represent your JSON data using custom Dart classes to improve code readability and maintainability.
- Implement robust error handling: Wrap `jsonDecode` calls in `try-catch` blocks to handle potential `FormatException`s.
- Validate JSON input: Before decoding, validate your JSON input to ensure it’s valid and conforms to your expectations.
- Leverage packages: Use packages like `json_serializable` to automate the conversion between Dart classes and JSON.
- Avoid unnecessary nesting: Keep your JSON structures as flat as possible to improve readability and parsing efficiency.
By adhering to these best practices, you’ll create more robust and maintainable Dart applications that effectively handle JSON data. Remember to always prioritize readability and efficiency in your code.
Advanced Dart JSON Techniques
Beyond the basics, several advanced techniques can streamline your dart json workflow. One such technique involves using custom converters for more complex data types not directly supported by `jsonDecode` and `jsonEncode`. You can create custom converters to handle dates, URIs, or other non-standard types.
Another useful technique involves using code generation tools like `build_runner` with `json_serializable`. This eliminates the need to manually write the code for converting between your Dart classes and JSON, saving significant development time and reducing the risk of errors. This greatly improves the efficiency of working with dart json in more complex projects.
Consider using the is dart free to create your applications for free. For more complex data management tasks, exploring packages that enhance JSON processing can be incredibly useful.
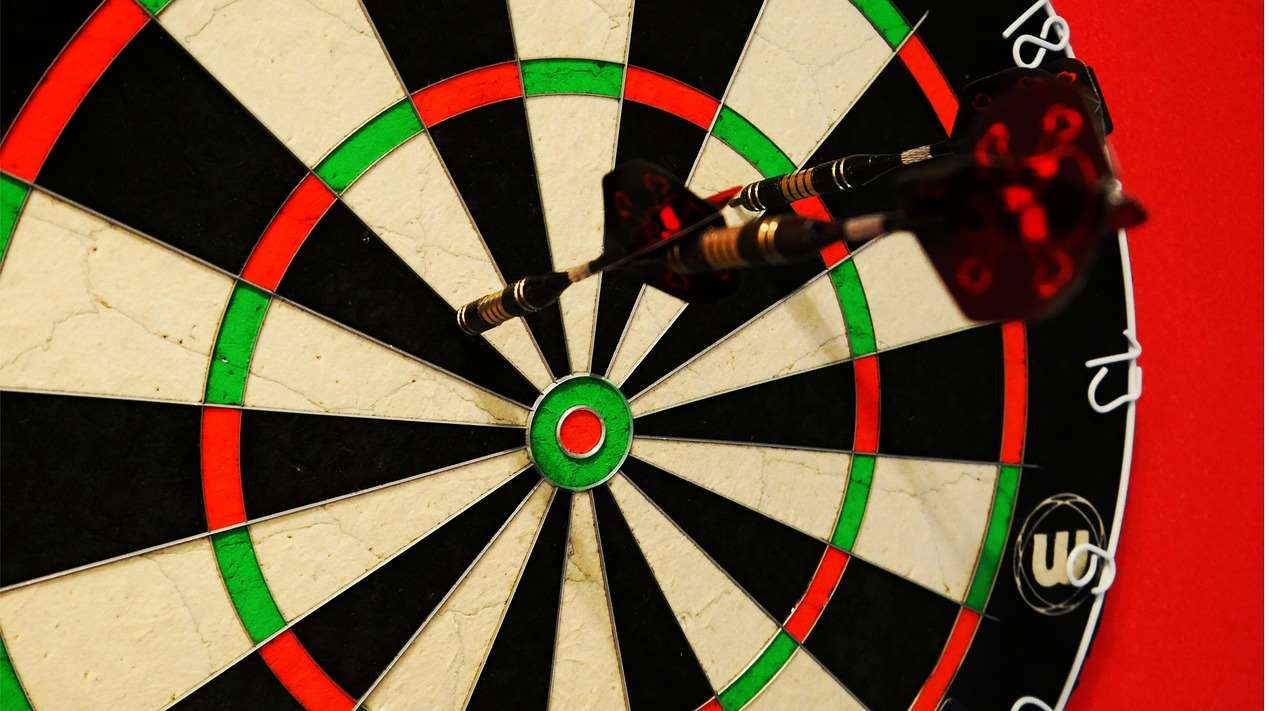
Troubleshooting Common Dart JSON Issues
Despite the simplicity of dart json, several issues may arise. Common problems include:
- Invalid JSON: Ensure your JSON string is properly formatted. Missing braces, commas, or incorrect quoting can lead to `FormatException`s.
- Type Mismatches: Verify that the data types in your JSON string match the expected types in your Dart objects. Type mismatches can result in runtime errors.
- Null values: Handle null values gracefully. Use null-aware operators (`?.` and `??`) to avoid null pointer exceptions.
- Large JSON files: For large JSON files, consider using streaming techniques to avoid memory issues.
By anticipating and addressing these potential issues proactively, you’ll significantly enhance the robustness of your applications.
Conclusion
Efficiently managing dart json is a critical skill for any Dart developer. From encoding and decoding simple data structures to handling complex nested JSON and integrating with HTTP APIs, a solid understanding of dart json is essential for creating robust, scalable applications. Remember to leverage best practices, such as using custom Dart classes and implementing robust error handling, to ensure maintainability and efficiency. You can even improve your dart json interactions with the Digital dart score app.
This comprehensive guide has equipped you with the knowledge to tackle various dart json challenges. Now, it’s time to put your newfound skills to the test! Start building your next amazing Dart application, leveraging the power of dart json for seamless data handling. Learn more about darts score to see other applications of JSON.
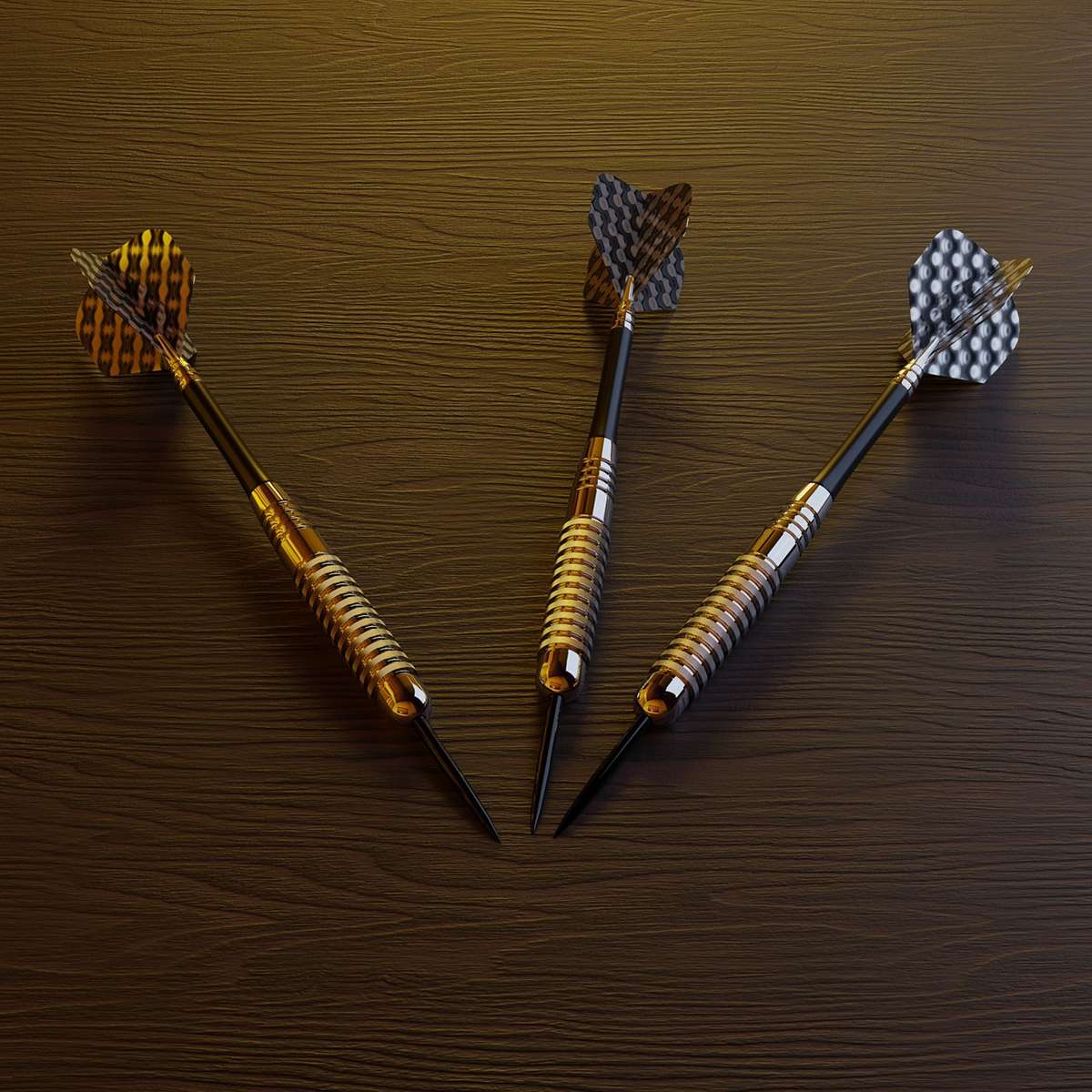
Hi, I’m Dieter, and I created Dartcounter (Dartcounterapp.com). My motivation wasn’t being a darts expert – quite the opposite! When I first started playing, I loved the game but found keeping accurate scores and tracking stats difficult and distracting.
I figured I couldn’t be the only one struggling with this. So, I decided to build a solution: an easy-to-use application that everyone, no matter their experience level, could use to manage scoring effortlessly.
My goal for Dartcounter was simple: let the app handle the numbers – the scoring, the averages, the stats, even checkout suggestions – so players could focus purely on their throw and enjoying the game. It began as a way to solve my own beginner’s problem, and I’m thrilled it has grown into a helpful tool for the wider darts community.