Understanding how to effectively use ‘dart copywith set null‘ is crucial for managing state in your Flutter applications. This article will explain precisely how to leverage this functionality and explore related concepts, ultimately helping you write cleaner, more maintainable Dart code.
⚠️ Still Using Pen & Paper (or a Chalkboard)?! ⚠️
Step into the future! The Dart Counter App handles all the scoring, suggests checkouts, and tracks your stats automatically. It's easier than you think!
Try the Smart Dart Counter App FREE!Ready for an upgrade? Click above!
The power of copywith in Dart lies in its ability to create a new instance of a class, copying the values of existing fields while allowing you to selectively modify specific properties. When you need to update parts of an object without affecting the original, dart copywith set null provides an elegant solution. This article will explore various scenarios where this technique proves invaluable, including handling complex data structures and managing state efficiently in your Flutter applications.
Understanding Dart CopyWith and Null Values
The copyWith method is a common pattern in Dart, particularly when using immutable data structures. It allows you to create a new object that’s essentially a copy of an existing one, but with one or more fields changed. Often, you might want to explicitly set a field to null. This is where mastering dart copywith set null becomes essential.
Imagine you have a Player
class with properties like name
, score
, and average
. Using copyWith
, you can easily create a new Player
object with an updated score, leaving other properties untouched. Setting a property to null, using dart copywith set null, might signify the absence of a value or a deliberate reset.
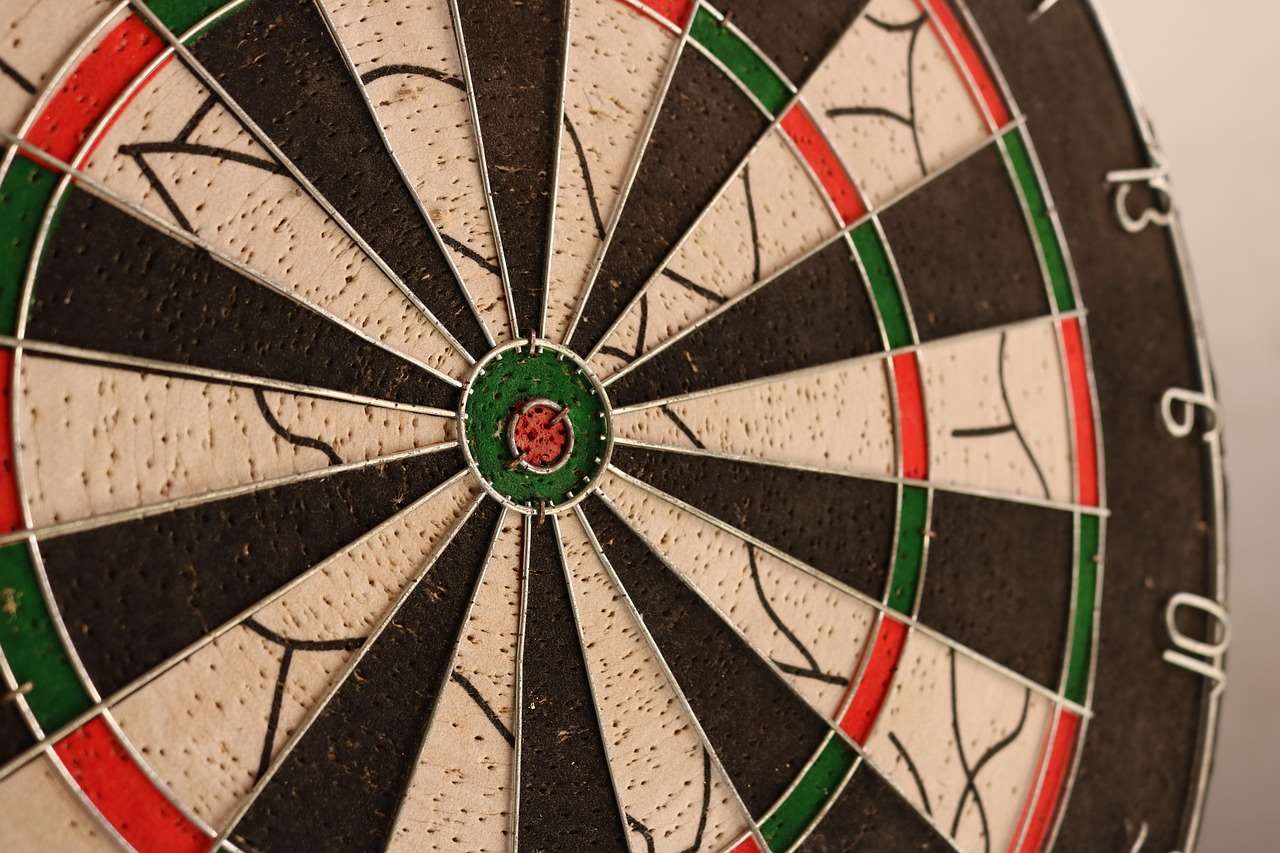
Practical Implementation of dart copywith set null
Let’s illustrate with a simple example:
class Player {
final String name;
final int? score; // Notice the nullable score
final double? average; //And a nullable average
Player({required this.name, this.score, this.average});
Player copyWith({String? name, int? score, double? average}) {
return Player(
name: name ?? this.name,
score: score, //Explicitly setting score to null is allowed
average: average, // same here
);
}
}
void main() {
var player1 = Player(name: 'John Doe', score: 150, average: 50.0);
var player2 = player1.copyWith(score: null); //Setting score to null using dart copywith set null
print(player1.score); // Output: 150
print(player2.score); // Output: null
}
This code demonstrates the core principle of dart copywith set null. The copyWith
method allows us to create player2
, which is a copy of player1
but with the score
explicitly set to null. The use of the null-aware operator (??
) ensures that if a parameter is not provided in the copyWith
call, the existing value is retained.
Advanced Scenarios with dart copywith set null
The power of dart copywith set null extends beyond simple data types. Let’s consider more complex scenarios:
Nested Objects
Suppose your Player
class contains a nested object, like a Dartboard
:
class Dartboard {
final String material;
Dartboard({required this.material});
Dartboard copyWith({String? material}) {
return Dartboard(material: material ?? this.material);
}
}
class Player {
final String name;
final Dartboard? dartboard; // Dartboard is nullable.
Player({required this.name, this.dartboard});
Player copyWith({String? name, Dartboard? dartboard}) {
return Player(
name: name ?? this.name,
dartboard: dartboard,
);
}
}
Here, you can use dart copywith set null to remove the dartboard
entirely by setting it to null in the copyWith
method. This allows for flexible state management.
Lists and Maps
Managing lists and maps within your classes adds another layer of complexity. However, dart copywith set null still offers a clean way to handle updates and null assignments. You can often copy the existing list/map and modify it directly, or create entirely new ones as needed, effectively allowing dart copywith set null for elements within the collection.
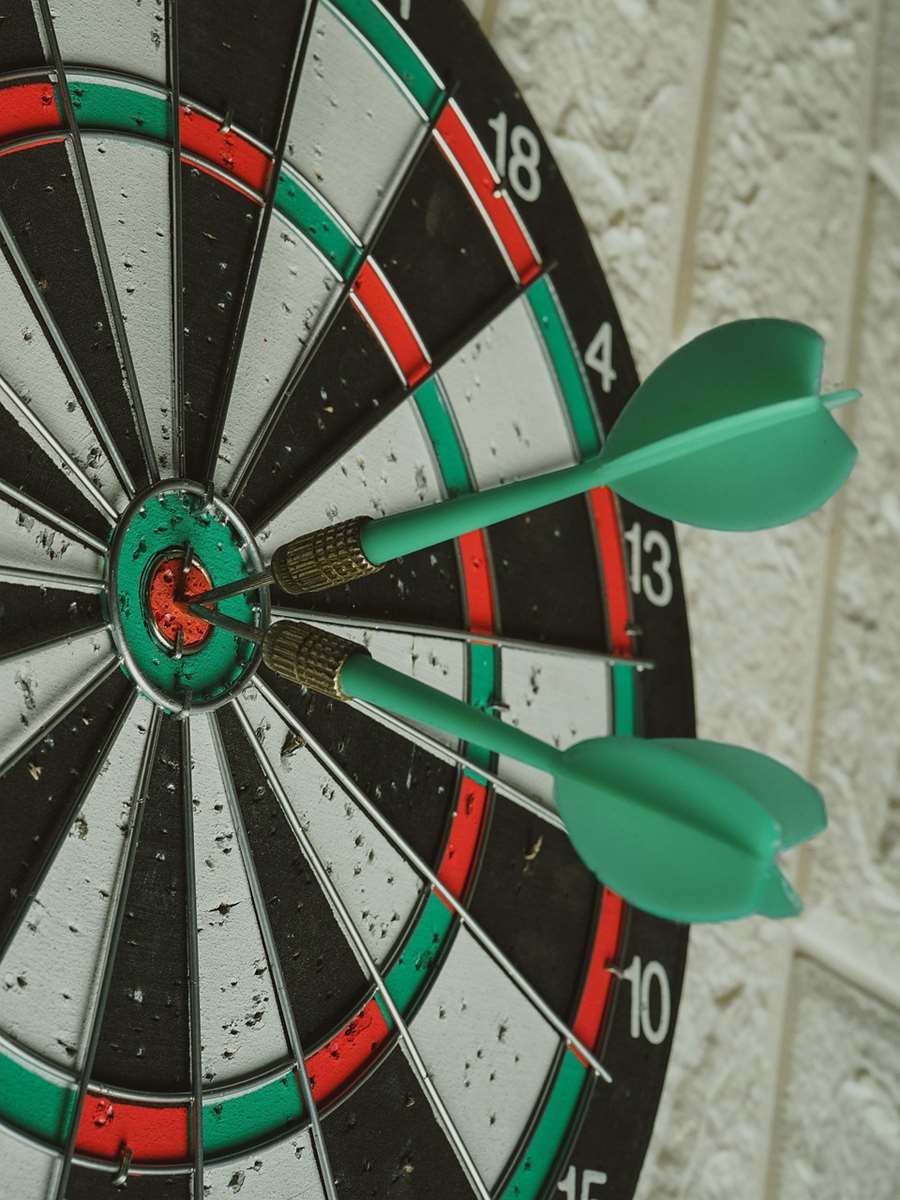
Best Practices When Using dart copywith set null
While dart copywith set null offers great flexibility, following best practices ensures clean and maintainable code:
- Use Null Safety: Leveraging Dart’s null safety features (
?
for nullable types) helps prevent unexpected null pointer exceptions. This is especially important when using dart copywith set null, ensuring that null values are handled gracefully. - Immutability: Embrace immutability whenever possible.
copyWith
promotes immutability by creating new objects instead of modifying existing ones. This reduces the risk of unexpected side effects in your application. - Thorough Testing: Always test your
copyWith
methods rigorously to catch potential errors related to null values or unexpected behavior when updating specific fields. - Consistency: Maintain consistency in how you handle null values across your classes. This improves code readability and maintainability. A consistent approach to dart copywith set null enhances the predictability of your code.
Troubleshooting Common Issues
Even with careful implementation, you might encounter issues. Here are a few common problems and solutions:
- Unexpected null values: Carefully review the logic in your
copyWith
method to ensure that null values are being handled correctly and according to your intentions. Using the null-aware operator (??
) helps prevent the accidental propagation of null values where they are not desired. - Complex object graphs: For complex objects, consider using libraries that can simplify the process of creating
copyWith
methods, ensuring efficient handling of null values even with deeply nested structures.
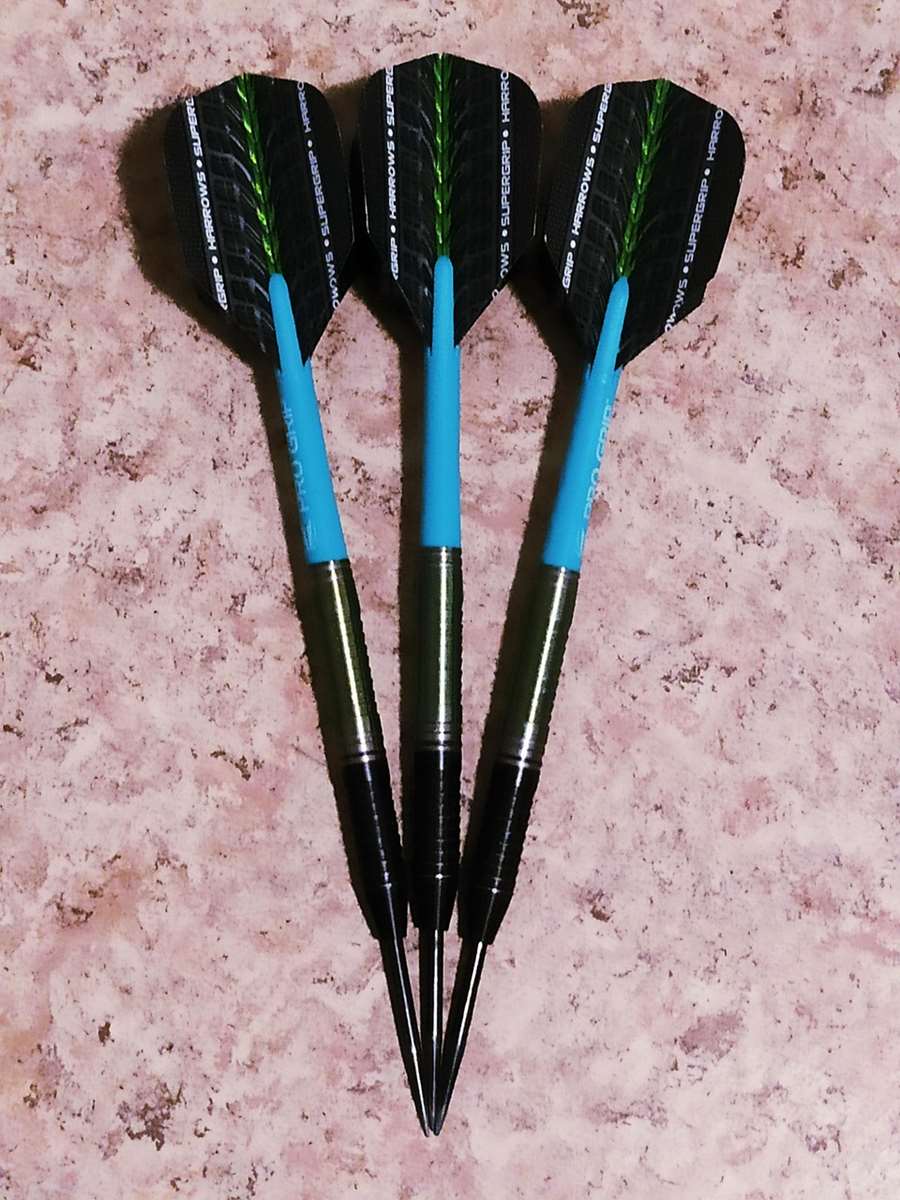
Alternative Approaches: When copyWith isn’t the best solution
While copyWith
is a powerful tool, there might be scenarios where other approaches are more appropriate. Consider these alternatives:
- Direct object creation: In cases with few fields to modify, creating a new object directly can be simpler than implementing a
copyWith
method. - Builder pattern: For classes with many fields, the Builder pattern offers a structured way to create objects, providing fine-grained control over the construction process.
Choosing the right method depends on the complexity of your class and the specific needs of your application. If you are dealing with simple classes and primarily focused on setting fields to null selectively, dart copywith set null remains an excellent choice.
Integrating dart copywith set null into your Flutter projects
The techniques discussed above are directly applicable within your Flutter projects. Efficient state management is crucial for Flutter applications, and leveraging dart copywith set null to create immutable copies of your data models helps to improve the predictability and maintainability of your codebase. Remember to always prioritize clean and efficient code practices when working with state management in Flutter, especially when handling null values.
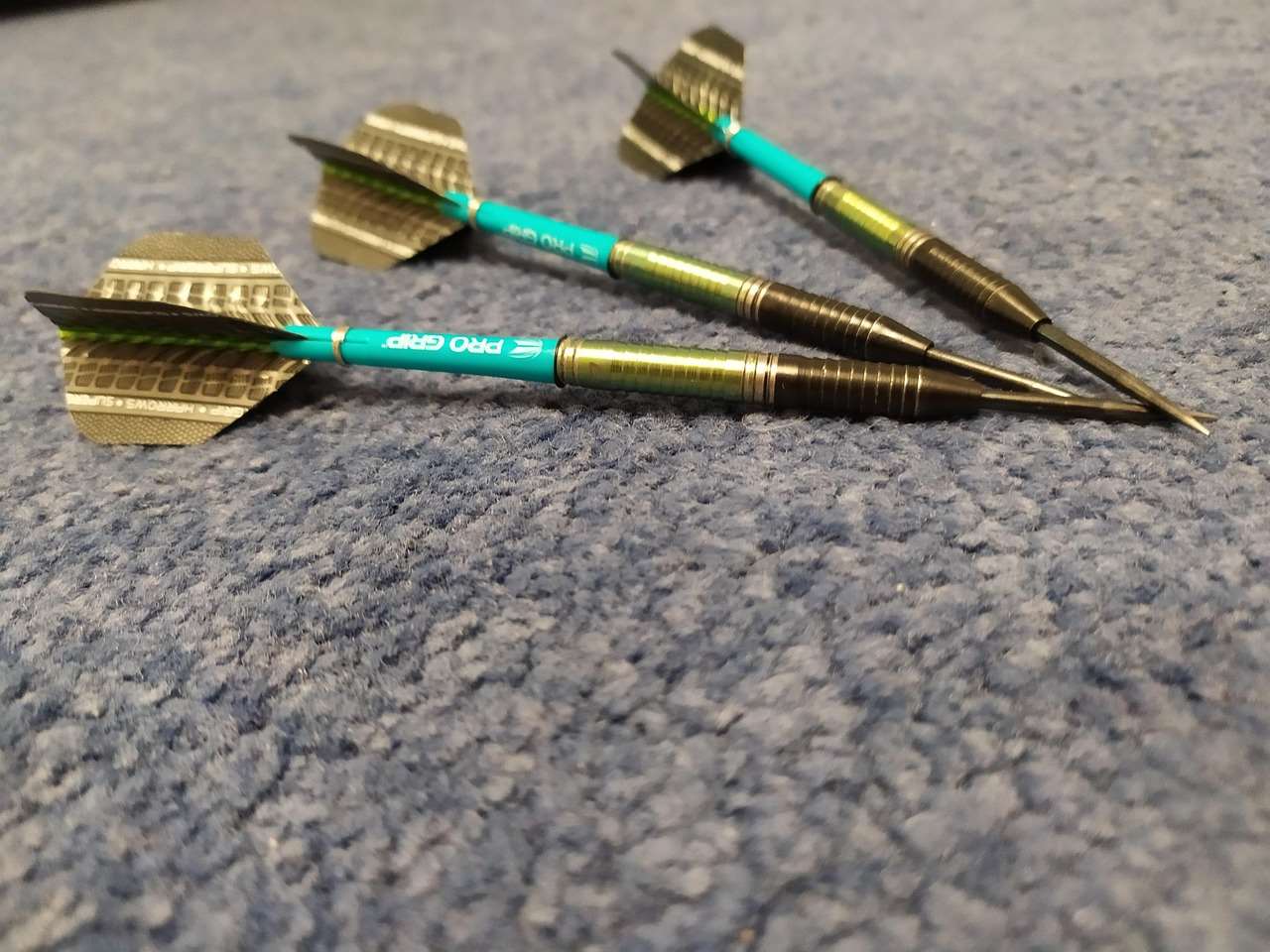
By mastering the art of dart copywith set null, you’ll significantly improve the quality and maintainability of your Dart code. Remember that careful planning and testing are vital when using this powerful feature, particularly when dealing with nested objects or complex data structures. This ensures that null values are handled correctly and according to your intended logic. A well-structured approach, combined with proper testing, eliminates ambiguity and unexpected behavior from your application. For more information on efficient Dart development, consider checking out resources like the official Dart website.
Remember to check out our helpful guides on darts player the count and darts woman for more insights into the exciting world of darts. If you encounter any issues with your darts counter not working, we’ve got solutions for that too! Learn about the dartboard numbers sequence to improve your game and explore the different individual darts game formats.
Conclusion
Utilizing dart copywith set null effectively streamlines the process of updating data structures in your Dart applications. By understanding its mechanics, implementing best practices, and troubleshooting potential issues, you gain a valuable tool for writing cleaner, more maintainable code. Remember to consider alternative approaches when necessary and to thoroughly test your implementation. This will lead to more robust and error-free applications. Mastering dart copywith set null will significantly improve your ability to manage complex state in your Flutter projects. Remember to explore our resources, such as the article on darts masters final time for more engaging content. For more advanced players, learn about target darts pixel grip, and if you need to improve your setup, consult our guide on foldable darts oche.
Need help with your dart game scoring? Check out our recommended Electronic dart score counter today!

Hi, I’m Dieter, and I created Dartcounter (Dartcounterapp.com). My motivation wasn’t being a darts expert – quite the opposite! When I first started playing, I loved the game but found keeping accurate scores and tracking stats difficult and distracting.
I figured I couldn’t be the only one struggling with this. So, I decided to build a solution: an easy-to-use application that everyone, no matter their experience level, could use to manage scoring effortlessly.
My goal for Dartcounter was simple: let the app handle the numbers – the scoring, the averages, the stats, even checkout suggestions – so players could focus purely on their throw and enjoying the game. It began as a way to solve my own beginner’s problem, and I’m thrilled it has grown into a helpful tool for the wider darts community.