Achieving consistent Dart development across teams and projects hinges on customizing Dart setup for consistency. This article explores how to standardize your Dart environment, manage dependencies effectively, and enforce coding style guides to promote collaboration and code maintainability. You’ll learn best practices for Dart SDK management, package management with pub, and code formatting with dartfmt, leading to a more predictable and efficient development workflow.
Still Using Pen & Paper (or a Chalkboard)?! 
Step into the future! The Dart Counter App handles all the scoring, suggests checkouts, and tracks your stats automatically. It's easier than you think!
Try the Smart Dart Counter App FREE!Ready for an upgrade? Click above!
The Importance of Customizing Dart Setup For Consistency
Customizing Dart setup for consistency is not just a nice-to-have; it’s a critical factor in ensuring project success, especially in larger teams or organizations. Without a standardized environment, developers can spend unnecessary time troubleshooting environment-specific issues, resolving dependency conflicts, and debating coding styles. This wasted time translates to increased development costs, slower project timelines, and a higher risk of errors.
Consider the scenario where one developer is using an outdated version of the Dart SDK, while another is using a beta version. This discrepancy can lead to code that works perfectly for one developer but crashes for the other. Similarly, inconsistent formatting can make code reviews a nightmare and introduce unnecessary merge conflicts. By establishing a consistent Dart setup, you eliminate these potential pitfalls and create a smoother, more efficient development process.
Furthermore, a consistent environment simplifies onboarding new team members. Instead of spending days configuring their development environment, new developers can quickly get up to speed with a pre-configured setup, allowing them to contribute to the project sooner. This contributes significantly to improved team productivity and morale.
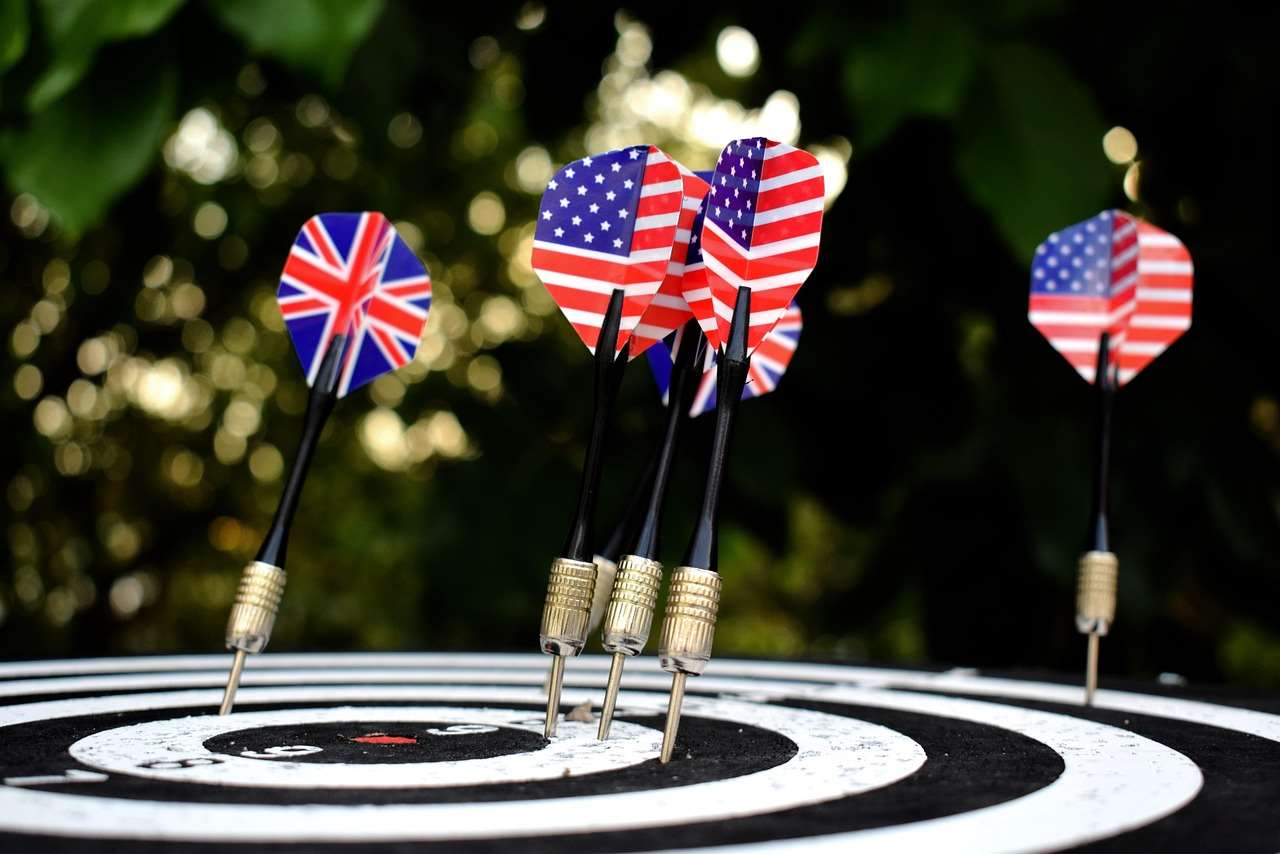
Managing the Dart SDK for Uniformity
The Dart SDK (Software Development Kit) is the foundation of any Dart development environment. Ensuring that all developers are using the same SDK version is paramount for consistency. Several tools and approaches can help achieve this:
Using fvm
(Flutter Version Management)
While primarily designed for Flutter, fvm
is also an excellent tool for managing Dart SDK versions. It allows you to specify the Dart SDK version on a per-project basis, ensuring that everyone working on the project is using the same version, regardless of their global Dart SDK installation. You can learn more about how specific dart equipment can help in your workflows by looking at resources to Choose Best Dart Equipment.
To install fvm
, run:
dart pub global activate fvm
Then, in your project directory, specify the Dart SDK version:
fvm install <dart-sdk-version>
For example:
fvm install 3.0.0
To use the specified SDK version, prefix your Dart commands with fvm dart
:
fvm dart --version
This ensures that the correct SDK is used for the project, even if a different version is installed globally.
Using asdf
Version Manager
asdf
is a versatile version manager that supports multiple languages and tools, including Dart. It allows you to define the Dart SDK version in a .tool-versions
file within your project. This file is automatically read by asdf
, ensuring that the correct SDK version is used.
After installing asdf
and the Dart plugin, you can specify the Dart SDK version by creating a .tool-versions
file in your project’s root directory:
dart <dart-sdk-version>
For example:
dart 3.0.0
Then, run asdf install
to install the specified SDK version.
Environment Variables
While less automated, setting the DART_SDK
environment variable can also help ensure consistency. However, this requires developers to manually configure their environment correctly. Setting environment variables can also help you avoid investing in premium dart equipment while optimizing your development workspace.
Standardizing Package Management with pub
Dart’s package manager, pub
, is essential for managing project dependencies. However, simply using pub get
or pub upgrade
without any constraints can lead to inconsistent dependency versions across different environments. This can result in unexpected behavior and compatibility issues.
Using pubspec.yaml
Effectively
The pubspec.yaml
file is the cornerstone of Dart package management. It defines your project’s dependencies and their version constraints. Use version constraints wisely to balance stability and access to the latest features. For example, instead of specifying an exact version like 1.0.0
, use a constraint like ^1.0.0
. This allows pub
to use the latest version within the 1.x.x range, ensuring that you get bug fixes and minor updates while maintaining compatibility.
Using pubspec.lock
for Reproducible Builds
The pubspec.lock
file is automatically generated by pub get
or pub upgrade
. It records the exact versions of all dependencies and their transitive dependencies. Including the pubspec.lock
file in your version control system (e.g., Git) is crucial for ensuring reproducible builds. When other developers run pub get
, pub
will use the versions specified in the pubspec.lock
file, guaranteeing that everyone is using the same dependencies.
Remember to always commit the pubspec.lock
file along with your code changes.
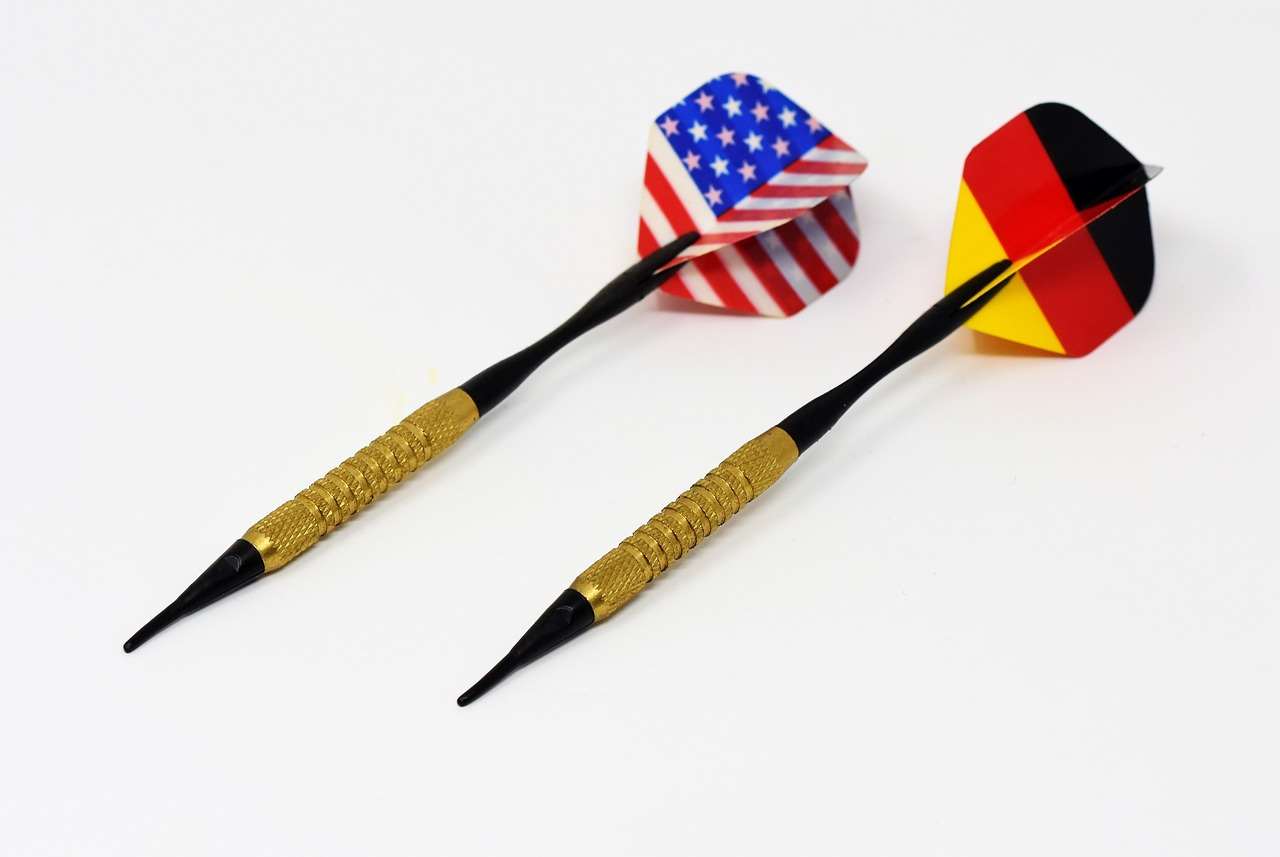
Dependency Overrides
In some cases, you may need to override a dependency version for a specific reason, such as fixing a bug or using a custom version of a package. You can use the dependency_overrides
section in your pubspec.yaml
file to achieve this. However, use dependency overrides with caution, as they can introduce compatibility issues and make your project more difficult to maintain.
Enforcing Coding Style with dartfmt
and Linter Rules
Consistent code formatting and coding style are essential for code readability and maintainability. Dart provides excellent tools for automating these tasks.
Using dartfmt
for Automatic Formatting
dartfmt
is Dart’s official code formatter. It automatically formats your Dart code according to a set of predefined rules. Using dartfmt
ensures that all code in your project has a consistent look and feel, regardless of who wrote it.
To format your code, simply run:
dart format .
This will format all Dart files in the current directory and its subdirectories. It might even help to improve the Quality Comparison Budget Premium Darts of your current project.
Configuring Linter Rules with analysis_options.yaml
Dart’s analyzer can be configured to enforce a wide range of coding style rules and best practices. The configuration is done through the analysis_options.yaml
file. This file allows you to enable or disable specific linter rules, customize their severity, and even define your own custom rules.
Here’s an example of an analysis_options.yaml
file:
include: package:lints/recommended.yaml
linter:
rules:
always_put_required_named_parameters_first: true
avoid_print: false
prefer_const_constructors: true
This configuration includes the recommended linter rules from the lints
package and enables a few additional rules. The always_put_required_named_parameters_first
rule enforces a specific order for named parameters, while the avoid_print
rule discourages the use of print
statements in production code. The Investing In Premium Dart Equipment for your code formatting process can improve efficiency.
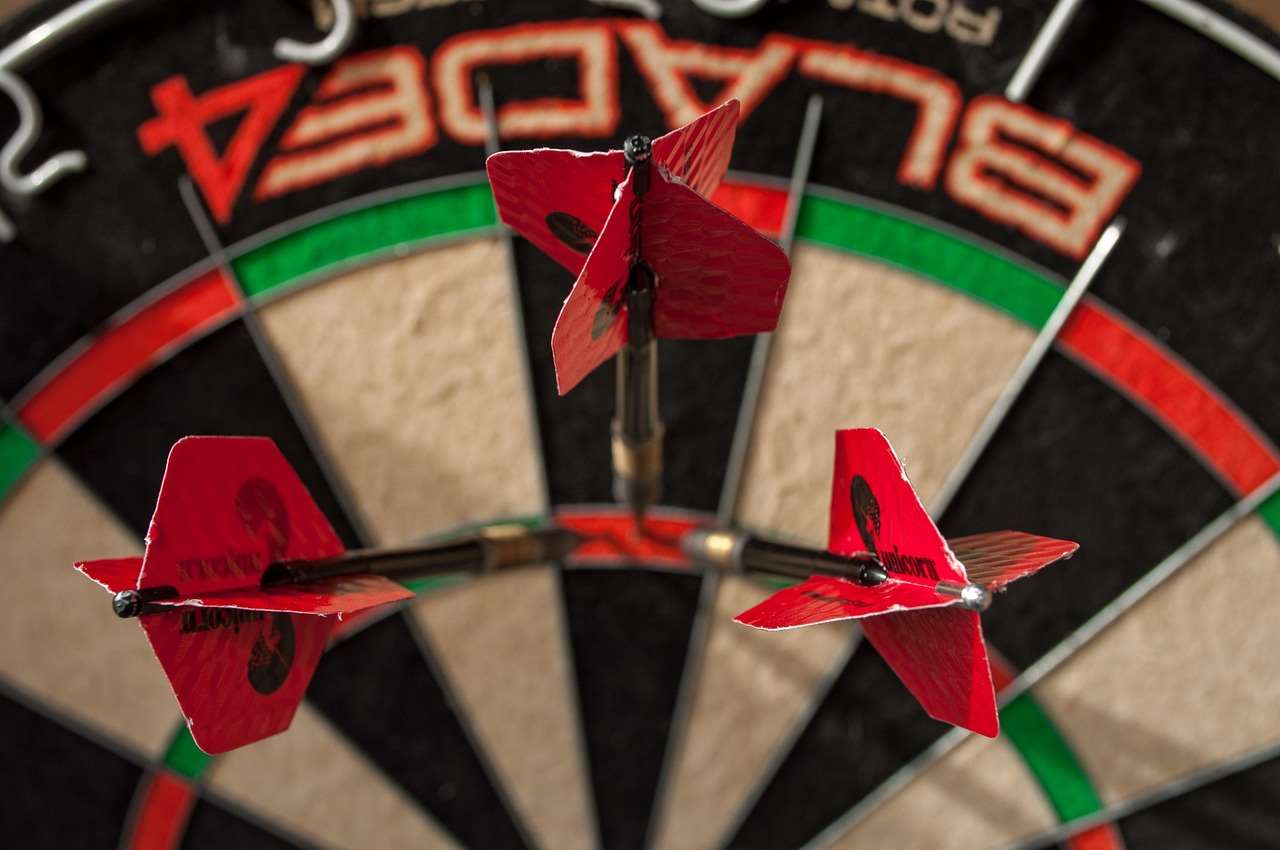
Integrating with CI/CD
To ensure that code formatting and linter rules are consistently enforced, integrate dartfmt
and the Dart analyzer into your CI/CD pipeline. This will automatically check your code for formatting and style violations before it’s merged into the main branch. If any violations are found, the CI/CD pipeline can fail the build, preventing the code from being deployed.
Best Practices for Collaboration
Customizing Dart setup for consistency is a collaborative effort. Here are some best practices for ensuring that everyone on your team is on board:
- Document your setup: Create a document that describes your team’s Dart development environment, including the SDK version, package management approach, and coding style guidelines. Make this document easily accessible to all team members.
- Use a template project: Create a template project that includes all the necessary configuration files (
pubspec.yaml
,pubspec.lock
,analysis_options.yaml
) and a basic project structure. This will help new team members get started quickly and ensure that they are using the correct setup from the beginning. - Conduct code reviews: Code reviews are an excellent opportunity to enforce coding style guidelines and identify potential inconsistencies. Encourage team members to actively participate in code reviews and provide constructive feedback.
- Automate: Automate as much as possible, including SDK management, package management, code formatting, and linting. This will reduce the risk of human error and ensure that consistency is maintained throughout the development process.
Advanced Customization Techniques
Beyond the basics, you can further customize your Dart setup to meet specific project needs. This might involve creating custom linter rules, developing code generation tools, or integrating with other development tools. Investing in premium dart equipment can help you refine your customization process and optimize performance.
Custom Linter Rules
If the standard linter rules don’t meet your needs, you can create your own custom rules using Dart’s analyzer plugin API. This allows you to enforce project-specific coding conventions and best practices.
Code Generation
Code generation can be a powerful tool for reducing boilerplate code and ensuring consistency. Dart provides several code generation tools, such as build_runner
, which allows you to automatically generate code based on annotations or other metadata.
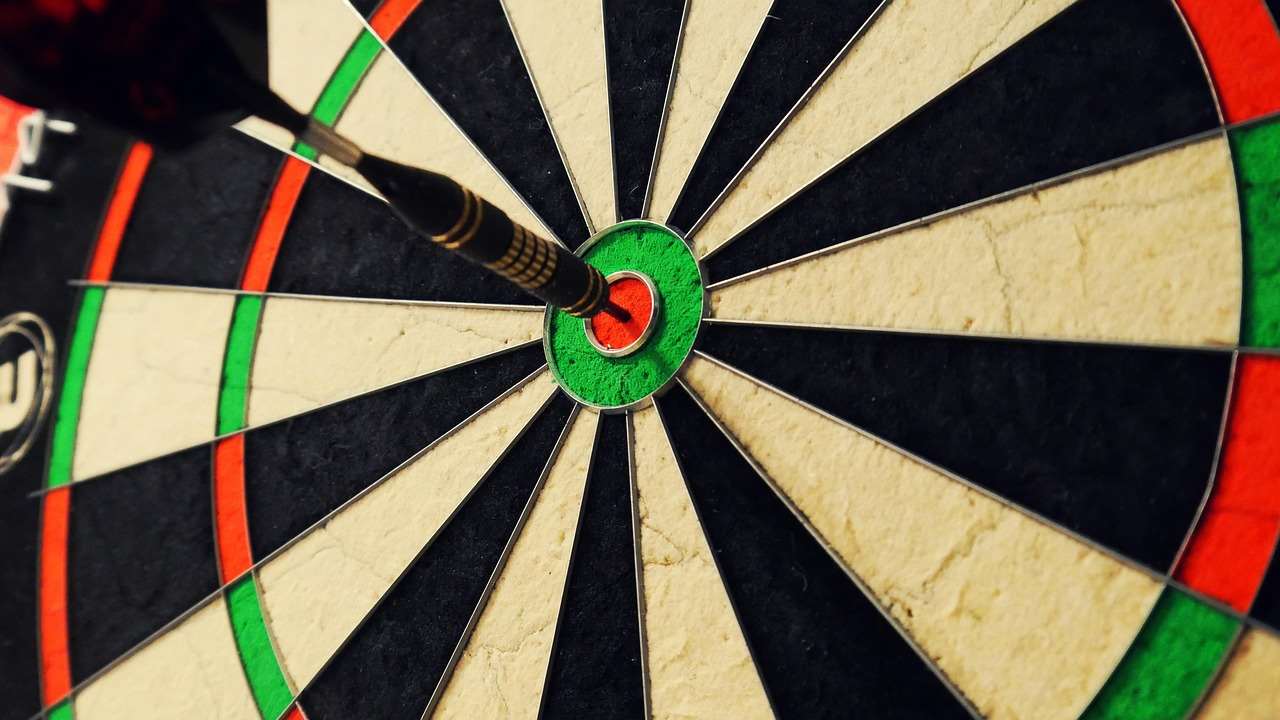
Integrating with Other Tools
Dart can be easily integrated with other development tools, such as IDEs, build systems, and testing frameworks. This allows you to create a seamless and efficient development workflow. For example, you can configure your IDE to automatically format your code on save or run the Dart analyzer on every build.
Troubleshooting Common Issues
Even with a well-defined setup, you may still encounter issues related to Dart environment consistency. Here are some common problems and their solutions:
- Dependency conflicts: Dependency conflicts can occur when two or more packages depend on different versions of the same package. To resolve dependency conflicts, try using
pub upgrade --major-versions
to update your dependencies to the latest compatible versions. If that doesn’t work, you may need to use dependency overrides to force a specific version of the conflicting package. - SDK compatibility issues: If you’re using an outdated Dart SDK, you may encounter compatibility issues with newer packages or language features. To resolve this, upgrade your Dart SDK to the latest stable version.
- Formatting inconsistencies: Formatting inconsistencies can occur if developers are not using the same version of
dartfmt
or if their IDEs are not configured to usedartfmt
automatically. To resolve this, ensure that all developers are using the same version ofdartfmt
and that their IDEs are properly configured.
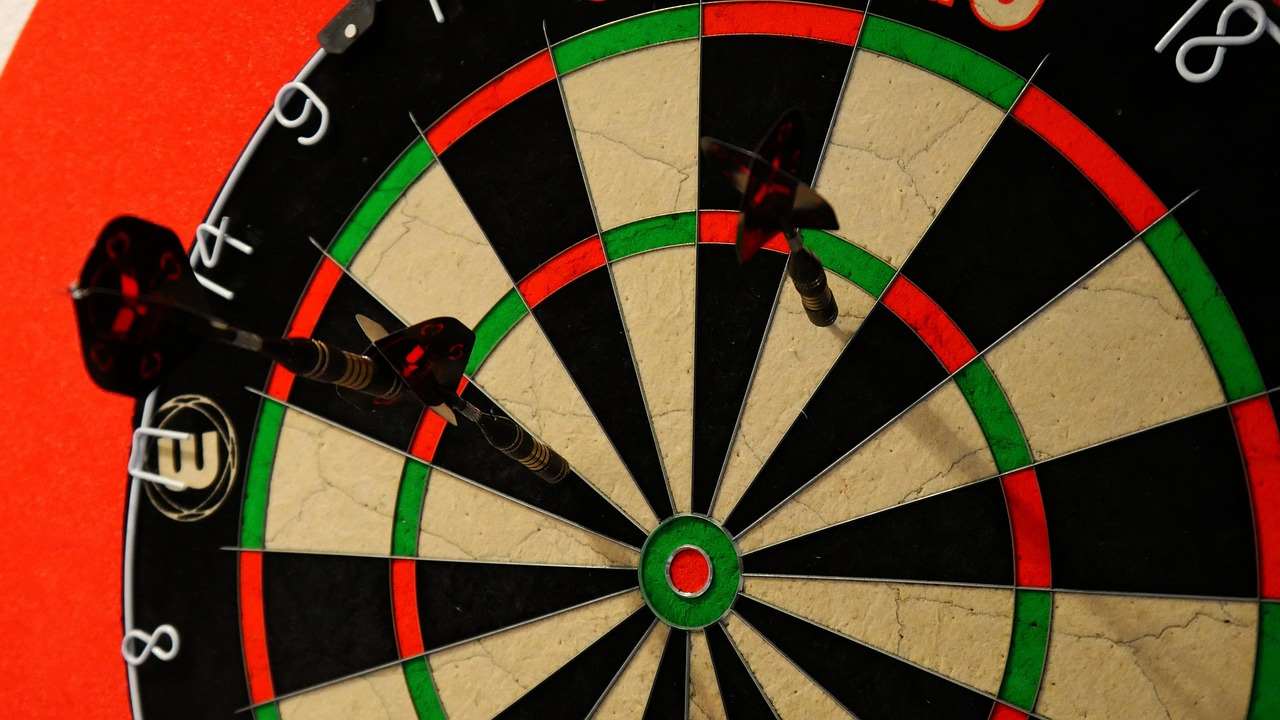
Conclusion
Customizing Dart setup for consistency is a crucial investment that pays off in increased productivity, reduced errors, and improved code maintainability. By standardizing your Dart SDK management, package management, and coding style, you can create a more predictable and efficient development workflow. Remember to document your setup, use a template project, conduct code reviews, and automate as much as possible. Embrace the tools and techniques discussed in this article to unlock the full potential of Dart and build high-quality applications more effectively. Consider comparing Budget vs Premium Darts Compared to see where your own project falls on the spectrum. Start implementing these practices today to streamline your Dart development and achieve greater consistency across your projects. Take the first step now and review your current Dart setup to identify areas for improvement and implement the necessary changes.
Hi, I’m Dieter, and I created Dartcounter (Dartcounterapp.com). My motivation wasn’t being a darts expert – quite the opposite! When I first started playing, I loved the game but found keeping accurate scores and tracking stats difficult and distracting.
I figured I couldn’t be the only one struggling with this. So, I decided to build a solution: an easy-to-use application that everyone, no matter their experience level, could use to manage scoring effortlessly.
My goal for Dartcounter was simple: let the app handle the numbers – the scoring, the averages, the stats, even checkout suggestions – so players could focus purely on their throw and enjoying the game. It began as a way to solve my own beginner’s problem, and I’m thrilled it has grown into a helpful tool for the wider darts community.