In Dart, efficiently counting characters within a string is straightforward using the built-in length
property. This article will show you exactly how to dart count characters in string and explore various related techniques for string manipulation in Dart, providing you with a comprehensive understanding of character counting and its applications.
⚠️ Still Using Pen & Paper (or a Chalkboard)?! ⚠️
Step into the future! The Dart Counter App handles all the scoring, suggests checkouts, and tracks your stats automatically. It's easier than you think!
Try the Smart Dart Counter App FREE!Ready for an upgrade? Click above!
Understanding how to dart count characters in string is a fundamental skill for any Dart developer. This seemingly simple task forms the basis of more complex string operations and data analysis. This guide will delve into the core method, examine edge cases, and offer practical examples to solidify your understanding. We’ll also explore related concepts like handling Unicode characters and working with different character encoding schemes.
Dart Count Characters in String: The Basic Approach
The most direct way to dart count characters in string in Dart involves leveraging the length
property. This property directly returns the number of characters in a string. For example:
void main() {
String myString = "Hello, world!";
int charCount = myString.length;
print("The string '$myString' has $charCount characters.");
}
This concise code snippet demonstrates the simplicity of obtaining character counts. The output will be: “The string ‘Hello, world!’ has 13 characters.”
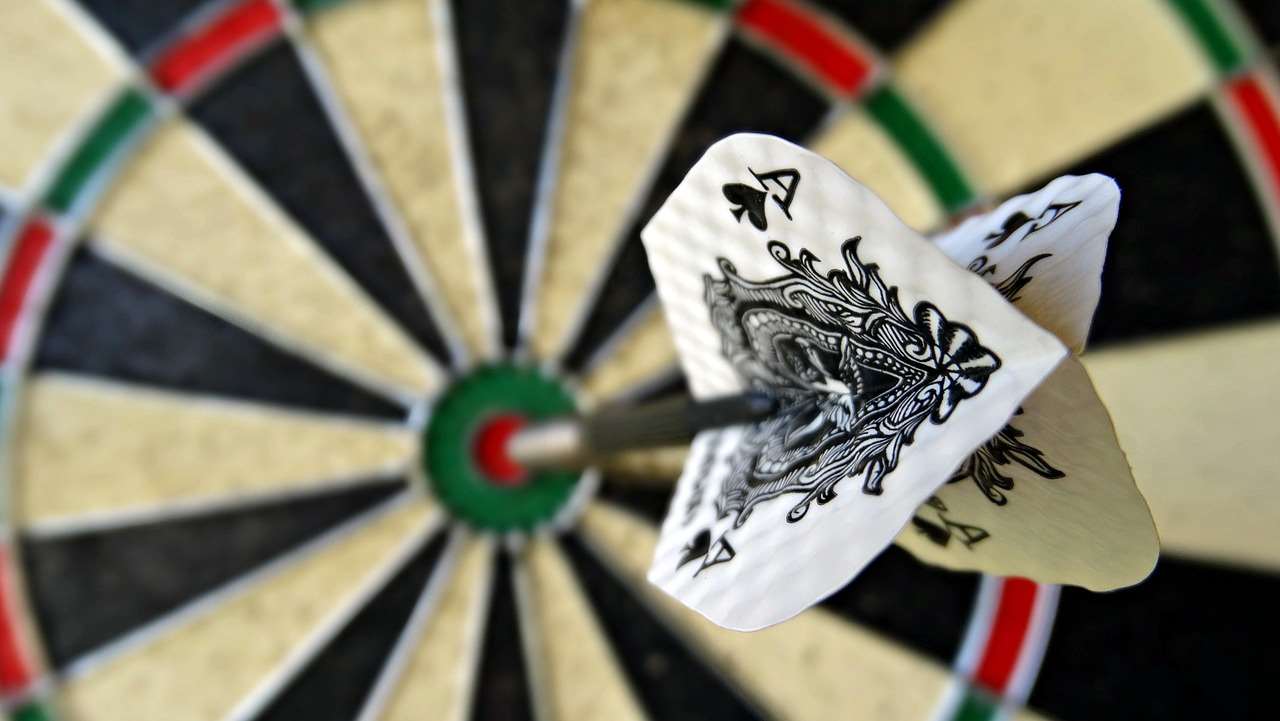
Handling Unicode Characters
While the length
property works seamlessly for basic ASCII characters, dealing with Unicode characters requires a slightly more nuanced approach. Unicode characters, which represent a broader range of characters across various languages and scripts, can sometimes have a different representation in terms of code units. This can lead to inconsistencies in counting if you’re not aware of the encoding scheme in play. The length
property will accurately reflect the number of Unicode code units but not necessarily the number of “characters” as perceived by a human. For more accurate handling of Unicode character count, consider using packages like characters.
Advanced String Manipulation Techniques
Beyond simply counting characters, Dart provides a rich set of tools for manipulating strings. Understanding these techniques allows for more sophisticated data processing and analysis. Let’s look at some relevant examples:
Substrings and Character Extraction
Dart allows you to extract specific parts of a string using the substring
method. This method takes two arguments: the starting index and the ending index (exclusive). This capability is crucial when you need to work with portions of strings based on character positions. For example:
void main() {
String myString = "This is a sample string.";
String subString = myString.substring(10, 15); // Extracts "sample"
print(subString); // Output: sample
}
This approach is useful when you need to isolate parts of a string for further processing, analysis, or display.
Character Replacement
The replaceAll
method allows replacing all occurrences of a specific substring with another. This is invaluable for data cleaning, standardization, or text transformation. For example, to replace all spaces with underscores, you could do this:
void main() {
String myString = "This is a sample string.";
String replacedString = myString.replaceAll(" ", "_");
print(replacedString); // Output: This_is_a_sample_string.
}
This method ensures consistent formatting or prepares data for specific systems or applications.
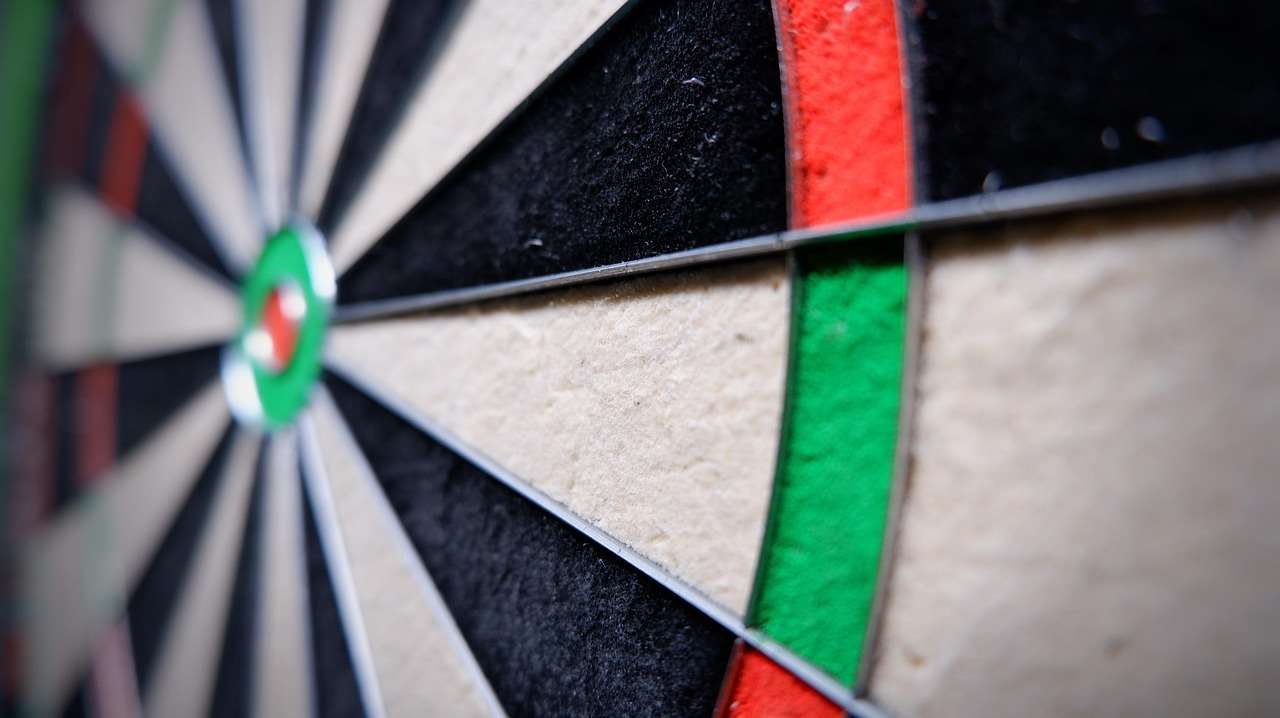
Error Handling and Edge Cases
While the basic approach to dart count characters in string is straightforward, it’s important to consider potential edge cases and handle them gracefully. For example, you should always validate the input string to ensure it’s not null before attempting to access its length
property. Failing to do so may result in a runtime exception. Always write robust code by using null checks, such as:
void main() {
String? myString; // String can be null
int charCount = myString?.length ?? 0; // Safe null-aware operator and default value
print("The string has $charCount characters.");
}
This code safely handles potential null values and prevents unexpected crashes.
Practical Applications of Dart Count Characters in String
Counting characters in strings has a wide range of applications in Dart development, extending beyond simple string length checks. Here are a few examples:
- Input Validation: Enforcing maximum length constraints on user input fields.
- Data Sanitization: Removing or truncating excessive characters to prevent overflows or data corruption.
- Text Formatting: Calculating the required space for text rendering or layout.
- Data Analysis: Analyzing textual data by counting character occurrences (e.g., frequency analysis).
- Game Development: Checking the length of player inputs for games that have length restrictions.
These examples illustrate how seemingly basic string manipulation can be a building block for more sophisticated functionalities. Knowing how to efficiently dart count characters in string and manipulate strings forms a cornerstone of effective Dart programming.
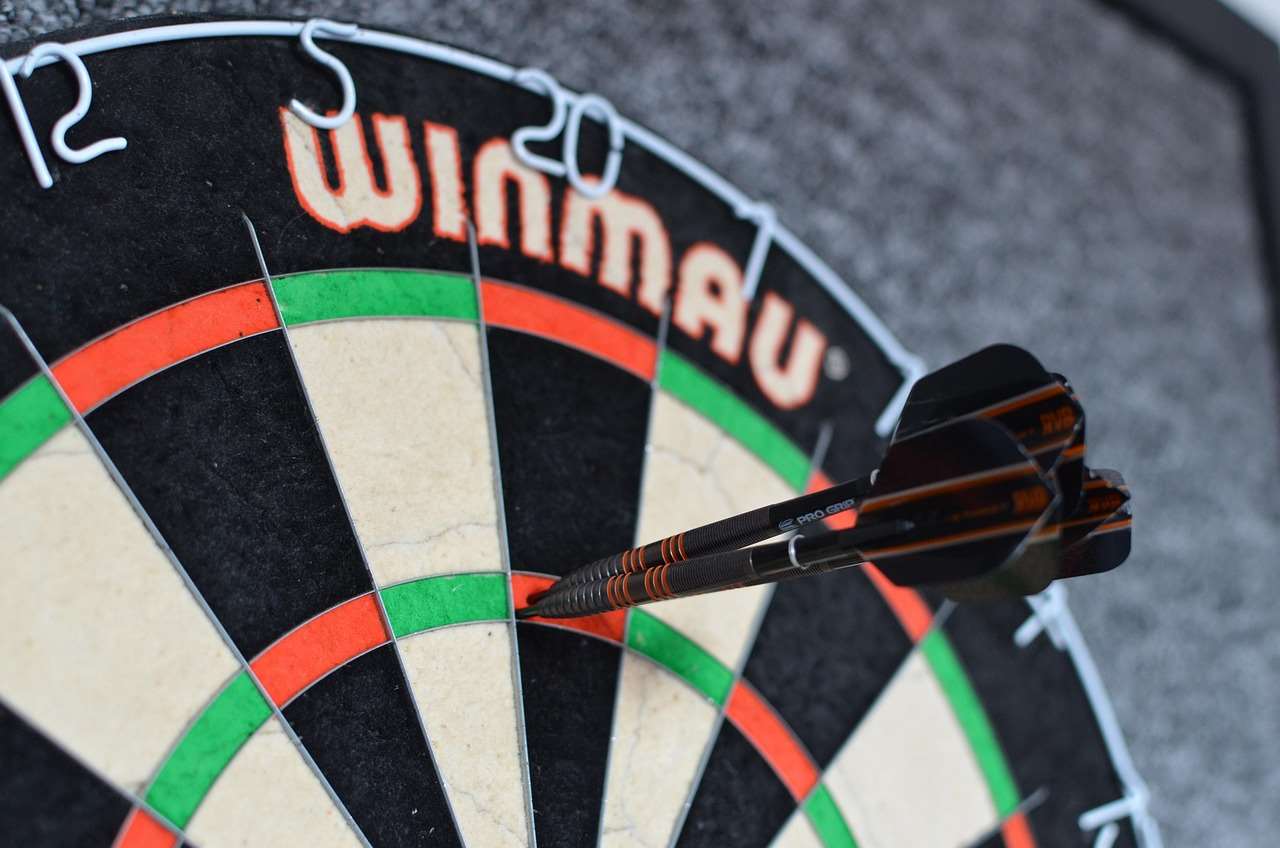
Integrating with External Libraries
For more advanced string manipulation tasks, especially those involving internationalization or complex text processing, you might consider integrating external libraries. Dart’s package ecosystem provides a rich collection of libraries designed for diverse string operations. For example, you could explore libraries that provide advanced Unicode support or regular expression-based text processing. These libraries offer specialized functionalities beyond the core Dart capabilities.
Performance Considerations
While the length
property is highly optimized, for extremely large strings, repeated calls to length
might have a small performance impact. For scenarios involving high-volume string processing, it’s advisable to cache the length value if it’s accessed repeatedly within a loop or computationally intensive operation. This caching strategy can enhance the overall efficiency of your code.
Choosing the Right Approach
The best approach to dart count characters in string depends on the specific context of your application. For most basic scenarios, the length
property suffices. However, when dealing with Unicode characters, potentially null strings, or situations requiring advanced string manipulation, it’s essential to adopt a more robust and context-aware strategy, potentially leveraging external libraries or custom functions for enhanced functionality and error handling. Remember always to prioritize readability and maintainability alongside performance optimization.
For instance, if you are developing a darts kasterlee scoring app, efficiently counting characters might be crucial for displaying scores correctly. In contrast, a simple text-based game might not require such intricate character counting techniques.
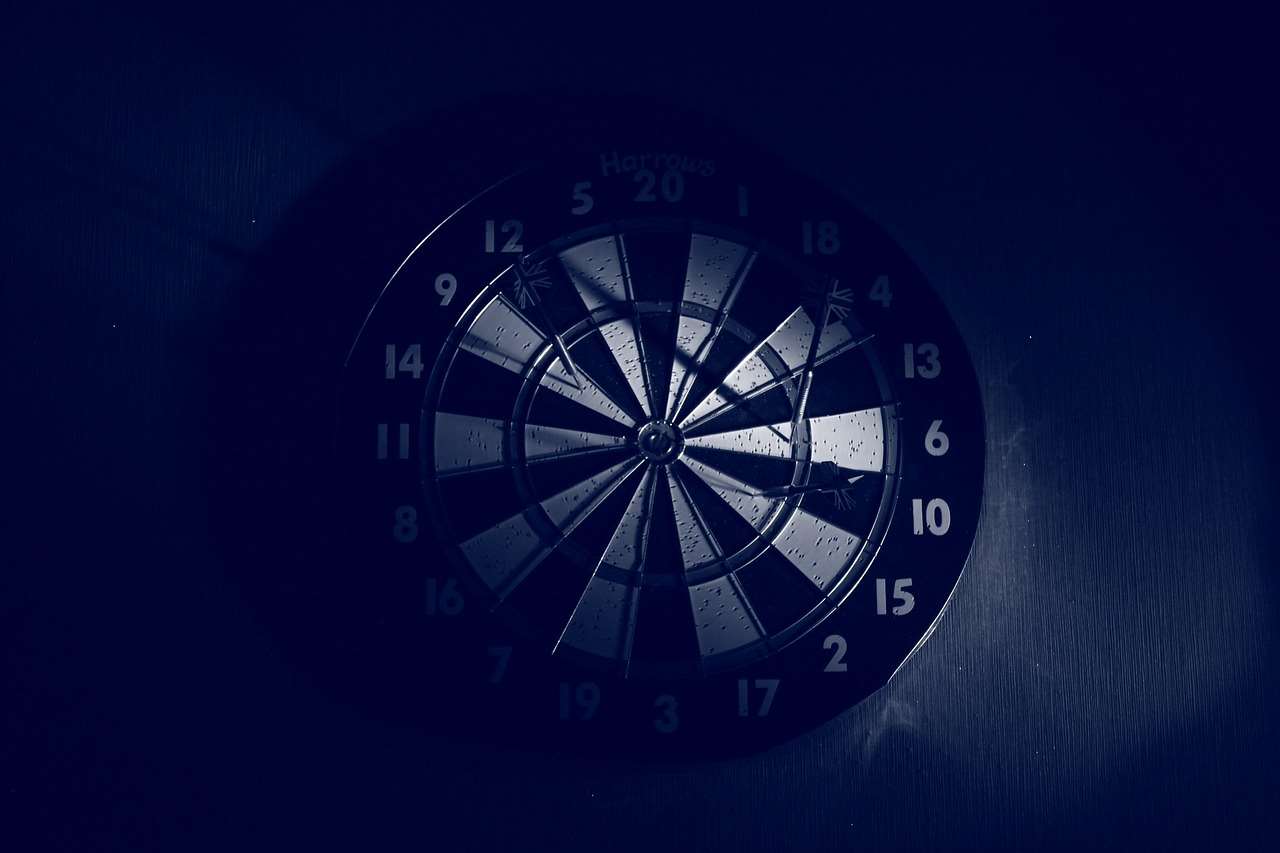
Further Exploration
This comprehensive guide has provided you with a thorough understanding of how to dart count characters in string in Dart. To further enhance your understanding, I recommend experimenting with different string manipulation techniques and exploring Dart’s rich ecosystem of libraries for more advanced operations. Remember that mastering string manipulation is crucial for developing robust and efficient Dart applications.
For example, consider how understanding character counting could improve your dart grip analysis software. Or perhaps, it could help in analyzing darts news latest articles for trending topics.
Additionally, consider utilizing the resources provided by Dart’s official documentation and community forums. These resources offer invaluable insights and support for addressing more complex scenarios. Furthermore, consider how this fundamental skill applies to broader software development principles and practices.
Understanding the nuances of string manipulation, including effectively counting characters, will significantly enhance your capabilities as a Dart developer, allowing you to build more sophisticated and user-friendly applications.
Remember to explore the capabilities of the darts treble calculation, which heavily relies on character manipulation and accurate counting.
Don’t forget to use this knowledge to build your next amazing project! Whether that involves building a comprehensive darts scoring application, creating a darts stand and light controller, or even designing an innovative target darts omni release date countdown timer, the ability to effectively count characters is a fundamental building block for success. In addition to these examples, consider using this knowledge to track player statistics in a darts winner luke littler-inspired game.
And don’t forget to check out the helpful Dart Counter App for more practical applications.
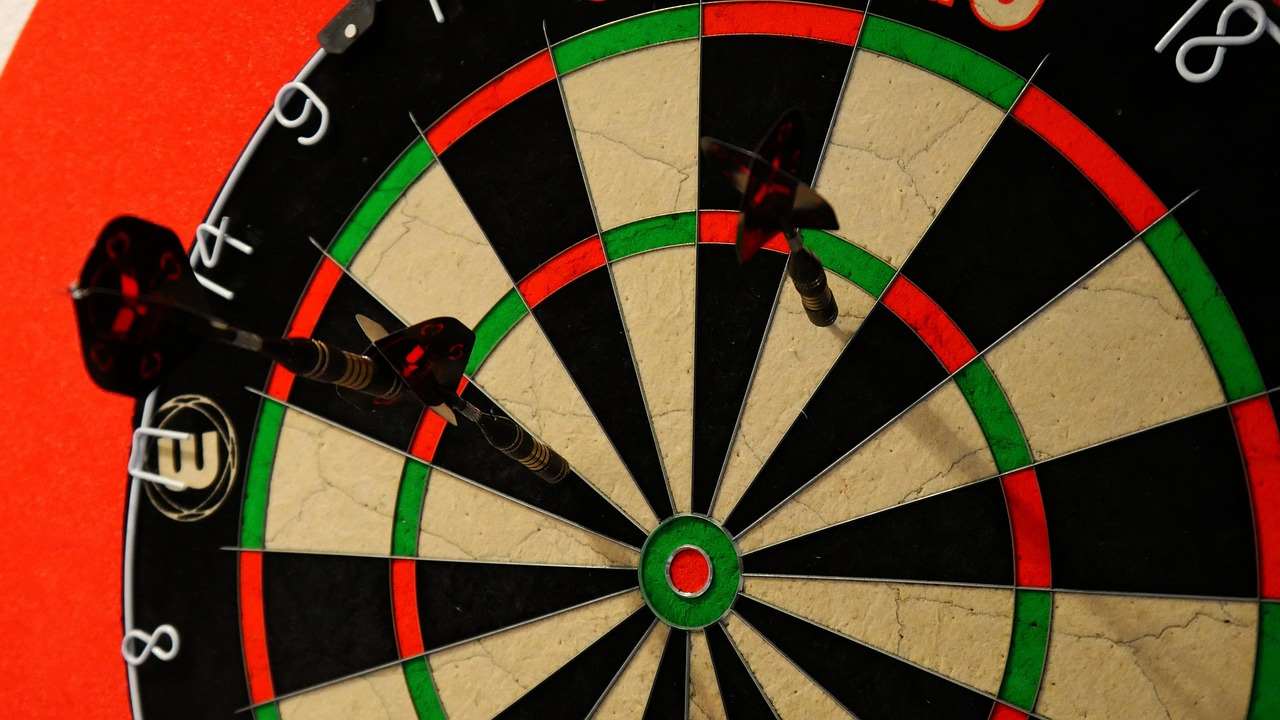
Conclusion
Effectively dart count characters in string is a vital skill for any Dart programmer. This article has provided you with a clear understanding of the basic approach using the length
property, as well as more advanced techniques for substring manipulation and error handling. We’ve also explored practical applications and considerations for performance optimization. By mastering these concepts, you can significantly improve the efficiency and robustness of your Dart applications. Now, go forth and build amazing things!
Hi, I’m Dieter, and I created Dartcounter (Dartcounterapp.com). My motivation wasn’t being a darts expert – quite the opposite! When I first started playing, I loved the game but found keeping accurate scores and tracking stats difficult and distracting.
I figured I couldn’t be the only one struggling with this. So, I decided to build a solution: an easy-to-use application that everyone, no matter their experience level, could use to manage scoring effortlessly.
My goal for Dartcounter was simple: let the app handle the numbers – the scoring, the averages, the stats, even checkout suggestions – so players could focus purely on their throw and enjoying the game. It began as a way to solve my own beginner’s problem, and I’m thrilled it has grown into a helpful tool for the wider darts community.